The `-contains` operator in PowerShell is used to check whether a specified collection contains a particular value, returning `$true` if it does and `$false` otherwise.
Here's a code snippet illustrating its usage:
$fruits = 'Apple', 'Banana', 'Cherry'
$containsApple = $fruits -contains 'Apple'
Write-Host $containsApple # This will output: True
Understanding the Contains Operator
What is the Contains Operator?
The `-contains` operator in PowerShell is a useful feature that allows you to check if a particular item exists in a collection, such as an array or a hashtable. Its primary function is to determine membership, essentially verifying whether a specified value is part of a group.
This operator is especially valuable in scenarios involving data manipulation, where filtering or validating elements in collections is necessary. For instance, it can help you manage user lists, search through data, and streamline your scripts by avoiding unnecessary loops or condition checks.
Difference Between -contains and -like
While both the `-contains` and `-like` operators serve as mechanisms for checking membership, their applications are distinctly different.
-
`-contains`: This operator is used for checking whether a specific item exists within a collection. It works with discrete values and arrays. For example, if you have a collection of usernames, you can use `-contains` to see if a particular name is present.
-
`-like`: This operates based on wildcard characters and is used for pattern matching within strings. It’s beneficial for more flexible searches, such as verifying if a string starts, ends, or includes specific characters. For example, you might use `-like` to check if a filename starts with "report".
Example:
$array = @("Apple", "Banana", "Cherry")
$check1 = "Banana" -contains $array # Returns $false
$check2 = "Banana" -like "*Banana*" # Returns $true
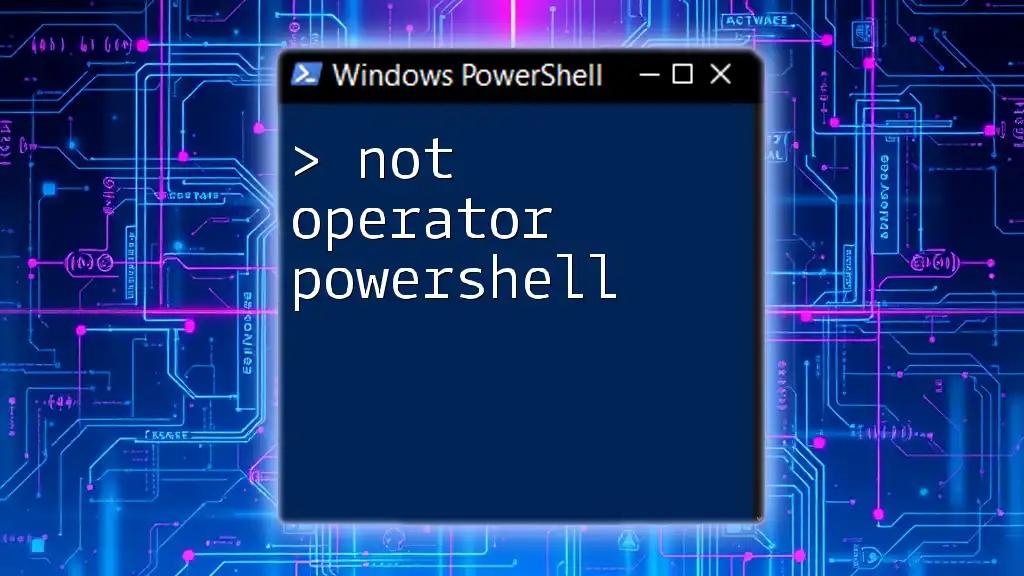
Syntax of the Contains Operator
Basic Syntax
The basic syntax of the `-contains` operator involves the item being checked on the left-hand side, followed by the `-contains` keyword, and then the collection on the right-hand side.
$element -contains $collection
Example Use Cases
To illustrate, consider a scenario where you want to check if a particular name exists within an array of names. The `-contains` operator allows you to do this succinctly.
Example:
$names = @("Alice", "Bob", "Charlie")
$result = "Bob" -contains $names
In this instance, `$result` will return `false`, as `-contains` checks whether `"Bob"` is an element of the `$names` array, which is not how the user meant to structure this check. Instead, you should use:
$result = $names -contains "Bob" # Returns $true
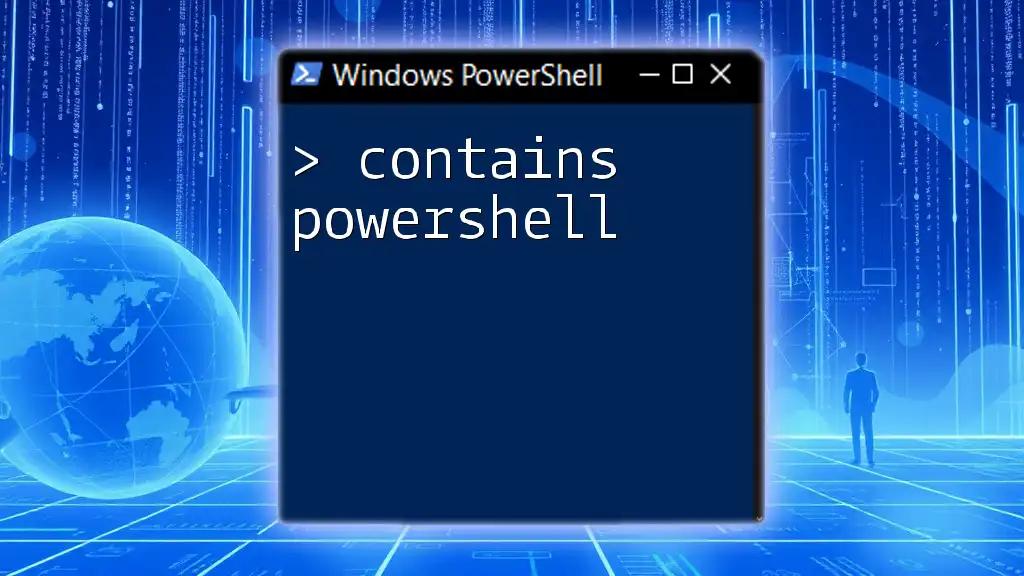
Practical Examples of Using the Contains Operator
Example 1: Checking for Values in Arrays
Scenario
You might want to verify if a specific user exists in an array of usernames.
