To retrieve the password expiration date of a user in PowerShell, you can use the following command:
Get-ADUser -Identity username -Properties PasswordLastSet | Select-Object Name, PasswordLastSet, @{Name='PasswordExpirationDate';Expression={[DateTime]::FromFileTime($_.PasswordLastSet).AddDays(90)}}
Replace `username` with the specific user's username to get their password expiration information.
Understanding Password Expiration
What is Password Expiration?
Password expiration refers to the security policy that requires users to change their passwords after a specific time period. This practice is essential for maintaining cybersecurity, as it reduces the risk of unauthorized access from stale or compromised passwords.
Why Check Password Expiration?
Monitoring password expiration dates is crucial for several reasons:
- Security Compliance: Many organizations must adhere to strict security standards that dictate password management practices.
- User Awareness: Regular notifications about upcoming expirations prepare users for necessary password changes, minimizing disruptions.
- Administrative Efficiency: System administrators can efficiently manage user accounts and reduce the risk of account lockouts.
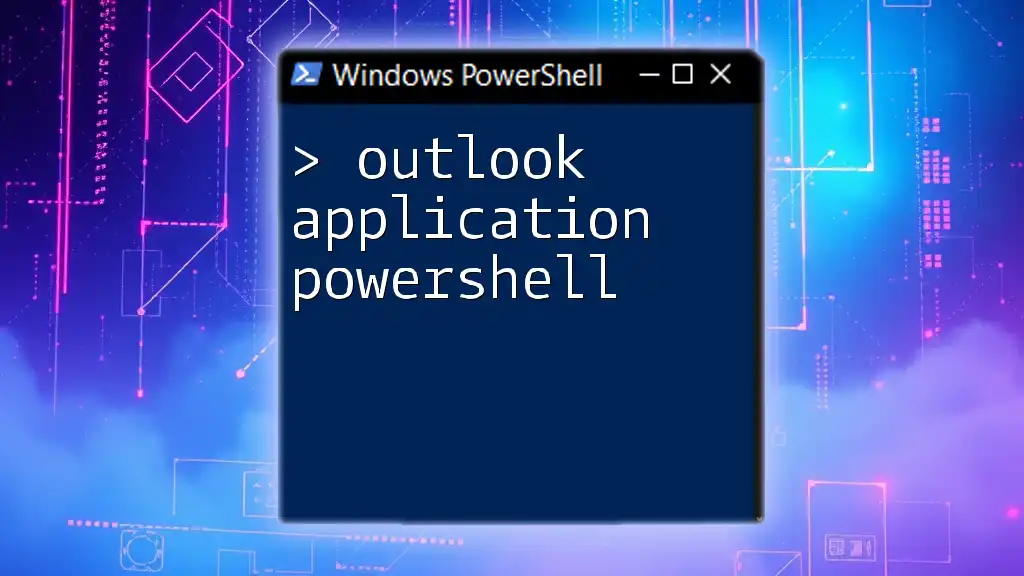
PowerShell and Active Directory
Introduction to Active Directory
Active Directory (AD) is a directory service used for managing computers and other devices on a network. It stores information about users, groups, and computers, including password policies. Understanding how to interact with AD using PowerShell is critical for efficiently managing user accounts and ensuring security compliance.
Using PowerShell with Active Directory
PowerShell provides a robust set of cmdlets specifically designed for Active Directory management. These cmdlets enable administrators to automate tasks, streamline processes, and execute complex queries with ease.
