You can check if a registry key exists in PowerShell by using the `Test-Path` cmdlet along with the registry path you want to verify.
$registryPath = "HKCU:\Software\YourKeyName"
if (Test-Path $registryPath) { Write-Host "Registry key exists." } else { Write-Host "Registry key does not exist." }
Understanding the Windows Registry
What is the Windows Registry?
The Windows Registry is a hierarchical database used by the operating system to store configuration settings and options for the operating system and installed applications. It contains information, settings, and options for both the operating system and applications that opt to use the Registry for their configuration needs.
This database is divided into several sections, known as hives, and each hive consists of keys and values. Changes made to the Registry can affect system functionality, user preferences, and even application performance. Because of its crucial role, understanding the Registry is imperative for anyone looking to manage Windows configurations effectively.
Importance of Registry Keys and Values
Registry keys and values are critical components that define how the operating system behaves. For instance, they manage settings related to user profiles, system hardware, and installed applications. Some common registry keys include:
- HKEY_LOCAL_MACHINE (HKLM): Contains configuration settings applied to the entire computer.
- HKEY_CURRENT_USER (HKCU): Contains settings specific to the logged-in user.
The ability to manage registry keys gives administrators and advanced users control over their systems, making it essential for troubleshooting, configuration, and security management.
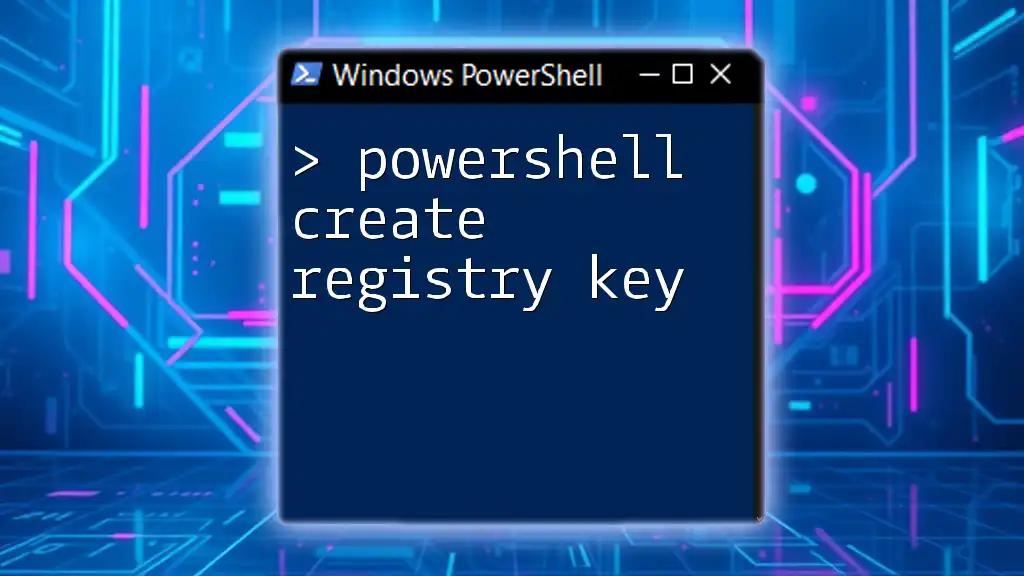
PowerShell and the Registry
Why Use PowerShell for Registry Management?
PowerShell is a powerful scripting language and command-line shell designed for system administration. Its capacity for dealing with the Windows Registry allows users to efficiently create scripts for automating routine registry tasks, making it a superior option compared to traditional methods such as using the Registry Editor (regedit).
Using PowerShell, you can execute complex queries, manipulate registry entries, and integrate commands efficiently, increasing productivity and reducing the chance of errors.
PowerShell Cmdlets for Registry Access
PowerShell provides several cmdlets to interact with the Registry, including:
- Get-Item: Retrieves a specified registry key.
- Get-ItemProperty: Retrieves the properties of a registry key.
- Test-Path: Checks whether a specified path exists within the Registry.
Understanding these cmdlets enables users to navigate and manipulate the Registry effectively.
