To retrieve Azure Enterprise Applications using PowerShell, you can utilize the `Get-AzADApplication` cmdlet to access the application details in your Azure Active Directory.
Get-AzADApplication | Where-Object { $_.DisplayName -like "*YourAppName*" }
What are Azure Enterprise Applications?
Azure Enterprise Applications are crucial components within the Microsoft Azure ecosystem, allowing organizations to integrate, manage, and control access to various services and applications. These applications exist within the Azure Active Directory (Azure AD) and enable organizations to leverage features like single sign-on (SSO) and application-specific permissions.
Understanding Azure Enterprise Applications is key to enhancing your organization’s security posture and management efficiency. By managing these applications effectively, you can streamline user access and ensure that your data remains secure across multiple platforms.
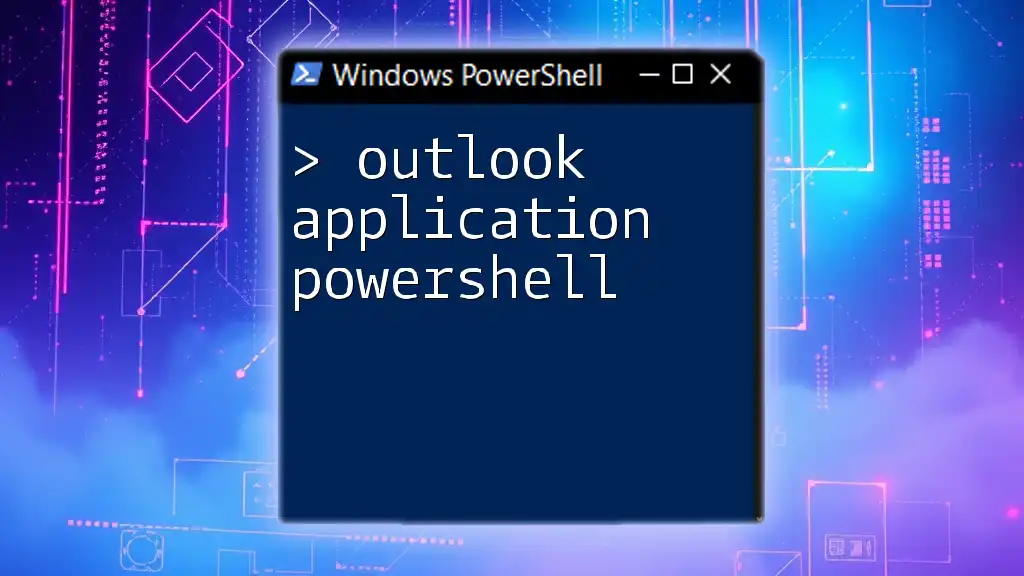
Prerequisites for Using PowerShell with Azure
To efficiently use PowerShell for managing Azure Enterprise Applications, you must meet a few prerequisites.
Installing Azure PowerShell
First and foremost, ensure you have the Azure PowerShell module installed. There are a couple of methods to do this, but the recommended approach is to use the PowerShell Gallery:
Install-Module -Name Az -AllowClobber -Scope CurrentUser
Make sure to install the latest version of the module to utilize the most up-to-date features.
Required Permissions
Before running PowerShell commands, verify that you have the appropriate permissions within the Azure environment. Generally, you'll need to be assigned roles such as Global Administrator or Application Administrator to access and manage Azure Enterprise Applications fully.
Connecting to Azure Account
To interact with Azure services via PowerShell, you'll need to authenticate your account. Here’s how to do it:
Connect-AzAccount
This command opens a login window for your Azure account and allows you to authenticate seamlessly to execute subsequent commands.
