In PowerShell, an inline if statement allows you to execute a command based on a conditional check within a single line of code, using the `if` operator in a streamlined format.
Here's an example of an inline if statement:
$age = 18; $isAdult = if ($age -ge 18) {'Yes'} else {'No'}; Write-Host "Is adult: $isAdult"
Understanding Conditional Statements
What are Conditional Statements?
Conditional statements are essential constructs in programming which allow you to execute different actions based on given conditions. In essence, they help the program make decisions. Most programming languages, including PowerShell, offer constructs like `if`, `else`, and `switch` to handle such conditions.
Why Use Inline Ifs?
PowerShell inline if statements enable the execution of conditional logic in a more concise format. Instead of lengthy `if-else` blocks, inline ifs allow for single-line shortcuts. This becomes especially handy in scripting where space and readability matter. Using inline conditions can lead to cleaner, more maintainable scripts, particularly in simple decision scenarios.
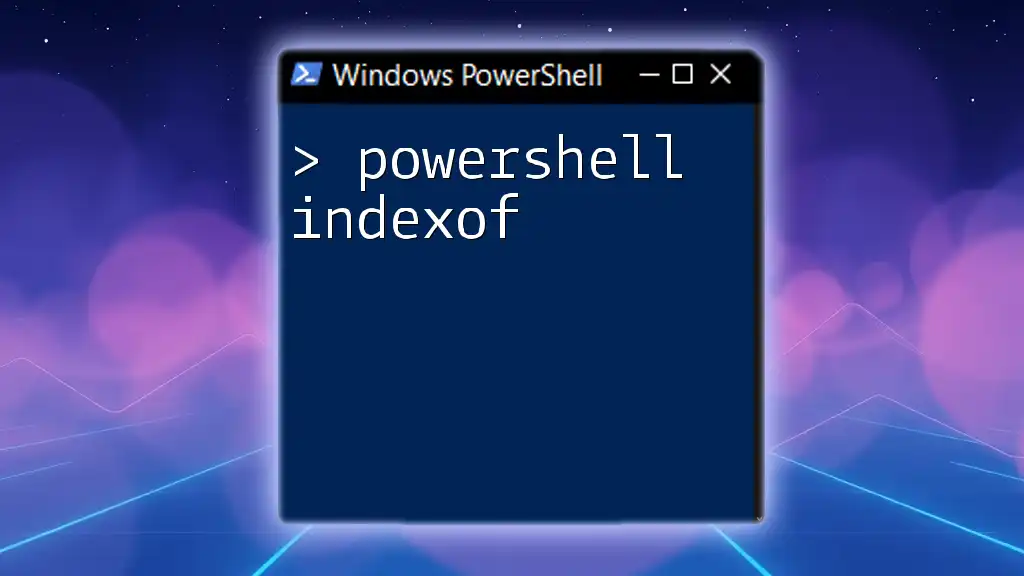
Overview of the Inline If Syntax in PowerShell
Basic Syntax
The basic syntax for an inline if statement in PowerShell employs the `? :` operator. The structure is as follows:
$result = $condition ? $valueIfTrue : $valueIfFalse
In this syntax:
- $condition is evaluated.
- If it’s true, $valueIfTrue is returned; otherwise, $valueIfFalse is returned.
Example: A simple inline if usage might look like this:
$isAdult = $age -ge 18 ? $true : $false
PowerShell `If` Statement vs. Inline If
Traditional `if` statements provide a full block that can be expanded to include multiple lines of code. For instance:
if ($age -ge 18) {
$category = "Adult"
} else {
$category = "Minor"
}
While the above method is clear and straightforward, it can be verbose for simpler conditions. In contrast, the inline if simplifies such scenarios into a single line. However, it’s essential to recognize when readability may suffer for the sake of brevity.