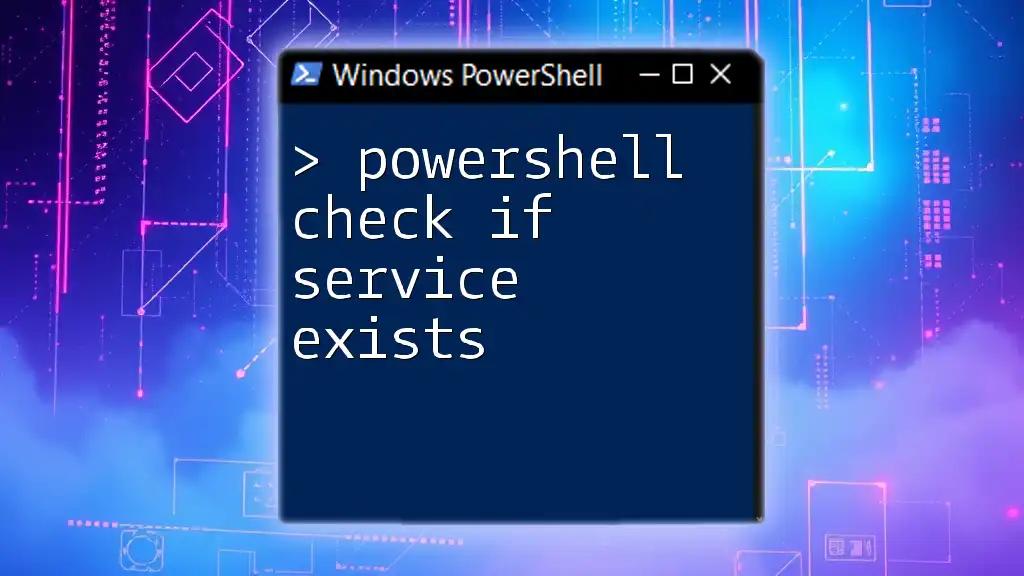
Checking if a Registry Key Exists
Syntax and Basic Command
To check if a registry key exists, you typically use the `Test-Path` cmdlet, which verifies the existence of a path. The basic syntax looks like this:
Test-Path 'HKLM:\Software\YourSoftwareName'
Understanding the Test-Path Cmdlet
The `Test-Path` cmdlet returns a boolean value indicating whether the specified path exists. This simple yet effective cmdlet serves as the foundation for verifying registry keys and implementing conditional checks in your scripts.
Practical Example: Checking a Registry Key
Here’s a complete example demonstrating how to check for the existence of a registry key:
$keyPath = 'HKCU:\Software\MyApplication'
if (Test-Path $keyPath) {
Write-Host "The registry key exists."
} else {
Write-Host "The registry key does not exist."
}
In this example, if the specified registry key exists, PowerShell will output "The registry key exists." Otherwise, it will state that the key does not exist. This can be particularly useful in scripts where the existence of a key informs subsequent actions.
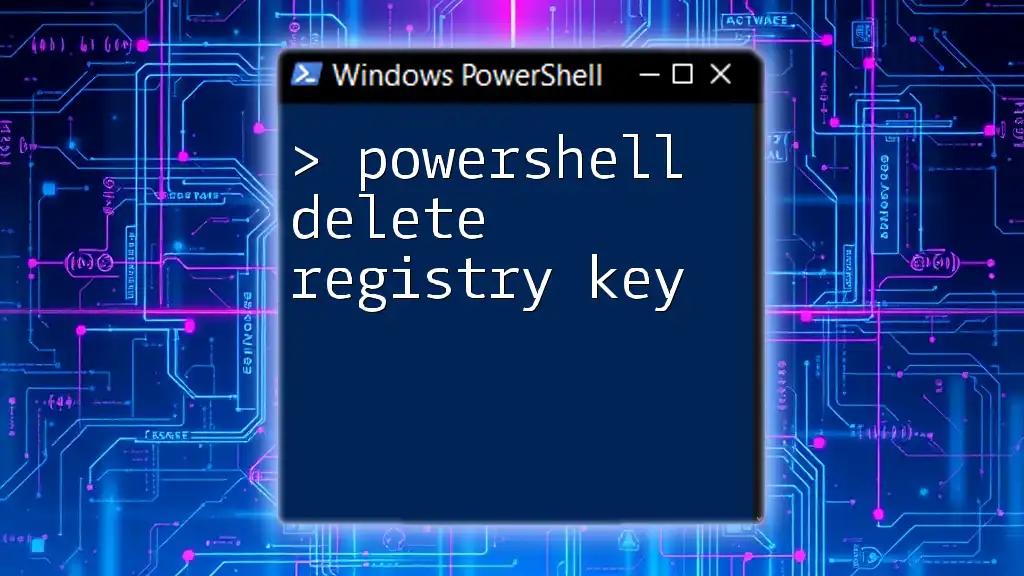
Checking if a Registry Value Exists
Syntax and Basic Command for Registry Values
To check for specific registry values, you can use `Get-ItemProperty` alongside an error action preference:
$valueExists = Get-ItemProperty -Path 'HKCU:\Software\MyApplication' -Name 'MyValue' -ErrorAction SilentlyContinue
Practical Example: Checking a Specific Registry Value
Here’s a complete example illustrating how to check for the presence of a specific registry value:
$keyPath = 'HKCU:\Software\MyApplication'
$valueName = 'MyValue'
if (Get-ItemProperty -Path $keyPath -Name $valueName -ErrorAction SilentlyContinue) {
Write-Host "The registry value exists."
