To check if a string is empty in PowerShell, you can use the following command to determine if the variable contains any value:
if ([string]::IsNullOrEmpty($myString)) { Write-Host 'String is empty' } else { Write-Host 'String is not empty' }
Understanding Strings in PowerShell
What is a String?
In programming, a string is a sequence of characters used to represent text. In PowerShell, strings can be defined using single quotes (`'`) or double quotes (`"`). For instance:
$singleQuoteString = 'This is a single-quoted string.'
$doubleQuoteString = "This is a double-quoted string."
Both types of string declarations can include letters, numbers, symbols, and whitespace.
The Concept of Empty Strings
An empty string is defined as a string that contains no characters, indicated by two double quotes (`""`). It's crucial to distinguish between an empty string and a null value, which means that a variable has not been initialized or contains no data. Here’s how they differ:
- Empty String: `""`
- Null Value: `$null`
Understanding these distinctions is essential in scripting to avoid logical errors and unexpected behaviors.

Checking if a String is Empty
Using the `-eq` Operator
One of the simplest ways to check if a string is empty in PowerShell is to use the `-eq` operator. This operator checks if two values are equal. Here's how you can use it:
$string = ""
if ($string -eq "") {
"The string is empty."
}
In the example above, since `$string` is an empty string, the output is “The string is empty.”
Using the `String.IsNullOrEmpty()` Method
PowerShell provides a built-in method, `IsNullOrEmpty`, which is a part of the `String` class. This method checks both for null and empty values, making it a robust option for string validation. Here's how it works:
$string = $null
if ([String]::IsNullOrEmpty($string)) {
"The string is null or empty."
}
In this case, because `$string` is `$null`, the method returns true, confirming that the string is either null or empty.
Using the `-not` Operator
You can also check for a non-empty string using the `-not` operator with `IsNullOrEmpty`. This allows you to perform conditional logic based on whether a string contains data:
$string = "Hello, World!"
if (-not [String]::IsNullOrEmpty($string)) {
"The string is not null or empty."
}
Since the string contains text, the output confirms that it’s not null or empty.

Practical Examples
Example 1: Basic Check for Empty String
To illustrate the basic usage of checking for an empty string, consider the following PowerShell script:
$string = ""
if ($string -eq "") {
"The string is indeed empty."
}
The output will demonstrate a clear indication that the string is empty, reinforcing the concept.
Example 2: Handling User Input
Another practical application can be found when dealing with user inputs, where it's common to see if the entered string is empty:
$userInput = Read-Host "Enter something"
if ([String]::IsNullOrEmpty($userInput)) {
"You entered an empty string!"
}
When the user does not input any text and simply presses Enter, they will see the message that indicates an empty string was submitted.
Example 3: Conditional Logic Based on String Check
When incorporating string presence checks into scripts, you can build out more complex functionality. For instance:
$input = Read-Host "Type your name"
if (-not [String]::IsNullOrEmpty($input)) {
"Hello, $input!"
} else {
"You didn't enter anything!"
}
In this scenario, the script welcomes the user by name if they provided input, otherwise indicating that nothing was entered. It showcases how string checking can enhance user interaction.
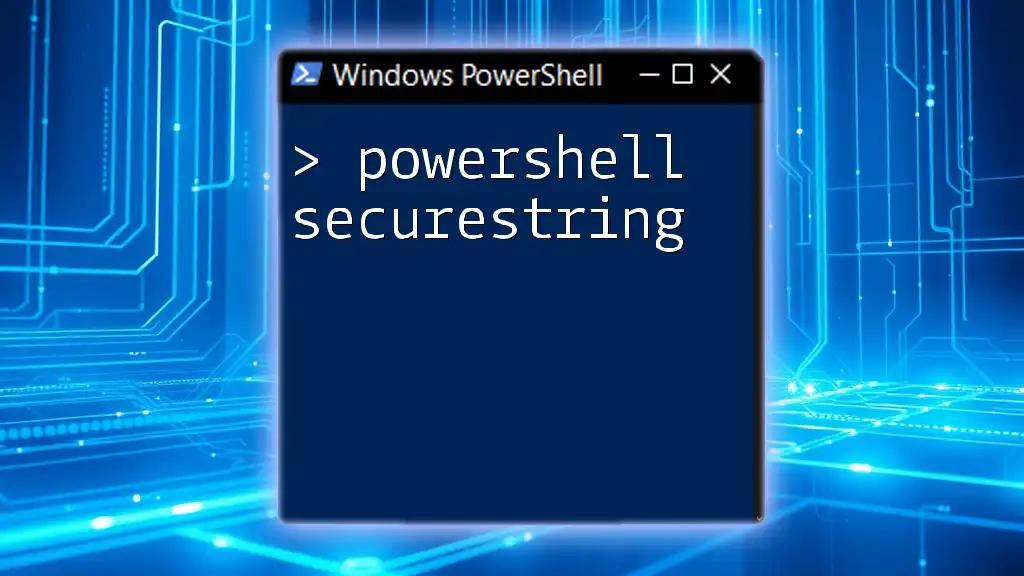
Common Mistakes
Forgetting to Check for Null
A frequent mistake when checking strings is neglecting to verify for null values. Failure to check whether a string is null can lead to null reference exceptions in scripts. Always incorporate checks like `IsNullOrEmpty` to guard against these issues.
Misusing String Comparison Operators
Another common pitfall is misapplying string comparison operators, such as using `-eq` for null checks without ensuring the variable is initialized. For example, the following code snippet can lead to errors:
$nullString = $null
if ($nullString -eq "") {
"This will throw an error."
}
Instead, use the `IsNullOrEmpty` method to prevent errors and clarify intent.
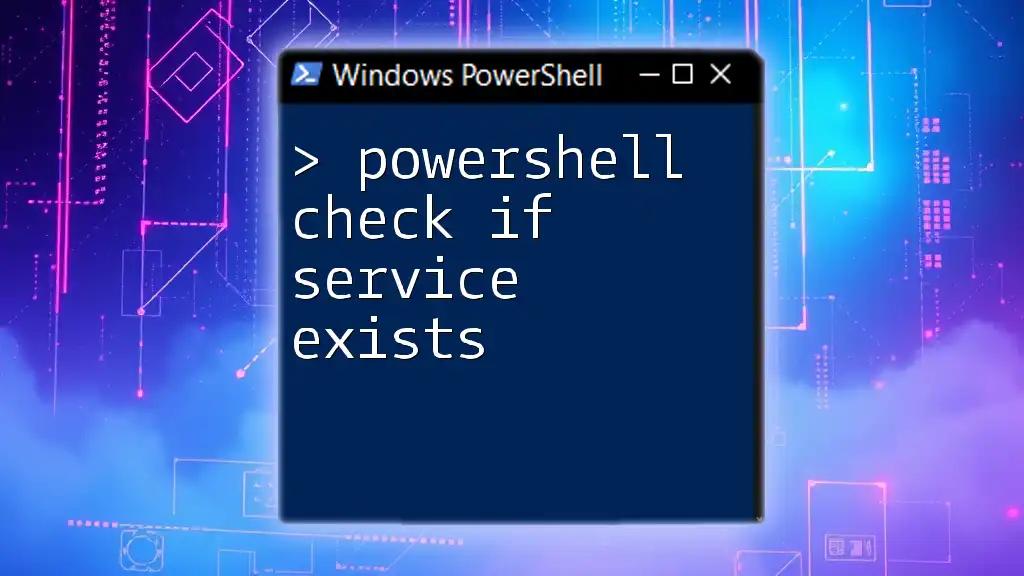
Conclusion
In summary, knowing how to check if a string is empty in PowerShell is a fundamental skill for scripting. Methods like `-eq`, `String.IsNullOrEmpty()`, and using the `-not` operator provide effective ways to validate strings. Proper handling of strings can significantly improve the reliability of your scripts while enhancing user experience.
By practicing these methods and learning from common mistakes, you’ll develop a deeper understanding of string manipulation in PowerShell. With mastery over checking for empty strings, you're one step closer to efficient and error-free scripting.

