String manipulation in PowerShell allows users to modify, format, and analyze text data efficiently using various commands and methods.
Here's a code snippet demonstrating string manipulation by replacing a substring within a string:
# Replace "World" with "PowerShell"
$originalString = 'Hello, World!'
$modifiedString = $originalString -replace 'World', 'PowerShell'
Write-Host $modifiedString
Understanding Strings in PowerShell
What is a String?
A string is a collection of characters used to represent text in programming. In PowerShell, strings are one of the fundamental data types, allowing for the manipulation of text, file names, and messages. Understanding how to manipulate strings in PowerShell is vital for automating tasks, processing data, and interacting with users effectively.
Creating Strings
In PowerShell, creating strings is straightforward. You can define strings using either single quotes (`'`) or double quotes (`"`).
-
Single Quotes: Text defined within single quotes is treated as a literal string. For example:
$singleQuoteString = 'Hello, World!'
-
Double Quotes: Strings in double quotes allow for variable interpolation, meaning you can include variable values within the string. For instance:
$name = "John" $doubleQuoteString = "Hello, $name" # Result: "Hello, John"
Additionally, PowerShell offers a convenient way to create multiline strings using here-strings, which are enclosed in `@"` and `"@`. This is especially useful for long text blocks:
$multiLineString = @"
This is a
multiline string.
"@
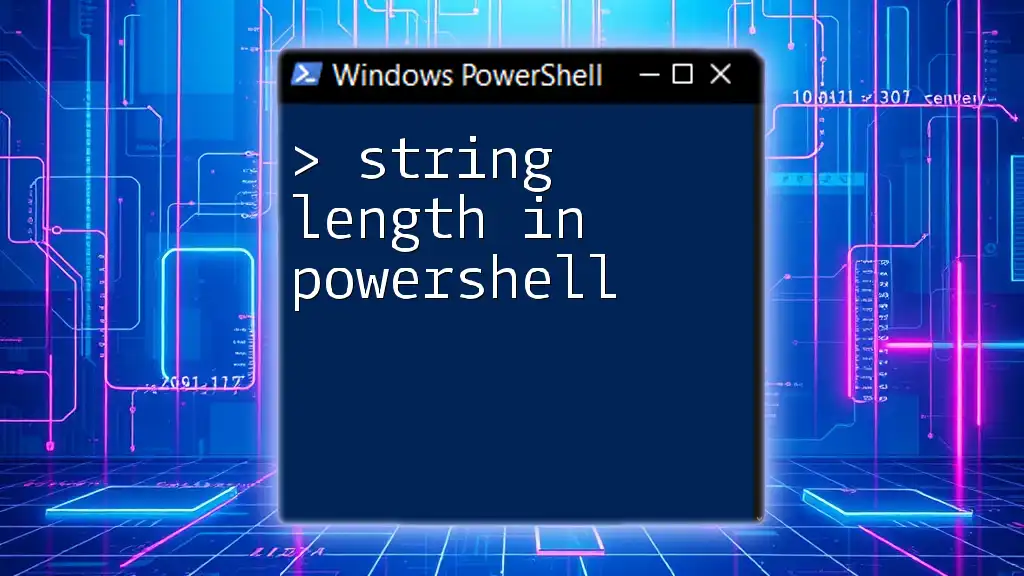
Common String Manipulation Techniques
Accessing Characters in a String
In PowerShell, you can access specific characters within a string using indexing. Strings are zero-based arrays, meaning the first character is at index 0. For example:
$string = "PowerShell"
$firstCharacter = $string[0] # Result: P
$thirdCharacter = $string[2] # Result: w
String Length
To find the length of a string, PowerShell provides the `.Length` property. This is useful when you need to perform operations based on the size of the string:
$length = $string.Length # Result: 10
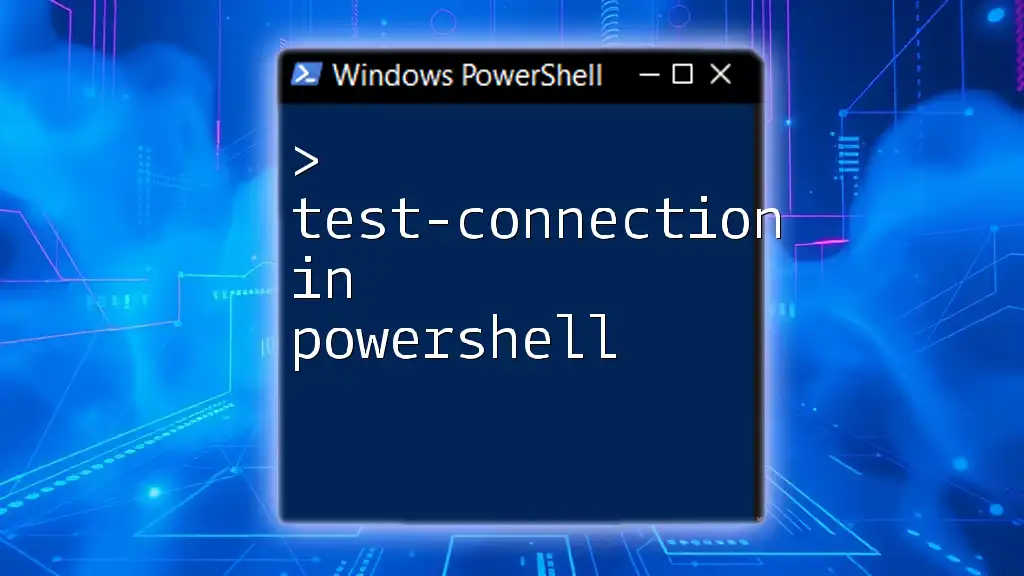
String Methods and Properties
Built-in String Methods
PowerShell comes equipped with several built-in string methods that make string manipulation intuitive and effective. Some commonly used methods include:
-
ToUpper(): This method converts all characters in the string to uppercase.
