To upload a file to Dropbox using PowerShell, you can utilize the Dropbox API with the following code snippet to authenticate and perform the upload.
$filePath = "C:\path\to\your\file.txt"
$accessToken = "your_access_token"
$dropboxPath = "/file.txt"
Invoke-RestMethod -Uri "https://content.dropboxapi.com/2/files/upload" -Method Post -Headers @{ "Authorization" = "Bearer $accessToken"; "Dropbox-API-Arg" = "{`"path`": `"$dropboxPath`", `"mode`": `"`"add`"`, `"autorename`": true, `"mute`": false}"; "Content-Type" = "application/octet-stream" } -InFile $filePath
Understanding the Dropbox API
Overview of Dropbox API
The Dropbox API is a powerful tool that enables developers to interact programmatically with Dropbox services. It allows users to upload, download, and manage their files effortlessly through HTTP requests. Utilizing this API to upload files can streamline your workflow and enhance productivity, especially when working with large volumes of files.
Setting Up a Dropbox Account
Before diving into the API, having a Dropbox account is necessary. You can create an account by visiting the [Dropbox website](https://www.dropbox.com). Once you've entered your email and verified your account, you'll be ready to create your first application.
Creating a Dropbox App
To interface with Dropbox via the API, you’ll need to create a new app. Go to the [App Console](https://www.dropbox.com/developers/apps), where you’ll find the option to create a new app. Choose the level of access you want—Full Dropbox gives complete access, while App Folder restricts access to a designated area. After creating the app, you’ll be granted an access token essential for authentication.
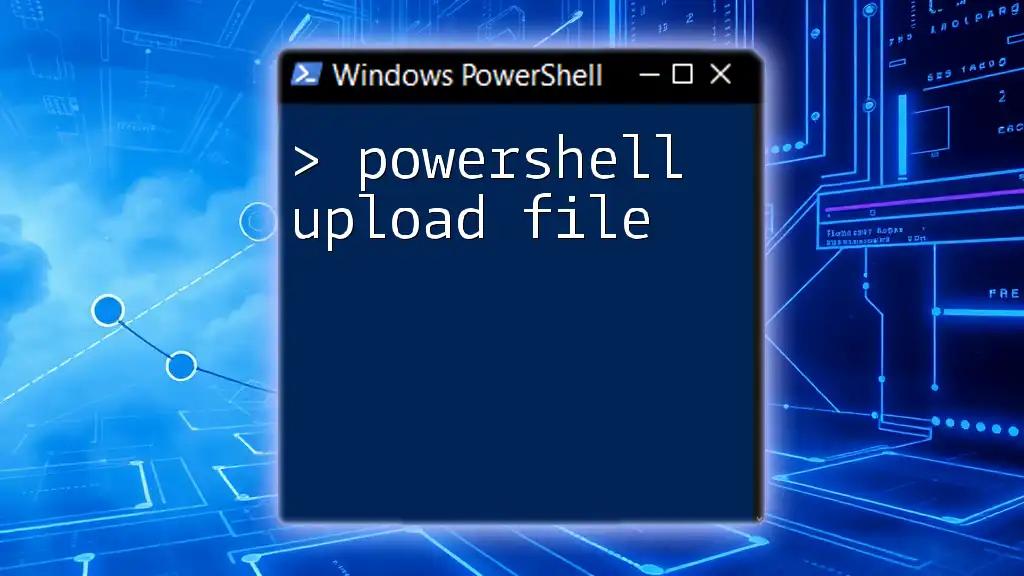
PowerShell Overview
What is PowerShell?
PowerShell is a versatile command-line tool and scripting language designed for task automation and configuration management. Its capabilities extend far beyond simple commands, making it an ideal choice for managing operations with cloud services like Dropbox.
Installing and Configuring PowerShell
If you don't already have PowerShell installed, you can download it from the official Microsoft site. On Windows, it usually comes pre-installed, but you can check for updates to ensure you’re working with the latest version. Certain modules, such as `Invoke-RestMethod`, are built-in and facilitate making web requests, which are crucial for interacting with APIs.