Code Implementation
$users = @("Alice", "Bob", "Charlie")
$userExists = $users -contains "Bob" # Returns $true
In this example, you're checking if `"Bob"` is present in `$users`, resulting in `$userExists` being `true`.
Example 2: Filtering with the Where-Object Cmdlet
Scenario
Filtering user objects from an array based on their roles is another common application.
Code Implementation
$users = @(
@{Name="Alice"; Role="Admin"},
@{Name="Bob"; Role="User"},
@{Name="Charlie"; Role="Admin"}
)
$admins = $users | Where-Object { $_.Role -contains "Admin" }
In this code snippet, you filter the users to get only those with the role of Admin. The `Where-Object` cmdlet leverages the `-contains` operator to evaluate whether each user’s role matches.
Example 3: Nested Use of Contains
Scenario
You may find yourself needing to check if an array contains multiple items or if one array is a subset of another.
Code Implementation
$fruits = @("Apple", "Banana", "Cherry")
$desired = @("Banana", "Kiwi")
$hasDesiredFruit = $desired | Where-Object { $fruits -contains $_ }
Here, you are checking whether any of the `$desired` fruits exist within the `$fruits` array. The result will include `"Banana"`, showing that membership checks can be conducted in bulk.
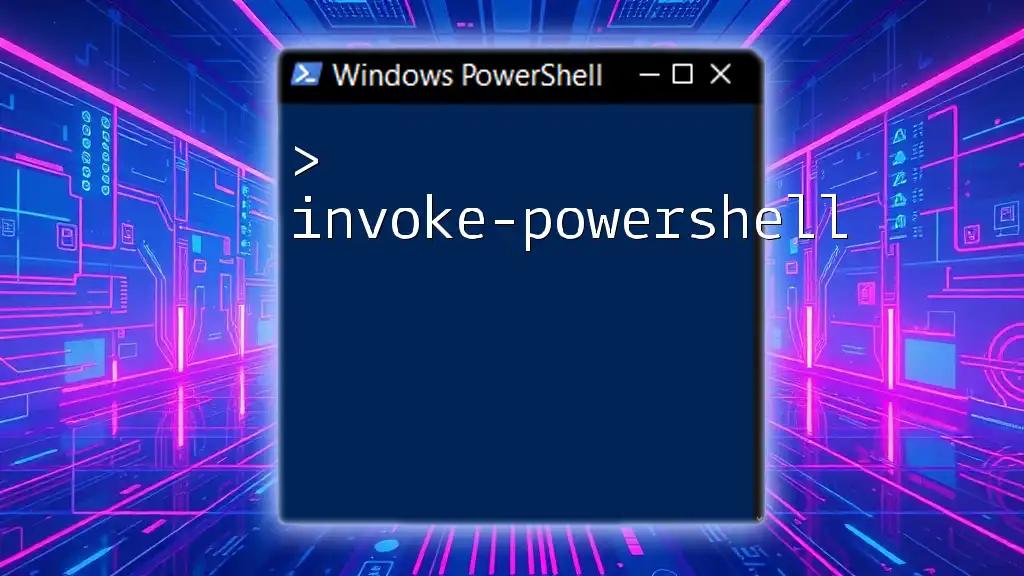
Common Pitfalls and How to Avoid Them
Case Sensitivity
It’s essential to note that the `-contains` operator is case-sensitive. This means that `"banana"` is not considered the same as `"Banana"`.
To effectively handle case sensitivity, you can convert both values to the same case—either all upper or lower—before the comparison.
Example:
$caseSensitiveCheck = "banana" -contains $names.ToLower() # This won't return true.
$result = $names.ToLower() -contains "banana" # This will return true.
Misunderstanding the Return Value
Another common misconception is around the return value of the `-contains` operator. It returns a Boolean value: either `$true` if the item exists in the collection or `$false` if it does not. Ensure you understand that it evaluates membership rather than providing the matched item.