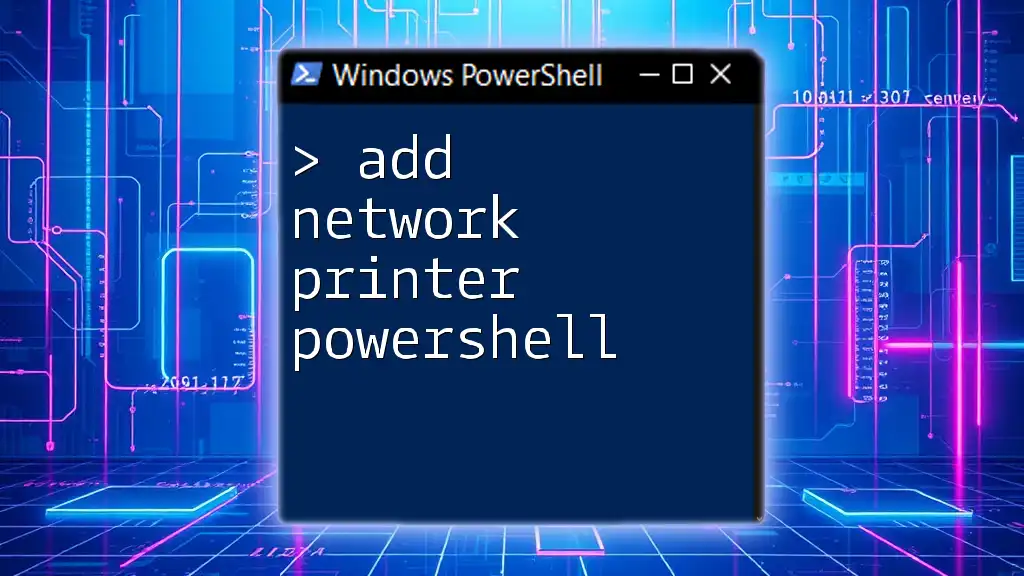
How to Get Password Expiration Date with PowerShell
Basic Cmdlet for Checking Password Expiration
To get a user's password expiration, the primary cmdlet you'll use is `Get-ADUser`. This cmdlet allows you to retrieve information about Active Directory users.
Here’s a basic example:
Get-ADUser username -Properties PasswordLastSet, PasswordNeverExpires | Select-Object PasswordLastSet, PasswordNeverExpires
In this command:
- `username` should be replaced with the actual user's username.
- The output will show the date when the password was last set and whether the password never expires.
Check Password Expiration Date for a Single User
To find out the exact expiration date for a user's password, use the following code snippet:
$user = Get-ADUser username -Properties PasswordLastSet
$expirationDate = $user.PasswordLastSet.AddDays((Get-ADDefaultDomainPasswordPolicy).MaxPasswordAge.Days)
$expirationDate
This code does the following:
- It retrieves the user's last password set date.
- It computes the expiration date by adding the maximum password age defined in your domain’s policy to the last set date.
Check Password Expiration Dates for All Users
If you want to see the expiration dates for all users, you can loop through them using the following script:
Get-ADUser -Filter * -Properties PasswordLastSet | ForEach-Object {
$expiration = $_.PasswordLastSet.AddDays((Get-ADDefaultDomainPasswordPolicy).MaxPasswordAge.Days)
[PSCustomObject]@{
UserName = $_.SamAccountName
PasswordExpirationDate = $expiration
}
}
In this script:
- `Get-ADUser -Filter *` retrieves all users from Active Directory.
- The `ForEach-Object` cmdlet processes each user to calculate and generate an object that contains the username and their respective password expiration date.