$upperString = $string.ToUpper() # Result: POWERSELL
-
ToLower(): Conversely, this method changes all characters to lowercase.
$lowerString = $string.ToLower() # Result: powershell
-
Trim(): Use this method to remove leading and trailing whitespace from a string.
$trimmedString = " Hello ".Trim() # Result: "Hello"
-
Substring(): This method returns a portion of the string based on specified starting index and length.
$subString = $string.Substring(0, 5) # Result: Power
String Interpolation
A powerful feature of PowerShell is string interpolation. This allows you to create dynamic strings that contain variable values directly. For example:
$name = "John"
$greeting = "Hello, $name" # Result: "Hello, John"
You can even include complex expressions by using the `$()` syntax:
$greeting = "Hello, $(Get-Date -Format 'dddd')!" # "Hello, Monday!" if today is Monday
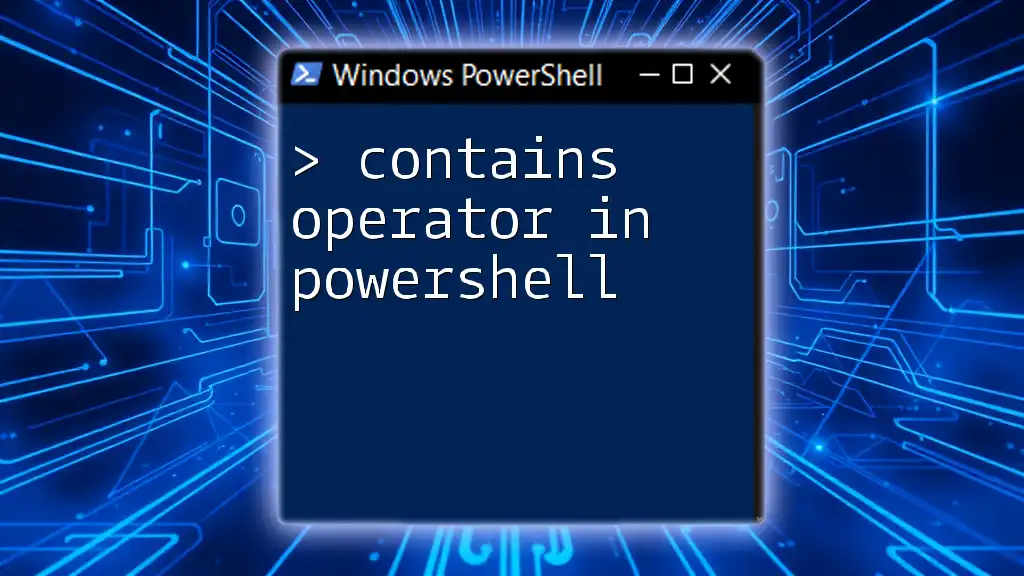
Advanced String Handling Techniques
Regular Expressions for String Manipulation
Regular Expressions (regex) provide a robust way to perform complex string manipulations. In PowerShell, you can use regex for tasks such as validation, searching, and replacing text patterns.
-
Introduction to Regex: Regular expressions are sequences of characters that form a search pattern. They are used with various PowerShell operators for string searching and replacements.
-
Using `-match` Operator: This operator allows you to check if a string matches a specific regex pattern. For example:
if ("abc123" -match "\d+") { "Contains numbers" } # Result: "Contains numbers"
-
Using `-replace` Operator: This operator is convenient for replacing text that matches a regex pattern. For instance:
$newString = "abc123" -replace "\d+", "456" # Result: "abc456"
Splitting and Joining Strings
PowerShell provides efficient methods for splitting and joining strings.
