Home Assistant PowerShell allows you to automate and manage your home automation tasks efficiently using PowerShell cmdlets for seamless integration.
Invoke-RestMethod -Uri 'http://<your-home-assistant-url>/api/services/light/turn_on' -Method Post -Headers @{Authorization="Bearer <your-long-lived-access-token>"} -Body '{"entity_id": "light.living_room"}'
Setting Up Your Environment
Installing PowerShell
PowerShell on Windows
To get started with Home Assistant PowerShell, ensure you have PowerShell installed on your Windows system. PowerShell is included with Windows 10, but you can check the installed version by entering the following command in a PowerShell prompt:
$PSVersionTable.PSVersion
If you need to install or update to the latest version, visit the [PowerShell GitHub releases page](https://github.com/PowerShell/PowerShell/releases) for instructions.
PowerShell Core on Other Platforms
For Linux and macOS users, PowerShell Core allows you to use the same commands across different platforms. Install PowerShell Core by following the instructions available [here](https://docs.microsoft.com/en-us/powershell/scripting/install/installing-powershell-core-on-linux).
Setting Up Home Assistant
Installing Home Assistant
To harness the power of Home Assistant PowerShell, you’ll need a Home Assistant installation. Home Assistant can be installed on various platforms, such as Raspberry Pi, Docker, or even in a virtual environment. Visit the [official documentation](https://www.home-assistant.io/getting-started/) for detailed installation instructions tailored to your setup.
Accessing Home Assistant API
One of the standout features of Home Assistant PowerShell is the ability to interact with the Home Assistant API. This allows for programmatic control over your devices and states. To access the API, you'll need an API token, which can be obtained via the Home Assistant user interface in the Profile section. Store this token securely, as it grants access to your Home Assistant instance.
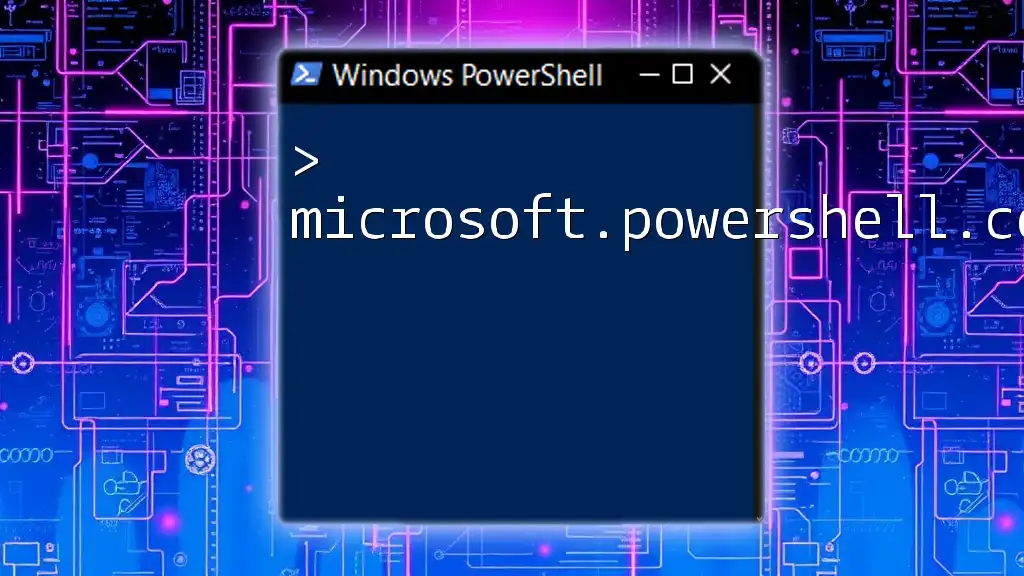
Basic PowerShell Commands for Home Assistant
Connecting to Home Assistant
Using Invoke-RestMethod
To issue commands to Home Assistant, you can use the `Invoke-RestMethod` cmdlet to interact with the API. Here’s how to make a basic GET request to fetch the current states of entities:
$url = "http://your-home-assistant-url:8123/api/states"
$token = "YOUR_API_TOKEN"
$headers = @{
"Authorization" = "Bearer $token"
"Content-Type" = "application/json"
}
$response = Invoke-RestMethod -Uri $url -Method Get -Headers $headers
$response
This command retrieves the state of all entities and stores it in the `$response` variable.
Understanding the Response
The response you receive is structured in JSON format, which is easy to work with in PowerShell. Each entity will include properties like `entity_id` and `state`. Use the following command to display a list of entity IDs and their states:
$response | Select-Object entity_id, state
Retrieving Entity States
Listing All Entities
Entities are the core of Home Assistant, representing various devices and components. You can simply print out the entire `$response` if you want to see all details, but if you only need some specific data, filter it using PowerShell.
