Passing parameters to a PowerShell script allows you to make your script more dynamic and tailored to specific needs by providing inputs at runtime.
Here's a simple code snippet demonstrating how to pass a parameter to a PowerShell script:
param(
[string]$Name
)
Write-Host "Hello, $Name!"
To run this script and pass a parameter, you would use the command:
.\YourScript.ps1 -Name "Alice"
Understanding Parameters in PowerShell
What are Parameters?
In PowerShell, parameters allow you to pass data into your scripts, enabling dynamic behavior and enhancing the reusability of your code. They serve as inputs that define how your script should operate based on user specifications. Parameters can be categorized into two types:
- Mandatory Parameters: Must be provided to run the script successfully.
- Optional Parameters: Can be omitted, with default values assigned if necessary.
Types of Parameters
Named Parameters
Named parameters are specified using their names during script execution, allowing for clarity and flexibility. This approach reduces errors since parameters can be provided in any order.
Positional Parameters
Positional parameters are identified by their position in the command line. For example, if you have a script that accepts a name and an age, the first argument is interpreted as a name and the second as age.
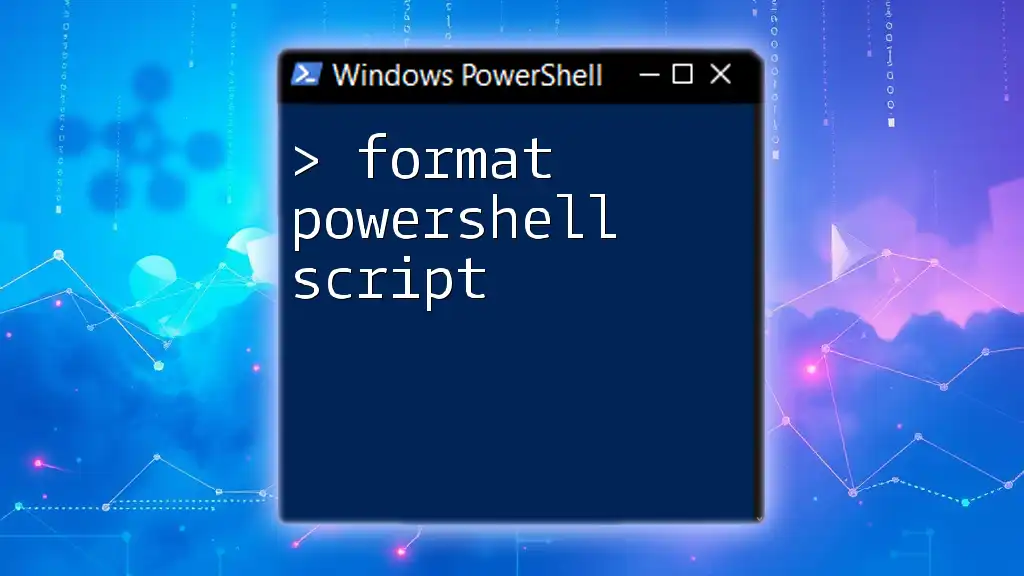
How to Pass Parameters to PowerShell Scripts
Basic Syntax for Passing Parameters
PowerShell scripts can accept parameters directly when invoked from the command line. The general syntax to pass parameters looks like this:
.\YourScript.ps1 -Parameter1 Value1 -Parameter2 Value2
To demonstrate, consider the following simple script named `ExampleScript.ps1`:
param (
[string]$Name,
[int]$Age
)
Write-Output "Hello, $Name! You are $Age years old."
You would execute it using:
.\ExampleScript.ps1 -Name "Alice" -Age 30
Using Positional vs. Named Parameters
Using positional parameters, you can skip the parameter names if you provide them in the correct sequence. For instance:
.\ExampleScript.ps1 "Bob" 25
Conversely, with named parameters, you explicitly specify parameter names, enhancing readability and flexibility:
.\ExampleScript.ps1 -Age 25 -Name "Bob"
Handling Default Values
You can assign default values to parameters, thus allowing a script to execute even if certain parameters are omitted. Modifying the previous example to include defaults would look like this:
param (
[string]$Name = "User",
[int]$Age = 18
)
Now, if you run `.\ExampleScript.ps1` without arguments, it will output:
Hello, User! You are 18 years old.
