The `tail -f` command in PowerShell allows you to continuously monitor and display the last few lines of a file, updating in real-time as new content is added.
Here’s a code snippet to demonstrate its usage:
Get-Content "C:\path\to\your\file.log" -Wait -Tail 10
Understanding the Basics of `tail -f`
Definition and Purpose
`tail -f` is a command commonly used in Unix-like operating systems to monitor the end of a file in real-time. In the context of PowerShell, this functionality can be achieved using the `Get-Content` cmdlet with the `-Wait` parameter. This command is particularly useful for tracking log files as they are updated, allowing users to see new entries immediately as they are written.
Common Scenarios for Using `tail -f`
You might find the need for real-time log monitoring in several scenarios, including:
- Monitoring Application Logs: When running an application, developers often need to view logs for errors or operational insights.
- Checking System Event Logs: System administrators may want to keep track of system events as they occur to quickly respond to issues.
- Debugging Scripts or Services: If you are developing scripts or services, using `tail -f` can help identify problems as they happen during execution.
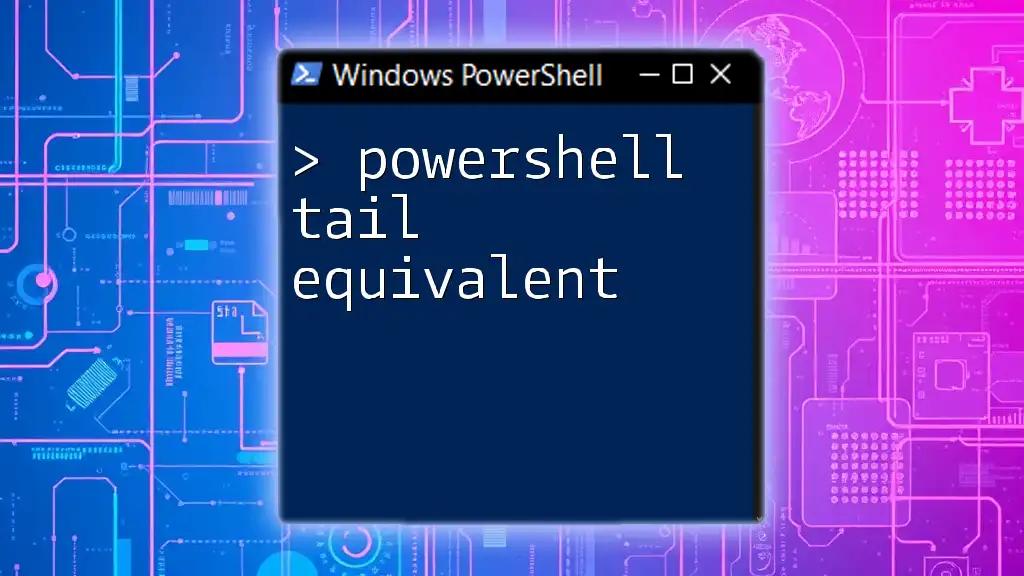
Getting Started with PowerShell
Setting Up Your PowerShell Environment
To begin, ensure you have PowerShell properly installed. PowerShell comes pre-installed on Windows, and recent versions are also available for macOS and Linux. Verify your version by typing the following command in your PowerShell window:
$PSVersionTable.PSVersion
This command will return the version of PowerShell you are using. For optimal experiences with `Get-Content`, ensure you are using PowerShell 5.1 or later.
Basic Command Syntax
The primary way to achieve the `tail -f` functionality in PowerShell is through the `Get-Content` cmdlet followed by the `-Wait` option. Here’s the basic command:
Get-Content "C:\path\to\your\logfile.log" -Wait
In this command:
- `Get-Content` retrieves content from the specified file.
- `-Wait` keeps the command running and displays new content as it is written to the file.
