To retrieve the size of a folder using PowerShell, you can utilize the following command that measures the total size of all files within the specified directory.
(Get-ChildItem "C:\Your\Folder\Path" -Recurse | Measure-Object -Property Length -Sum).Sum / 1MB
This command will return the folder size in megabytes.
Understanding Folder Size in PowerShell
What is Folder Size?
Folder size refers to the total amount of disk space that a specific directory and all of its contents (files and subdirectories) occupy on a disk. Knowing the folder sizes can help in effective disk management, as it allows users to identify which directories consume substantial space and need cleanup or attention.
Why Use PowerShell for This Task?
Using PowerShell to get folder sizes offers several advantages over graphical user interfaces (GUIs):
- Speed: PowerShell commands can quickly process large amounts of data without the delays associated with GUI applications.
- Automation: Once you have a script ready, you can automate folder size analysis and integrate it into scheduled tasks.
- Scripting Capabilities: PowerShell allows for more complex operations, such as logging results, fetching sizes across multiple directories, or even integrating alerts when a folder exceeds a certain size.
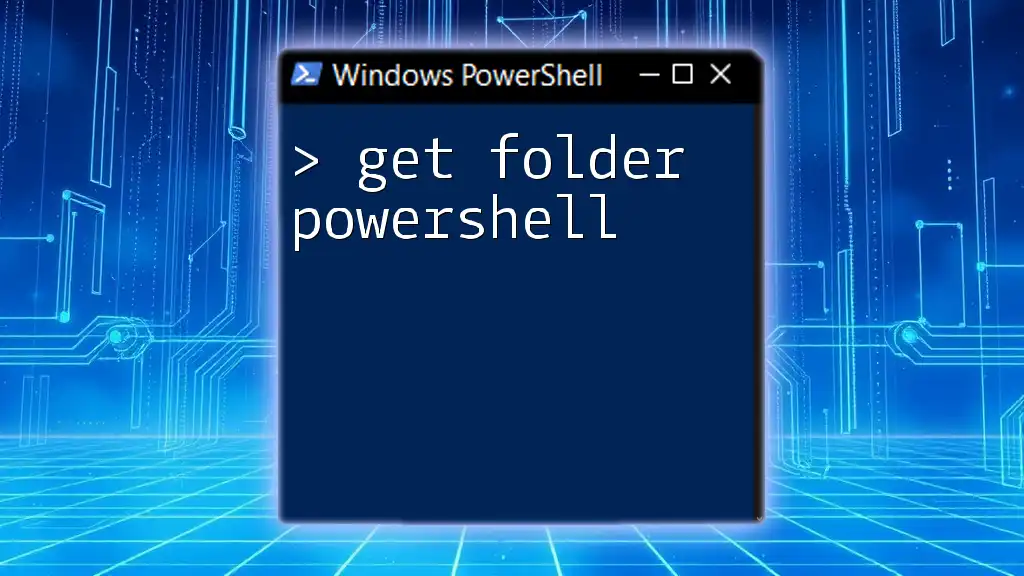
Basic Command to Get Folder Size
Using the Get-ChildItem Command
The primary command to retrieve folder sizes is `Get-ChildItem`, which lists all items in a specified directory.
To get the size of a specific folder, combine `Get-ChildItem` with the `Measure-Object` cmdlet to calculate the total length (size in bytes) of the files in that folder. Here’s a basic example:
Get-ChildItem "C:\YourFolder" | Measure-Object -Property Length -Sum
This command performs the following actions:
- Get-ChildItem: Retrieves all items in `"C:\YourFolder"`.
- Measure-Object: Sums the `Length` property of each file, providing a total size in bytes.
Understanding Output Values
When you run the command, it returns several properties, including `Count`, `Average`, and `Sum`. The value in `Sum` indicates the total size of the folder in bytes. This insight can help in quickly identifying space utilization.