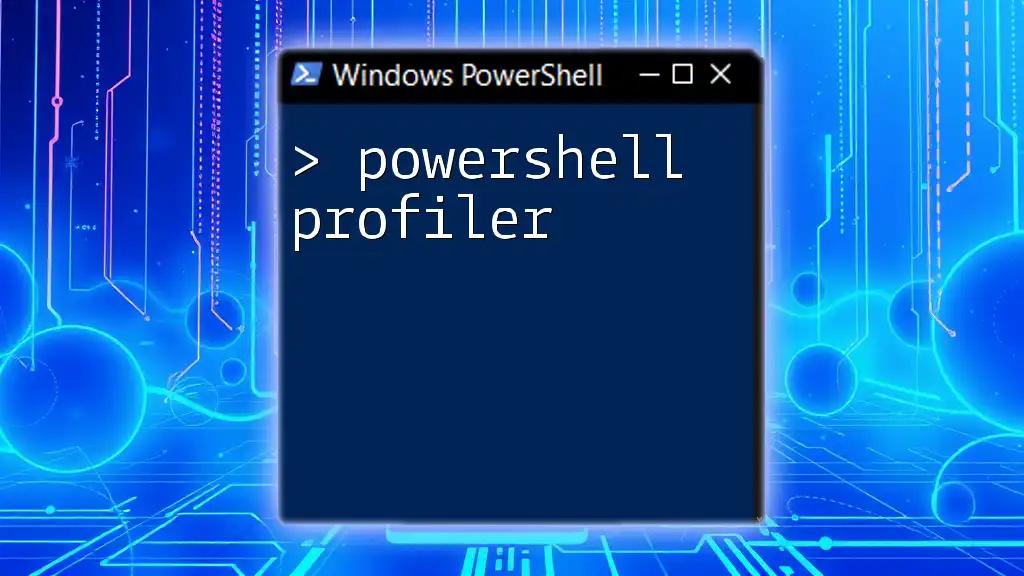
Connecting PowerShell to Dropbox
Installing Required PowerShell Modules
To start working with the Dropbox API in PowerShell, you don’t need any special modules beyond what's standard. Ensure that your PowerShell environment can run scripts by checking the `Execution Policy`. You can set it (if necessary) with the following command:
Set-ExecutionPolicy RemoteSigned
Authenticating with Dropbox
Authentication is crucial for achieving secure communications with Dropbox. You'll utilize your access token to authenticate your API requests. Store your access token securely, as it provides access to your Dropbox.
$accessToken = "your_access_token_here"
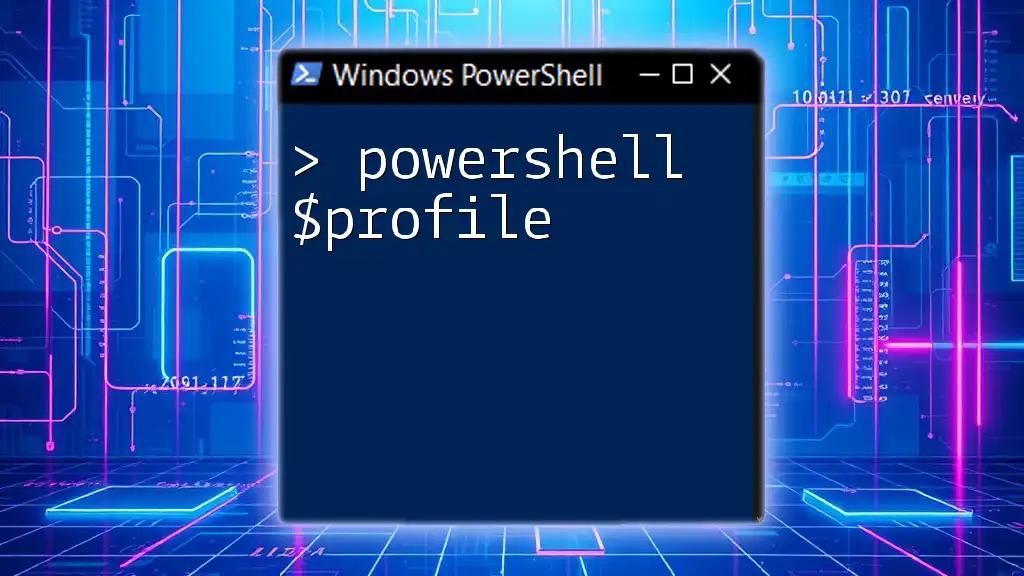
Uploading a File to Dropbox
Preparing Your File for Upload
Before uploading a file, confirm that its format is supported by Dropbox, which includes most common formats (e.g., text, images, documents). Choose the file you wish to upload by specifying its path correctly.
