The Outlook Application in PowerShell allows users to automate email tasks, manage contacts, and interact with their Outlook environment programmatically for enhanced productivity.
# Create a new Outlook application instance
$outlook = New-Object -ComObject Outlook.Application
# Display a message box
[outlook.Application]::MessageBox("Hello from Outlook!")
Understanding Outlook Application in PowerShell
What is Outlook Application Object?
The Outlook Application Object serves as the primary interface for automating Microsoft Outlook through PowerShell. This object allows you to manipulate Outlook's data structures—like emails, appointments, and contacts—by creating, modifying, or retrieving these items programmatically. Essentially, it acts as a bridge for PowerShell to communicate with Outlook.
Why Use PowerShell with Outlook?
Using PowerShell to automate tasks within Outlook can streamline workflows significantly. Here are some compelling reasons:
- Streamlining Repetitive Tasks: Simple tasks like sending recurring emails or organizing calendar entries can be automated, saving valuable time.
- Improving Productivity: Automating these tasks reduces the mental load on users, allowing them to focus on more critical activities.
- Scripting Capabilities: Through PowerShell scripts, you can perform complex tasks with minimal error, improving overall reliability.
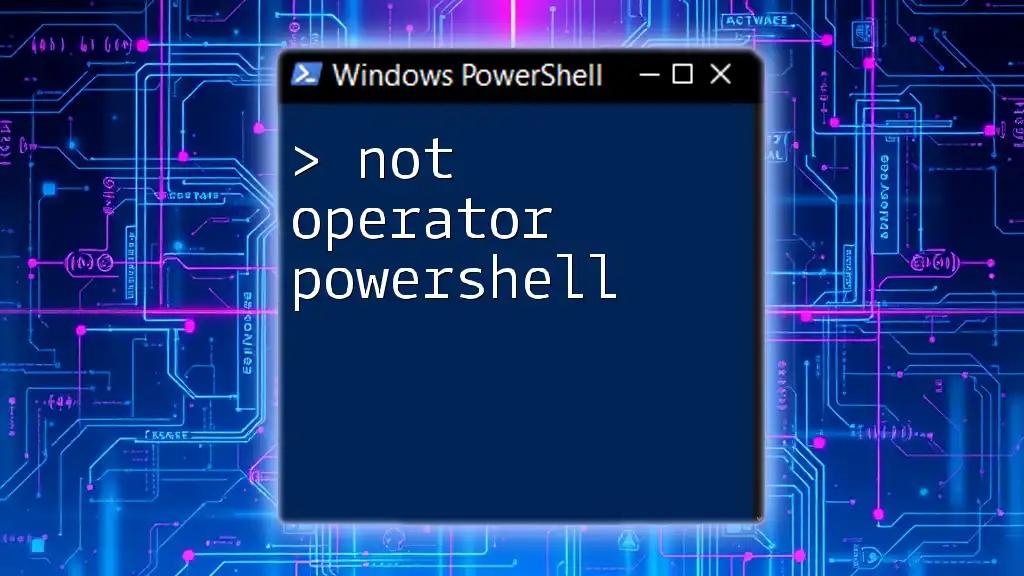
Setting Up Your Environment
Requirements
Before you start, ensure that you have the following:
- Microsoft Outlook: It must be installed and configured on your computer. The version should be compatible with your operating system.
- PowerShell: While most versions will work, using the latest stable version is advisable for optimal functionality.
Enabling PowerShell for Outlook Tasks
To leverage the Outlook Application Object, you need to load the necessary assemblies in PowerShell. Here’s a snippet to help you get started:
# Load the Outlook application
$Outlook = New-Object -ComObject Outlook.Application
This command creates a new Outlook Application instance, making it ready for further automation tasks.