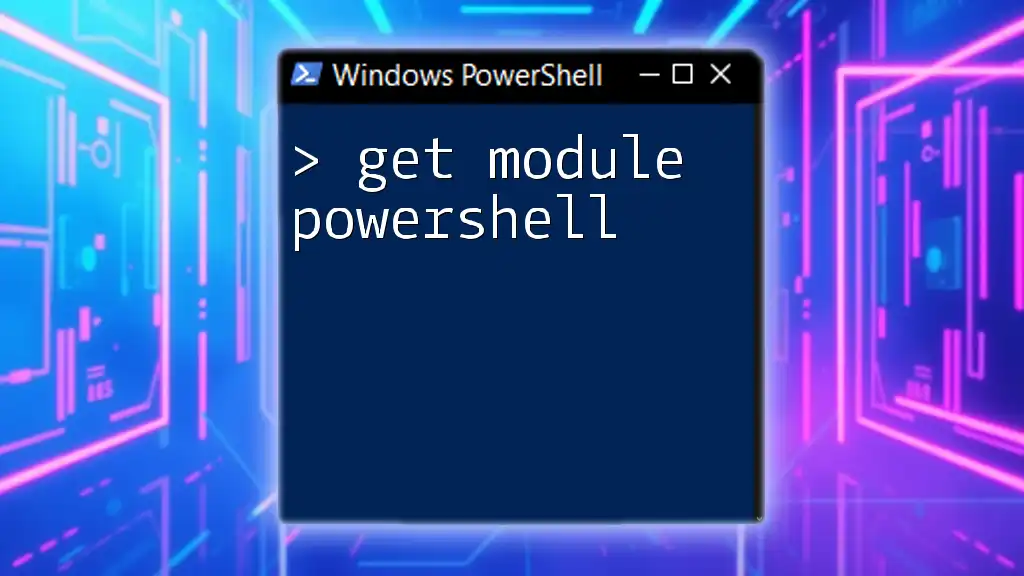
Getting Folder Size Including Subfolders
Using Recursive Commands
To get a true representation of a folder's size, including all its subfolders and their files, you can employ the `-Recurse` parameter. This instructs PowerShell to traverse through all the subdirectories.
Here’s how you could do it:
Get-ChildItem "C:\YourFolder" -Recurse | Measure-Object -Property Length -Sum
In this command:
- The `-Recurse` option makes sure that all subfolders are included in the size calculation.
Understanding the Recursive Process
When executing a recursive command, PowerShell explores each directory and its nested directories systematically. However, beware that calculating sizes for directories with a significant number of files can cause performance issues, especially if done frequently. It can be beneficial to limit the depth of the recursion; for instance, by processing just the first level of subfolders at times.
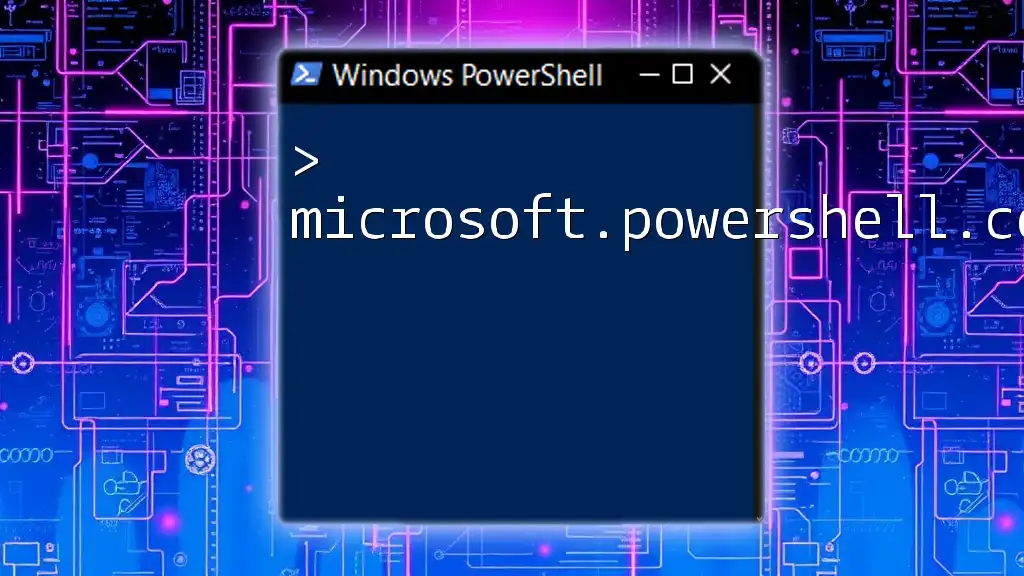
Formatting the Output
Human-Readable Format
Displaying folder sizes in bytes is not always user-friendly. It’s often more practical to convert these values into megabytes or gigabytes for easier interpretation.