$filePath = "C:\path\to\your\file.txt"
Constructing the Upload Request
When uploading a file, you need to construct the appropriate HTTP request. Dropbox has a specific endpoint dedicated to file uploads. The API URL you’ll need is as follows:
$dropboxApiUrl = "https://content.dropboxapi.com/2/files/upload"
Executing the File Upload
With your file prepared and the request URL constructed, you can now execute the upload command in PowerShell. The essential headers for the request include your Authorization token and the Dropbox-API-Arg containing specific parameters, such as the file path.
$headers = @{
"Authorization" = "Bearer $accessToken"
"Dropbox-API-Arg" = '{"path":"/file.txt","mode":"add"}'
"Content-Type" = "application/octet-stream"
}
Invoke-RestMethod -Uri $dropboxApiUrl -Method Post -Headers $headers -InFile $filePath -ContentType "application/octet-stream"
In this snippet, the upload request is executed using `Invoke-RestMethod`, which sends the file to the specified Dropbox folder.
Error Handling
During the upload process, various issues may arise, from path errors to authentication problems. It’s crucial to implement error handling to alert you if something goes wrong. In PowerShell, you can use `try-catch` blocks:
try {
Invoke-RestMethod -Uri $dropboxApiUrl -Method Post -Headers $headers -InFile $filePath -ContentType "application/octet-stream"
}
catch {
Write-Host "An error occurred: $_"
}
By using this structure, you can capture and display any errors that occur during the upload process, allowing for easier troubleshooting.
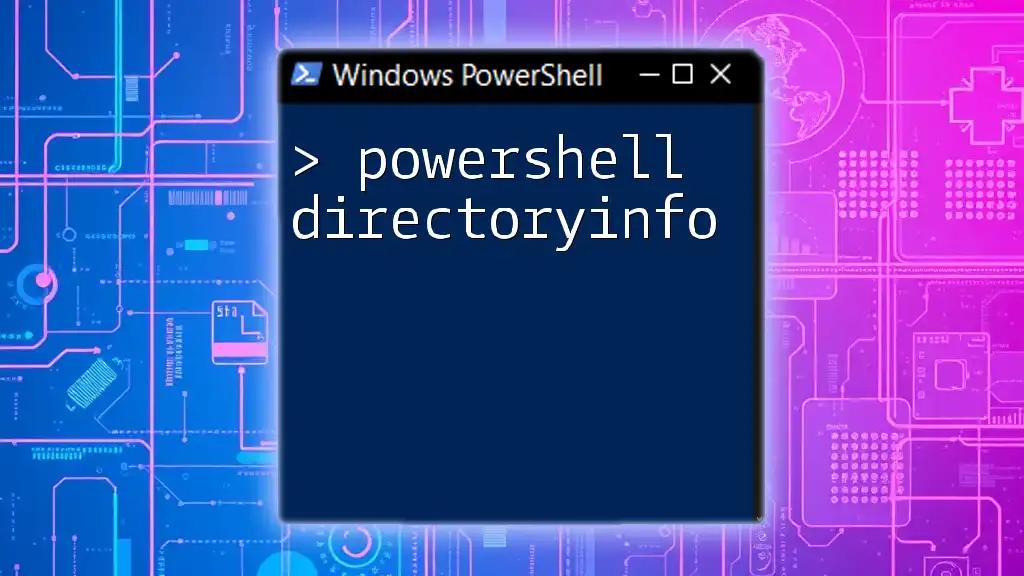
Verifying the Upload
How to Check Uploaded Files in Dropbox
After a successful upload, it’s advisable to verify that your file appears in Dropbox. You can log in to your Dropbox account and navigate to the folder where you uploaded the file.
