In PowerShell, you can sort objects by a specific column using the `Sort-Object` cmdlet, allowing you to organize data efficiently.
Here’s an example of sorting a list of users by their `LastName`:
Get-ADUser -Filter * | Sort-Object LastName
Understanding Objects in PowerShell
What Are Objects?
In PowerShell, everything is an object. This means that when you work with data, you interact with objects rather than plain text or numbers. Each object has properties and methods, allowing for flexible and powerful data manipulation. Understanding the object-oriented nature of PowerShell is essential for effectively using commands like Sort-Object, especially when sorting by column.
Commonly Used Objects for Sorting
The most common objects you’ll sort in PowerShell include arrays, custom objects, and outputs from various cmdlets, such as `Get-Process` or `Get-Service`. By sorting these objects based on their properties, you can organize data in a meaningful way that enhances your productivity and efficiency.
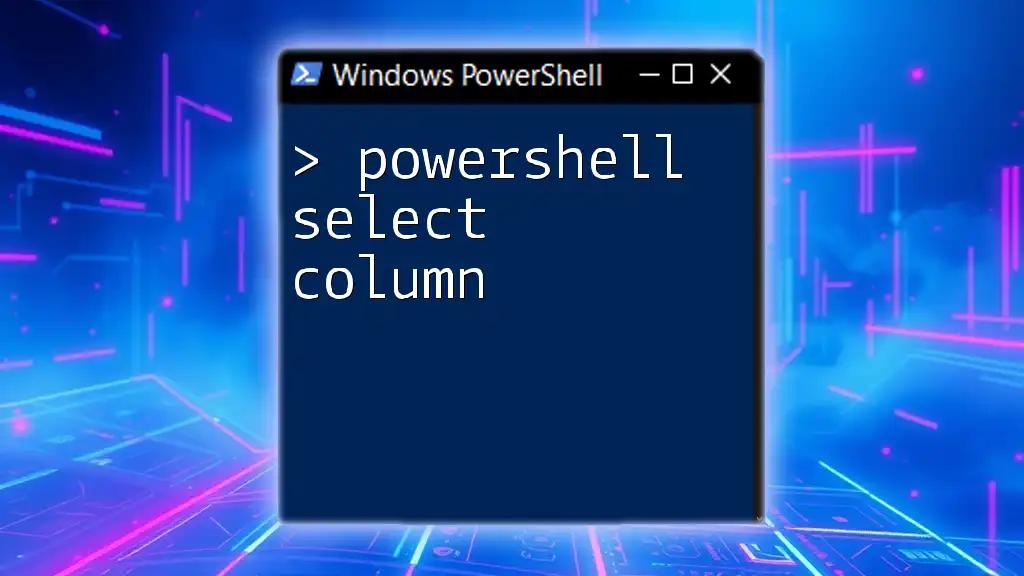
The Basics of Sorting
Using `Sort-Object` Cmdlet
The Sort-Object cmdlet is your primary tool for sorting data in PowerShell. The cmdlet can sort objects based on one or multiple properties and offers the ability to sort in ascending or descending order.
Syntax:
Sort-Object [-Property] <string[]> [-Descending] [-Unique]
This command gives you a lot of flexibility. When you provide the `-Property` parameter, you define which column (or property) you want to sort by.
Sorting Simple Data
Let's look at a straightforward example of sorting an array of strings. Suppose you have an array of fruits:
$fruits = "Banana", "Apple", "Orange"
$fruits | Sort-Object
When you execute this, you will receive an output where the fruits are sorted in alphabetical order. This basic sorting mechanism forms the basis for more complex data manipulation in PowerShell.
