Powershell Toast Notifications allow you to display brief, interactive alerts on a user's desktop, enhancing the interactivity of your scripts; here's a simple example of how to create one:
$ToastXml = @"
<toast>
<visual>
<binding template="ToastGeneric">
<text>Notification Title</text>
<text>Your message goes here!</text>
</binding>
</visual>
</toast>
"@
New-BurntToastNotification -ToastContent ([xml]$ToastXml)
What are Toast Notifications?
Definition and Purpose
Toast Notifications are brief messages that appear on a user's screen to communicate updates, alerts, or reminders without interrupting their workflow. They are designed to be unobtrusive, allowing users to continue their tasks while still receiving essential information. This is particularly useful for system notifications, reminders, and application messages.
History and Evolution
Toast Notifications first emerged in earlier Windows versions, providing a simple way to inform users about background processes and events. However, with the introduction of Windows 10 and its continued evolution into Windows 11, Toast Notifications have become more sophisticated, allowing for rich content, interactivity, and user engagement through various actions like buttons and images.
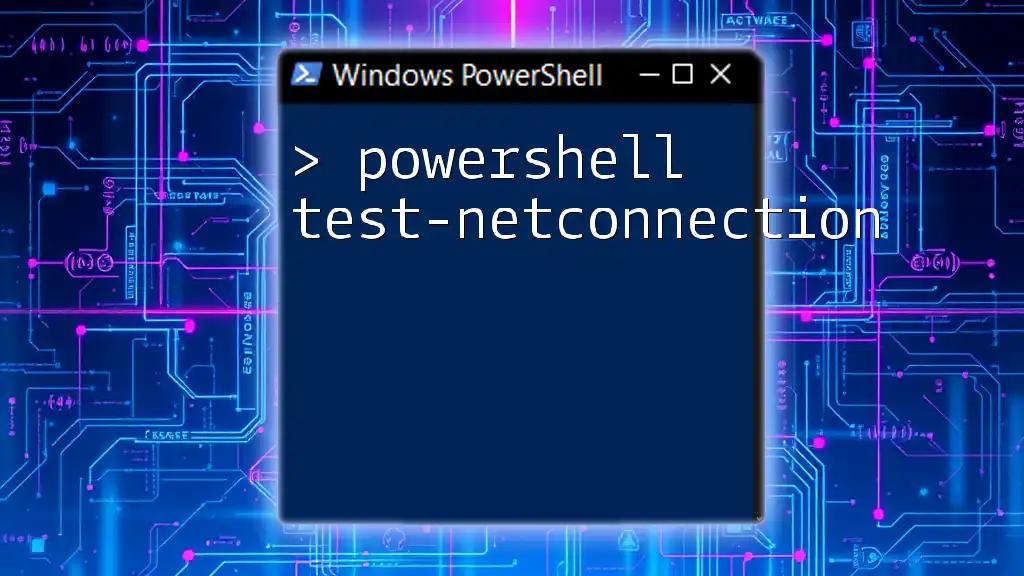
Setting Up PowerShell Environment
Installing PowerShell
Before diving into creating PowerShell Toast Notifications, you need to ensure that you have PowerShell installed on your system. To check the version of PowerShell you currently have, open PowerShell and run:
$PSVersionTable.PSVersion
If your version is outdated or you do not have it installed, you can download the latest version from the official [Microsoft PowerShell GitHub page](https://github.com/PowerShell/PowerShell).
Ensuring Necessary Permissions
When working with Toast Notifications, appropriate permissions are essential. You should run PowerShell as an administrator to ensure that you have the necessary privileges to create and send notifications. Right-click on the PowerShell icon and select "Run as Administrator."