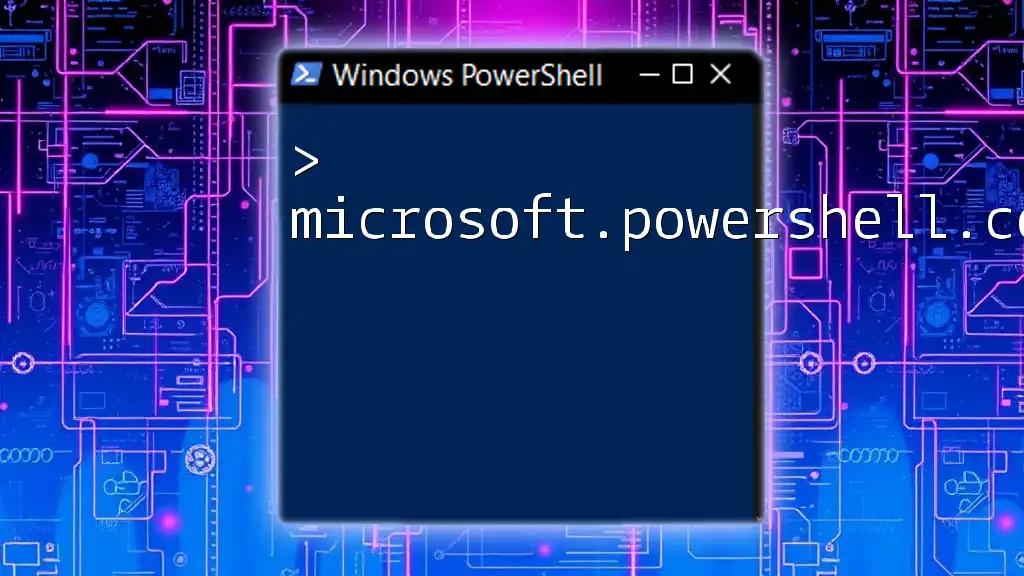
Basic Outlook Application Commands
Opening Outlook
To open Outlook using PowerShell, you can use the following commands:
$Outlook = New-Object -ComObject Outlook.Application
$Outlook.Visible = $true
This script not only launches Outlook but also ensures it is visible on your desktop.
Sending Emails with PowerShell
Crafting and Sending an Email
Sending emails programmatically enhances efficiency. You can easily create and send emails with just a few lines of code. Here's how:
$Mail = $Outlook.CreateItem(0) # 0 indicates a Mail Item
$Mail.Subject = "Test Email"
$Mail.Body = "Hello, this is a test email sent via PowerShell."
$Mail.To = "recipient@example.com"
$Mail.Send()
This script crafts an email with a subject and body, and then sends it to the specified recipient.
Accessing the Inbox
Retrieving Emails from the Inbox
Accessing your inbox is straightforward. The following script fetches the last 10 emails:
$Inbox = $Outlook.Session.GetDefaultFolder(6) # 6 is the Inbox folder
$Items = $Inbox.Items | Select-Object -First 10
$Items | ForEach-Object { Write-Host $_.Subject }
This command retrieves the last ten emails and outputs their subjects to the console.
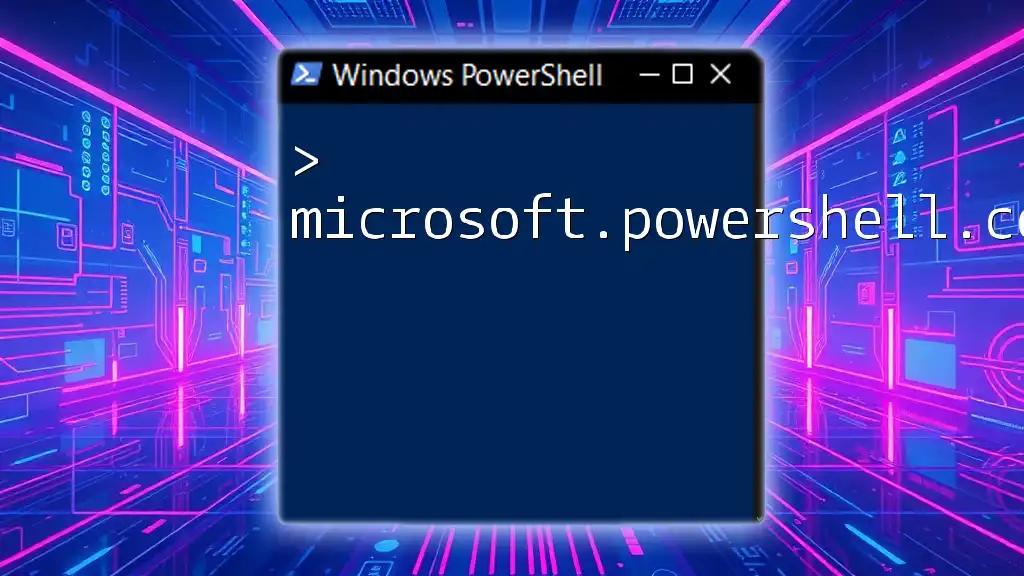
Advanced PowerShell Outlook Commands
Automating Calendar Events
Creating Calendar Appointments
You can easily create calendar appointments through PowerShell. The following example creates and saves a new appointment:
$Appointment = $Outlook.CreateItem(1) # 1 indicates an Appointment Item
$Appointment.Subject = "Team Meeting"
$Appointment.Start = [datetime]"2023-12-01 10:00:00"
$Appointment.Duration = 60
$Appointment.Location = "Conference Room"
$Appointment.Save()
This script sets up a meeting with details such as subject, start time, duration, and location.
