To check if a specific application is installed using PowerShell, you can query the registry or use the `Get-WmiObject` command.
$appName = "YourApplicationName"
if (Get-WmiObject -Class Win32_Product | Where-Object { $_.Name -eq $appName }) {
Write-Host "$appName is installed."
} else {
Write-Host "$appName is not installed."
}
Understanding PowerShell Commands
What is PowerShell?
PowerShell is a powerful scripting language and shell designed for system administration and automation. It allows users to control and automate tasks on their Windows operating systems. Unlike traditional command-line interfaces, PowerShell uses cmdlets, which are specialized .NET classes that carry out specific functions, providing flexibility and control over Windows environments.
Why Use PowerShell for Checking Applications?
Using PowerShell for application management brings several benefits:
- Automation: Automate repetitive checks, saving time and reducing effort.
- Consistency: Provide uniform results across multiple machines, useful for system administrators.
- Efficiency: Quickly gather information on installed software without navigating through multiple interfaces.
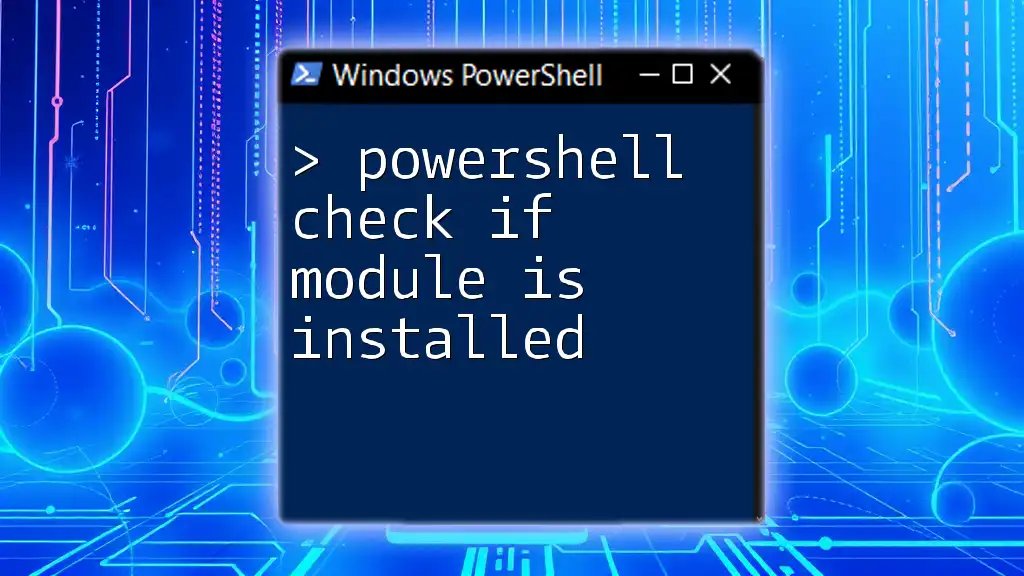
Methods to Check if an Application is Installed
Using Get-WmiObject Command
The `Get-WmiObject` cmdlet allows you to interact with Windows Management Instrumentation (WMI) to retrieve management data about local or remote systems. It is particularly useful for checking installed software.
Command Syntax:
Get-WmiObject -Query "SELECT * FROM Win32_Product WHERE Name = 'YourApplicationName'"
Example: Checking for 'Google Chrome':
Get-WmiObject -Query "SELECT * FROM Win32_Product WHERE Name = 'Google Chrome'"
Explanation of Output: If the command returns details about the application, it confirms that Google Chrome is installed. If nothing is returned, it indicates that the software is not installed.
Utilizing Get-Package Command
The `Get-Package` cmdlet is part of the PackageManagement module, facilitating the management of software packages. This is especially useful in modern Windows environments where applications may be installed via different package managers.
Command Syntax:
Get-Package -Name "YourApplicationName"
Example: Checking for 'Microsoft Office':
Get-Package -Name "Microsoft Office"
Output Analysis: The returned information will show if the application is installed, including relevant package details, versions, and providers. If you see details about 'Microsoft Office', then it is indeed installed on your system.
Querying the Registry
Accessing Registry for Installed Applications
The Windows Registry holds key information about the applications installed on a machine. Querying the registry can provide a comprehensive list of all installed software, including those not registered in WMI.
