To check if a specified path exists in PowerShell, you can use the `Test-Path` cmdlet, which returns a boolean value indicating the existence of the path.
Here’s a code snippet to demonstrate this:
$path = "C:\example\path"
if (Test-Path $path) {
Write-Host "The path exists."
} else {
Write-Host "The path does not exist."
}
Understanding Paths in PowerShell
What is a Path?
In the context of file systems, a path is a string that specifies the location of a file or a directory. PowerShell, like many programming languages and scripting environments, operates with two types of paths:
- Absolute Path: This provides the complete location of a file or directory from the root of the file system. For example, `C:\Users\Username\Documents\file.txt`.
- Relative Path: This is relative to the current working directory in PowerShell. For instance, just typing `file.txt` assumes the file is in the current directory.
Types of Paths in PowerShell
PowerShell deals with two primary types of paths:
- File Paths: Used to specify a location of specific files.
- Folder Paths: Indicate the location of a directory or folder.
Both types are essential when writing scripts, especially when automating tasks that involve file and directory manipulations.
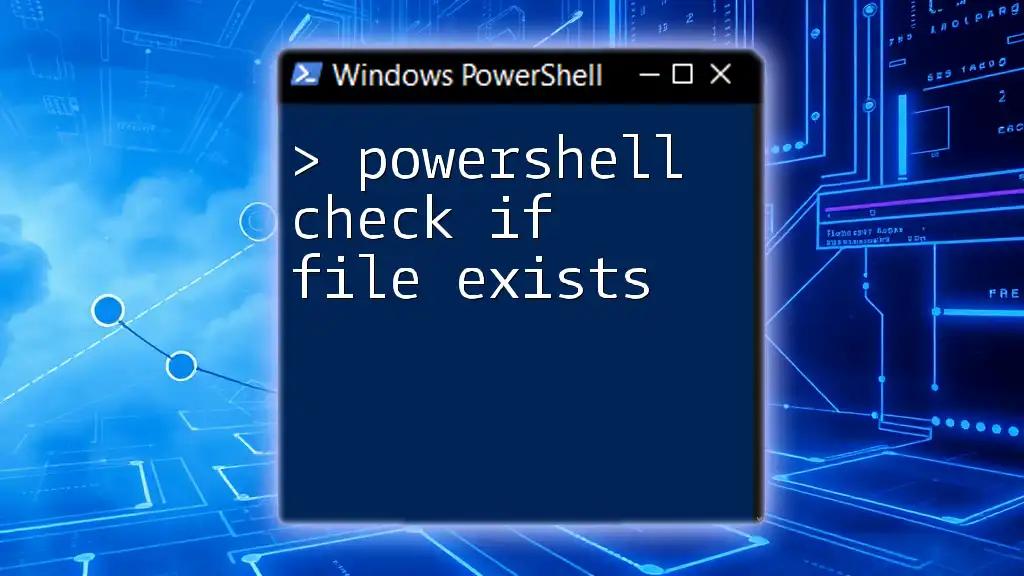
The `Test-Path` Cmdlet
Introduction to `Test-Path`
`Test-Path` is a built-in PowerShell cmdlet that checks whether a specified path exists. It is essential for validating whether a file or folder is available before attempting to manipulate it. The basic syntax is as follows:
Test-Path -Path <Path>
Parameters of `Test-Path`
-Path
The `-Path` parameter allows users to specify the path they want to verify. Let's see how it works in practice.
Example Usage: To check if a specific file exists, you can use the following command:
Test-Path -Path "C:\example\myfile.txt"
If the file exists, this command will return `True`; otherwise, it will return `False`.
-Credential
This parameter allows you to specify a user account that has permission to access the path, particularly useful when working with network shares.
-LiteralPath
The `-LiteralPath` parameter helps avoid issues with wildcard interpretations. It is especially valuable when the path contains special characters.
Code Snippet:
# Using -Path (may interpret wildcards)
Test-Path -Path "C:\example\*.txt"
# Using -LiteralPath (doesn't interpret wildcards)
Test-Path -LiteralPath "C:\example\*.txt"
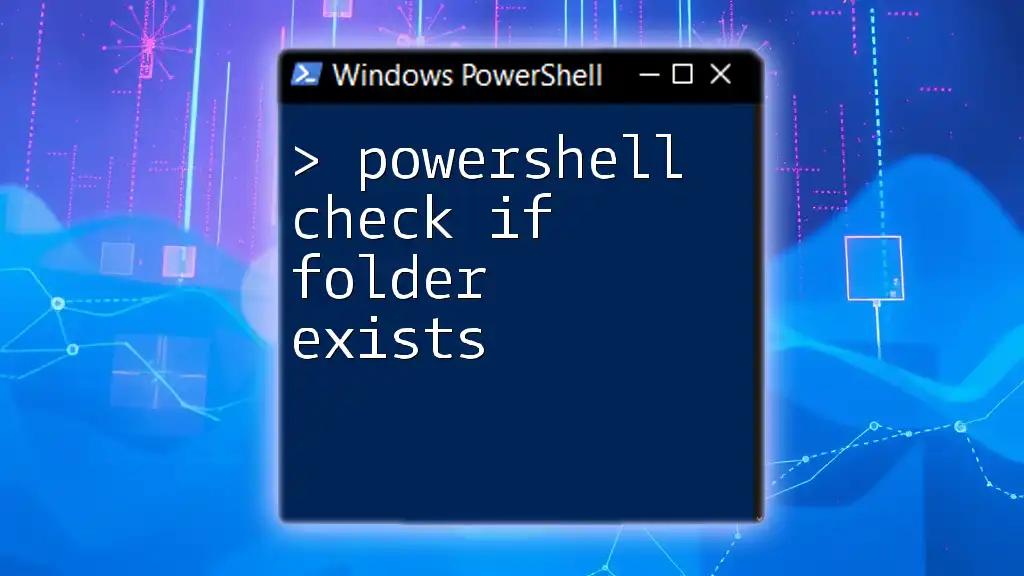
Using `Test-Path` to Check for Existing Paths
Checking a File Path
Verifying the existence of a file is a common task. Here’s how you can do it:
Example Code Snippet:
$filePath = "C:\example\myfile.txt"
if (Test-Path -Path $filePath) {
Write-Host "The file exists."
} else {
Write-Host "The file does not exist."
}
In this snippet, PowerShell checks if `myfile.txt` exists and outputs the result accordingly.
Checking a Folder Path
Similarly, checking if a folder exists is straightforward.
Example Code Snippet:
$folderPath = "C:\example\myfolder"
if (Test-Path -Path $folderPath) {
Write-Host "The folder exists."
} else {
Write-Host "The folder does not exist."
}
This will notify you if `myfolder` is present in the specified location.