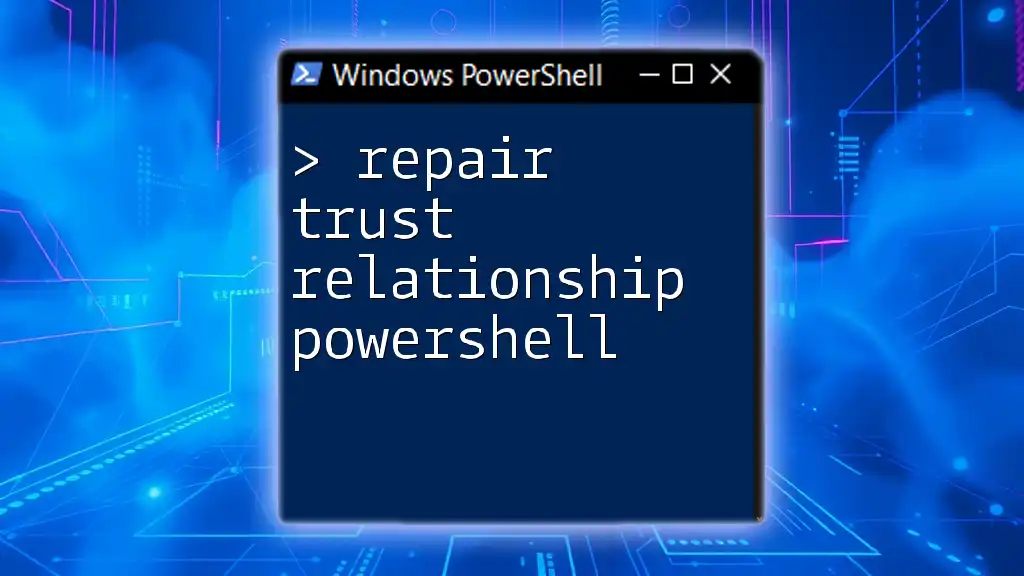
Overview of PowerShell Cmdlets for Azure Enterprise Applications
PowerShell provides a range of cmdlets specifically designed to manage Azure resources, including Enterprise Applications. These cmdlets simplify complex tasks and can tremendously speed up your workflow.
Key Cmdlets
The following cmdlets are essential for managing Azure Enterprise Applications:
Get-AzADServicePrincipal
The `Get-AzADServicePrincipal` cmdlet retrieves information about service principals associated with Azure Enterprise Applications.
Get-AzADServicePrincipal
This command returns a list of service principals, including important attributes such as the `ApplicationId` and `DisplayName`. Understanding these outputs is crucial for effective management.
Get-AzADApplication
Similar to the previous cmdlet, `Get-AzADApplication` allows you to gather details about registered applications in Azure.
Get-AzADApplication
This cmdlet returns details such as the app ID, creation date, and homepage, which further assist in managing applications.
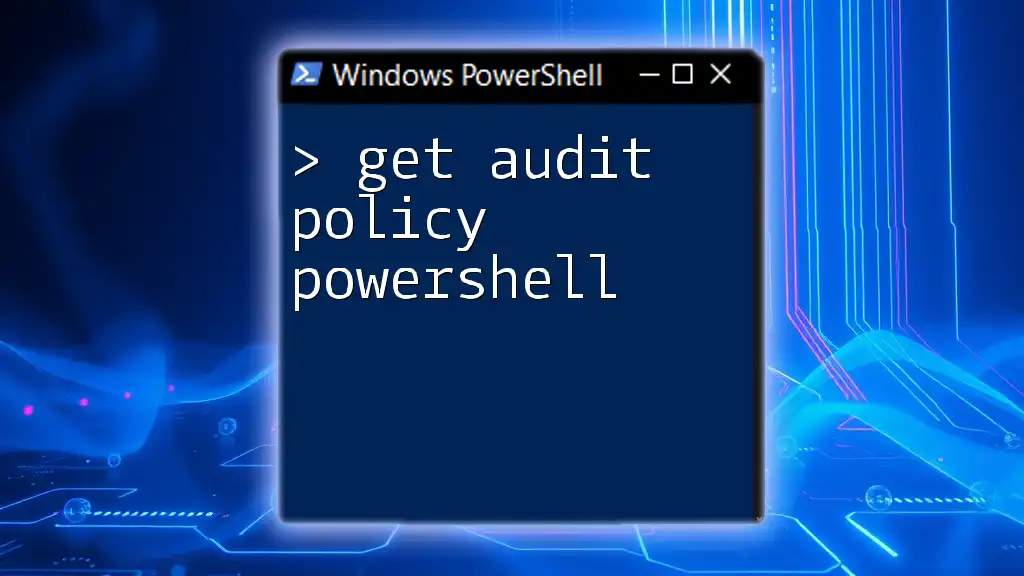
Using Get-AzADServicePrincipal to Retrieve Enterprise Applications
The `Get-AzADServicePrincipal` cmdlet is widely used to obtain a list of Enterprise Applications. Here’s how to use it effectively.
