To remove trailing spaces from a string in PowerShell, you can use the `TrimEnd()` method as shown in the following code snippet:
$trimmedString = "Hello, World! ".TrimEnd()
Write-Host $trimmedString
Understanding Trailing Spaces
What Are Trailing Spaces?
Trailing spaces are any blank characters that follow the last visible character in a string. For example, in the string `"Hello World! "`, the four spaces after the exclamation mark are considered trailing spaces. Trailing spaces can often be unnoticed, leading to various issues, especially during data processing or string comparisons.
Why Trim Trailing Spaces?
Trimming trailing spaces is crucial for a variety of reasons. Common issues caused by trailing spaces include:
-
Data Integrity Problems: Trailing spaces can cause data mismatches and inconsistencies, especially when comparing strings or in database operations.
-
Errors in Comparisons and String Manipulation: If you compare strings that look the same but have trailing spaces, the comparison may yield false negatives.
-
Impact on File Sizes and Storage: While a few trailing spaces might seem trivial, they can accumulate in large datasets, leading to unnecessary increases in file size.
For instance, imagine a script that checks if a user’s name matches a predefined value. If the stored name ends with trailing spaces, the comparison will fail even if the visible characters match perfectly.
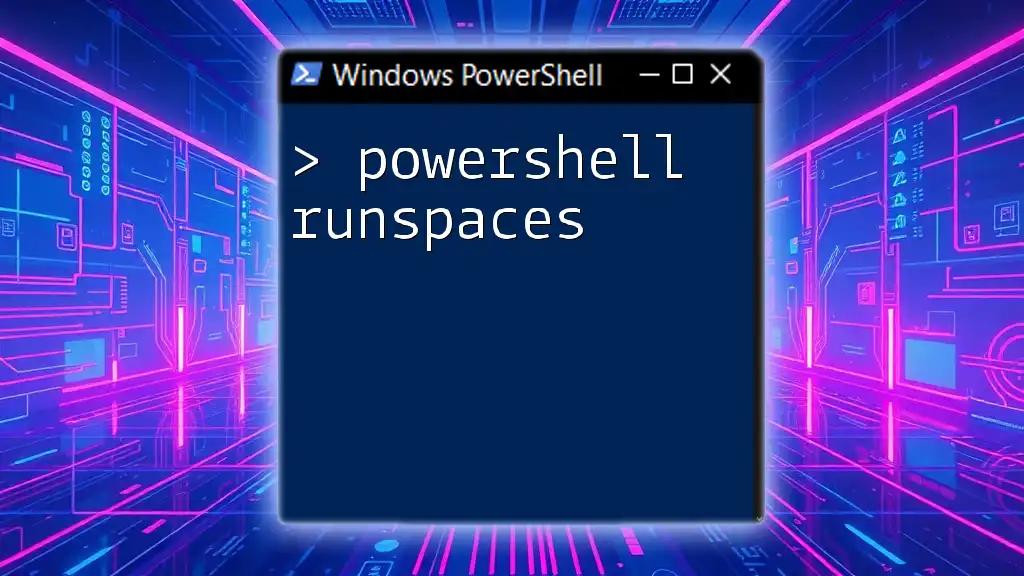
PowerShell Basics
Introduction to PowerShell String Manipulation
PowerShell provides robust capabilities for string handling, which includes methods and properties designed to manipulate strings efficiently. It is essential to be familiar with these features to effectively trim trailing spaces and maintain clean data.