Command Syntax:
Get-ItemProperty -Path "Registry::HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall*"
Example: Checking for applications by querying the registry:
Get-ItemProperty -Path "Registry::HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall*" | Where-Object { $_.DisplayName -like "*YourApplicationName*" }
Output Explanation: The output will contain properties of the installed applications. If any entries are returned that match your query (using `-like` for partial matches), it indicates the application is installed, thus providing a reliable method to check installed software.
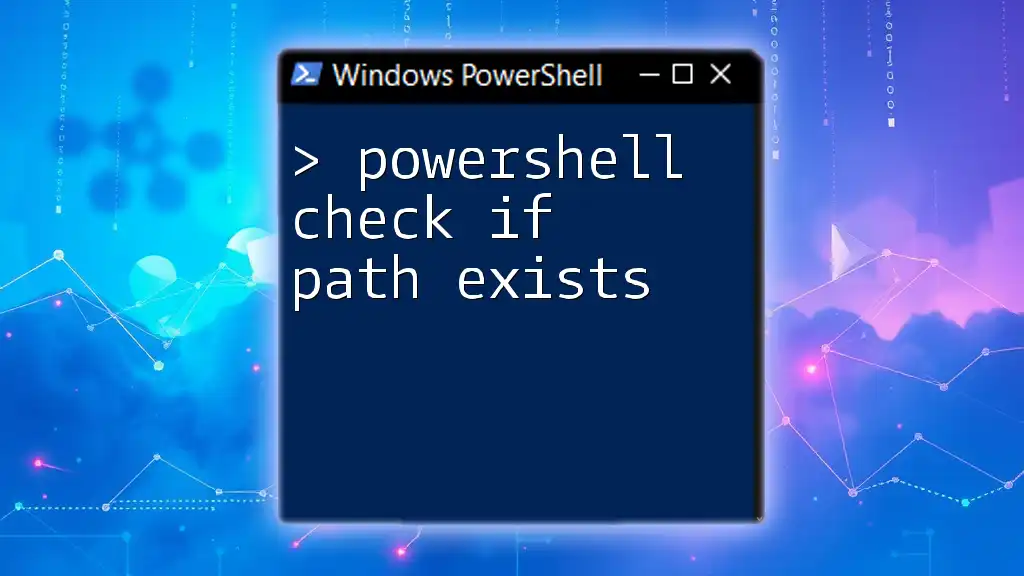
Automating the Check
Creating a Function to Simplify the Process
Creating a reusable PowerShell function can streamline the process of checks, making it easier to reuse the same logic without rewriting commands each time.
Code Snippet:
function Check-IfInstalled {
param(
[string]$ApplicationName
)
# Command Logic Here
}
Full Example Implementation
Here’s a complete PowerShell function that checks if a specific application is installed.
Code Snippet:
function Check-IfInstalled {
param(
[string]$ApplicationName
)
$installed = Get-WmiObject -Query "SELECT * FROM Win32_Product WHERE Name = '$ApplicationName'"
if ($installed) {
Write-Host "$ApplicationName is installed."
} else {
Write-Host "$ApplicationName is NOT installed."
}
}
This function checks for the specified application and outputs a clear message about its installation status, enhancing usability when needing to perform multiple checks.
