To check if a service exists in PowerShell, you can use the `Get-Service` cmdlet and verify if it returns any output for the specified service name.
$serviceName = "YourServiceName"
if (Get-Service -Name $serviceName -ErrorAction SilentlyContinue) {
Write-Host "$serviceName exists."
} else {
Write-Host "$serviceName does not exist."
}
Understanding Windows Services
What are Windows Services?
Windows services are applications that run in the background of the operating system, typically without a user interface. They can start automatically at boot time, run continuously, or activate in response to events. Services are crucial for managing system operations and running business applications efficiently.
The Relevance of Managing Services
Being able to identify whether a specific service exists on a system is vital for several reasons. For system administrators, scripts that check for service existence can help in troubleshooting, automating deployment processes, and ensuring that essential services are operational. For instance, if a service is critical for an application and it is not running, it could lead to significant downtime, affecting productivity.

PowerShell Basics
What is PowerShell?
PowerShell is a task automation framework from Microsoft, consisting of a command-line shell and an associated scripting language. It provides the ability to automate administrative tasks and manage system configurations. PowerShell is built on the .NET framework, giving it the capability to interact with various components of Windows and other systems.
Getting Started with PowerShell
To begin using PowerShell, simply follow these steps:
- Open the Start menu.
- Type "PowerShell."
- Select "Windows PowerShell" or "PowerShell (Admin)" for elevated privileges.
Understanding the basic syntax is essential, as it allows you to construct effective commands. A PowerShell command typically follows the Verb-Noun format, such as `Get-Service`, where "Get" is the verb indicating the action.
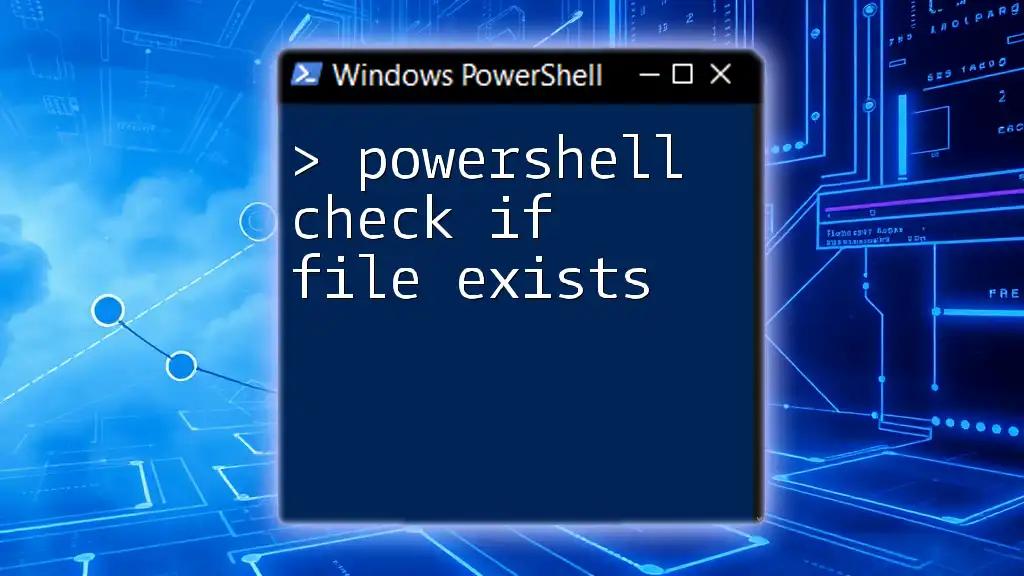
Checking if a Service Exists in PowerShell
Overview of the `Get-Service` Cmdlet
The `Get-Service` cmdlet is a built-in command in PowerShell that retrieves the status of services on a Windows machine. You can use this cmdlet to check if a service exists, its status (running, stopped, etc.), and other properties.
Checking for a Specific Service
Using `Get-Service` to Check If a Service Exists
Using the `Get-Service` command is straightforward. For example, to check if the Windows Update service exists, you would run:
Get-Service -Name "wuauserv"
If the service exists, this command will return details about it, including its status. If it does not exist, you will receive a `Cannot find any service` error message.
Handling Non-Existent Services
To gracefully manage situations where a service might not exist, a common approach is to use a Try-Catch block for error handling. Here’s how you can implement this:
try {
Get-Service -Name "nonexistentService" -ErrorAction Stop
} catch {
Write-Host "Service does not exist."
}
This script attempts to get the service. If it doesn't exist, it catches the error and outputs a friendly message instead of halting your script with an unhandled error.
Checking Multiple Services
If you need to check the existence of multiple services simultaneously, you can use an array to loop through the services. Here’s an example that demonstrates this:
$services = @("wuauserv", "bits", "nonexistentService")
foreach ($service in $services) {
if (Get-Service -Name $service -ErrorAction SilentlyContinue) {
Write-Host "$service exists."
} else {
Write-Host "$service does not exist."
}
}
In this script, we check each service in the `$services` array. The `-ErrorAction SilentlyContinue` parameter suppresses errors for non-existent services, allowing the script to continue running smoothly.
Using `Where-Object` for Advanced Checks
For more complex scenarios where you might want to filter services based on specific criteria, you can combine `Get-Service` with `Where-Object`. This approach allows you to check for services with specific statuses or attributes. For instance, if you want to list all running services, you can use the following command:
Get-Service | Where-Object { $_.Status -eq 'Running' } | Select-Object Name
This command retrieves all services and filters them by their status, outputting only those that are currently running.
