A PowerShell style guide is a set of best practices and conventions that helps maintain consistency and readability in your PowerShell scripts. Below is an example of a simple command that demonstrates a common practice:
Write-Host 'Hello, World!'
What is PowerShell?
PowerShell is a powerful command-line shell and scripting language built on the .NET framework, designed specifically for system administrators and power-users to automate the administration of the operating system and applications. Unlike other scripting languages, PowerShell uses cmdlets, which are specialized .NET classes designed for specific tasks, making it a versatile tool for managing Windows-based systems. Its capabilities extend far beyond those of traditional shells, thanks to its object-oriented nature, allowing users to work with data as objects rather than plain text.
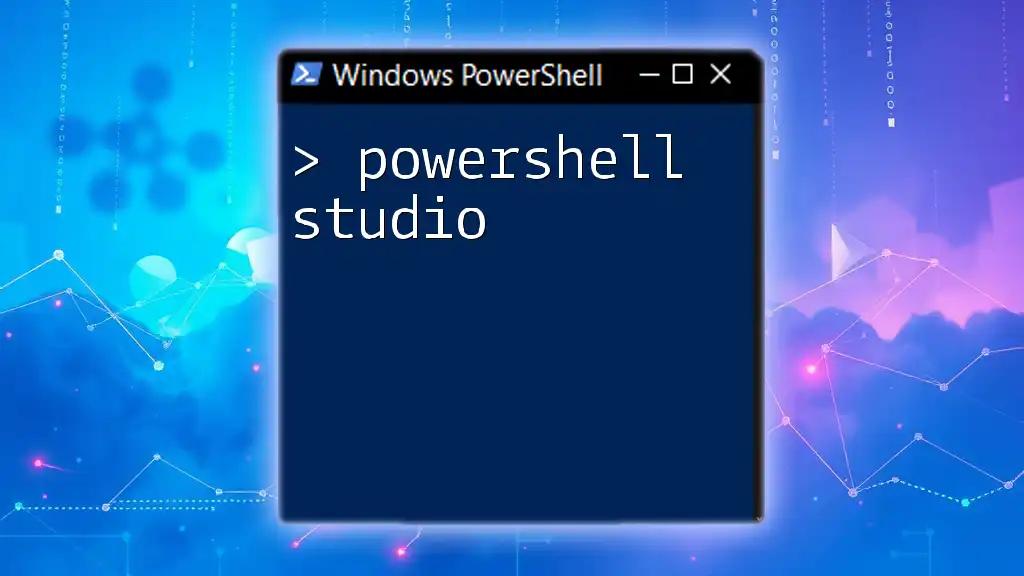
Benefits of Following a Style Guide
Adhering to a PowerShell style guide brings numerous benefits:
-
Consistency in Scripts: A consistent style across scripts helps create a cohesive codebase that is easier to navigate. When everyone follows the same guidelines, it reduces confusion and allows team members to focus on functionality.
-
Improved Readability: Well-structured code is easier to read and understand. This is particularly important when scripts are shared among team members or revisited after a long time.
-
Easier Collaboration: When scripts are uniform in style, collaboration becomes smoother as team members can quickly grasp each other’s work without having to get used to different styles.
-
Reduced Errors and More Maintainable Code: Consistent styling reduces the likelihood of errors and increases code maintainability, as the next person can easily follow the logic and format of previous scripts.