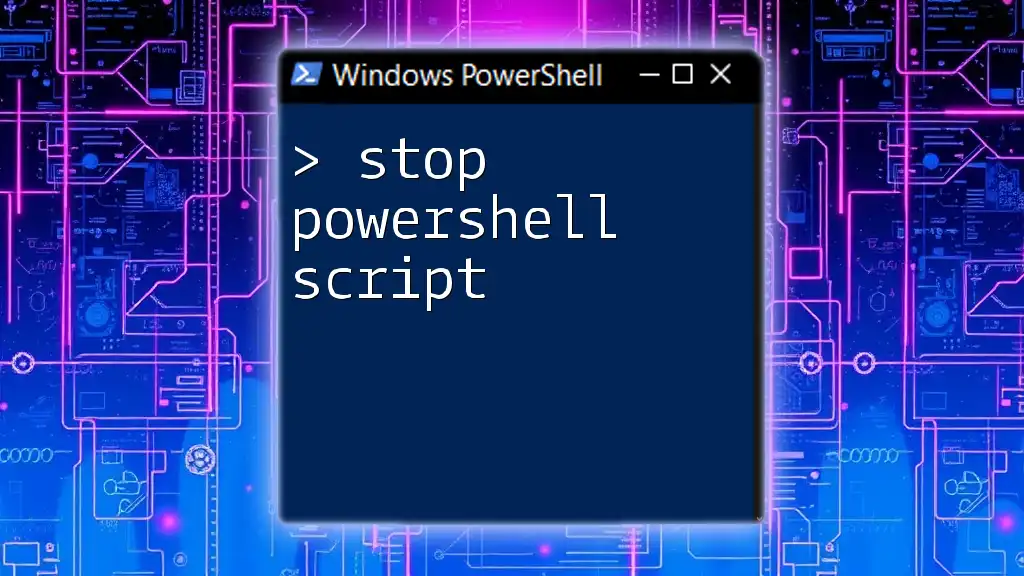
Advanced Parameter Features
Mandatory Parameters
To enforce that certain parameters must always be supplied, use the `Mandatory` attribute. This prevents the script from executing without the required input. Here’s how to define a mandatory parameter:
param (
[Parameter(Mandatory=$true)]
[string]$Name
)
If you attempt to run the script without providing a name, PowerShell will prompt you to input it.
Using Parameter Validation
PowerShell offers validation attributes that can be applied to parameters to ensure the data meets certain criteria. For instance, you can use `[ValidateSet()]` to restrict values to a predefined list, and `[ValidateRange()]` to define acceptable numeric ranges.
Here’s an example utilizing `[ValidateSet()]`:
param (
[ValidateSet("Admin", "User", "Guest")]
[string]$Role
)
This code snippet allows only the roles "Admin", "User", and "Guest" to be passed.
Similarly, you can validate numerical inputs with:
param (
[ValidateRange(1, 100)]
[int]$Age
)
Collecting an Arbitrary Number of Parameters
In scenarios where the number of inputs is variable, the `params` keyword can be handy. Here’s how to allow for an arbitrary number of string values:
param (
[string[]]$Names
)
This allows users to pass any number of names, such as:
.\YourScript.ps1 -Names "Alice", "Bob", "Charlie"
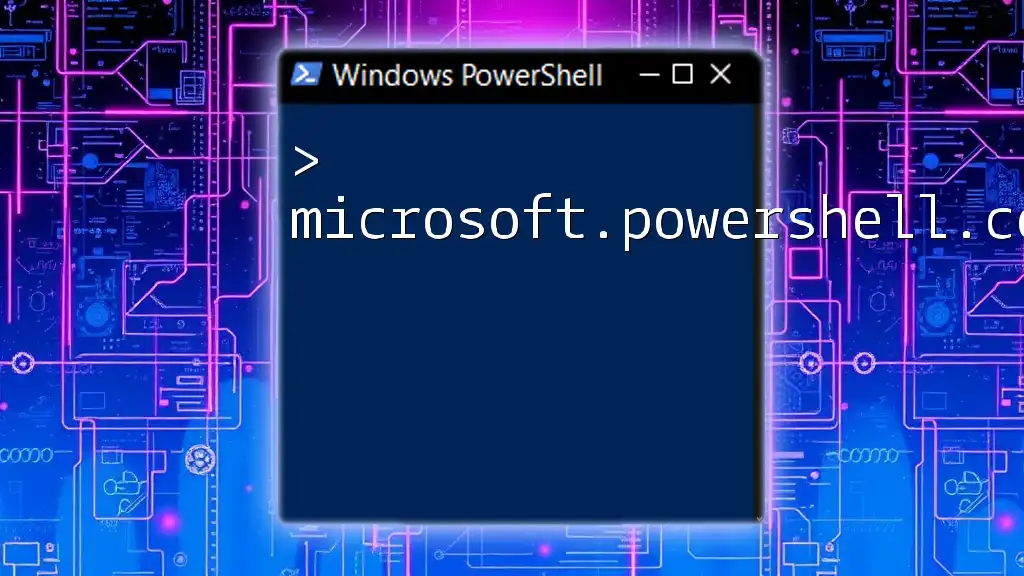
Good Practices for Passing Parameters
Documentation and Help
An essential best practice is to well-document your scripts. Using comments to describe each parameter ensures others (and your future self) can easily understand what each does. Moreover, leveraging the `Get-Help` cmdlet to provide built-in documentation can significantly aid users.