Reading Calendar Events
Fetching your day’s events can similarly be done efficiently. Use the following script to list today’s events:
$Calendar = $Outlook.Session.GetDefaultFolder(9) # 9 is the Calendar folder
$TodayEvents = $Calendar.Items | Where-Object { $_.Start -ge (Get-Date) -and $_.Start -lt (Get-Date).AddDays(1) }
$TodayEvents | ForEach-Object { Write-Host $_.Subject, $_.Start }
This code retrieves all calendar events for the current day and prints their subjects and start times.
Managing Contacts
Adding New Contacts
Adding new contacts is straightforward with PowerShell. The following code snippet shows how to add a new contact:
$Contact = $Outlook.CreateItem(2) # 2 indicates a Contact Item
$Contact.FirstName = "John"
$Contact.LastName = "Doe"
$Contact.Email1Address = "john.doe@example.com"
$Contact.Save()
This script creates a new contact with specified first name, last name, and email address.
Searching for Contacts
Finding contacts can also be done easily. The following script searches for all contacts whose names start with "John":
$ContactsFolder = $Outlook.Session.GetDefaultFolder(10) # 10 is the Contacts folder
$ContactsFolder.Items | Where-Object { $_.FullName -like "John*" } | ForEach-Object { Write-Host $_.FullName }
This script displays the full names of all matching contacts in the console.
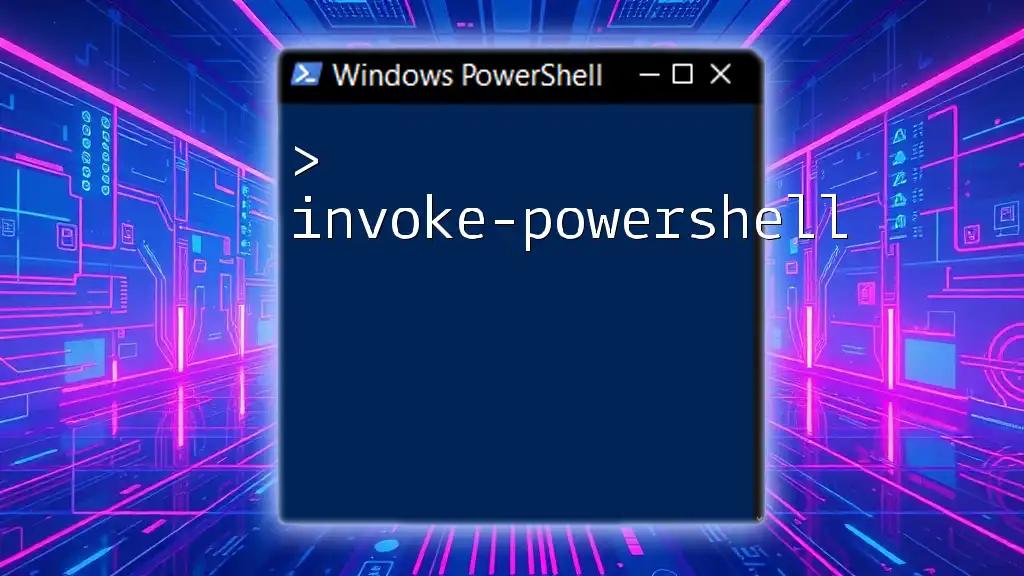
Troubleshooting Common Issues
Access Denied Errors
Access denied errors often occur when there are restrictions applied to Outlook or PowerShell. Ensure that both applications have the necessary permission levels. Running PowerShell as an administrator may resolve some of these issues.
Performance Issues
If you find that automating tasks is slow, consider breaking large scripts into smaller, manageable functions. This not only enhances performance but also makes troubleshooting easier.