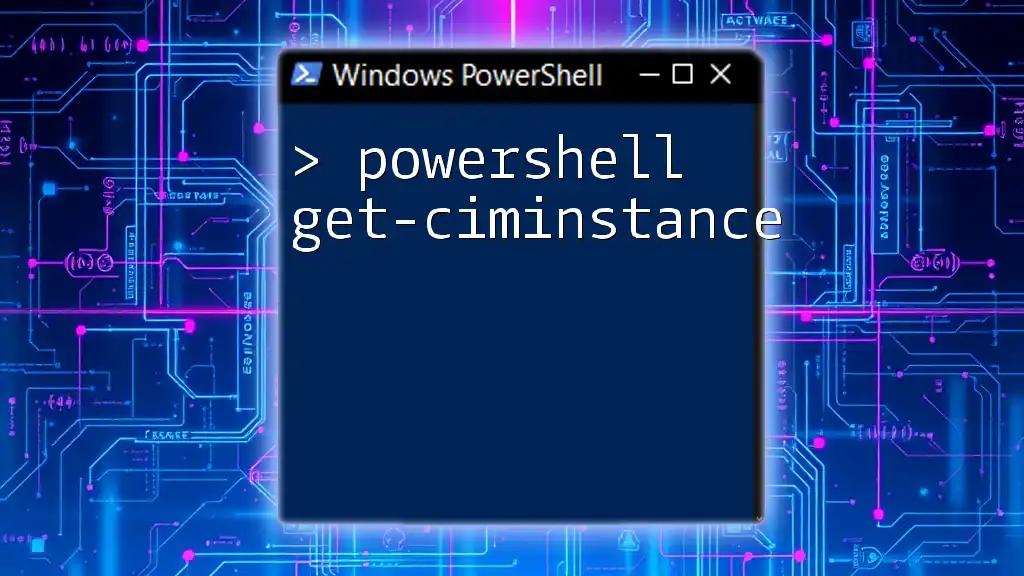
Methods to Trim Trailing Spaces
Using the `TrimEnd()` Method
The `TrimEnd()` method is specifically designed to remove trailing whitespace characters from a string. This method is straightforward to use and is a go-to method for many PowerShell users.
Syntax:
$trimmedString = $originalString.TrimEnd()
Example:
$stringWithSpaces = "Hello World! "
$trimmedString = $stringWithSpaces.TrimEnd()
Write-Output $trimmedString # Output: "Hello World!"
Explanation: In the above snippet, the original string has spaces at the end. By applying `TrimEnd()`, these spaces are removed, resulting in a clean string. This method is quick and reduce any risks of data mismatches due to unnoticed spaces.
Using Regular Expressions
Introduction to Regex in PowerShell
Regular expressions (regex) are a powerful tool for pattern matching in strings. They can be utilized to not only trim trailing spaces but also to handle more complex text processing requirements.
Example of Trimming Trailing Spaces with Regex
You can employ regex to trim trailing spaces as follows:
Code Snippet:
$stringWithSpaces = "Hello World! "
$trimmedString = $stringWithSpaces -replace '\s+$', ''
Write-Output $trimmedString # Output: "Hello World!"
Explanation: The `-replace` operator utilizes the regex pattern `'\s+$'`, which matches any whitespace character (`\s`) at the end of the string (`$`). This is an effective way to ensure the removal of all trailing spaces, regardless of how many there are.
Custom Function to Trim Trailing Spaces
Creating a custom function for trimming trailing spaces can encapsulate this functionality for reuse across scripts or modules.
Creating a Custom Function:
Function Trim-TrailingSpaces {
param (
[string]$inputString
)
return $inputString.TrimEnd()
}
Example Usage:
$result = Trim-TrailingSpaces "Hello World! "
Write-Output $result # Output: "Hello World!"
Explanation: This custom function enables you to easily remove trailing spaces. By encapsulating the logic, you can maintain cleaner scripts and improve maintainability.
