The `Do While` loop in PowerShell allows you to execute a block of code repeatedly as long as a specified condition remains true.
Here’s a simple example:
$counter = 0
do {
Write-Host "Counter is $counter"
$counter++
} while ($counter -lt 5
This snippet will output the value of `Counter` from 0 to 4 before exiting the loop.
Understanding the Do While Loop in PowerShell
Definition of Do While Loop PowerShell
In PowerShell, the do while loop is a fundamental programming construct that allows code to be executed repeatedly based on a specified condition. Unlike the standard while loop, which evaluates the condition before executing the loop body, the do while loop guarantees that the body is executed at least once, as it checks the condition after executing the block of code. This unique characteristic makes it particularly useful for cases where you want to ensure that a certain task occurs before verifying its continuation.
Syntax of Do While Loop Powershell
The syntax of a do while loop in PowerShell is quite straightforward. Here’s the general structure:
do {
# Code to execute
} while (condition)
- The `do` block contains the code that will run.
- The `while` condition determines whether the loop should continue executing after the code block completes.
How Do While Powershell Works
Execution Flow of a Do While Loop
The flow of execution for the do while loop can be summarized in the following steps:
- Execute the Code Block: The code inside the do block is executed first.
- Evaluate the Condition: After executing the code, PowerShell evaluates the condition specified in the while statement.
- Repeat if Necessary: If the condition evaluates to true, the loop runs again; if false, the loop exits.
This structure allows for scenarios where the initial actions must take place before deciding whether to continue the loop.
Example of a Basic Do While Loop
Here’s a simple example that demonstrates how a do while loop works:
$count = 0
do {
$count++
Write-Host "Current count is $count"
} while ($count -lt 5)
In this example, the loop executes the code that increments the `$count` by 1 and prints its value. The loop will keep executing until `$count` is no longer less than 5, resulting in the following output:
Current count is 1
Current count is 2
Current count is 3
Current count is 4
Current count is 5

Practical Applications of PowerShell Do While
Scenarios to Use the Do While Loop
The do while loop in PowerShell is particularly effective in situations where an action must occur at least once, regardless of the conditions that may follow. For instance, it can be helpful when:
- Validating user input: Ensuring that a user provides acceptable data before proceeding.
- Running a command repeatedly, such as until a specific state is achieved, like waiting for a service to start.
Example 1: User Input Validation
A common use case for the do while loop is user input validation. Here's an example where we ensure that a user enters a number greater than 10:
do {
$input = Read-Host "Enter a number greater than 10"
} while ($input -le 10)
In this scenario, the code repeatedly prompts the user until they provide an appropriate input, ensuring data quality before moving forward.
Example 2: Continuously Running a Command Until Condition is Met
Another practical application is continuously running a command until a condition is satisfied. The following example increments a counter and prints its value until it reaches a predetermined limit:
$count = 0
do {
$count++
Write-Host "Current count is $count"
} while ($count -lt 5)
Just like the previous example, this will print numbers from 1 to 5, demonstrating how tasks can be conducted in a loop until a certain threshold is reached.
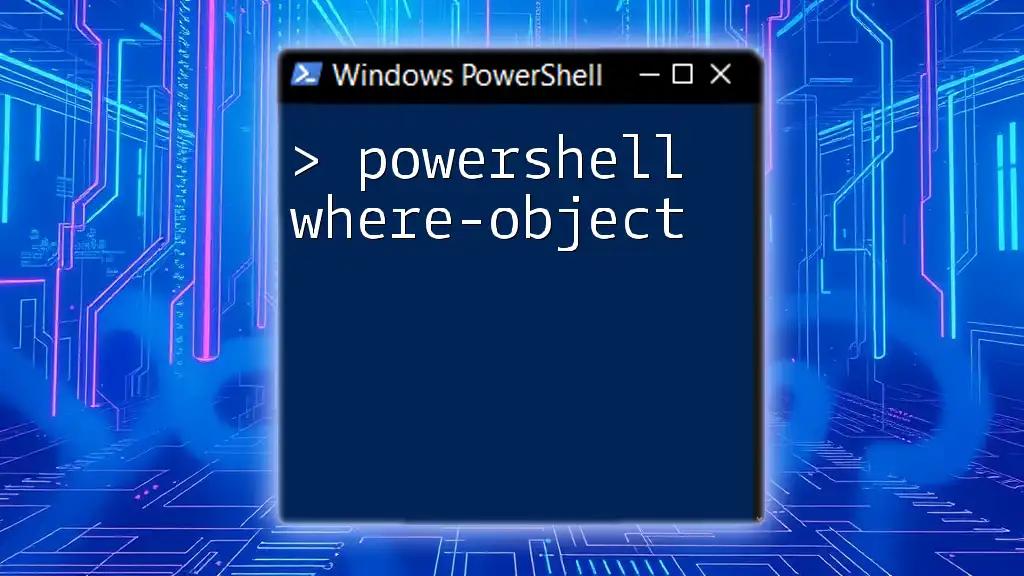
Best Practices for Using Do While PowerShell
Keep Loops Efficient
When using do while loops, strive to write efficient code. Consider how many iterations the loop may require and what operations are being performed during each iteration. Ensure that any commands within the loop are optimized to prevent unnecessary strain on system resources.