Example Code for Listing Files
If you prefer to programmatically verify the upload, you can utilize the API to list the files in your Dropbox. Use the following endpoint for listing files:
$listFilesUrl = "https://api.dropboxapi.com/2/files/list_folder"
Here’s a snippet to list the files in your Dropbox folder:
$headers = @{
"Authorization" = "Bearer $accessToken"
"Content-Type" = "application/json"
}
$result = Invoke-RestMethod -Uri $listFilesUrl -Method Post -Headers $headers -Body '{"path":""}'
$result.entries | ForEach-Object { $_.name }
This will iterate through the contents of your Dropbox and print out the names of the files, making it easy to confirm your upload.
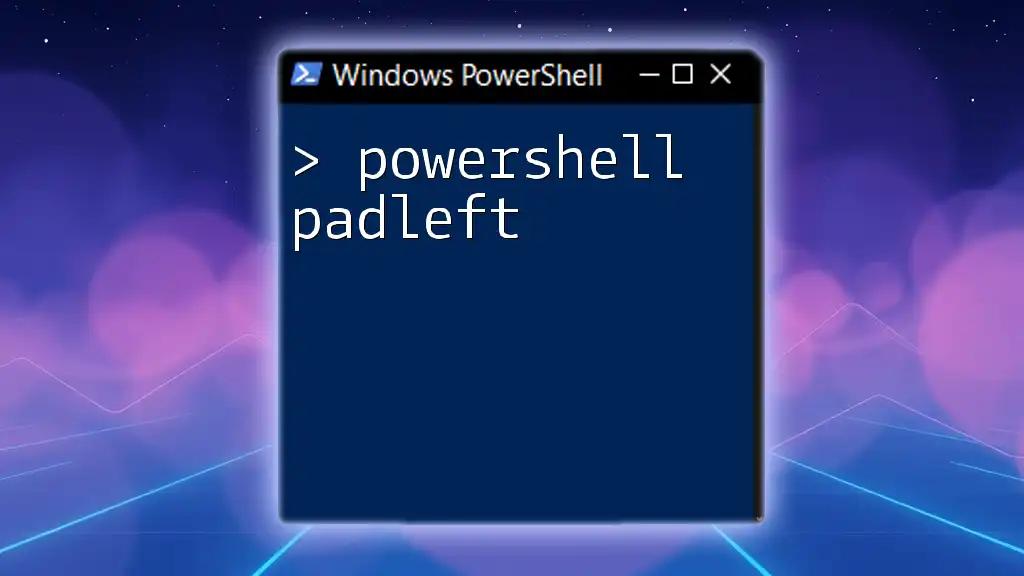
Conclusion
In this guide, we explored how to use PowerShell to upload files to Dropbox, leveraging the Dropbox API for automation. From creating an API app and generating access tokens to executing the upload commands and verifying the uploads, each step is essential for mastering file management in the cloud. Now that you are familiar with these processes, you’re encouraged to delve deeper into the API to unlock even greater functionality. Don't hesitate to share your experiences or ask questions as you embark on your journey with PowerShell and Dropbox!

Additional Resources
Further Reading and Tutorials
For those eager to learn more about PowerShell and the Dropbox API, numerous resources are available online. Dive into the official [Dropbox API documentation](https://www.dropbox.com/developers/documentation) and explore community forums for additional support.
FAQs
If you have common questions regarding PowerShell and Dropbox interactions, consider checking community discussions or contributing to existing threads focused on troubleshooting and advanced usage tips.