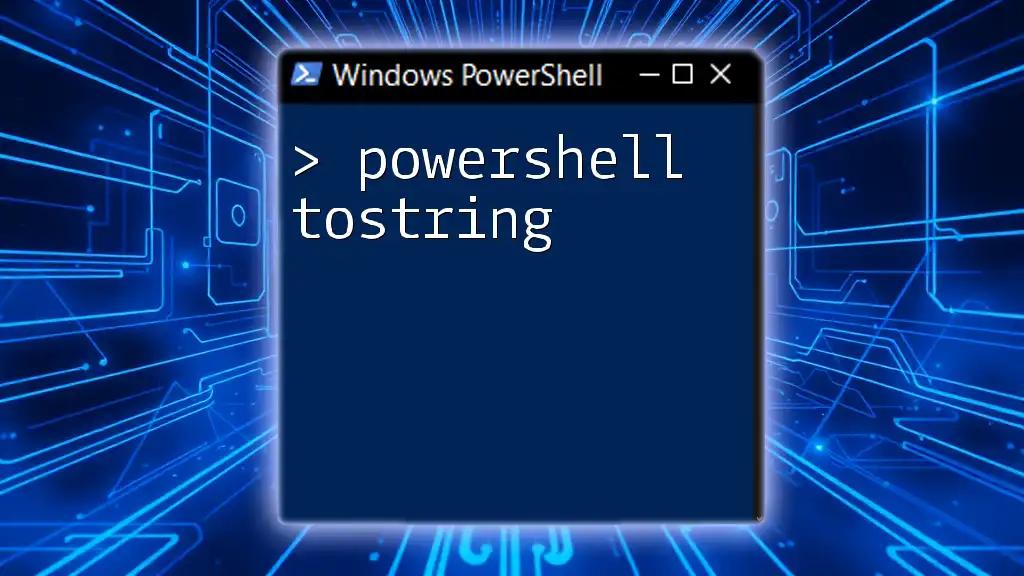
Practical Applications
Data Cleaning in CSV Files
Data quality is essential when processing large datasets. Trailing spaces can mask issues and skew results. If you have a CSV file and want to ensure it’s clean, one effective solution is to trim trailing spaces.
Example:
Import-Csv .\data.csv | ForEach-Object {
$_.Name = $_.Name.TrimEnd()
$_
} | Export-Csv -Path .\cleaned_data.csv -NoTypeInformation
Explanation: This snippet imports a CSV file, processes each record by trimming trailing spaces from the 'Name' field, and exports the cleaned data back to a new CSV file. This kind of data cleaning is essential to maintain the integrity of information.
User Input Scenarios
When prompting users for input, it’s common for them to inadvertently enter trailing spaces. This can lead to complications, especially when processing or storing the input.
Example:
$userInput = Read-Host "Enter your name"
$cleanedInput = $userInput.TrimEnd()
Write-Output "Your name is: $cleanedInput"
Explanation: By trimming the user input upon entry, you can ensure that the processed name matches expected formats without unintended trailing spaces, hence improving user experience and accuracy in data handling.
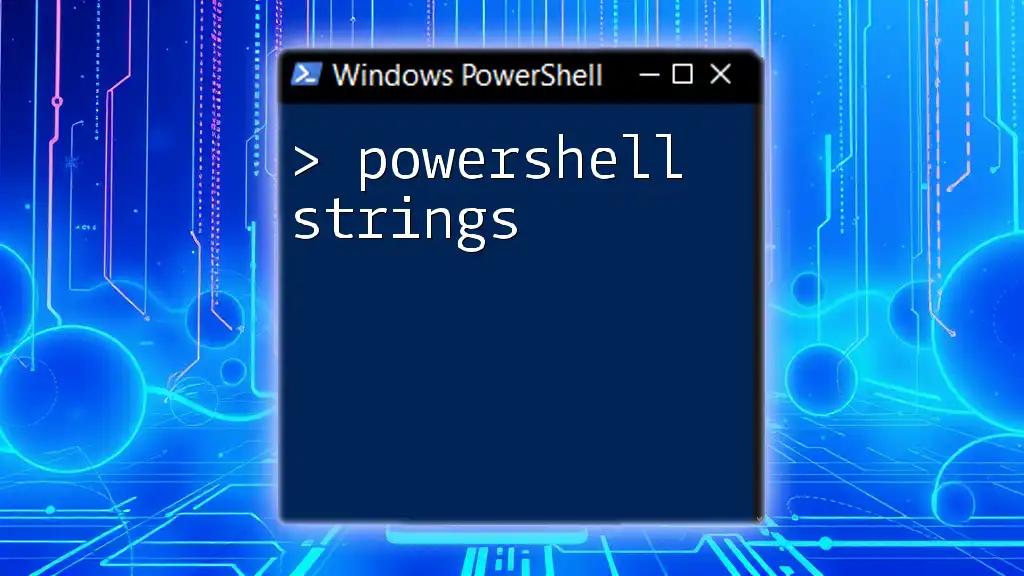
Common Pitfalls and Best Practices
Avoid Over-Trimming
One should be cautious not to over-trim spaces when formatting data. In some cases, trailing spaces might be intentional and meaningful, such as in formatted data strings. Always assess the context before implementing trimming operations.