You can refine your commands by applying filters to return only the necessary items. For example, if you want to search for a specific application by its name, utilize:
Get-AzADServicePrincipal | Where-Object { $_.DisplayName -like "*example*" }
This command returns service principals whose display names contain the word "example," allowing you to quickly access relevant applications.
Exploring Output Data
The output from cmdlets may include various fields, such as:
- ObjectId: A unique identifier for the service principal.
- ApplicationId: A unique identifier for the application associated with the service principal.
- DisplayName: The name that users see in the Azure portal.
Understanding the significance of each field will enhance your ability to manage Azure resources effectively.
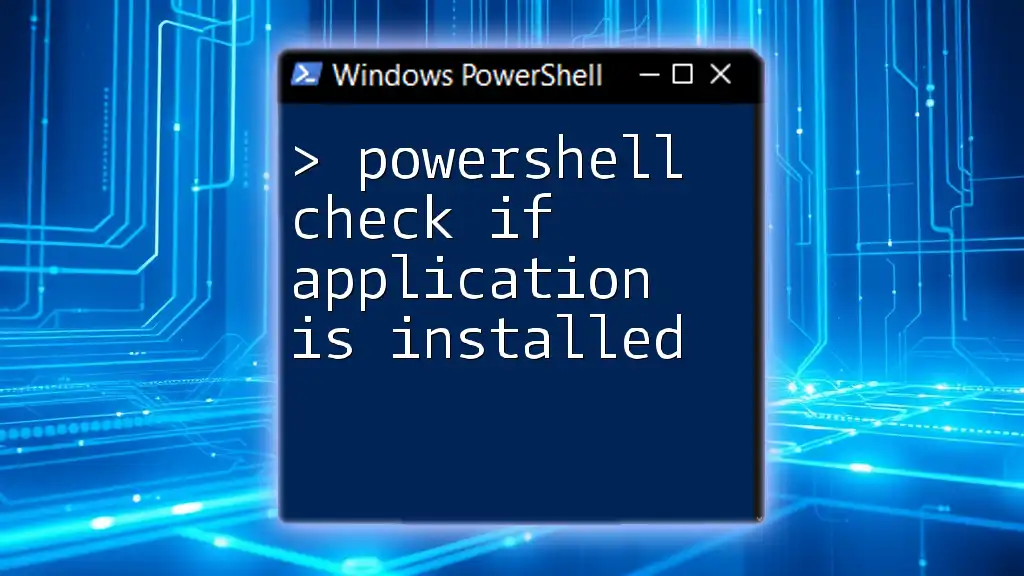
Specific Use Cases for Retrieving Azure Enterprise Applications
Scenario 1: Listing all Enterprise Applications
To quickly obtain a comprehensive list of all Enterprise Applications, simply run:
Get-AzADServicePrincipal
This command will provide a straightforward view of all service principals currently configured in the Azure Active Directory.
Scenario 2: Finding an Application by Name
If you need to find a specific Enterprise Application, use filtering mechanisms:
Get-AzADServicePrincipal | Where-Object { $_.DisplayName -eq "MyApplication" }
This allows for targeted searches, reducing time spent sifting through unnecessary data.
Scenario 3: Checking Permissions of an Application
You can check the permissions associated with a particular application. Using the `Get-AzADServicePrincipal` with the `-ObjectId` parameter helps retrieve permissions linked to that service principal:
$sp = Get-AzADServicePrincipal -ObjectId "<Your-Service-Principal-ObjectId>"
Get-AzADAppRoleAssignment -ObjectId $sp.Id
This sequence enables you to review the permissions assigned to the specific application, ensuring that they align with your organization’s security policies.
Example Code Snippets
Providing complete examples helps illustrate how to use these cmdlets in practice. Repeat the earlier scenarios with inline notes summarizing what each command achieves. This guides readers on how to implement solutions effectively.