} else {
Write-Host "The registry value does not exist."
}
In this example, the script checks for the registry value named `MyValue` within `MyApplication`. If it exists, PowerShell will confirm its presence. If not, the script will indicate its absence.
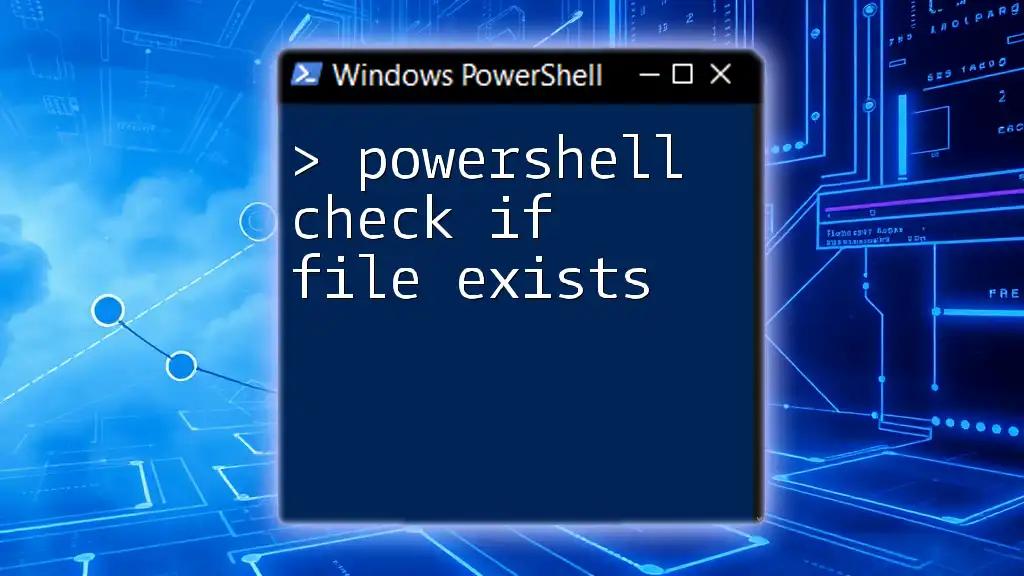
Advanced Techniques
Using Functions for Registry Checks
To enhance reusability in your scripts, you can create a function that checks for registry keys. This encapsulates the logic and allows for cleaner code:
function Check-RegistryKey {
param (
[string]$path
)
return Test-Path $path
}
if (Check-RegistryKey 'HKCU:\Software\MyApplication') {
Write-Host "The registry key exists."
} else {
Write-Host "The registry key does not exist."
}
This function simplifies the checking process, promoting better organization within your scripts.
Error Handling in PowerShell Scripts
Error handling is essential when working with the registry to avoid unintended outcomes. Utilizing the `try-catch` construct allows you to capture non-terminating errors, providing a fallback or alternative action when issues arise. Here’s an example:
try {
$valueExists = Get-ItemProperty -Path $keyPath -Name $valueName -ErrorAction Stop
Write-Host "The registry value exists."
} catch {
Write-Host "Error encountered: $_"
}
In this script, if there’s an error in retrieving the registry value, PowerShell will catch it and display the error message, ensuring a smoother user experience.