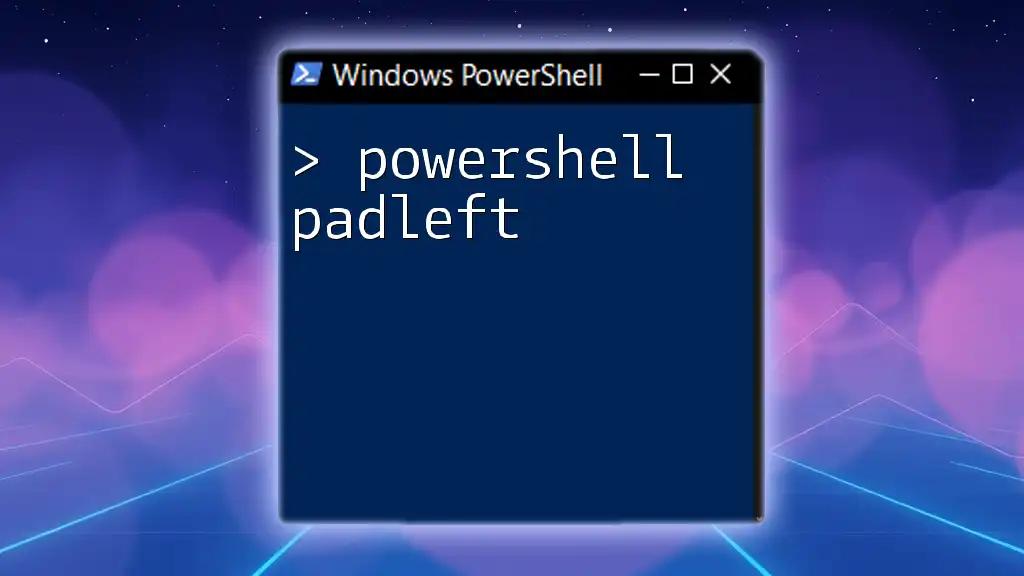
Utilizing `tail -f` Functionality
Monitoring Logs in Real-Time
To use `Get-Content` effectively, you may often want to see a specific number of recent lines and keep the command running. For example:
Get-Content "C:\path\to\logfile.log" -Tail 10 -Wait
This command pulls the last 10 lines from the log file and continues to monitor for new lines. This is incredibly useful in situations where you need to catch errors or notices right as they happen.
Understanding the Processes Behind `tail -f`
When you run `Get-Content` with the `-Wait` parameter, PowerShell maintains a buffered stream that continuously checks for new entries in the specified file. This ensures that you're always seeing the most up-to-date content without needing to rerun the command manually.
Customizing Output for Better Readability
Formatting Log Output
To enhance message visibility, you can format the log output. For instance, you might want to filter for specific error messages. Using `Select-String`, you can streamline this process:
Get-Content "C:\path\to\logfile.log" -Wait | Select-String "Error"
In this example, the command will monitor the log file and only display lines containing the word "Error," making it easier to locate problematic entries.
Highlighting Specific Entries
For added clarity, you can include color-coding to your output with ANSI color codes. Here’s a simple example of how to highlight error messages in red:
Get-Content "C:\path\to\logfile.log" -Wait | ForEach-Object {Write-Host $_ -ForegroundColor Red}
This command not only monitors the log file but also colors error messages, making them stand out immediately.
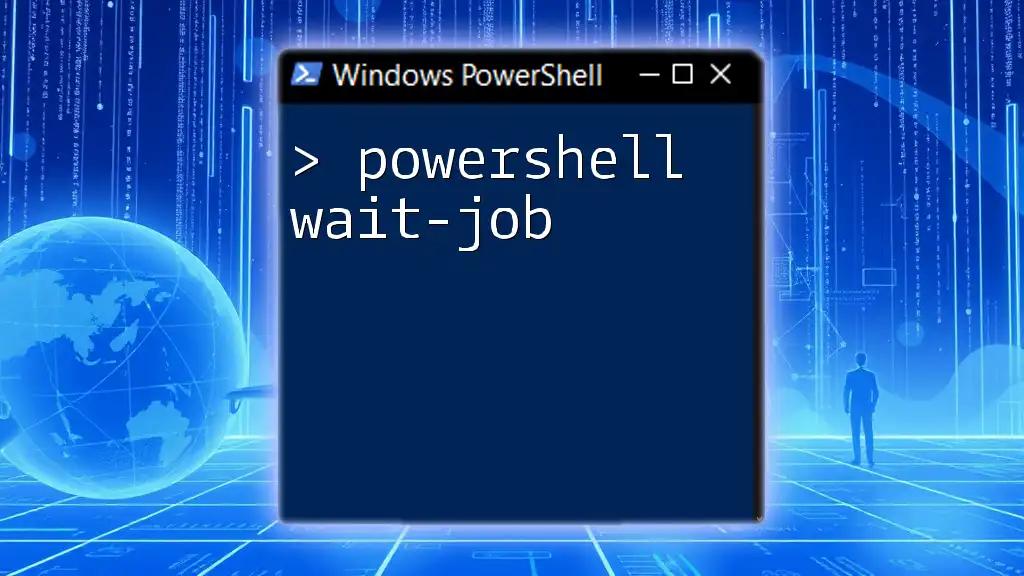
Advanced Usage of `tail -f` in PowerShell
Combining with Other PowerShell Cmdlets
The real power of `Get-Content` comes when you pipe its output to other cmdlets for enhanced data processing. For example, if you want to count how many times an error appears in your log file, you could use this command:
Get-Content "C:\path\to\logfile.log" -Wait | Where-Object {$_ -match "Error"} | Measure-Object
In this command:
- `Where-Object` filters the log entries for those matching the term "Error."