To convert bytes to megabytes and format the output, you can use the following code:
$folderSize = (Get-ChildItem "C:\YourFolder" -Recurse | Measure-Object -Property Length -Sum).Sum
[math]::round($folderSize / 1MB, 2)
Here, we use `[math]::round()` to round the size to two decimal places, making it more digestible.
Displaying Folder Size with Additional Info
For better context, you might want to display the folder name alongside its size:
$folder = "C:\YourFolder"
$size = (Get-ChildItem $folder -Recurse | Measure-Object -Property Length -Sum).Sum
Write-Output "$folder size is: $([math]::round($size/1MB, 2)) MB"
This command declares a variable for the folder path and captures the calculated size, outputting a well-formatted message that presents both the folder name and its size in megabytes.
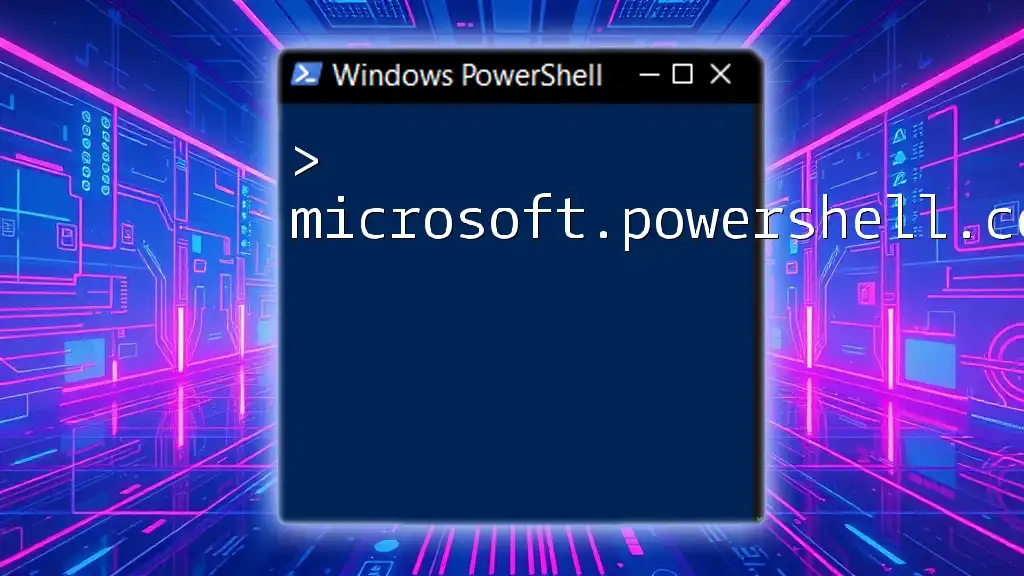
Advanced Techniques to Analyze Directory Sizes
Listing All Folders with Their Sizes
When managing multiple directories, you may want to list the sizes for all folders in a parent directory. Here is how you can do this:
Get-ChildItem "C:\YourParentFolder" |
ForEach-Object {
$size = (Get-ChildItem $_.FullName -Recurse | Measure-Object -Property Length -Sum).Sum
[PSCustomObject]@{ FolderName = $_.FullName; SizeMB = [math]::round($size / 1MB, 2) }
}
In this example:
- The `ForEach-Object` cmdlet processes each subfolder one at a time.