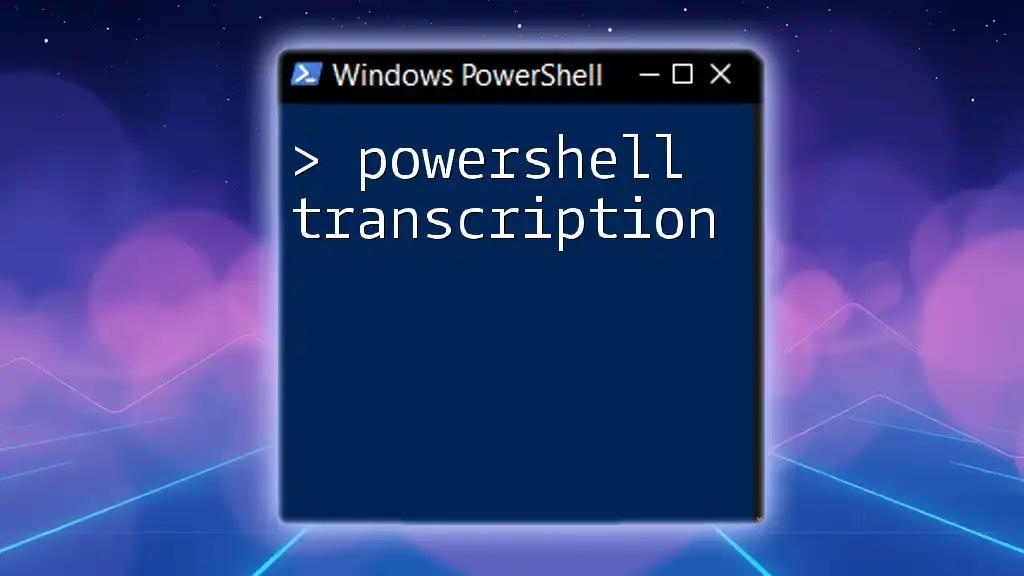
Creating Your First Toast Notification in PowerShell
Basic Syntax
To create a simple Toast Notification in PowerShell, you can use the `New-BurntToastNotification` cmdlet, which is part of a community module called BurntToast. To create a notification that simply says "Hello World," you would use the following command:
New-BurntToastNotification -Text "Hello World"
Code Snippet Breakdown
- `New-BurntToastNotification`: This cmdlet is essential for sending notifications in PowerShell. It creates a new Toast Notification based on the parameters you specify.
- `-Text`: This parameter accepts strings that define the title and content of the notification. In this case, using "Hello World" will create a notification that appears to the user.
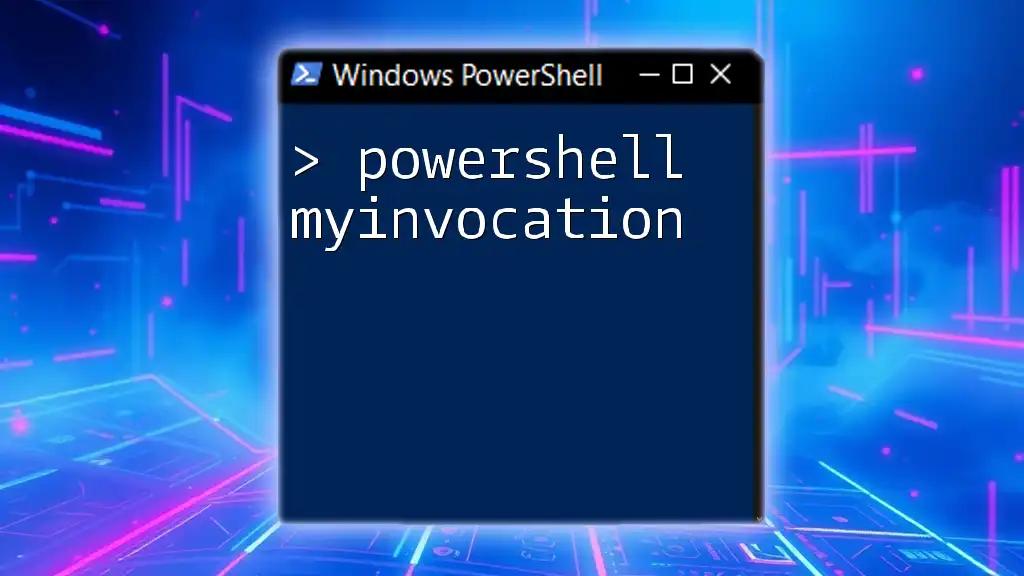
Advanced Toast Notification Options
Customizing Notifications
You can enhance the appearance and utility of your Toast Notifications by adding images and modifying colors. To include an image, such as an app logo, use the following command:
New-BurntToastNotification -Text "Notification Title", "Your message here" -AppLogo "C:\path\to\image.png"
In this example, the `-AppLogo` parameter allows you to specify an image that complements your message, making your notifications more visually appealing.