Filtering Entities
Suppose you're interested specifically in light entities. You can filter them like this:
$lights = $response | Where-Object { $_.entity_id -like "light.*" }
This code will create a collection of light entities that you can manipulate later.
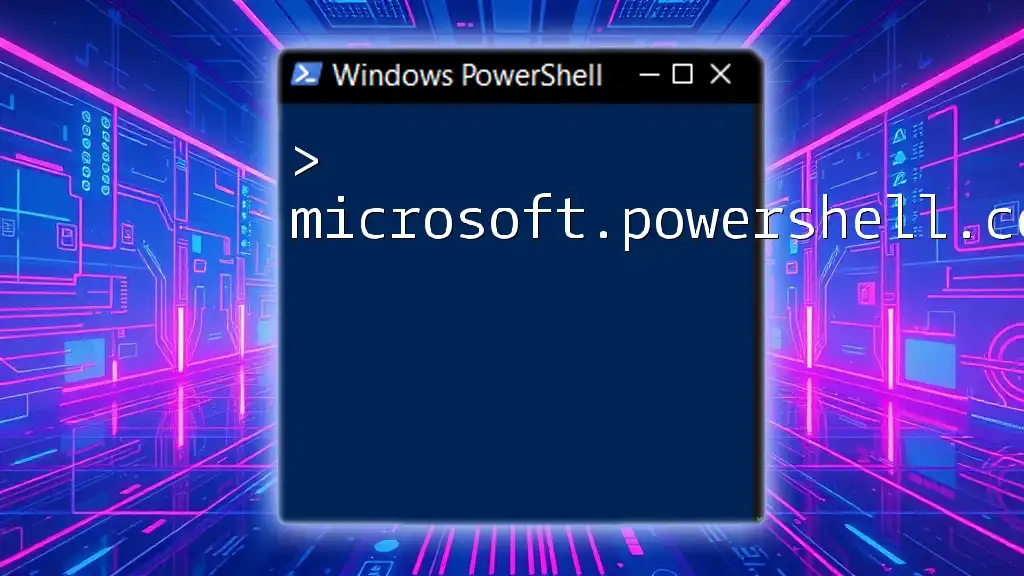
Automating Tasks with PowerShell
Controlling Smart Devices
Turning Devices On/Off
With PowerShell, you can issue commands to turn devices on or off. For example, if you want to turn a light on, you can use the following command:
$lightId = "light.living_room"
$body = @{
state = "on"
} | ConvertTo-Json
Invoke-RestMethod -Uri "http://your-home-assistant-url:8123/api/services/light/turn_on" -Method Post -Headers $headers -Body $body
This command sends a POST request to the relevant endpoint, instructing Home Assistant to turn on the specified light.
Adjusting Device Settings
You can also adjust settings like brightness or color. Here’s how you can dim the lights:
$body = @{
entity_id = $lightId
brightness = 100
} | ConvertTo-Json
Invoke-RestMethod -Uri "http://your-home-assistant-url:8123/api/services/light/turn_on" -Method Post -Headers $headers -Body $body
In this case, you define the `brightness` level and send it in the command.
Scheduling Tasks with PowerShell
Creating a Scheduled Task
If you want to automate tasks, you can create a scheduled task in Windows that runs PowerShell scripts at a specified time. Use the Task Scheduler to achieve this.
To create a task that runs a PowerShell script daily at 8 AM, you can use the following code:
$action = New-ScheduledTaskAction -Execute 'PowerShell.exe' -Argument '-File "C:\path\to\your\script.ps1"'
$trigger = New-ScheduledTaskTrigger -Daily -At 8AM
Register-ScheduledTask -Action $action -Trigger $trigger -TaskName "MorningLight" -User "SYSTEM"
This way, your PowerShell script will run automatically at the scheduled time.