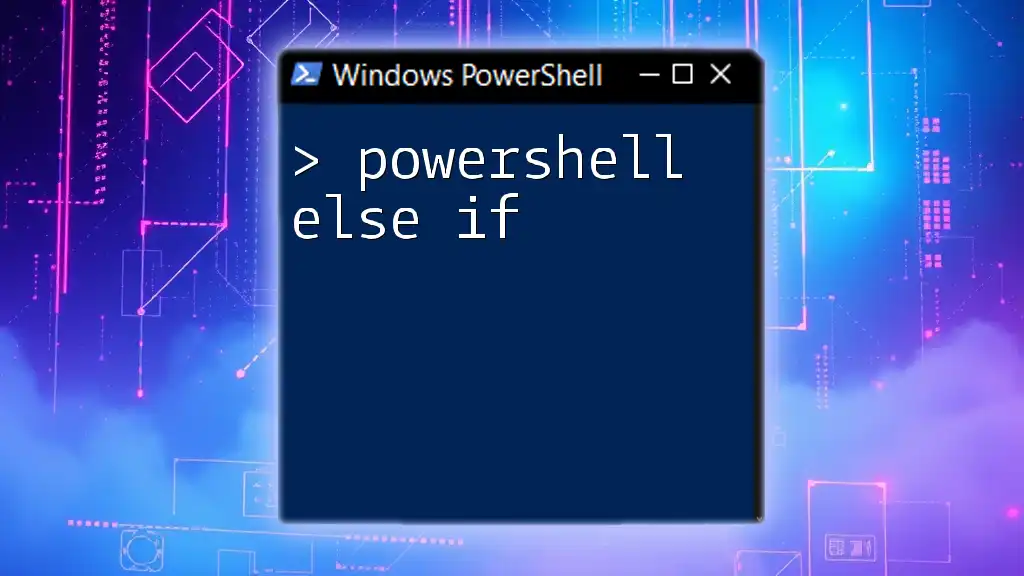
The `iif` Function in PowerShell
Introduction to the `iif` Function
PowerShell also supports the `iif` function found within .NET frameworks. This serves a similar purpose to inline ifs but offers distinctive syntax and evaluation methods. A notable advantage is that `iif` is designed to handle expressions more like a single function call.
Syntax of the `iif` Function
The syntax for the `iif` function is as follows:
$result = [System.Convert]::ToBoolean($status) ? "Yes" : "No"
Here’s how it operates:
- The expression is evaluated similarly to an inline if.
- However, since it operates as a function, it may be more appealing or clear to some developers.
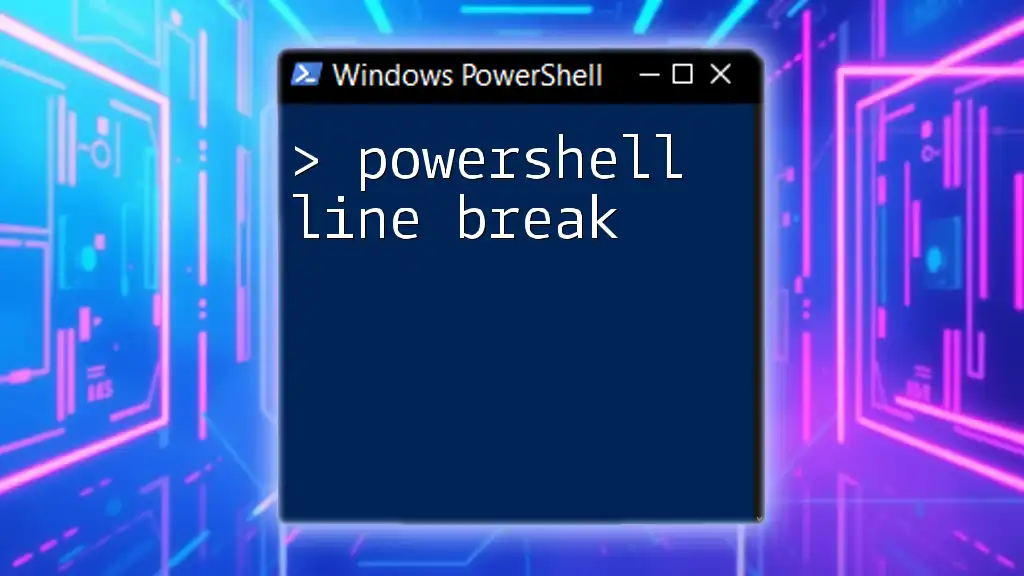
Key Differences Between Inline If and `iif`
Performance Factors
When performance is a consideration, it’s crucial to note that inline ifs can sometimes outperform the `iif` function since they are more straightforward and generally optimized in PowerShell. Evaluate the complexity of your code; if performance is vital (e.g., in loops), inline ifs might offer better efficiency.
Readability Comparisons
In terms of readability, inline ifs are generally more concise, while `iif` may provide better context when dealing with especially complex conditions due to the explicit nature of function calls. However, both can fall into “readability traps” if overcomplicated.