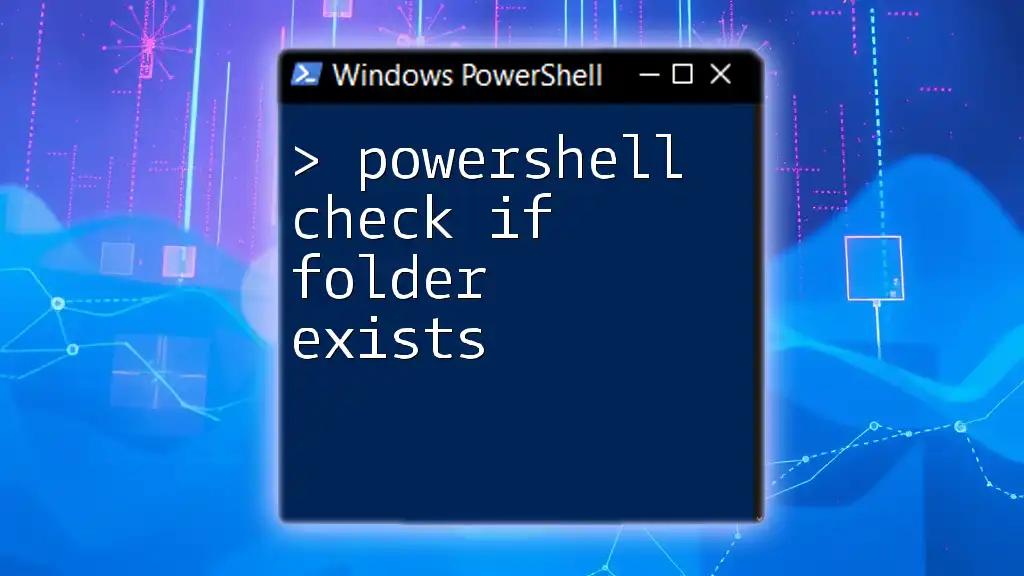
Best Practices for Service Checks
Error Handling
Incorporating robust error handling into your scripts is critical. Using `Try-Catch` not only helps capture errors but also allows you to take corrective action or provide informative feedback. It's a best practice to implement error handling for every PowerShell script that checks for service existence to ensure that it behaves as expected, even under error conditions.
Performance Considerations
When checking for service existence, be mindful of performance implications, especially if checking multiple services or running these checks frequently. Using `-ErrorAction SilentlyContinue` can improve efficiency by preventing the process from stopping when it encounters a non-existent service. Additionally, filtering services with `Where-Object` can help minimize the amount of data processed.
Automating the Check
To streamline repetitive tasks, consider creating a PowerShell script to check the existence of services. Below is a simple automation example that can be easily modified according to your needs:
$serviceName = "YourService"
if (Get-Service -Name $serviceName -ErrorAction SilentlyContinue) {
Write-Host "$serviceName is running."
} else {
Write-Host "$serviceName does not exist."
}
This script checks for a specified service and informs the user about its existence, making it a practical tool for routine service monitoring.
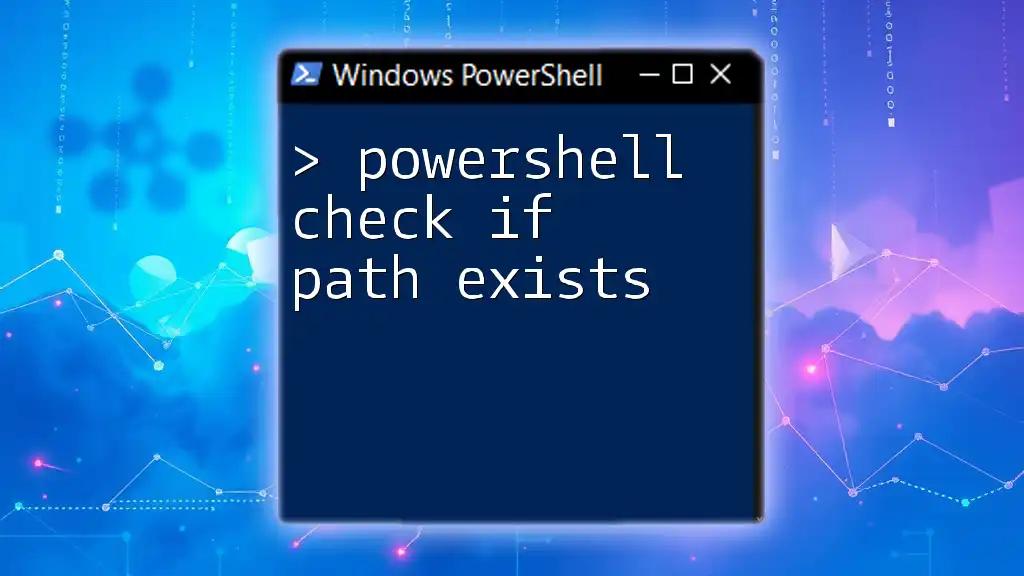
Conclusion
In summary, knowing how to PowerShell check if service exists is an essential skill for anyone managing Windows systems. Leveraging PowerShell commands like `Get-Service`, implementing error handling, and considering performance practices will significantly enhance your ability to manage and automate service checks efficiently. As you practice these techniques, you'll find that PowerShell can dramatically improve your system administration tasks.

Additional Resources
For further learning, consider visiting the official Microsoft documentation on PowerShell and service management, participating in community forums, and exploring additional reading materials related to PowerShell scripting and Windows service management.