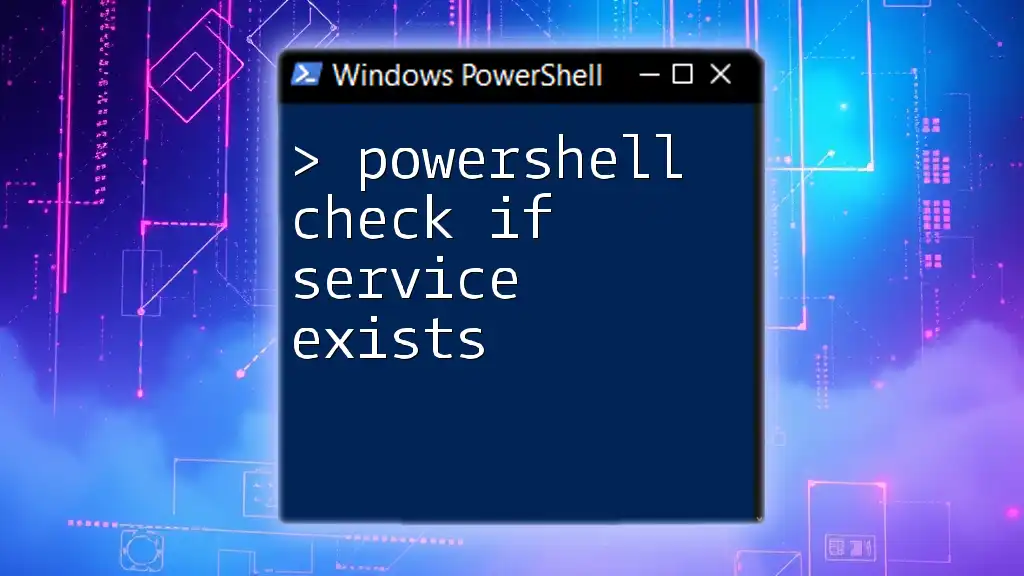
Troubleshooting Common Issues
Permission Problems
Sometimes, you may encounter permission issues when executing these commands. Always ensure to run PowerShell as Administrator to avoid these hurdles. Right-click on the PowerShell icon and select Run as Administrator to elevate your permissions for these tasks.
Errors in Command Execution
Common errors might include syntax mistakes or incorrect paths. Double-check the command for typos, ensure the application name is correct, and verify paths when querying the registry. When working with scripts, use the `Try-Catch` block to handle and troubleshoot exceptions that may occur during execution.
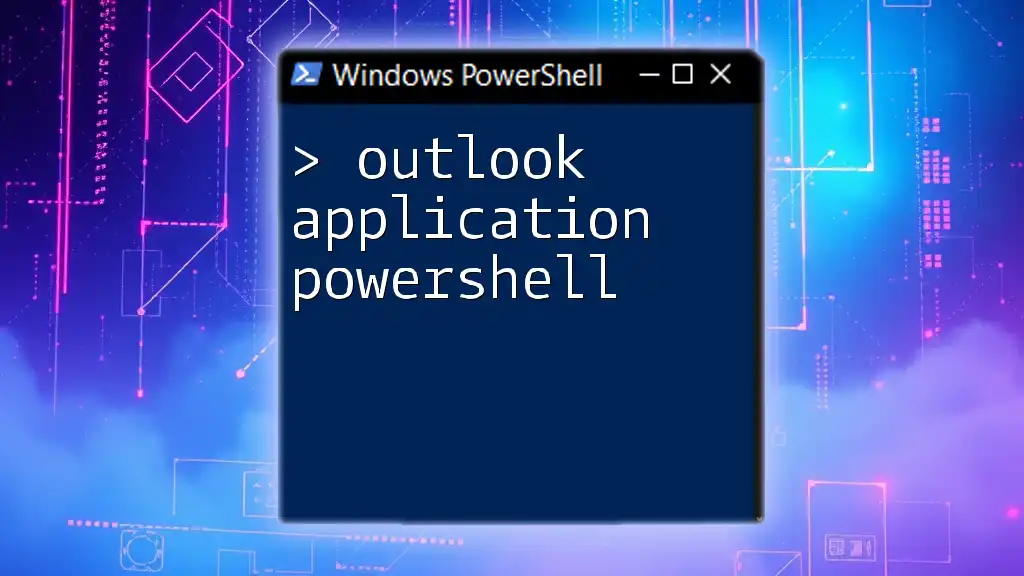
Best Practices for Using PowerShell to Check Installed Applications
To enhance the effectiveness of your PowerShell commands, consider these best practices:
- Comment Your Code: Add comments to your scripts for clarity on what each section does.
- Incorporate Error Handling: Use error handling mechanisms, such as `Try-Catch`, to manage exceptions and provide better debugging information.
- Use Meaningful Variable Names: This makes it easier to read and maintain your script in the long term.
- Test Scripts in a Safe Environment: Before deploying scripts in production environments, test them in a controlled setting to ensure they work as expected.