Further Resources
- For more detailed information, refer to the official PowerShell documentation.
- Explore additional tutorials and reading materials to deepen your PowerShell skills.
- Consider taking on exercises that involve string handling to apply what you’ve learned.
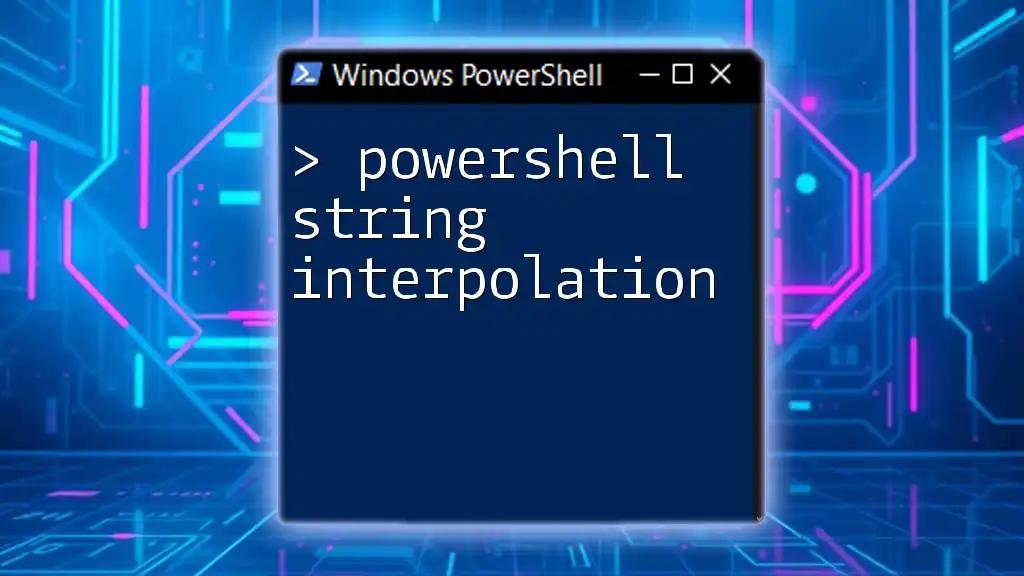
Call to Action
Share your experiences with PowerShell string handling in the comments below, or sign up for our courses to further develop your skills!