Adding Buttons to Toast Notifications
Toast Notifications can also be interactive. You can add buttons for users to respond to notifications. For instance, here’s how to create a notification that includes actionable buttons:
$ToastContent = @"
<toast>
<visual>
<binding template="ToastGeneric">
<text>Button Test</text>
<text>Click a button!</text>
</binding>
</visual>
<actions>
<action content="Open" arguments="https://github.com">Open GitHub</action>
<action content="Close" arguments="close">Close Notification</action>
</actions>
</toast>
"@
In this snippet, the `<actions>` section allows users to open a URL or close the notification directly, enhancing interactivity.
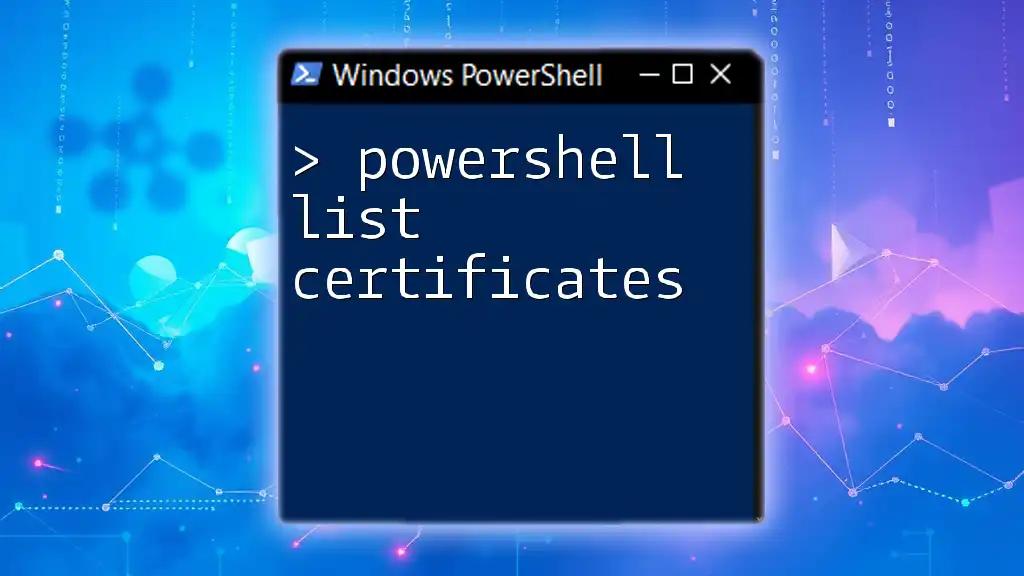
Using Variables in Notifications
Dynamically Changing Notifications
To make Toast Notifications more user-specific and dynamic, you can utilize variables. This is especially useful for tailoring messages based on user input or system status. For instance:
$userMessage = "This is your custom message!"
New-BurntToastNotification -Text "Dynamic Message", $userMessage
In this example, the variable `$userMessage` allows you to include customized text in your notification, making it more relevant to the user.
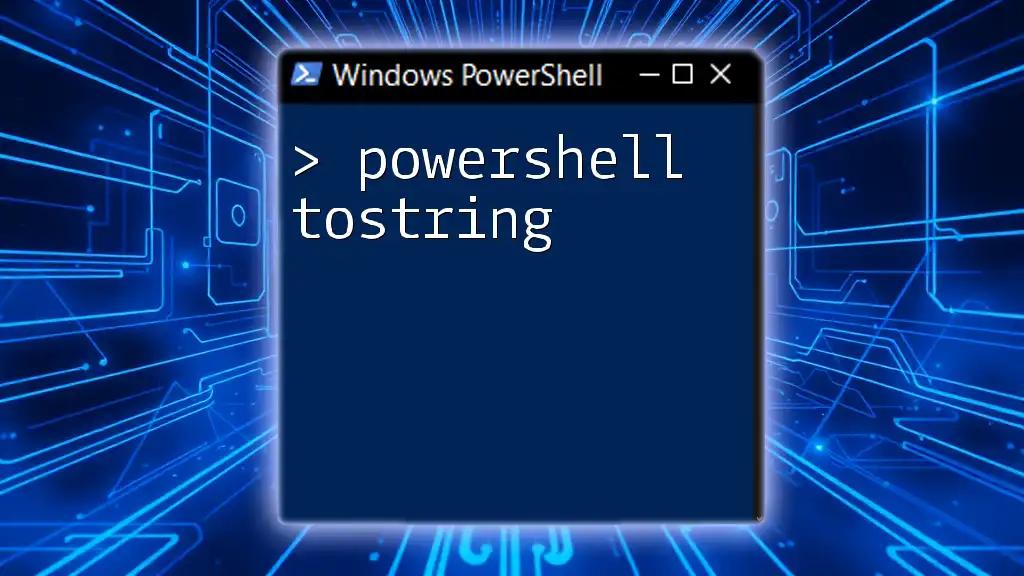
Troubleshooting Common Issues
Missing Cmdlets
If you encounter errors indicating that cmdlets are missing, it typically means that the BurntToast module is not installed. To install this module, run the following command:
Install-Module -Name BurntToast -Force
Remember to run PowerShell as an administrator while executing this command.