Consistency in Parameter Names
Choosing a consistent naming convention for parameters enhances code readability. Opt for descriptive names that clearly indicate their purpose, which can reduce confusion during script execution.
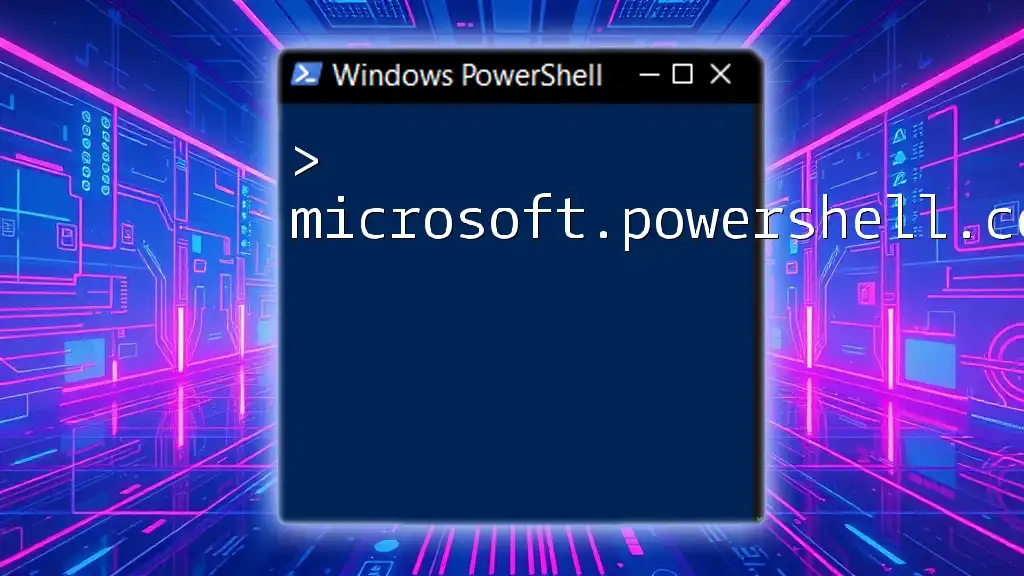
Debugging Parameter Passing Issues
Common Errors and Troubleshooting
When passing parameters, users may encounter various issues like type mismatches or omitted mandatory parameters. One common error occurs when a string is expected but a number is provided. For instance, if a script expects a string for `$Name`, supplying an integer could lead to a runtime error.
Leveraging `Try-Catch` for Error Handling
Utilizing `try-catch` blocks allows for structured error handling. This enables scripts to recover from errors gracefully and provide useful feedback.