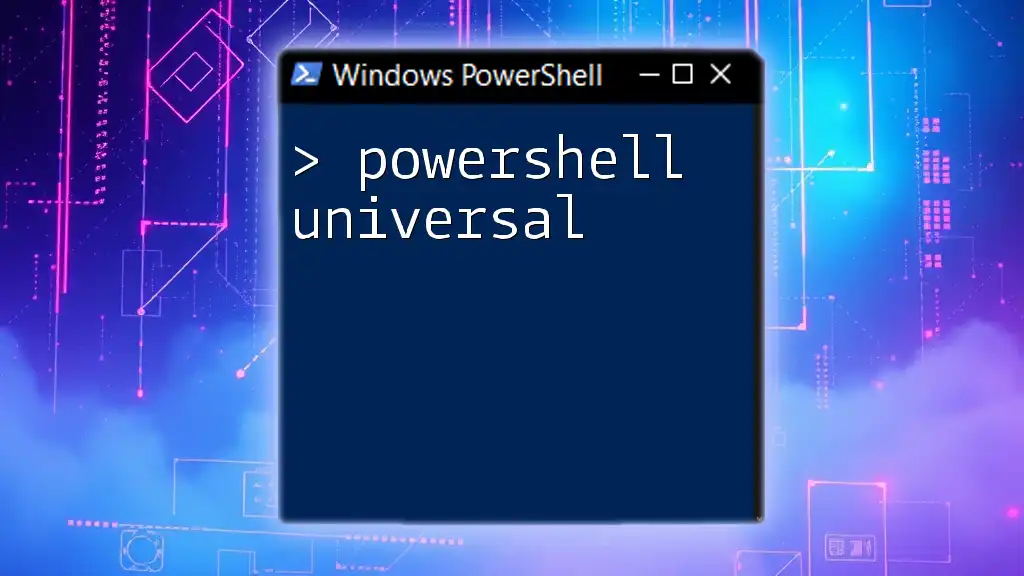
Practical Examples of Inline If
Example 1: Simple Conditional Assignment
Consider the following example, which assigns a value based on a straightforward condition:
$age = 18
$category = $age -ge 18 ? "Adult" : "Minor"
Here, if the age is greater than or equal to 18, the category is set to "Adult"; otherwise, it is "Minor". This demonstrates the efficiency of inline ifs in cases where outcomes are easily defined.
Example 2: Using Inline If with Functions
Inline if statements can also be effectively used within functions. Take a look at the following function:
function Check-Status($status) {
return $status -eq "active" ? "Active" : "Inactive"
}
In this case, the function determines the status of an item by returning "Active" if the status is equal to "active". This reduces the overall footprint of the code and maintains clarity.
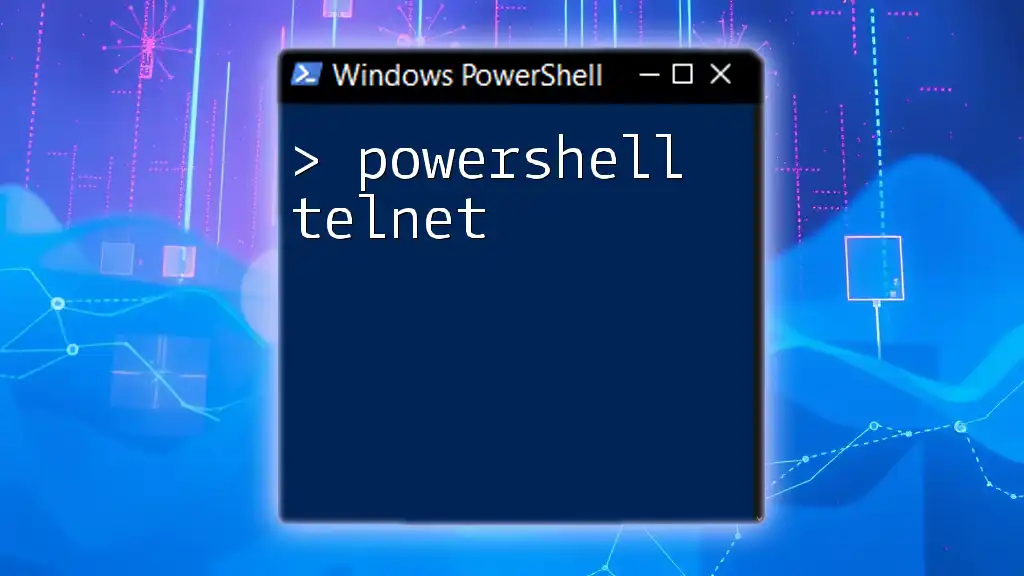
Best Practices for Using Inline Ifs and `iif`
When to Use Inline Ifs
Using inline ifs works best when the conditional logic is simple and straightforward. Conditions that lead to straightforward assignments or returns fit well. If the conditional statement requires a multi-step process or affects several outputs, consider traditional `if` statements instead for better organization and maintainability.