Avoid Infinite Loops
A common pitfall with any looping structure, including the do while loop, is the risk of creating an infinite loop. This occurs when the exit condition is never met due to improper logic or escaped scenarios. Always ensure that the condition within the while statement will eventually evaluate to false to avoid unintended consequences.
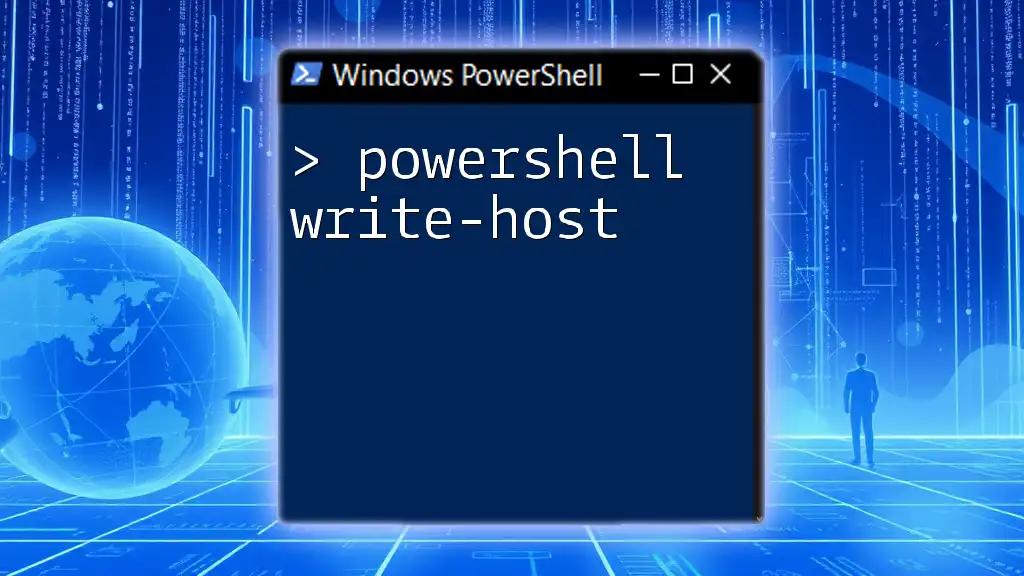
Common Issues and Troubleshooting
Debugging Do While Loops
If you encounter issues while executing a do while loop, several strategies can help identify and rectify problems:
- Check Your Logic: Review the conditions in the while statement to ensure they will eventually be met.
- PowerShell Debugger: Use built-in PowerShell debugging tools to step through your code line by line, allowing you to track variable states and loop iterations.
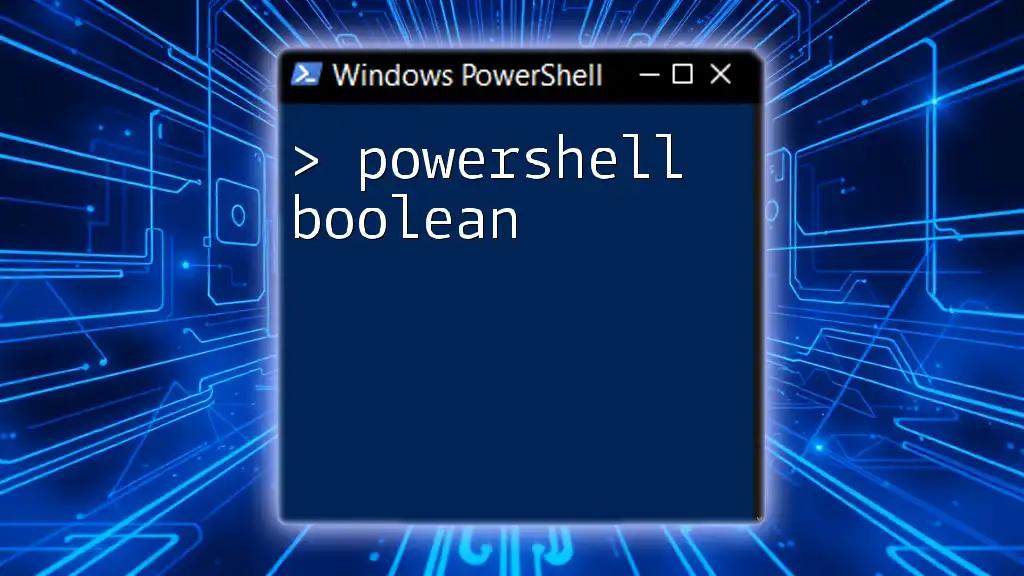
Conclusion
The PowerShell do while loop is a powerful tool that can streamline processes by enabling repetitive execution as long as a specific condition holds true. Understanding how to effectively use it enhances programming capabilities and increases efficiency in automation tasks.
Encourage experimentation with do while loops in various scenarios, as practical application solidifies learning. As you delve deeper into PowerShell, consider exploring additional resources and engage with online PowerShell communities to further expand your understanding and skills in scripting.