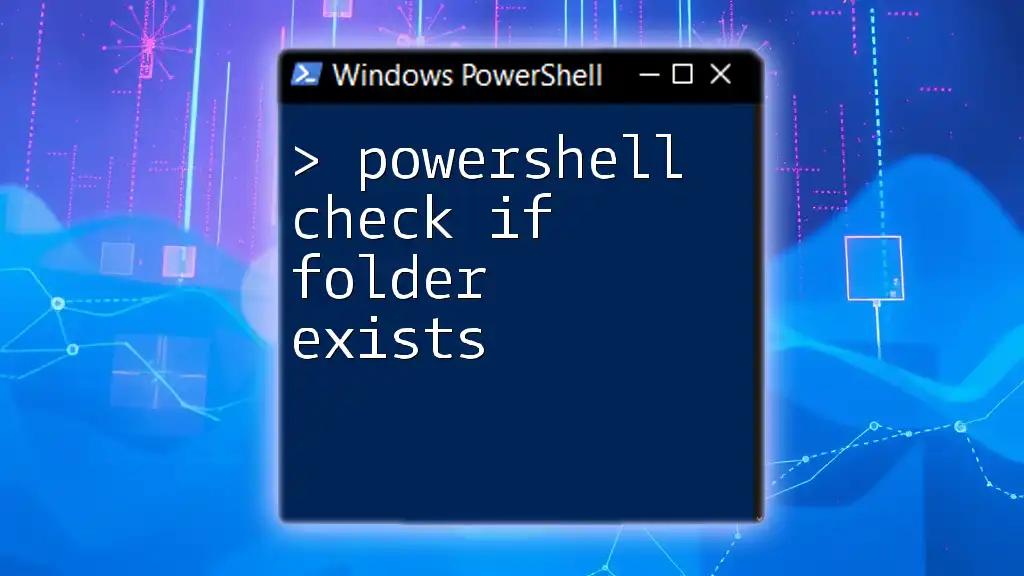
Common Mistakes to Avoid
Careless Editing of Keys and Values
One of the most common pitfalls is making careless edits within the registry. Given that the Registry is integral to system functionality, it’s essential to take precautions before making any changes. Always back up the registry or create a system restore point prior to editing.
Using Wrong Registry Paths
Another frequent error is using incorrect registry paths. Always consider double-checking your paths to ensure accuracy before executing any scripts. PowerShell makes it manageable to explore valid paths, but mistakes can lead to wasted time or unintended modifications.
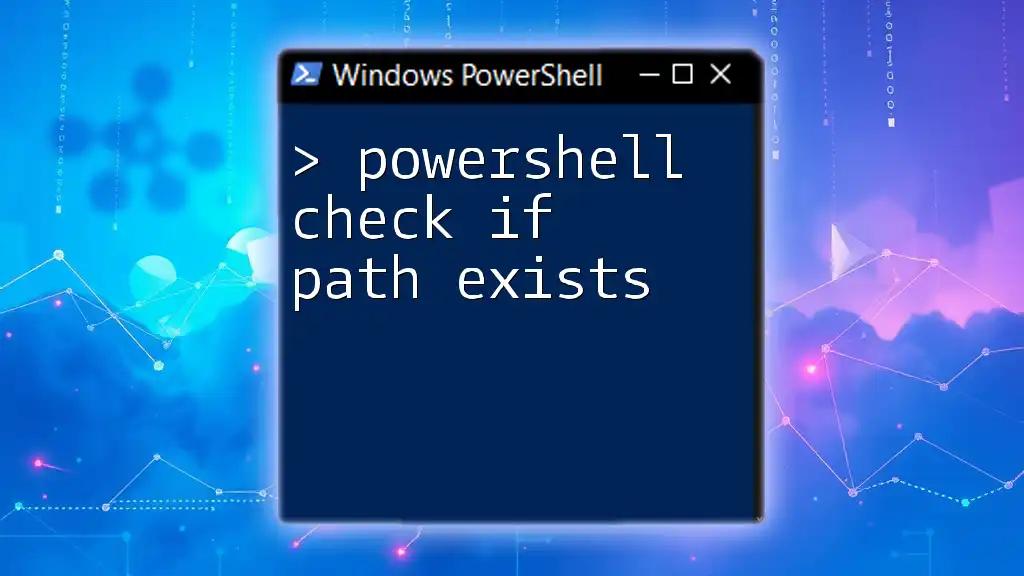
Conclusion
Checking the existence of registry keys and values using PowerShell is a valuable skill that allows users to harness the power of Windows' configuration settings. By understanding and applying the methodologies outlined in this article, users gain greater control over their systems.
Whether you’re automating scripts or performing system audits, mastering the command `powershell check if registry key exists` offers significant advantages. Keep exploring further PowerShell topics to enhance your skills and efficiency in system administration!

Additional Resources
To deepen your knowledge, consider exploring the following topics on your website:
- Advanced PowerShell Scripting Techniques
- Managing Software Installations via the Registry
- Troubleshooting Common PowerShell Errors
These resources will further equip you in your journey with PowerShell and the Windows Registry.