-
Using `-split` Operator: This operator separates a string into an array based on a specified delimiter. For example, you can split a list of fruits:
$fruits = "apple,banana,cherry" -split ","
After executing this snippet, `$fruits` will contain an array: `("apple", "banana", "cherry")`.
-
Joining Strings with `-join` Operator: Conversely, you can join elements of an array into a single string using this operator. For example:
$joinedString = $fruits -join "; " # Result: "apple; banana; cherry"
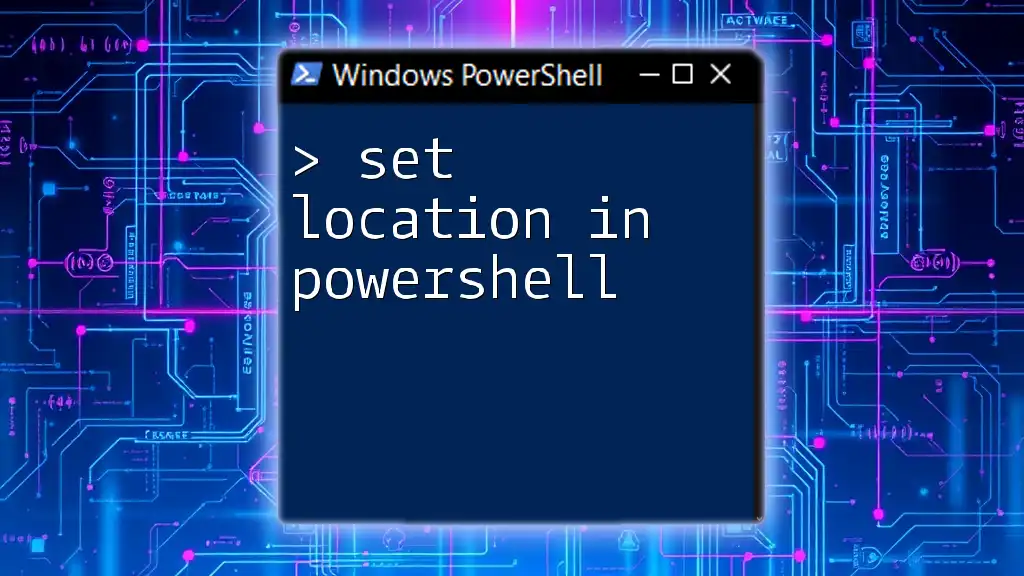
Error Handling in String Manipulations
Common Pitfalls
When manipulating strings, it is essential to handle potential errors gracefully. One common pitfall is working with null or empty strings. Always check for null or empty values to prevent runtime errors:
if (![string]::IsNullOrEmpty($string)) {
# Proceed with string manipulation
}
By incorporating these checks, you ensure your scripts are robust and less prone to fail due to unexpected string states.
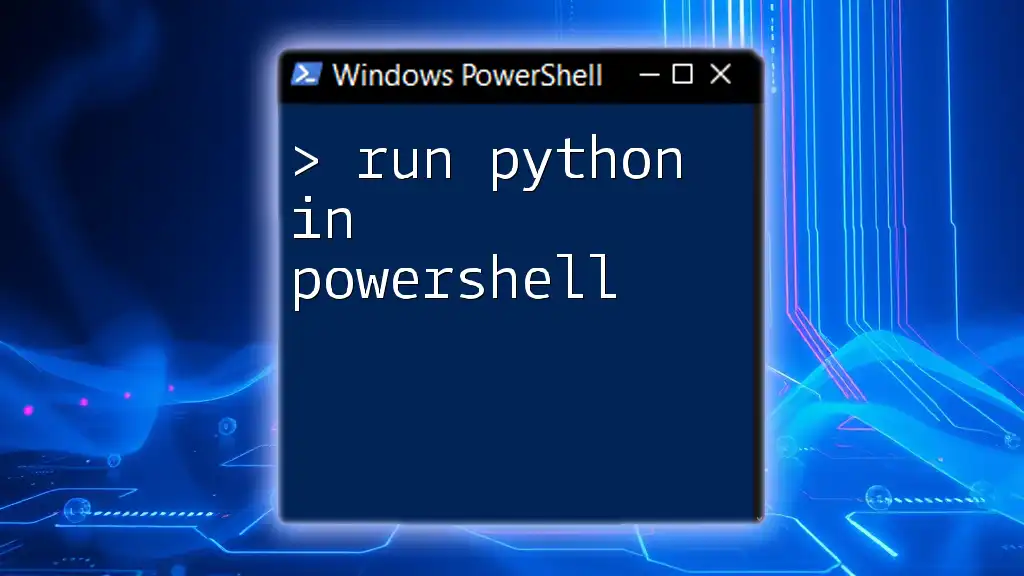
Conclusion
String manipulation in PowerShell is both powerful and essential for effective scripting. By mastering the various methods, properties, and techniques available for string handling, you can enhance your automation tasks and data processing capabilities.