- `Measure-Object` counts how many such instances occur.
Creating a Custom Monitor Script
For continuous log monitoring, you can encapsulate the monitoring process in a script. The following snippet creates an infinite loop that checks the last 10 lines of the logfile at five-second intervals:
while ($true) {
Get-Content "C:\path\to\logfile.log" -Tail 10 -Wait
Start-Sleep -Seconds 5
}
This simple loop will keep the log file monitored, refreshing at regular intervals, which can be particularly useful during heavy debugging sessions.
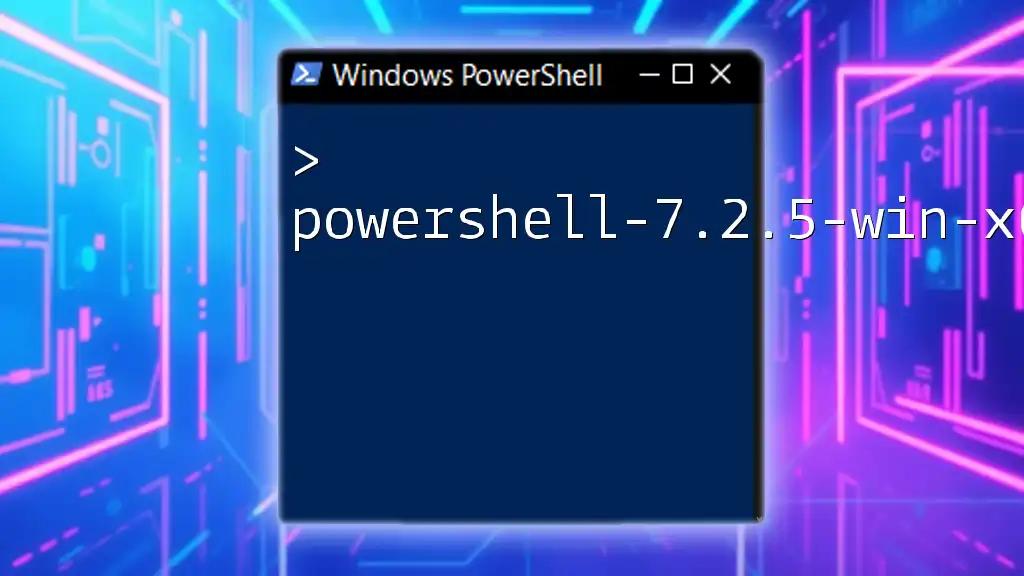
Common Issues and Troubleshooting
Common Pitfalls When Using `tail -f`
There are certain challenges users may encounter when utilizing PowerShell `tail -f` functions. Common issues include:
- File Permission Errors: Ensure that the user has the appropriate permissions to read the log file.
- Performance Concerns: Monitoring very large files in real-time could lead to performance slowdowns.
Tips for Effective Log Monitoring
To minimize issues and make log monitoring efficient:
- Reduce Noise: Use filtering options to limit the output to relevant log entries.
- Optimize Resource Usage: Regularly monitor your system performance while running intensive log monitoring commands to prevent system slowdowns.