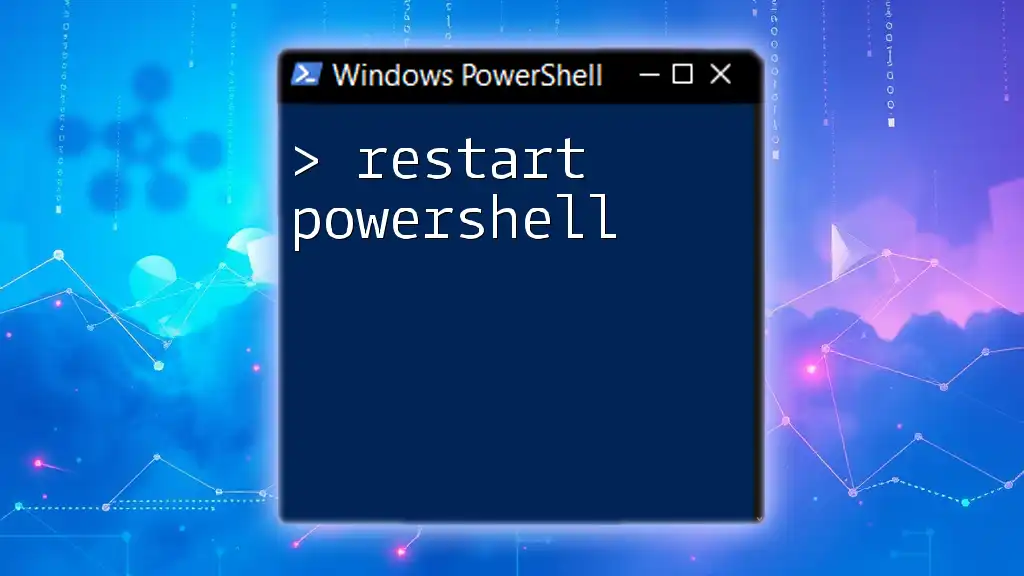
Troubleshooting Common Issues
Authentication Errors
Common Authentication Issues
If you encounter authentication errors when using Home Assistant PowerShell, check the following aspects: ensure that you're using the correct API token and that the token has permissions for the actions you're trying to perform.
Connection Problems
Network Connectivity
If you face connection issues, verify your network settings. Ensure that your Home Assistant instance is accessible from your PowerShell environment. Check firewall settings that might be blocking requests.
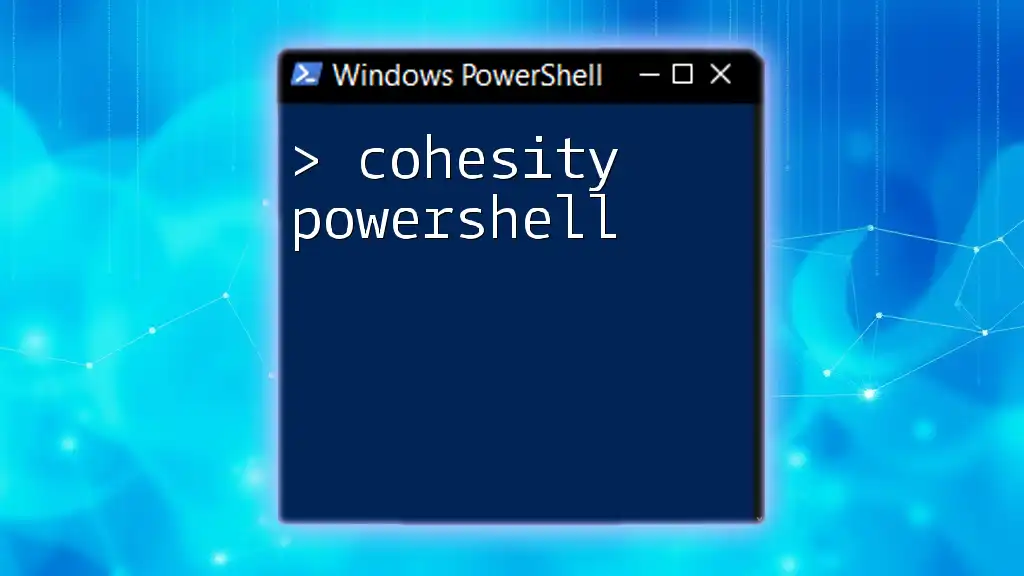
Advanced PowerShell Techniques
Using Functions to Wrap API Calls
Creating Functions for Reusability
To make your scripts cleaner and more manageable, wrap common API calls in functions. For example, here's a basic function for turning a light on:
function Turn-OnLight {
param (
[string]$entityId
)
$body = @{
entity_id = $entityId
state = "on"
} | ConvertTo-Json
Invoke-RestMethod -Uri "http://your-home-assistant-url:8123/api/services/light/turn_on" -Method Post -Headers $headers -Body $body
}
Incorporating Loops and Conditional Statements
Dynamic Control of Multiple Devices
If you want to take actions on multiple devices dynamically, use loops. For instance, to turn on all lights collected in your `$lights` variable, run:
foreach ($light in $lights) {
Invoke-RestMethod -Uri "http://your-home-assistant-url:8123/api/services/light/turn_on" -Method Post -Headers $headers -Body @{ entity_id = $light.entity_id } | ConvertTo-Json
}
This command iterates through each light entity and sends an API call to turn them on.