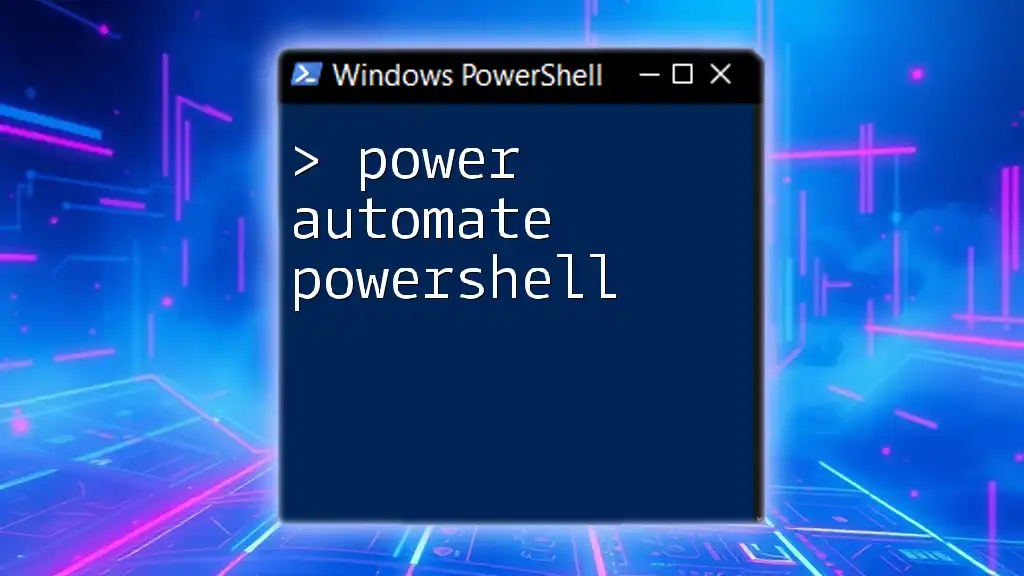
Advanced Techniques for Managing Password Expiration
Filtering Users Based on Password Expiration Date
It may be helpful to identify users whose passwords are expiring soon. Here’s how you can filter for users with passwords expiring in the next 14 days:
$thresholdDate = (Get-Date).AddDays(14)
Get-ADUser -Filter * -Properties PasswordLastSet | Where-Object {
($_.PasswordLastSet.AddDays((Get-ADDefaultDomainPasswordPolicy).MaxPasswordAge.Days)) -lt $thresholdDate
}
This command:
- Calculates a threshold date for 14 days from the current date.
- Filters users based on whether their password expiration date is less than that threshold.
Notifying Users of Upcoming Expiration
To enhance user experience, you can automate notifications sent to users whose passwords are about to expire. Here is an example of how to send email reminders:
$expiringUsers = # code to get users with expiring passwords
foreach ($user in $expiringUsers) {
Send-MailMessage -To $user.Email -Subject "Password Expiration Warning" -Body "Your password will expire on $($user.PasswordExpirationDate)" -SmtpServer "smtp.example.com"
}
In this example:
- You loop through each user whose password is expiring and send an email warning them about the upcoming expiration. Adjust the SMTP server address and body text as required.
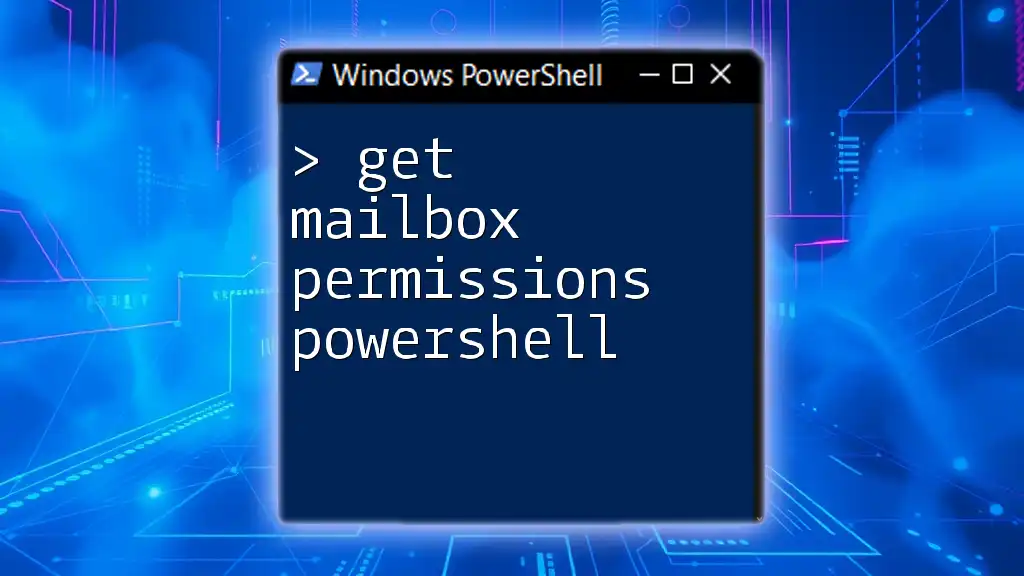
Common Errors and Troubleshooting
Common Issues When Checking Password Expiration
When working with PowerShell to check password expiration, you may encounter issues such as:
- Insufficient permissions: Ensure you have the necessary Active Directory permissions to query user properties.