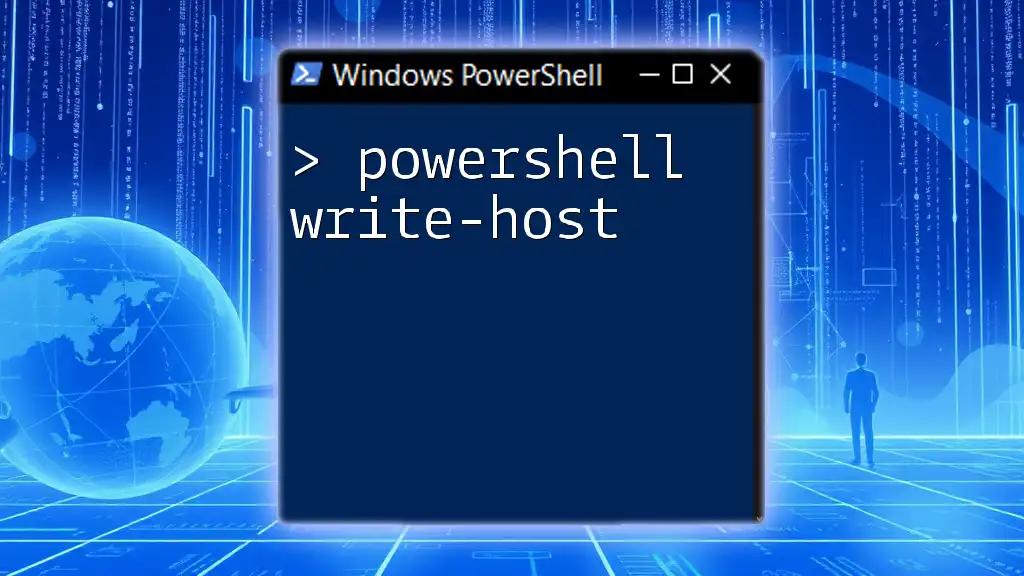
Conclusion
PowerShell `tail -f` functionality through `Get-Content` with parameters like `-Wait` provides an essential tool for system administrators, developers, and anyone needing real-time log monitoring. By exploring the capabilities discussed in this guide, you can maximize your efficiency in tracking and diagnosing issues as they happen.
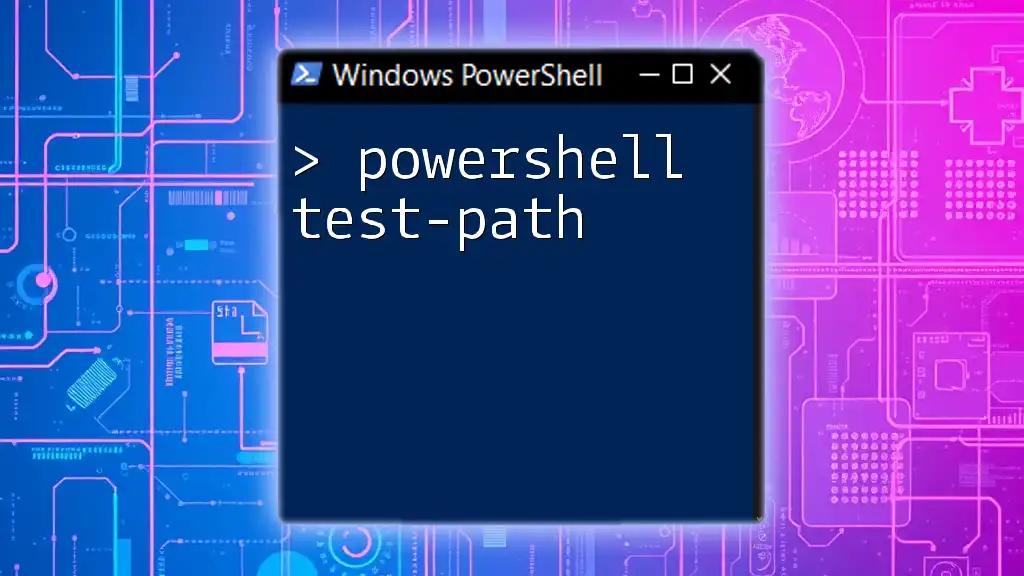
Call to Action
If you found this article helpful, feel free to share your experiences or any additional tips in the comments below. Engaging with the community helps everyone improve their PowerShell skills, especially regarding essential commands like `tail -f`.