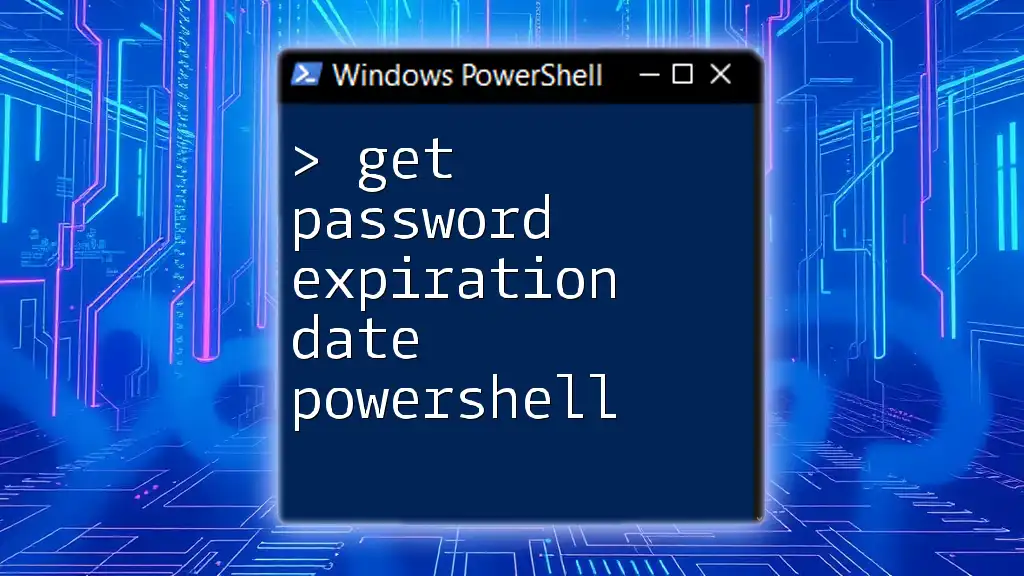
Troubleshooting Common Errors
Working with PowerShell and Azure can sometimes lead to encountering errors. One common issue is the “Insufficient privileges” message. This typically occurs when the account used for connecting to Azure lacks the necessary permissions.
To resolve this error:
- Verify the roles assigned to your account.
- Ensure that the account is part of the Azure Active Directory with sufficient privileges, and reattempt the command once adjustments have been made.
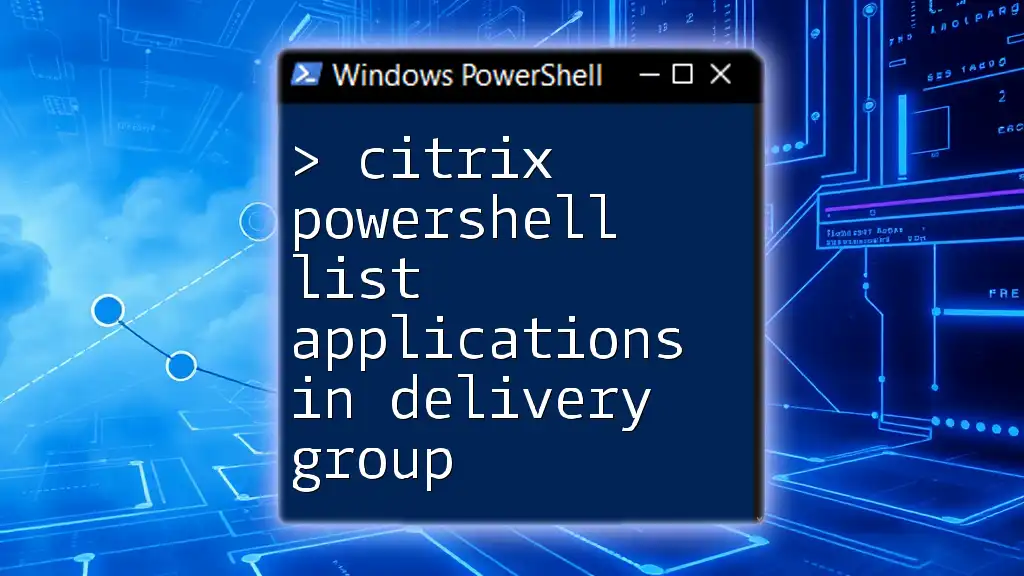
Conclusion
Utilizing PowerShell commands to manage Azure Enterprise Applications can greatly enhance organizational efficiency and security. Your ability to retrieve and manipulate these applications is vital in today’s cloud-centric environments. By following the guidelines outlined in this article, you’ll master the process of retrieving Azure Enterprise Applications using PowerShell.
Encouragement to explore further will lead to even greater mastery of Azure. Experimenting with additional cmdlets and functionalities can provide deeper insights and capabilities.