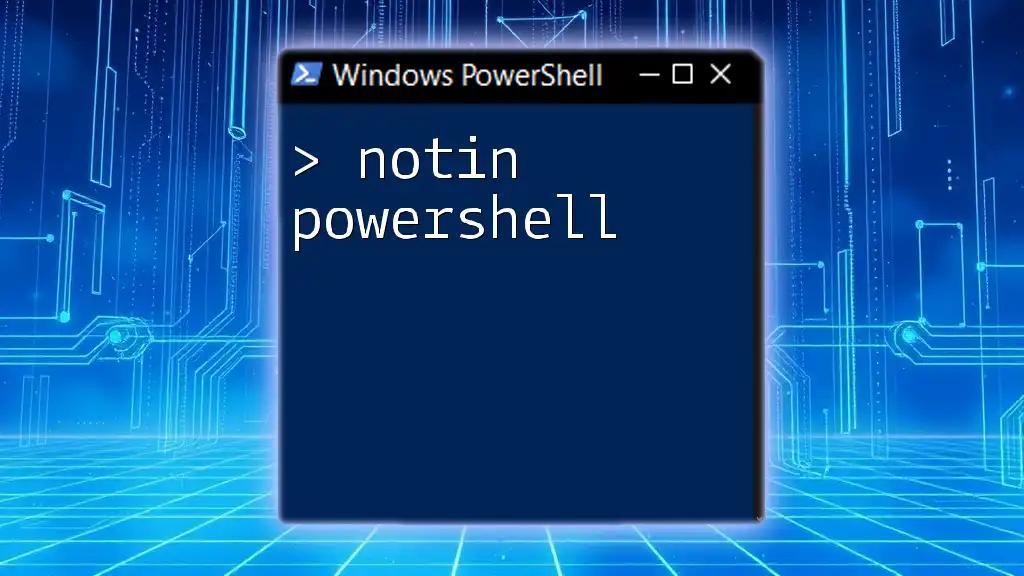
Best Practices
When writing PowerShell scripts to automate Outlook tasks, consider these best practices:
- Keep Code Readable: Use clear variable names and consistent formatting.
- Comment Your Code: Document each step to explain what your code does. This aids future maintenance.
- Test Incrementally: Run your scripts in segments to catch errors more effectively.
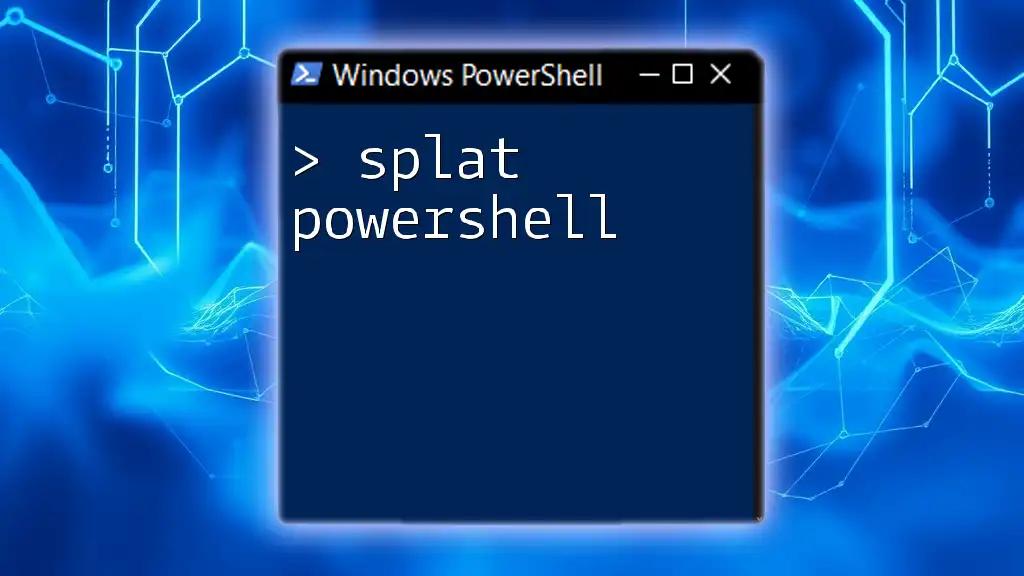
Conclusion
By understanding how to harness the Outlook Application PowerShell, you can vastly improve your productivity and automate repetitive tasks effectively. PowerShell not only simplifies managing Outlook applications but also opens up new possibilities for efficiency in your daily workflow.