Performance Considerations
Choosing between string methods and regex can impact performance. Using built-in methods like `TrimEnd()` is generally faster than regex patterns, especially when working with large datasets. Opt for methods when the operation is straightforward, and reserve regex for more complex scenarios.
Debugging Tips
When dealing with trailing spaces, debugging might help you identify hidden issues. One effective practice is to visualize string lengths using the `.Length` property to diagnose where unexpected spaces might lie.
$userInput = "Hello World! "
Write-Output "Length of input: $($userInput.Length)"

Conclusion
Trimming trailing spaces is an essential practice for ensuring data integrity and accuracy in PowerShell scripting. By understanding the various methods available—ranging from the `TrimEnd()` method to using regular expressions—you can effectively manage strings in your scripts. It’s vital to regularly apply these techniques to enhance your PowerShell skills and the quality of your data.
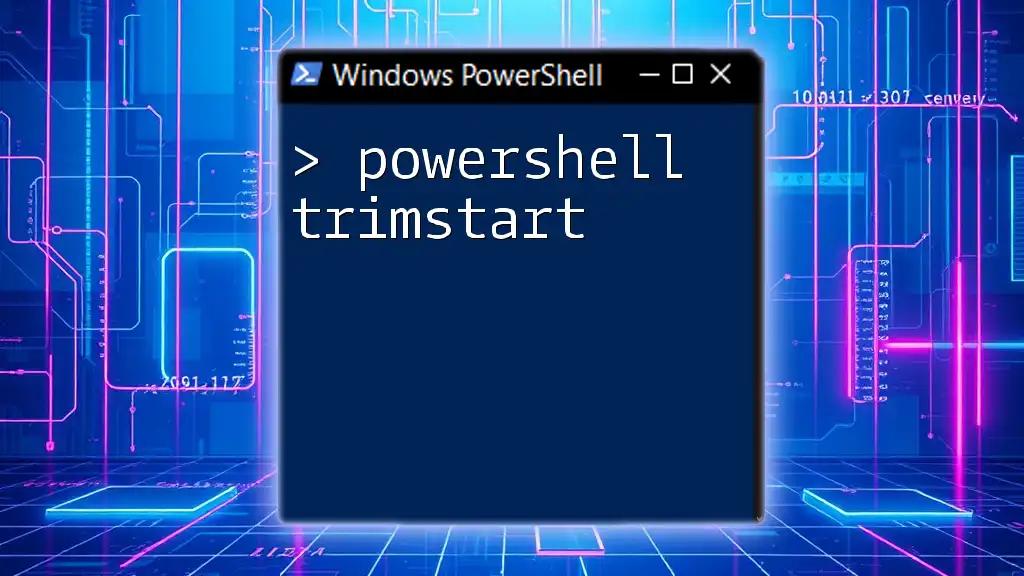
Call to Action
To learn more about effective PowerShell practices, subscribe to our updates and follow us on social media for additional tips and resources!