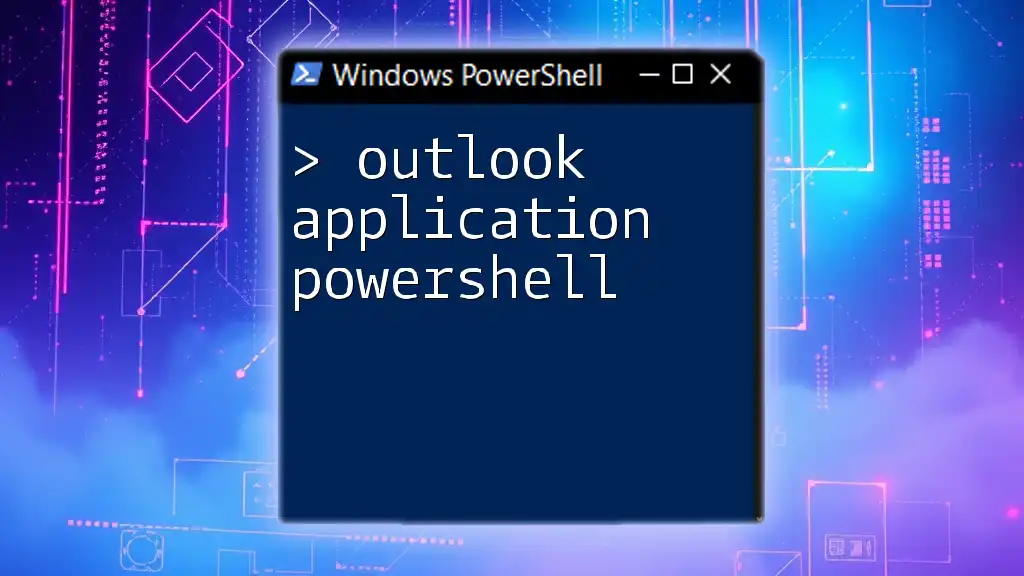
Additional Resources
For further exploration of string manipulation in PowerShell, consider reviewing the official PowerShell documentation on strings and exploring recommended books or online courses related to PowerShell scripting.
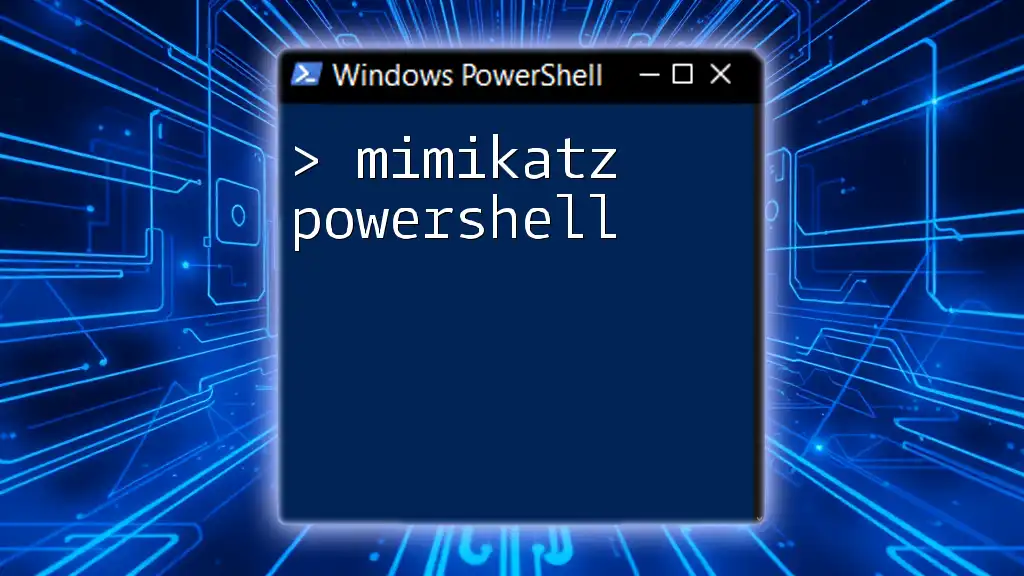
Call to Action
Have you tried any of these string manipulation techniques in your PowerShell scripts? Share your experiences or any additional tips in the comments! Don’t forget to check out our PowerShell courses for an in-depth look at mastering scripting skills!