Code Readability and Maintenance
While brevity is valued, maintainability should also be upheld. Always prioritize clarity over complexity. A good rule of thumb is that if you’re struggling to read your own inline if statement at a glance, it’s worth rewriting it as a traditional `if` block.
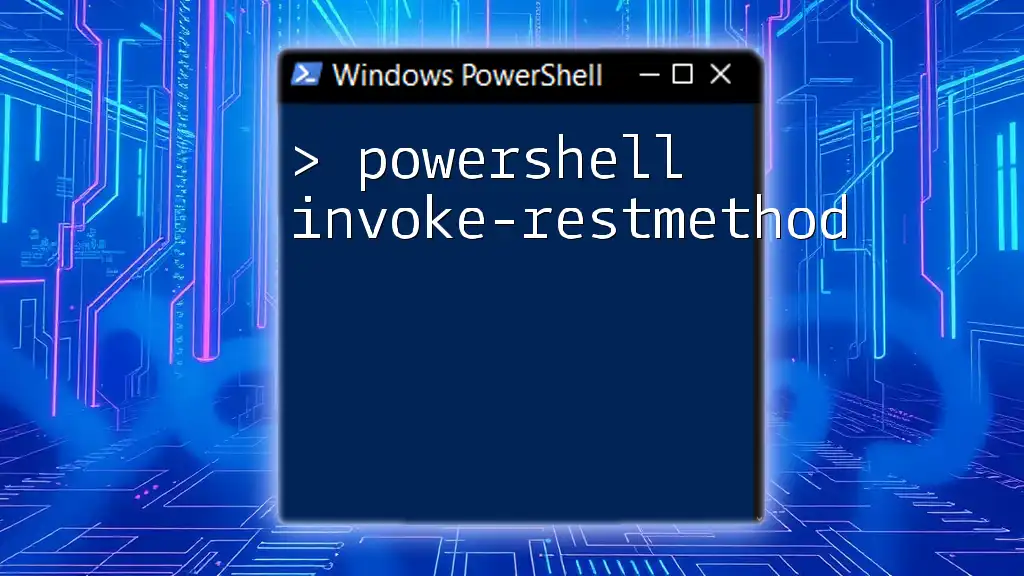
Common Pitfalls to Avoid
Overcomplicating Conditional Logic
A significant danger with inline ifs is the temptation to create intricate conditional expressions. Avoid making them overly complicated; if you find yourself introducing multiple layers of conditions, it’s better to revert to a standard `if` structure.
Debugging Inline If Statements
Debugging can be complicated when using inline conditions, especially if the condition is complex. If you encounter issues, consider breaking down the condition into simpler components or using explicit `if` statements for easier troubleshooting.
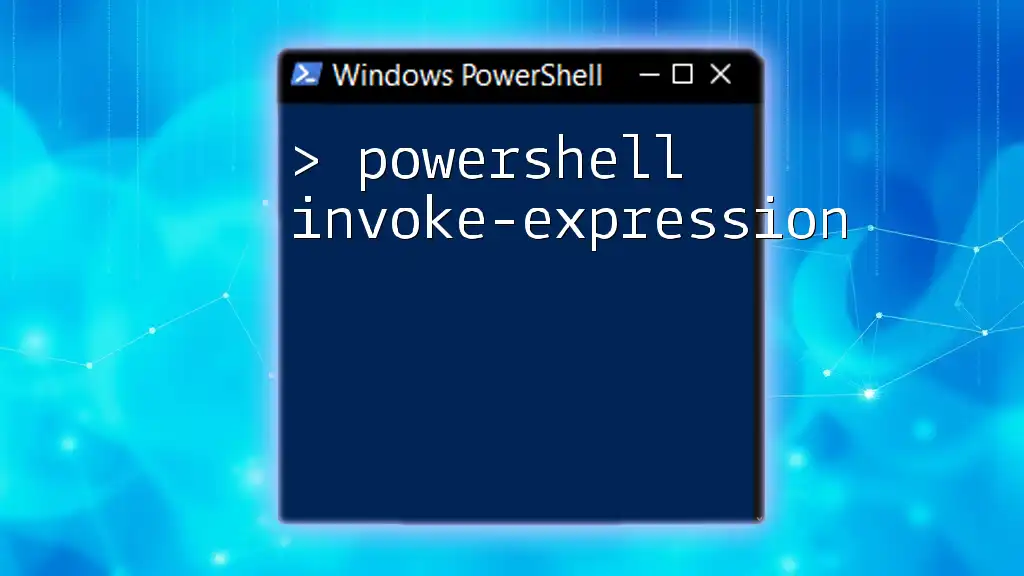
Summary
In summary, PowerShell inline if statements provide a powerful tool for programmers looking to write cleaner, more concise scripts. Understanding when to apply inline ifs vs. the `iif` function can greatly enhance your PowerShell programming efficiency. While inline ifs serve well in many situations, always weigh the need for clarity and maintainability in your scripts.