Example:
$check = $users -contains "Alice" # Returns $true
$check = $users -contains "George" # Returns $false
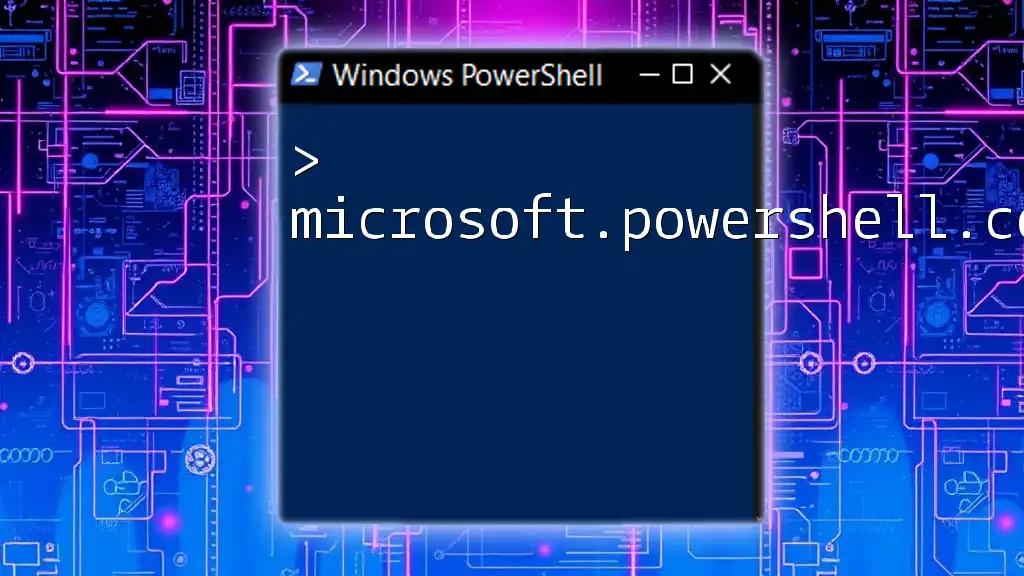
Best Practices for Using the Contains Operator
Structuring Your Code
Keep your PowerShell scripts clean and maintainable by adopting best practices. Use comments to describe the purpose of complex lines, and choose descriptive variable names for clarity.
Example:
# List of completed tasks
$completedTasks = @("Task1", "Task2", "Task3")
# Check if Task4 is completed
$isTask4Completed = $completedTasks -contains "Task4"
Performance Considerations
When dealing with arrays containing large datasets, performance may become a concern. The `-contains` operator generally performs well, but bear in mind that checking large arrays may exhibit slower performance. Instead, consider using hashed collections for faster membership checks if needed.
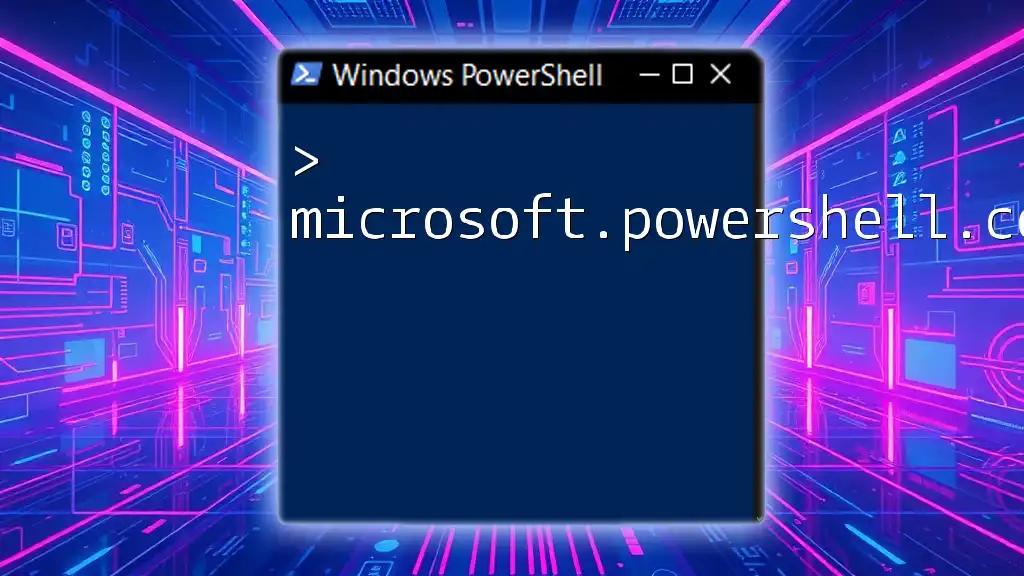
Conclusion
Mastering the contains operator in PowerShell is essential for effective scripting, particularly for data validation and manipulation. With its ability to simplify membership checks, the `-contains` operator is a powerful tool in your PowerShell arsenal. Whether you're managing user lists, filtering data, or refining conditions, this operator can greatly streamline your operations.
Further Reading and Resources
To expand your knowledge further, check the official PowerShell documentation on the `-contains` operator. Engage with community forums and consider taking advanced courses to deepen your understanding of PowerShell scripting.