Notification Not Appearing
If your notifications are not displaying even after executing the correct commands, check the following:
- Ensure that you are running PowerShell in an environment that supports Toast Notifications (such as Windows 10 or 11).
- Confirm that notifications are enabled in your Windows settings. Go to Settings > System > Notifications & actions and ensure that notifications for your applications are turned on.
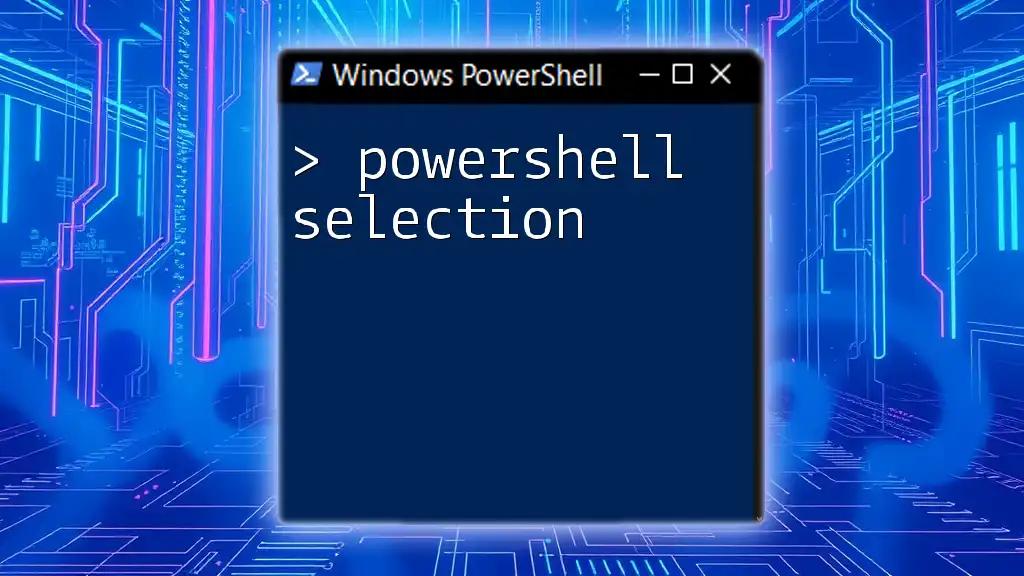
Practical Uses of PowerShell Toast Notifications
Real-World Applications
PowerShell Toast Notifications can serve various practical purposes that enhance user productivity and engagement. Some scenarios include:
- System Monitoring Alerts: Setting up notifications for system performance alerts such as high CPU usage or disk space warnings.
- Scheduled Task Reminders: Automating reminders for scheduled tasks or system maintenance activities.
- Real-Time Updates for Applications: Sending notifications for application updates, backup completions, or critical errors.
Engaging users through timely notifications can significantly improve workflows and keep them informed about essential events in real-time.