- A custom object is created capturing the folder's name and its corresponding size in megabytes, making the output very organized.
Sorting Folders by Size
You can easily sort the output of folder sizes by size:
Get-ChildItem "C:\YourParentFolder" |
ForEach-Object {
$size = (Get-ChildItem $_.FullName -Recurse | Measure-Object -Property Length -Sum).Sum
[PSCustomObject]@{ FolderName = $_.FullName; SizeMB = [math]::round($size / 1MB, 2) }
} | Sort-Object SizeMB -Descending
This command sorts the output in descending order by folder size, allowing you to quickly pinpoint which directories consume the most space.
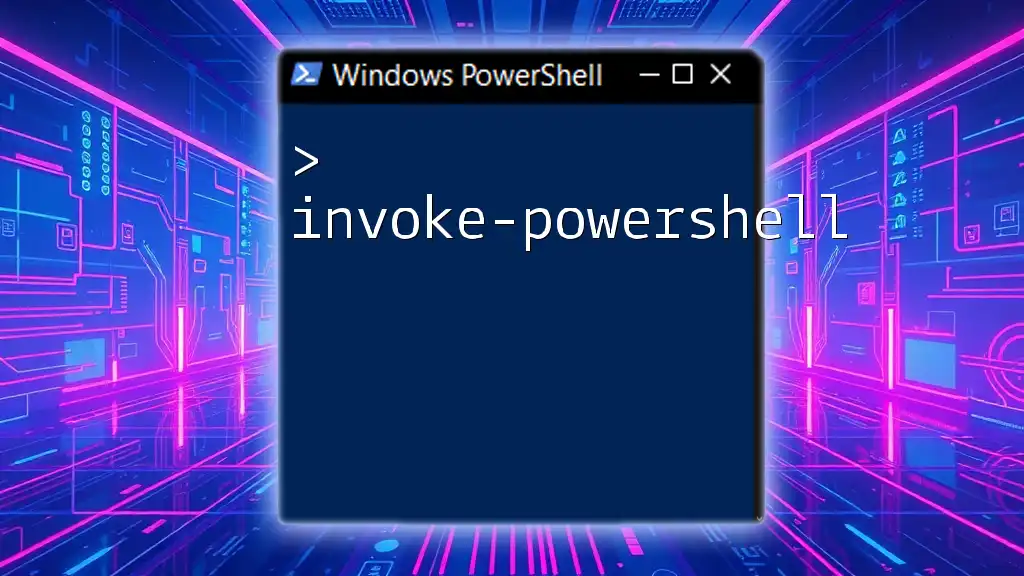
Common Errors and Troubleshooting
Handling Unauthorized Access Errors
Running commands that require access to certain files can sometimes lead to "Unauthorized Access" errors. To avoid this, run your PowerShell with Administrative privileges, enhancing your access rights when exploring system directories.
Empty Directories
If the specified directory contains no files, PowerShell may return a size of zero, which is typical. It’s essential to account for empty directories in your scripts if you wish to omit them from your results.
Performance Issues
When working with directories containing vast amounts of data, performance can take a hit. Test your scripts on smaller directories first and consider methods to limit the number of items processed or utilize parallel processing when necessary.