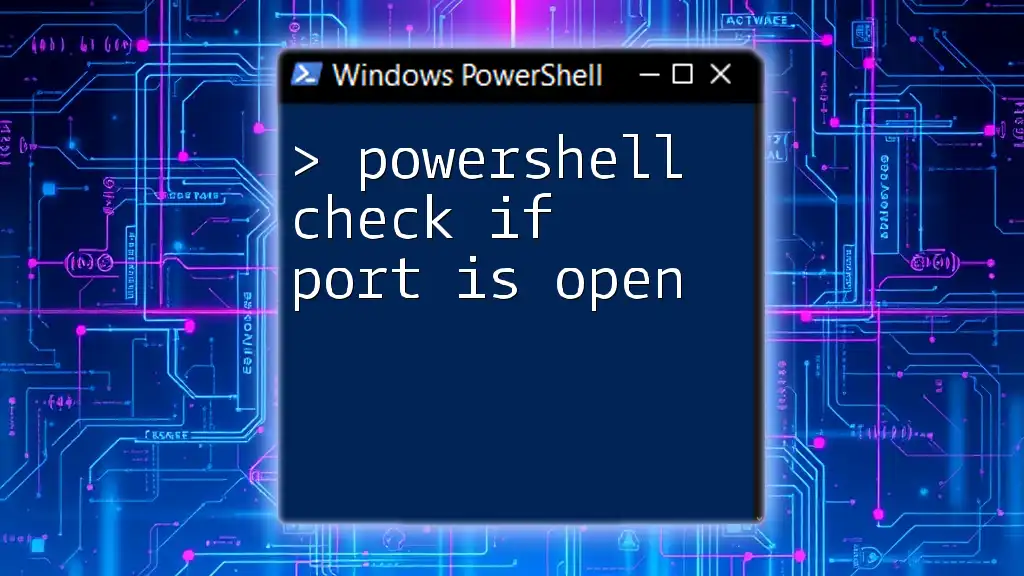
Conclusion
In this guide, we explored how to check if applications are installed using PowerShell, utilizing commands like `Get-WmiObject`, `Get-Package`, and registry queries. Understanding these methods empowers users to automate their software management processes efficiently. By practicing these techniques, you will gain proficiency in PowerShell and its vast capabilities for system administration.
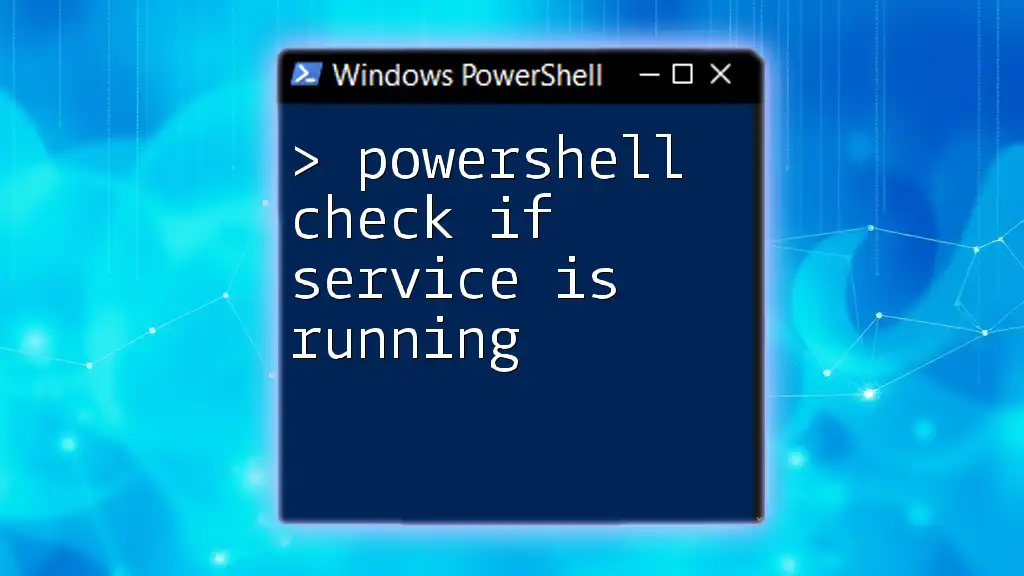
Additional Resources
For those looking to deepen their knowledge about PowerShell:
- Explore the official [PowerShell Documentation](https://docs.microsoft.com/en-us/powershell/)
- Seek online courses or tutorials on PowerShell scripting and system administration techniques.