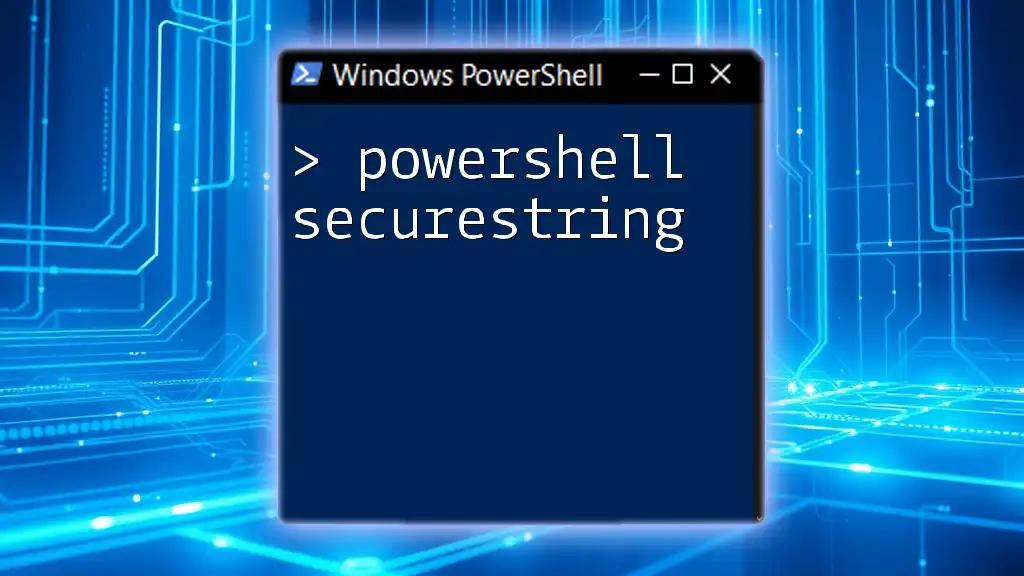
General Formatting Guidelines
Code Structure
Use spaces instead of tabs for indentation to ensure consistency across different environments. A commonly accepted practice in PowerShell is to use four spaces for each indentation level. For example:
if ($condition) {
Write-Host "Condition is true"
}
Line Length
Aim for a maximum line length of 80 to 120 characters. This helps keep your code manageable and readable on devices with smaller screens. If a line exceeds this limit, it’s best to use line breaks. For example:
$result = Get-Content "largefile.txt" |
Where-Object { $_ -like "*searchTerm*" }
Comments
Comments are essential for explaining the purpose of your code. They can make complex scripts much easier to understand.
- Inline Comments: Use inline comments sparingly to explain a single line or a small block of code. Here is an example:
$count = 0 # Initialize counter
- Block Comments: Use block comments when you need to explain a larger segment of code or logic:
<#
This block of code checks if the user exists in the database
and returns user details if found.
#>

Naming Conventions
Variables
Effective naming for variables is crucial. Choose meaningful names that convey the purpose of the variable clearly.
- Use CamelCase for variable names, such as `$UserName`, or use snake_case, like `$user_name`. Both styles are acceptable, but choose one and be consistent throughout your scripts.