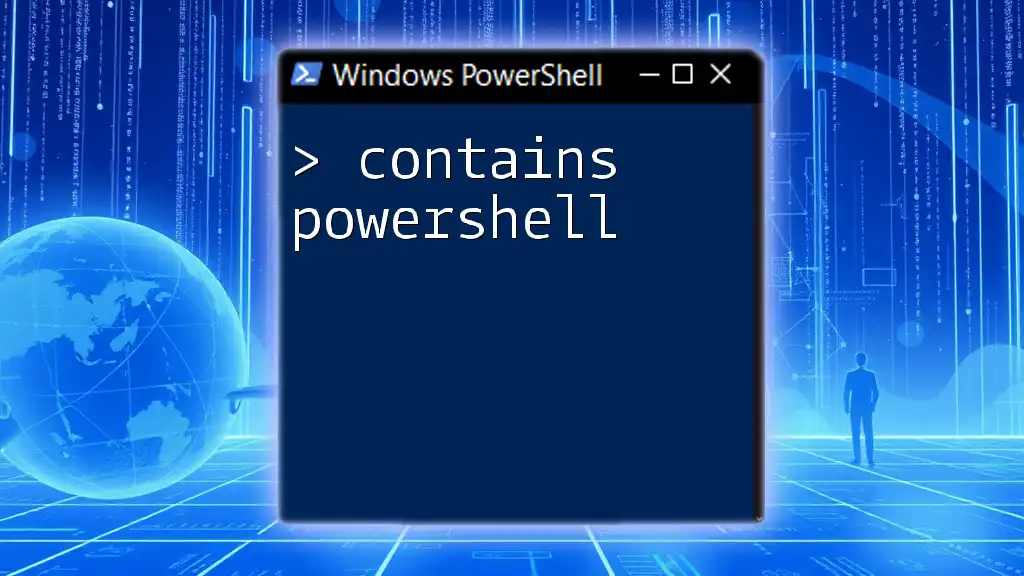
Conclusion
In summary, Home Assistant PowerShell provides an effective means to automate and control smart devices within your home. By setting up your environment, learning to connect with the API, and employing various PowerShell techniques, you can enhance your smart home experience significantly. Don't hesitate to dive deeper into the additional features of both PowerShell and Home Assistant as you continue to automate and streamline your tasks.
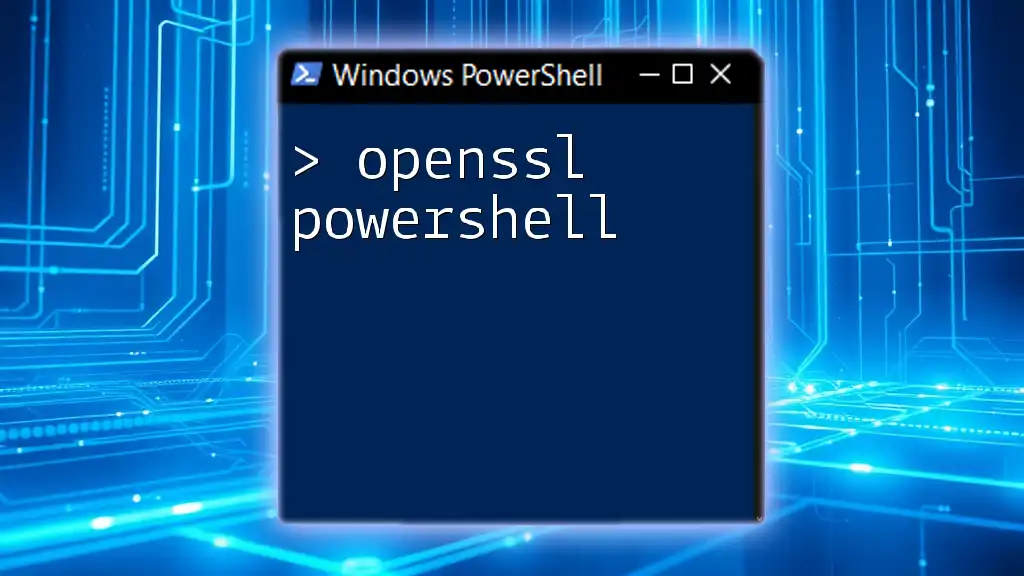
Additional Resources
For further learning, check out the official PowerShell documentation as well as the Home Assistant community forums where users share tips, scripts, and troubleshoot common issues.