Here's a simple example:
try {
# Assume some logic that might fail
$result = Do-Something -Parameter1 $input
} catch {
Write-Error "A parameter error occurred: $_"
}
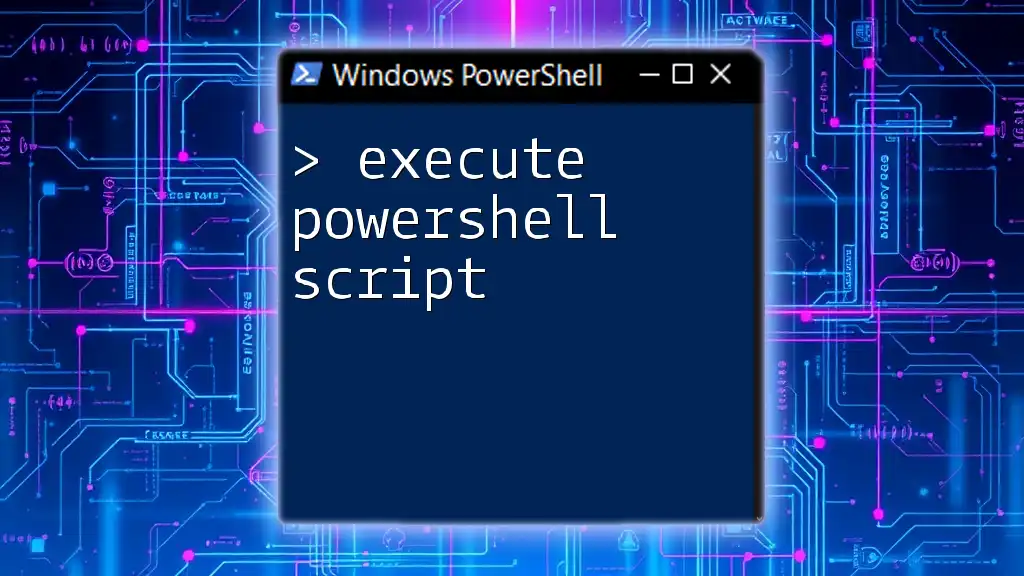
Conclusion
Passing parameters to PowerShell scripts is a fundamental skill that enhances the effectiveness and flexibility of your automation processes. By embracing parameters, you can create scripts that adapt to various input scenarios, making them powerful tools in your scripting toolkit.
Utilize the concepts outlined here, experiment with your own scripts, and continuously improve your parameter-passing skills to become more proficient in PowerShell. Consider this an ongoing journey—one that opens doors to automation and efficiency!
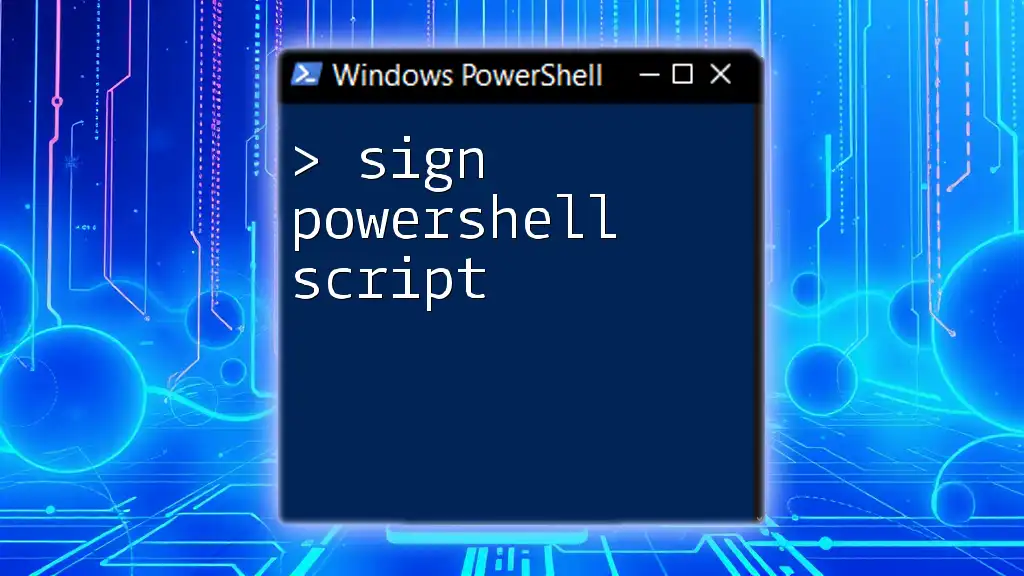
Additional Resources
As you further explore PowerShell, delve into community forums, blogs, and documentation to expand your knowledge. Continuous learning will empower you to excel in using this versatile scripting language.