- Cmdlet not found: If a cmdlet is not recognized, ensure you’re running PowerShell with the appropriate modules imported, such as the Active Directory module.
PowerShell Permissions
To successfully run these commands, make sure you have at least the Read permissions on user objects within Active Directory. If you're unsure about your permission level, you can verify it with your IT administrator.
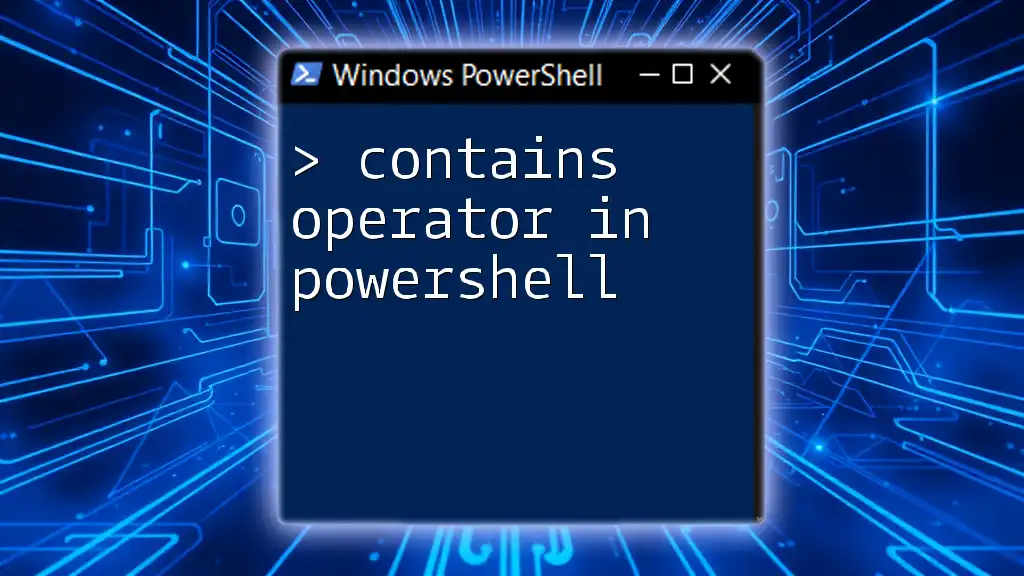
Conclusion
Understanding how to get password expiration dates using PowerShell is vital for maintaining security and compliance within your organization. By implementing the commands and techniques discussed, you can streamline the management of passwords and enhance user experience.
Encouragement to integrate these PowerShell commands into your regular administrative tasks will lead to more efficient Active Directory management. The tools are at your disposal—utilize them wisely!