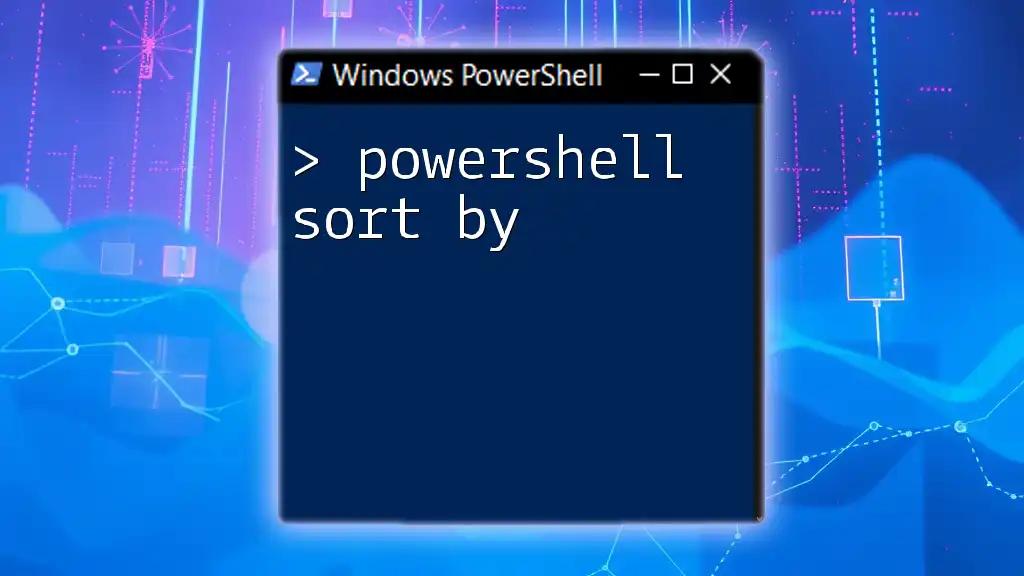
Sorting by Columns in Custom Objects
Creating Custom Objects
To leverage sorting effectively, it's often necessary to work with custom objects. You can create your own objects using `New-Object` or by leveraging `Select-Object` to define properties easily. Here is how you might create a collection of employee records:
$employees = @()
$employees += New-Object PSObject -Property @{Name="John Doe"; Age=30; Salary=60000}
$employees += New-Object PSObject -Property @{Name="Jane Smith"; Age=25; Salary=70000}
In this example, each employee object has three properties: Name, Age, and Salary.
Sorting Based on Multiple Properties
Sorting by multiple properties allows for more granular control over how data is organized. For instance, if you wanted to sort the employees first by age and then by salary, you would use:
$employees | Sort-Object -Property Age, Salary
This command sorts by age in ascending order, and for employees with the same age, it will then sort by salary. Understanding how to manipulate sorting through multiple properties is crucial for handling complex datasets.
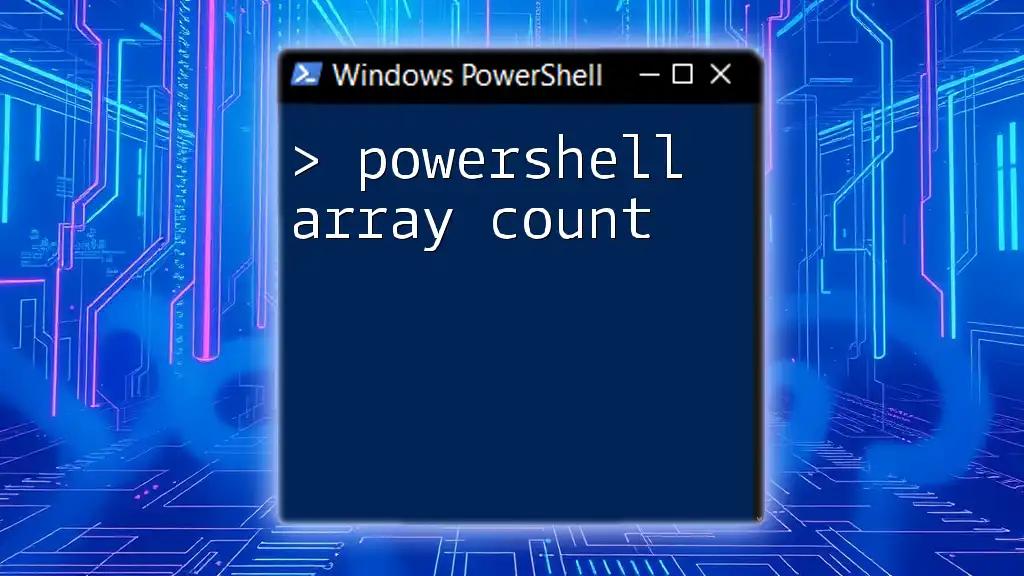
Advanced Sorting Techniques
Sorting in Descending Order
Sometimes, you may need to sort your data in descending order. This is easily achieved by using the `-Descending` flag with the `Sort-Object` cmdlet. For example, if you want to sort employees by their salary from highest to lowest:
$employees | Sort-Object -Property Salary -Descending
This command will give you the highest salary at the top of your sorted list, allowing you to quickly identify top earners in your dataset.