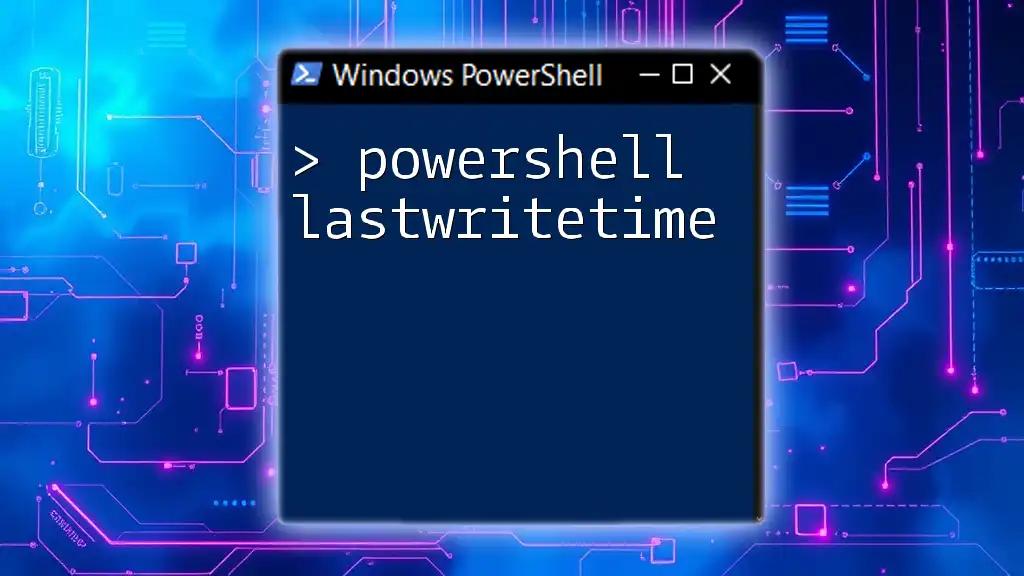
Conclusion
In summary, Toast Notifications in PowerShell provide a powerful way to engage users with timely updates and interactive alerts. By leveraging the capabilities of the `New-BurntToastNotification` cmdlet and exploring various customization options, you can enhance your workflow and keep users informed without being intrusive. Experimenting with these notifications in your daily tasks can uncover unique ways to streamline processes and improve productivity.
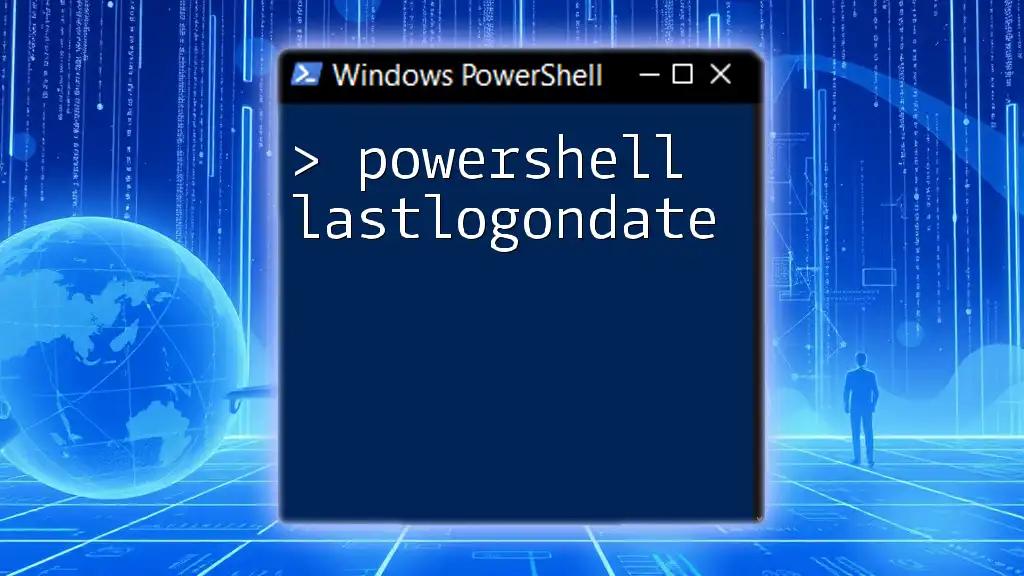
Additional Resources
Recommended Modules
For further exploration, consider checking out the BurntToast module documentation for advanced features. It is a rich resource for learning more about Toast Notifications.
Further Reading
To deepen your knowledge, explore additional articles and blogs focused on PowerShell scripting, automation, and user interface design for notifications. These will provide more insights into how to utilize PowerShell in enhancing user engagement through notifications.