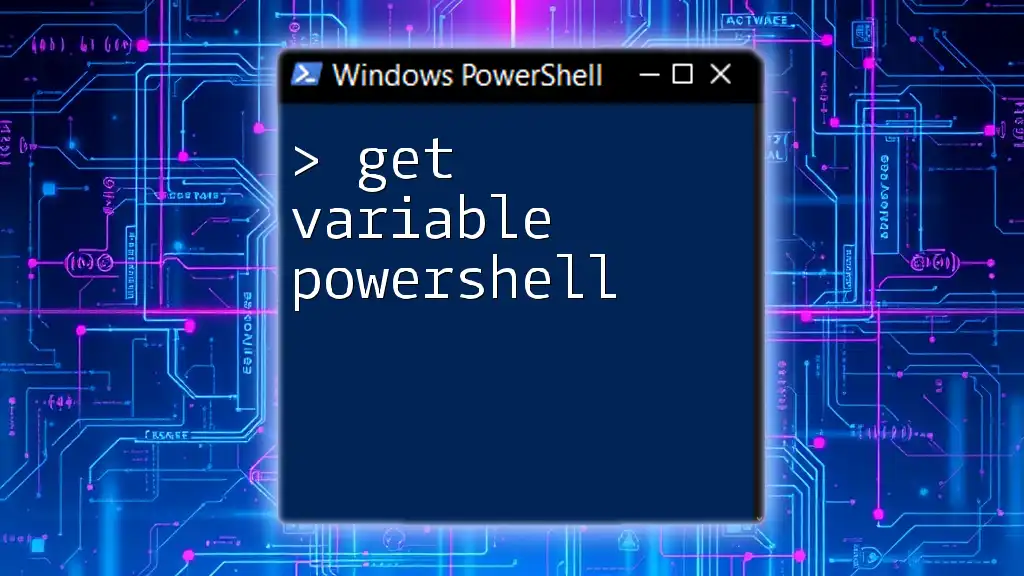
Conclusion
Understanding how to get folder size with PowerShell can significantly enhance your file management capabilities, allowing for effective organization and maintenance of disk space. By utilizing these commands and techniques, you can empower yourself to manage file systems more efficiently and intelligently. Don't hesitate to experiment with these PowerShell commands to find the best solutions tailored to your needs!
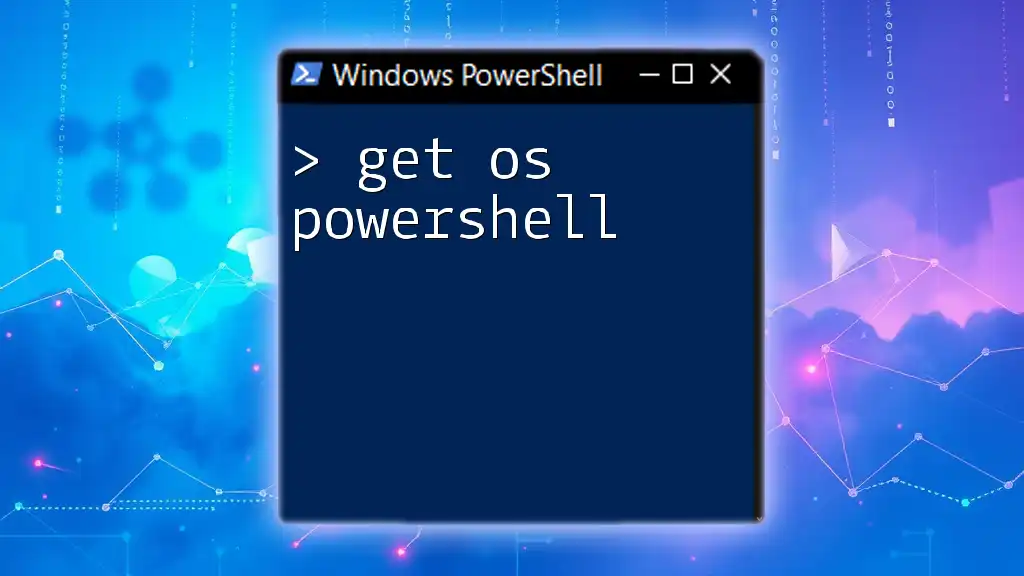
Additional Resources
For users interested in diving deeper into PowerShell, various online documentation and tutorials are available:
- The official [PowerShell documentation](https://docs.microsoft.com/en-us/powershell/)
- Community forums and user groups for practical tips and shared scripts.
- Books and online courses dedicated to mastering PowerShell.
By using these resources, you can continue to expand your skills and make the most of PowerShell’s powerful capabilities.