Unique Sorting
The `-Unique` flag allows you to filter out duplicate entries in your sorted list. For example, if you have an array with repeated values:
$values = 1, 2, 2, 3, 4, 5, 5
$values | Sort-Object -Unique
This will output a sorted array without any duplicates, simplifying your dataset and helping focus on distinct values.
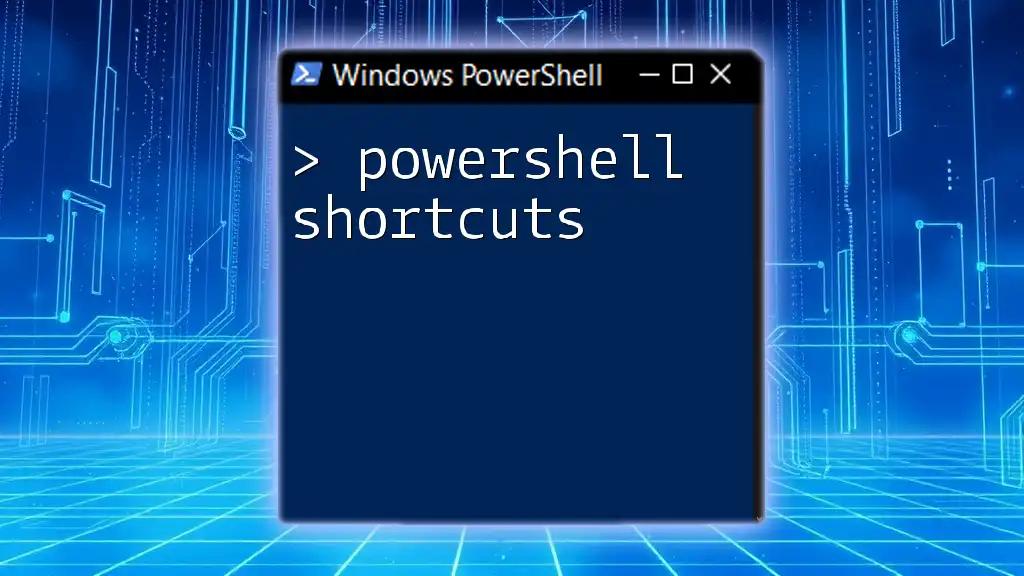
Sorting Data from Cmdlets
Sorting Process Output
PowerShell commands often output collections of objects that you might want to sort. For example, you can retrieve a list of running processes and sort them by CPU usage:
Get-Process | Sort-Object -Property CPU -Descending
This command gives you insight into which applications are consuming the most CPU resources, enabling you to manage system performance effectively.
Combining Sorting with Filtering
Integrating sorting with filtering can lead to more precise results. For example, suppose you want to list only running services sorted by their names:
Get-Service | Where-Object { $_.Status -eq 'Running' } | Sort-Object -Property Name
In this example, `Where-Object` filters the output to show only services that are running, and then `Sort-Object` organizes them in alphabetical order by their names. This combination is hugely beneficial when dealing with large numbers of objects.
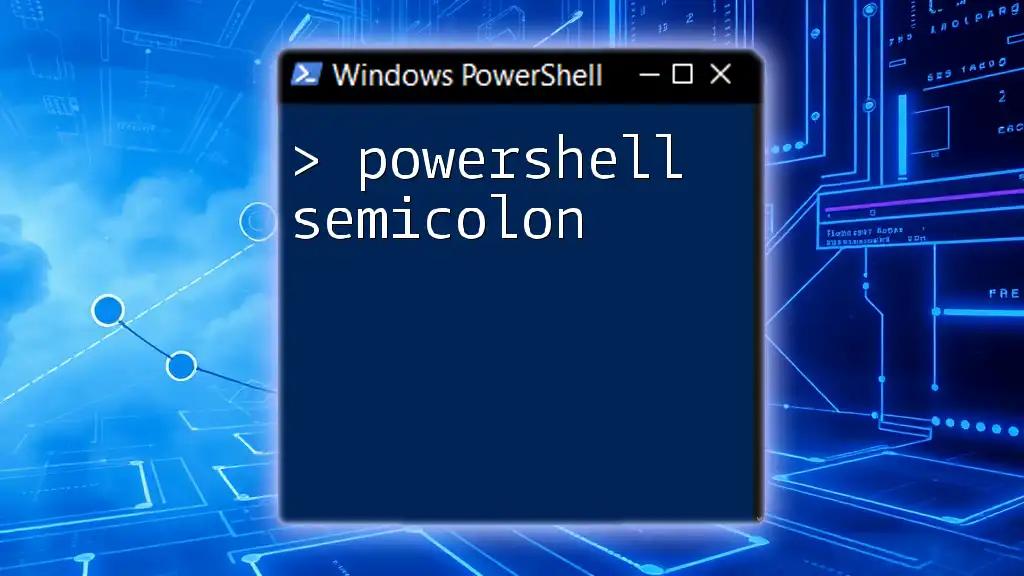
Using Sort in Arrays and Collections
Sorting Arrays of Numbers
Sorting isn’t limited to strings or objects. You can sort numerical arrays as well. Here’s how:
$numbers = 5, 3, 9, 1
$numbers | Sort-Object
This command will sort the numbers in ascending order, demonstrating the versatility of the `Sort-Object` cmdlet.
