In PowerShell, the error "the string is missing the terminator" typically occurs when a string literal is not properly closed with matching quotation marks, leading to syntax errors in your script. Here's a simple example of the error and its correction:
# Incorrect usage leading to the error
Write-Host 'Hello, World! # Missing the closing single quote
# Correct usage
Write-Host 'Hello, World!'
Understanding Strings in PowerShell
Definition of Strings
In programming, a string is a sequence of characters. Strings are vital in programming languages like PowerShell, where they represent text, numbers, and other data types encapsulated within quotes. For example, you can create a string to hold a simple message or a complex piece of data.
Types of Strings
PowerShell primarily uses two types of strings:
Single-quoted Strings are used when you want to treat everything inside them literally. Special characters (like variables) are not interpreted. Here's how you declare a single-quoted string:
$singleQuoted = 'This is a single-quoted string'
In this example, `$singleQuoted` will store the text exactly as written, without evaluating any variables or escape characters.
Double-quoted Strings, on the other hand, allow for more complex interactions. Variables within double quotes are evaluated:
$name = "John"
$doubleQuoted = "Hello, $name!"
Here, `$doubleQuoted` would contain the value "Hello, John!" because PowerShell replaces `$name` with its value.
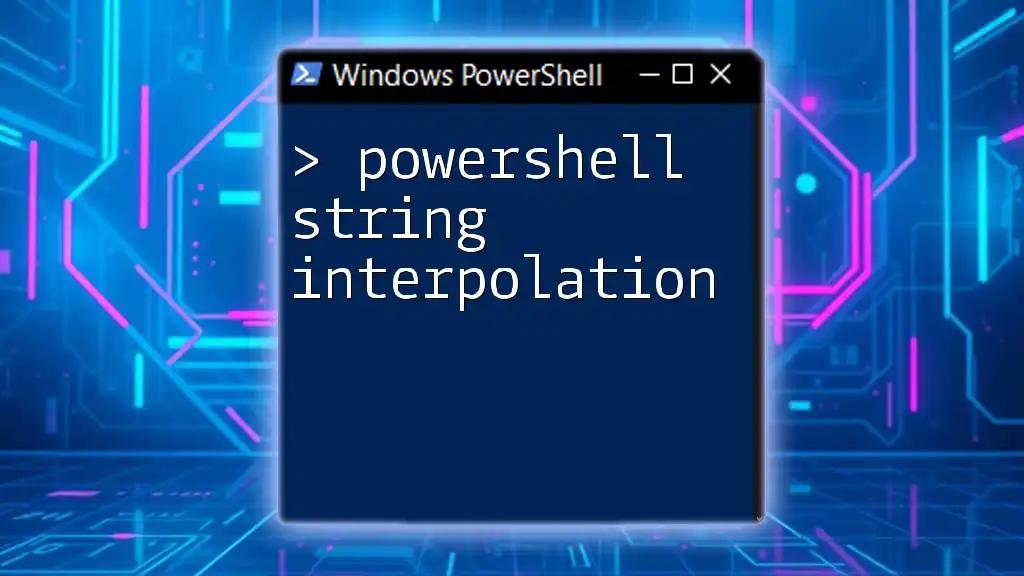
The Terminator Concept
What Are Terminators?
In the context of PowerShell strings, terminators are the closing quotes that signal the end of a string. Ensuring that strings have the correct opening and closing quotes is crucial for script integrity.
Common Terminator Types
Escape Characters—PowerShell utilizes backticks (`` ` ``) as escape characters to denote special sequences within strings. Escape characters allow you to include special characters without triggering their default behavior:
$escapedString = 'This is a string with an escape character: `n'
This string would include the text "This is a string with an escape character: " followed by a new line.
String Interpolation allows the use of variables within double-quoted strings. This feature is particularly powerful for dynamically inserting data into your strings:
$greeting = "Hello, $name!"
When executed, `$greeting` will yield "Hello, John!" if `$name` is set to "John".

Error: "The String is Missing the Terminator"
What Causes This Error?
The error message "The string is missing the terminator" appears when PowerShell detects a string that has an opening quote but cannot find a corresponding closing quote. This usually occurs due to typos, misspellings, or simply forgetting to close a string.
Identifying the Error
Recognizing the error message helps pinpoint issues in your scripts. A common scenario looks like this:
$missingTerminator = "This string does not have a terminator
In this example, PowerShell will throw an error because the opening double quote is not matched by a closing double quote.

How to Fix the Error
Basic Solutions
The most straightforward fix involves simply adding the missing terminator. This means ensuring every string has a proper closing quote.
$fixedString = "This string has a terminator"
Advanced Solutions
Sometimes, you may need to work with complex strings that contain additional quotes. In such cases, you can utilize Escape Sequences to avoid conflicts:
$quoteString = "The quote says, `"Hello, World!`""
Using the backtick here allows you to include the internal double quotes without confusing PowerShell.

Best Practices to Avoid Missing Terminators
Code Review Techniques
Regularly reviewing your code and identifying places where strings are declared is paramount. Utilize features within PowerShell, such as syntax highlighting in your IDE or command line editor, which can help you catch missing terminators instantly.
Consistent Quoting Style
Choose a quoting style—single or double quotes—based on your needs and maintain consistency throughout your script. This habit minimizes the friction of juggling between single and double-quoted strings and enhances readability.
Script Examples
Providing clear and comprehensive script examples allows for better understanding. For instance:
$exampleString = "This is a properly formatted string."
When executed correctly, this string maintains its integrity, and understanding its simplicity can bolster your scripting skills.

Conclusion
Properly managing string terminators is critical in PowerShell scripting. This ensures that your scripts run smoothly without unexpected errors like "The string is missing the terminator." Practicing these techniques and using the examples provided will help you gain confidence in handling strings effectively. Explore further resources to enhance your PowerShell expertise, and remember that consistent practice is key to mastering any programming language.