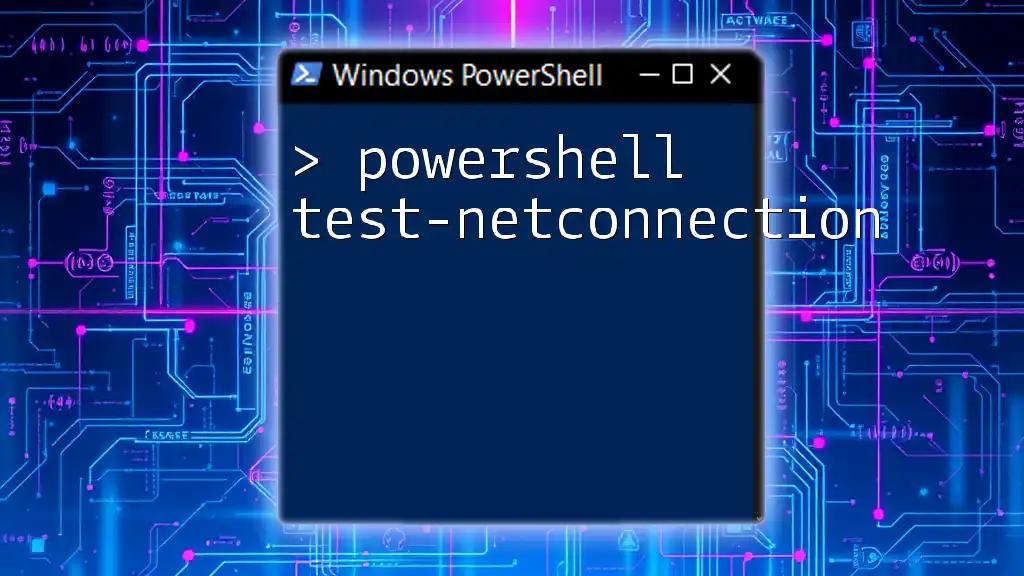
Additional Resources
For further learning, consider checking out online tutorials, official documentation, and community forums focused on PowerShell scripting and log management to deepen your understanding and skills.
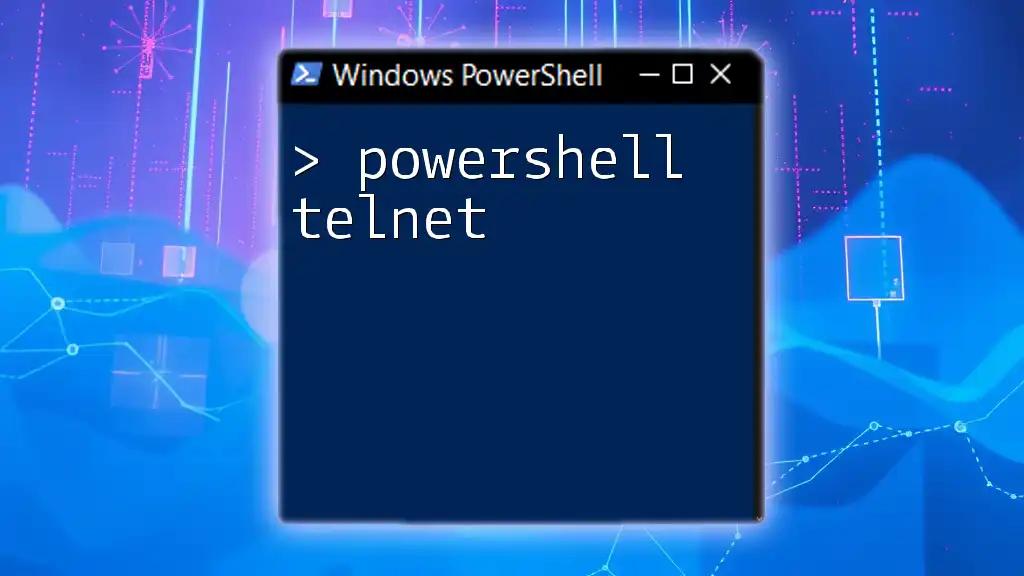
FAQs
A common question among users revolves around the differences in behavior between PowerShell and traditional Unix commands like `tail`. Understanding these differences is essential for effective usage and troubleshooting of real-time log monitoring techniques.