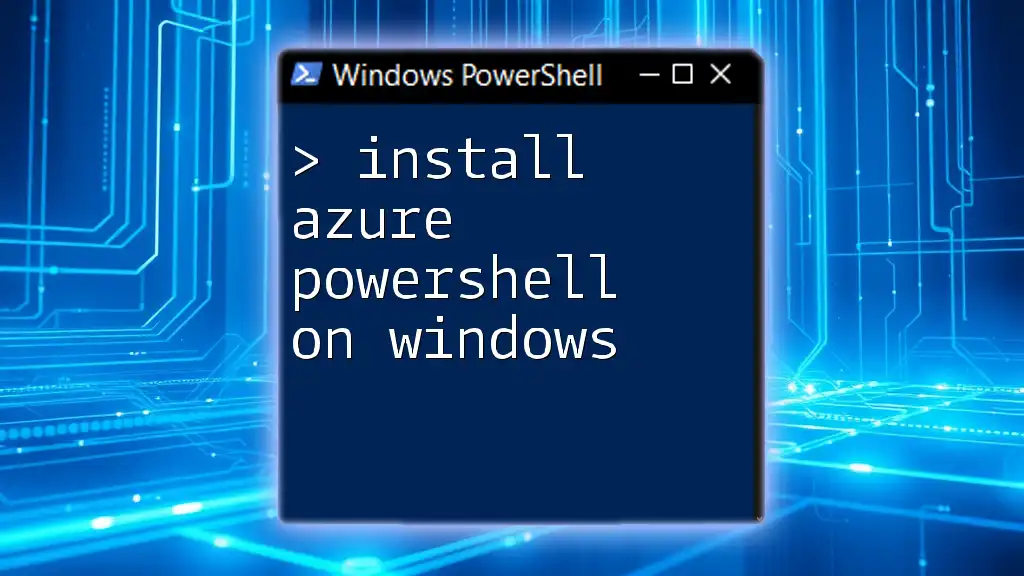
Additional Resources
While the fundamentals of using PowerShell with Azure Enterprise Applications have been covered, consider exploring the [Azure PowerShell documentation](https://docs.microsoft.com/en-us/powershell/azure/new-azureps-module-az?view=azps-7.1.0) for advanced strategies and updates. Engaging with community forums can provide ongoing support and insights from fellow users.
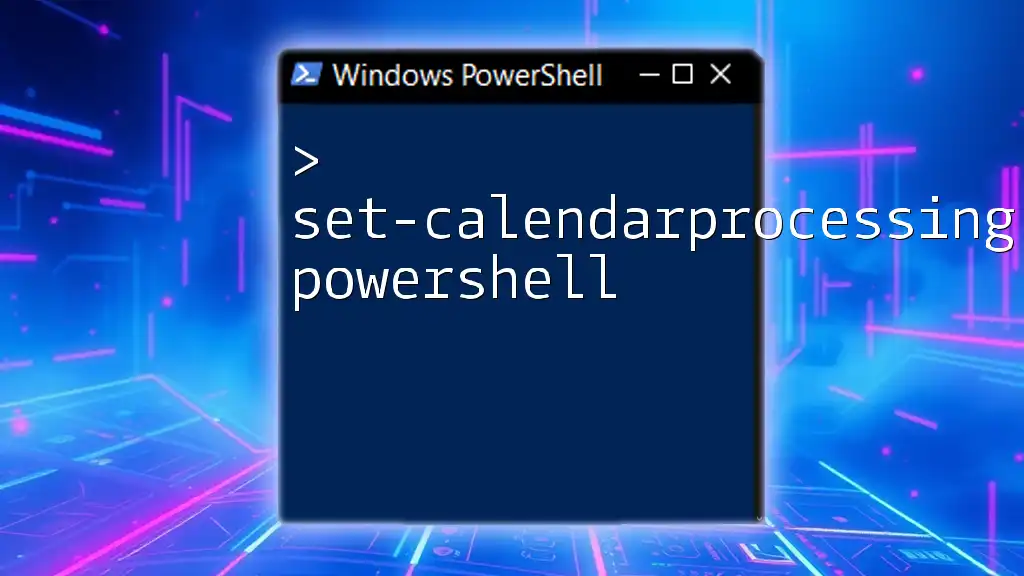
Call to Action
To enhance your learning experience, subscribe for updates, sign up for our courses, and follow our company on social media. Stay informed about the latest tutorials and developments in PowerShell and Azure technologies!