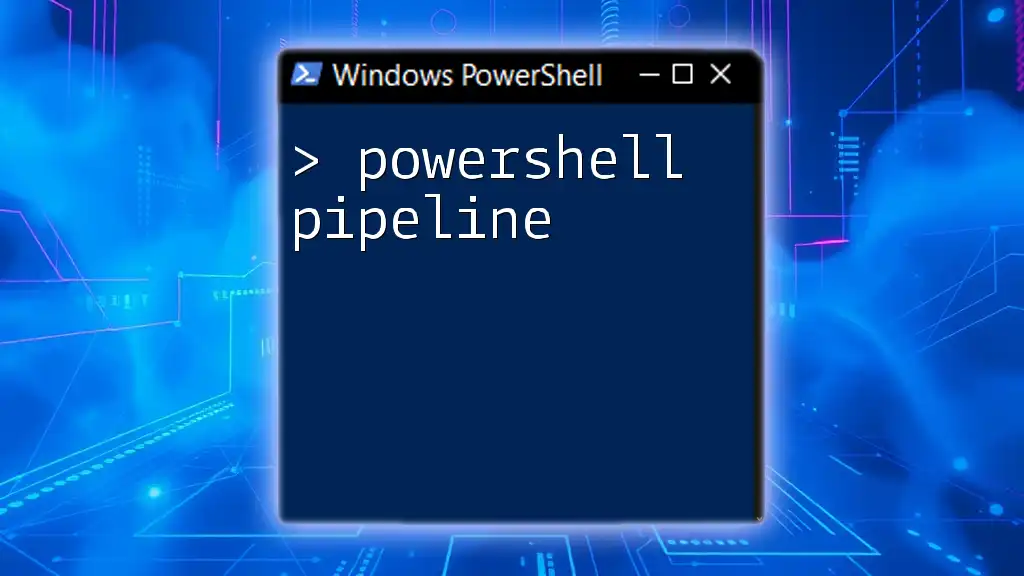
Additional Resources
For further exploration of PowerShell scripting, consider reviewing the official PowerShell documentation and additional programming references. Familiarizing yourself with these resources can lead to deeper insights into both inline conditions and overall PowerShell capabilities.
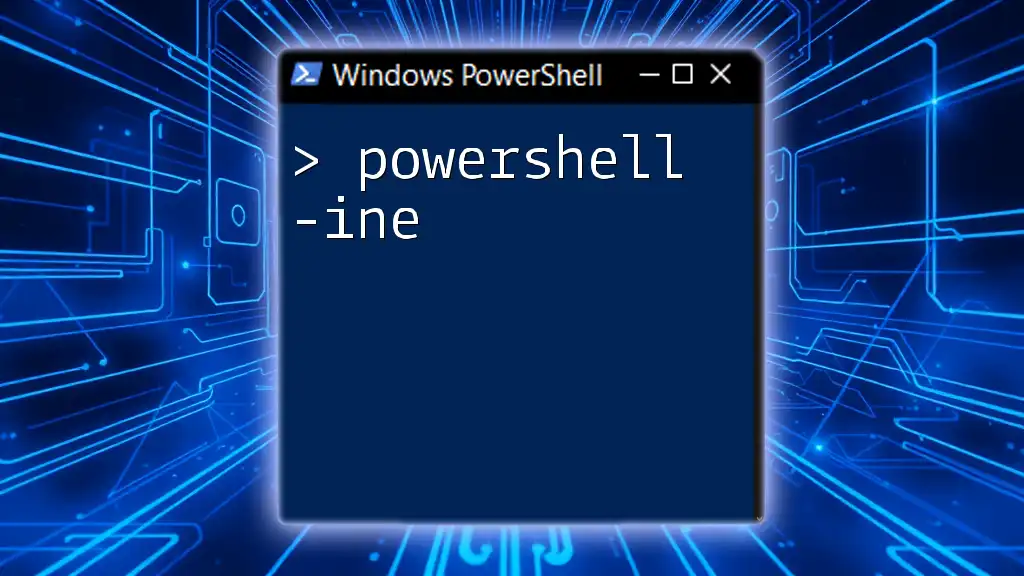
Call to Action
Take some time to practice implementing inline ifs in your own scripting projects. Experiment with different conditional scenarios and observe how they affect your code's structure. Share your experiences and challenge yourself to strike the perfect balance between brevity and clarity in your scripts!