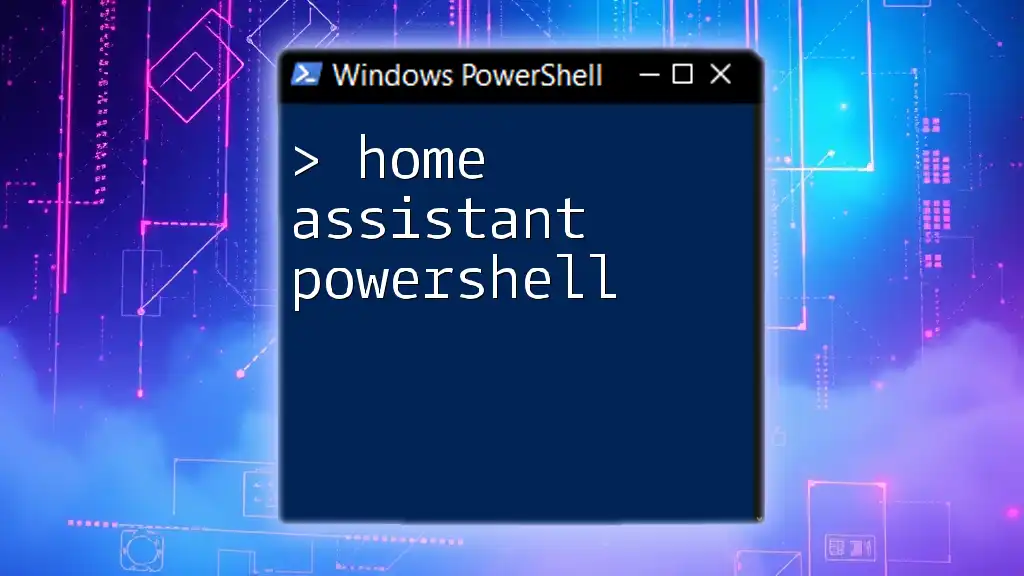
Call to Action
Consider joining a PowerShell course that dives deeper into these commands and offers practical exercises for mastering Active Directory management. Share your learnings, challenges, and successes with your peers, and contribute to a more secure working environment.
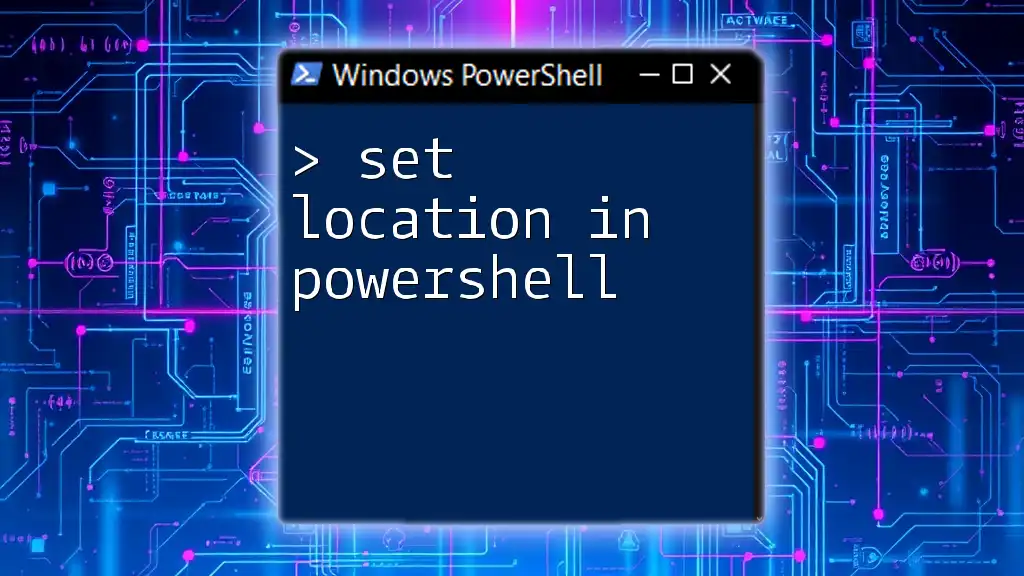
Additional Resources
For further reading, explore the official Microsoft documentation on PowerShell cmdlets for Active Directory. You can also look for books or online courses that specialize in PowerShell scripting and Active Directory management for enhanced learning and expertise.
Community Engagement
We encourage you to share your experiences with managing password expiration through PowerShell. Your insights can help others navigate similar challenges in their organizations.