Sorting Nested Collections
Sorting nested collections involves a bit more complexity. Suppose you have an array of records where each record can contain multiple values. You can sort by a specific attribute of nested objects:
$records = @(
@{Name='Alice'; Courses='Math, Science'},
@{Name='Bob'; Courses='History, Arts'},
@{Name='Charlie'; Courses='Math, Arts'}
)
$records | Sort-Object { $_.Courses.Count }
In this case, you sort the records based on the number of courses each person has taken, showcasing the adaptability of sorting in more complex scenarios.
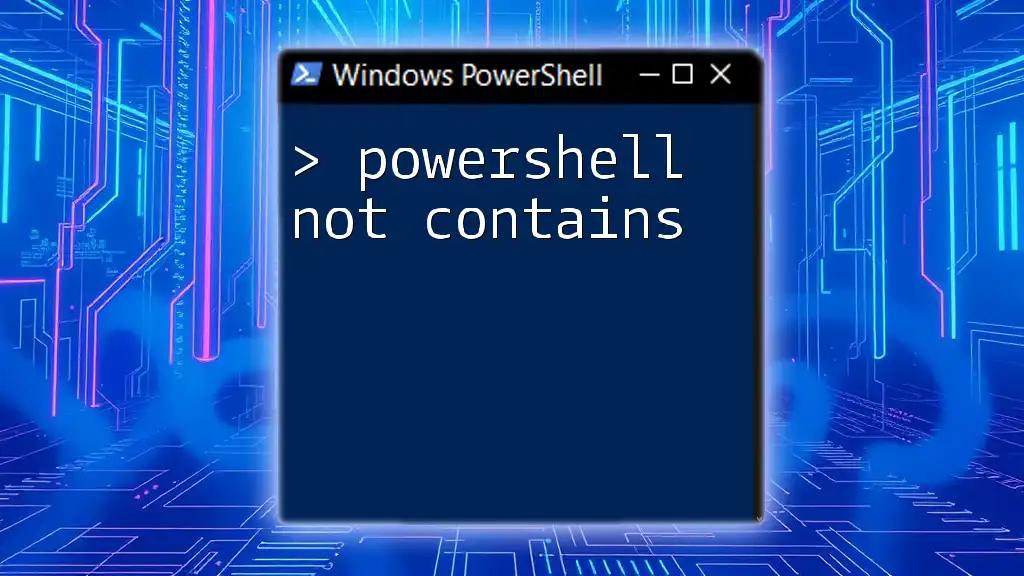
Best Practices for Sorting in PowerShell
Using Descriptive Property Names
When creating custom objects or handling data, always strive for descriptive property names. This practice makes it easier for you to sort and manipulate your data, particularly when you need to remember exactly what each property represents.
Performance Considerations
Sorting large datasets can have performance implications, especially if your sorting criteria require significant computation. Be mindful of this when designing your scripts. Try to limit the data size or use efficient filtering before sorting to optimize performance.
Consistent Formatting
To prevent sorting issues, ensure that the data you’re working with is consistently formatted. For example, if you attempt to sort strings mixed with numbers, it may yield unexpected results. Regularly validate the data types of the properties you sort.