Advanced Usage of `Test-Path`
Checking Network Paths
In many organizations, you'll find files stored on network shares. To validate whether these files are accessible, you can use `Test-Path`.
Example Code Snippet:
$networkPath = "\\server\share\file.txt"
if (Test-Path -Path $networkPath) {
Write-Host "The network file exists."
} else {
Write-Host "The network file does not exist."
}
This checks if the specified file on the network share is accessible from your script.
Error Handling with `Test-Path`
When working with paths, errors can occur, especially with network paths. You can use try-catch blocks to handle these errors gracefully.
Example Code Snippet:
try {
if (Test-Path -Path $filePath) {
Write-Host "The file exists."
} else {
Write-Host "The file does not exist."
}
} catch {
Write-Host "An error occurred while checking the path: $_"
}
This structure ensures that any exceptions generated while executing the `Test-Path` check are caught and handled appropriately.
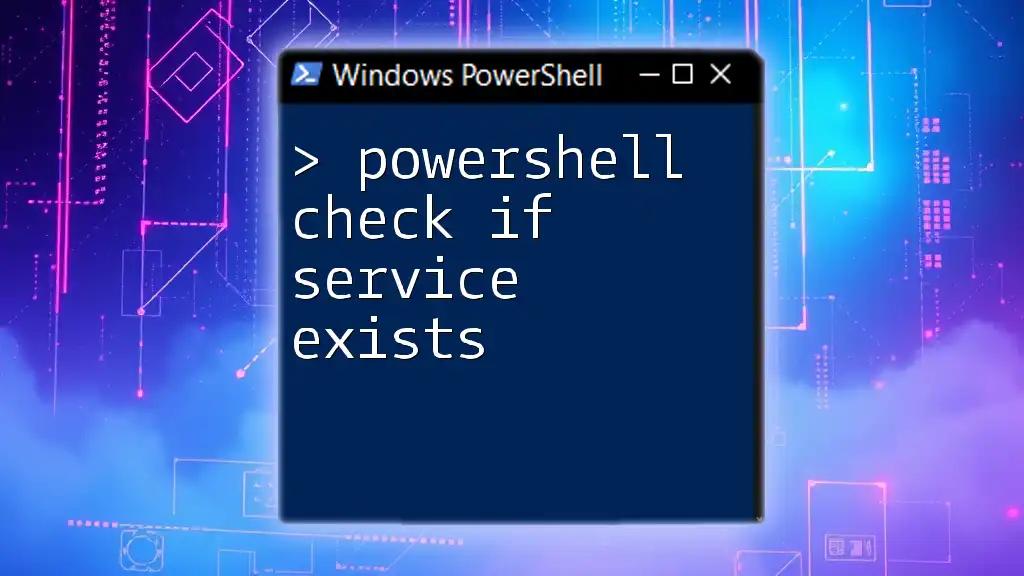
Alternatives to `Test-Path`
Using `Get-Item`
Another way to check for the existence of a path is by using the `Get-Item` cmdlet. If the path does not exist, it will throw an error, which can be caught for handling.
Example Code Snippet:
try {
$item = Get-Item -Path $filePath
Write-Host "Item exists."
} catch {
Write-Host "Item does not exist."
}
This method can be considered when you require not only path checking but also additional file or folder properties.

Conclusion
In this guide, we explored how to effectively check if a path exists in PowerShell using the `Test-Path` cmdlet. This powerful tool is crucial for validating user inputs and ensuring safe file manipulations in scripts. Remember to practice these commands to gain confidence and familiarity with path checks in your PowerShell scripts.

Call to Action
We encourage you to share your experiences and use cases on checking paths in PowerShell. Sign up for our newsletter for more valuable PowerShell tips and tutorials to enhance your skills and productivity!