Functions
Functions should follow the verb-noun format for their names, which enhances clarity and understanding of what the function does:
function Get-UserDetails {
# Function code
}
Constants
Constants often represent values that do not change. These should be easy to distinguish in your code; therefore, use ALL_CAPS for their names:
$MAX_RETRY_COUNT = 5
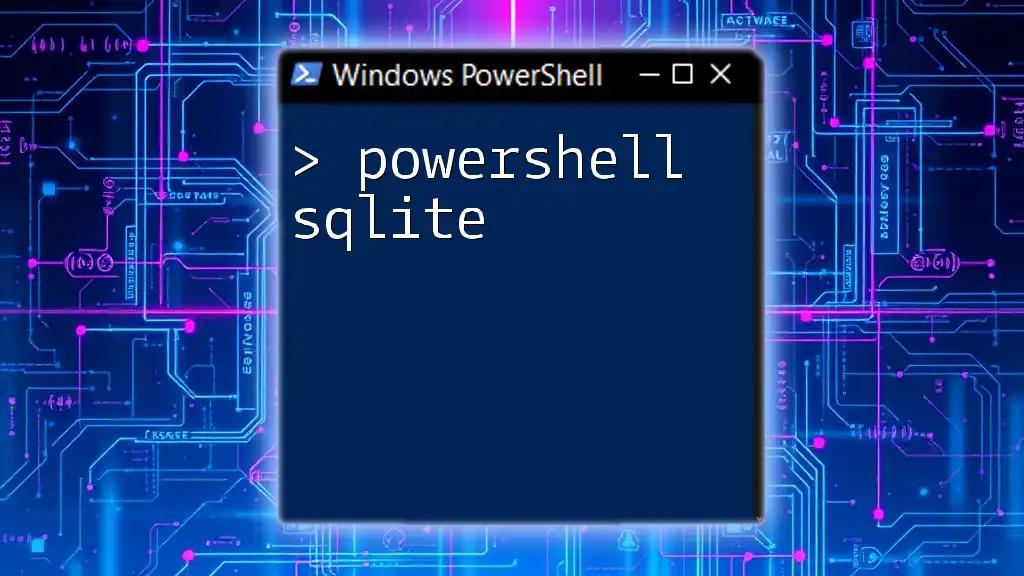
Cmdlets and Modules
Cmdlet Naming
Consistency is key when naming cmdlets. Maintain the Verb-Noun format to make the purpose clear. For instance, a cmdlet that retrieves processes can be named:
Get-Process
Module Organization
Organizing scripts into modules is essential for reusability and maintainability. Follow best practices for naming modules, so they are easily identifiable. A typical structure might look like this:
MyModule.psm1
- Public functions (exported)
- Private functions (not exported)
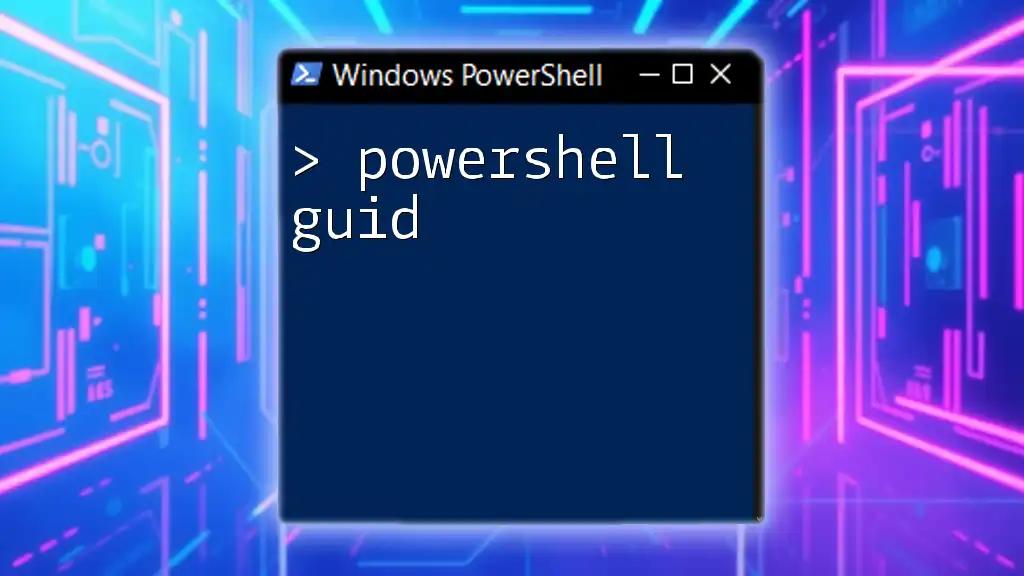
Error Handling
Try/Catch Blocks
Utilizing `try/catch` blocks is fundamental for error handling in PowerShell. This approach helps manage exceptions and ensures your script can handle errors gracefully:
try {
Get-Content "nonexistentfile.txt"
}
catch {
Write-Host "Error: $_"
}
Logging Errors
In addition to catching errors, consider logging them to track issues over time. Here’s a simple way to write errors to a log file:
$ErrorActionPreference = "Stop"
try {
# Code that may fail
}
catch {
Add-Content "error.log" -Value $_
}
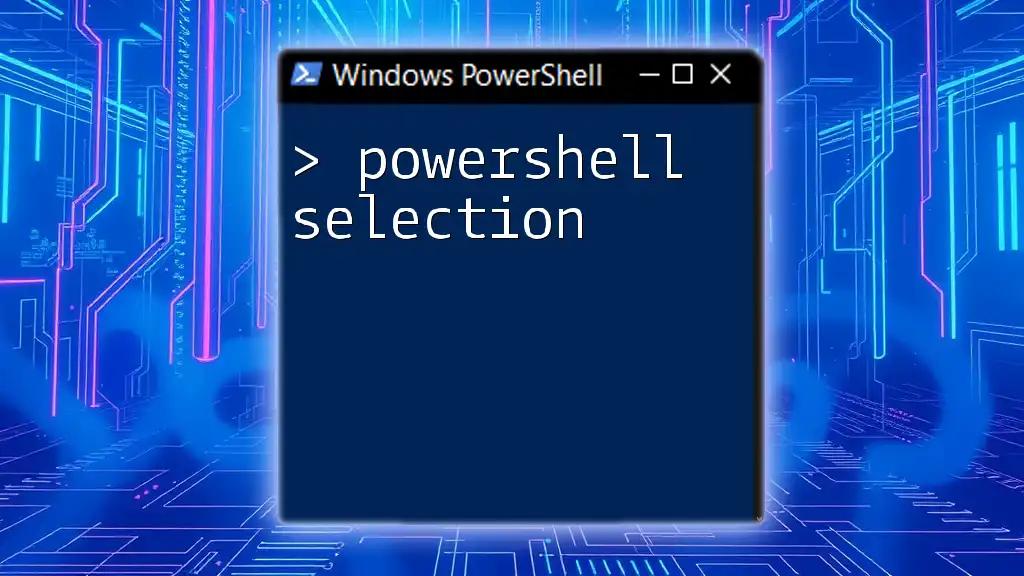
Best Practices for Function Design
Function Inputs
To enhance code clarity, define your function inputs using a `param` block. This approach clearly indicates what parameters the function expects:
function Get-UserDetails {
param (
[string]$UserName
)
# Function code
}
Output Types
Specifying output types for functions allows users to understand what the function will return. For example:
function Get-UserDetails {
[CmdletBinding()]
param (
[string]$UserName
)
[PSCustomObject]@{
UserName = $UserName
Status = "Active"
}
}
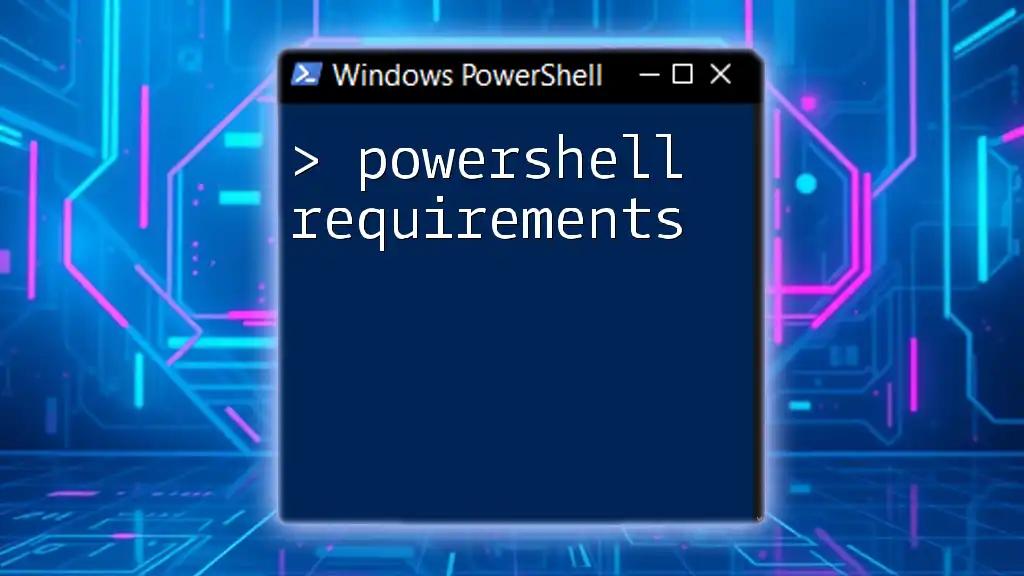
Conclusion
Following a comprehensive PowerShell style guide is not merely about aesthetics; it’s about creating a robust framework that fosters clarity, collaboration, and efficiency in scripting. By adopting these guidelines, you can produce scripts that are not only functional but also maintainable and understandable for both yourself and your colleagues.
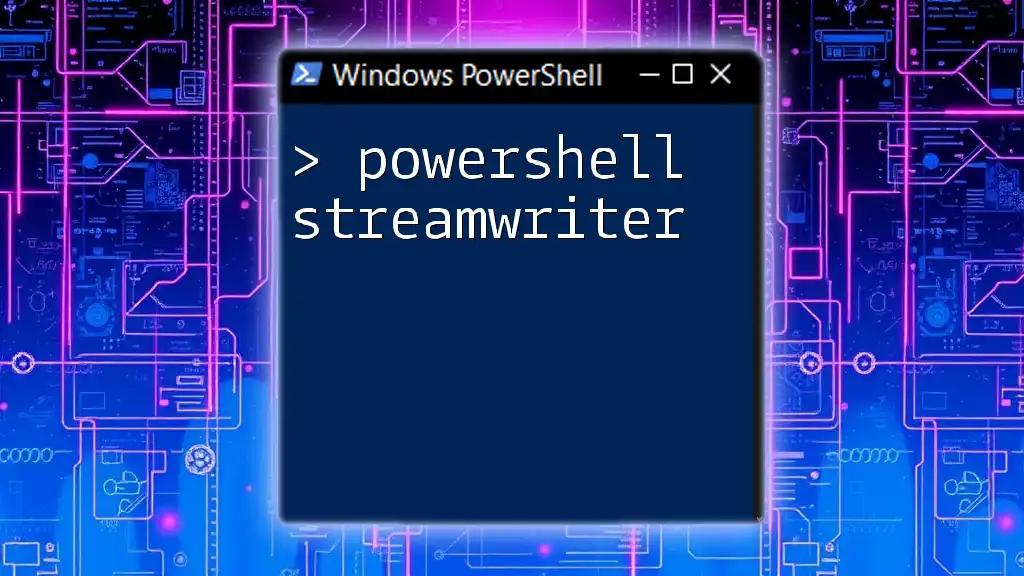
Additional Resources
For those looking for further reading on PowerShell practices, consult Microsoft’s official documentation, explore community forums, and engage with other PowerShell enthusiasts to expand your knowledge and skill set.