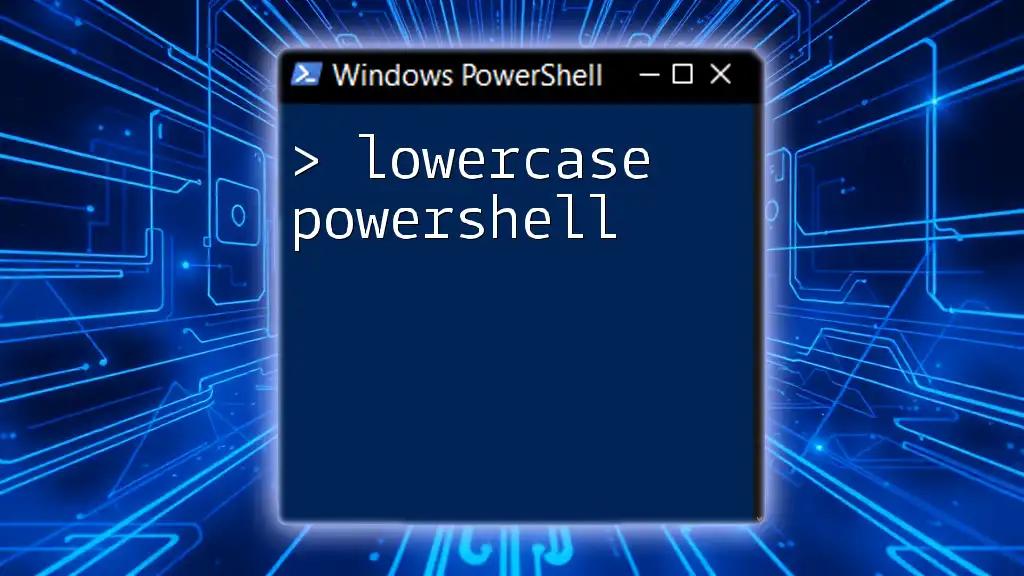
Additional Resources
To further improve your skills, refer to the following resources:
- Official Microsoft Documentation: Comprehensive guides and documentation on the Office Object Model.
- PowerShell Communities: Engage with other PowerShell enthusiasts through forums and discussion boards for collaborative learning.
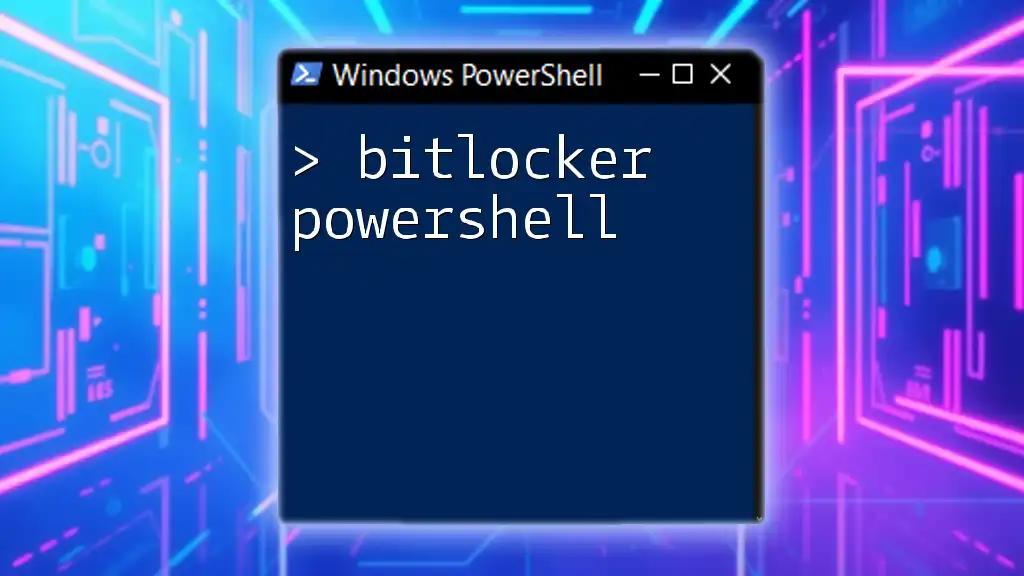
Call to Action
If this guide helped you, consider subscribing for more tips and tutorials on PowerShell and Outlook automation! Happy scripting!