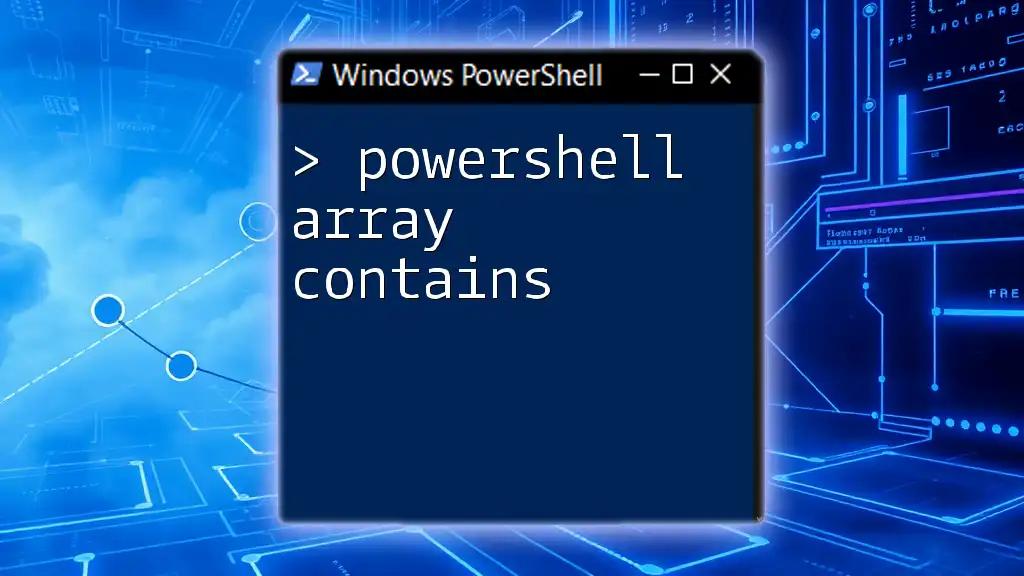
Troubleshooting Sorting Issues
Common Pitfalls
Some common mistakes include trying to sort incompatible data types. For example, sorting strings alongside numbers can lead to errors or unexpected ordering. Make sure to verify the properties you are sorting by and their corresponding data types.
Debugging Tips
Using `Get-Member` is an invaluable way to explore properties of objects you are working with prior to sorting. For example:
Get-Process | Get-Member
This command can help you confirm which properties are available for sorting, reducing the chances of errors.
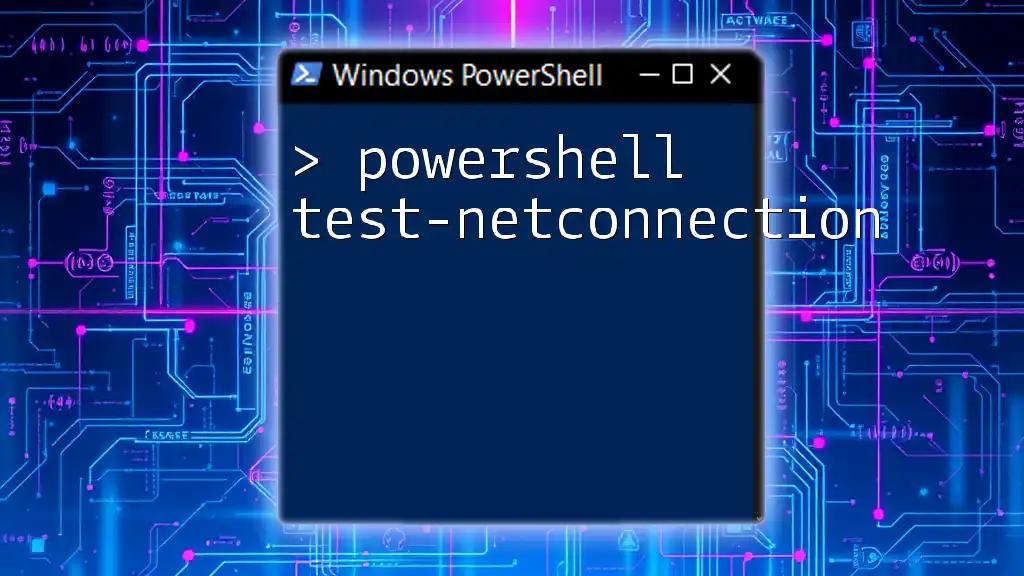
Conclusion
Sorting data in PowerShell using the `Sort-Object` cmdlet is a powerful way to organize and manage your datasets. By understanding how to work with objects, sort by properties, and troubleshoot common issues, you can enhance your scripting capabilities significantly.
It’s important to practice these techniques frequently to become proficient. As you grow familiar with the methods discussed, you’ll find that sorting becomes an instinctive part of your PowerShell skill set.