To check the TLS version supported by your PowerShell environment, you can use the following command:
[Net.ServicePointManager]::SecurityProtocol
Understanding TLS and Its Versions
What is TLS?
Transport Layer Security (TLS) is a cryptographic protocol that ensures secure communication over networks. It provides privacy and data integrity between two communicating applications, typically a web server and a client (like a browser). TLS is widely used in applications such as email, instant messaging, and VoIP.
TLS is the successor to the deprecated Secure Sockets Layer (SSL) protocol, and it is essential for protecting sensitive data during transmission. The importance of checking TLS versions stems from the need to ensure that your systems are using the most secure protocols available.
TLS Versions Overview
There are several major versions of TLS that have evolved over the years, each with improvements in security features:
- TLS 1.0: Released in 1999, this version has known vulnerabilities and is no longer considered secure.
- TLS 1.1: Introduced in 2006, this version addressed some vulnerabilities noted in TLS 1.0 but is also considered outdated due to recent security threats.
- TLS 1.2: Launched in 2008, TLS 1.2 brought significant improvements in encryption algorithms and security features, making it the most widely used version today.
- TLS 1.3: Released in 2018, this is the latest version that removes older cryptographic algorithms and simplifies the handshake process, enhancing security and performance.
To maintain a secure environment, it is critical to ensure that your systems are configured to use the latest protocol versions.
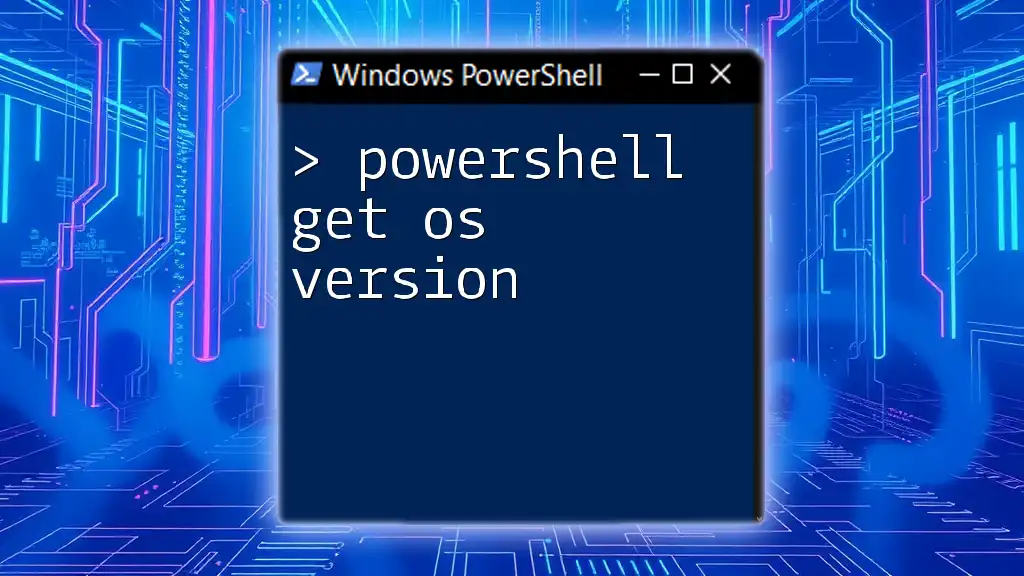
PowerShell Basics
What is PowerShell?
PowerShell is a task automation and configuration management framework from Microsoft, consisting of a command-line shell and an associated scripting language. PowerShell allows users to control and automate the administration of the Windows operating system and applications.
Its flexibility makes PowerShell powerful for IT professionals, simplifying tasks such as deploying system updates, managing Active Directory, and performing security audits.
PowerShell Cmdlets
Cmdlets are specialized .NET classes that PowerShell uses to perform specific operations. These commands are designed to be easy to use and cover a wide range of tasks, from file manipulation to system management.
An example of a basic cmdlet is:
Get-Process
This command retrieves a list of all the processes currently running on the system.
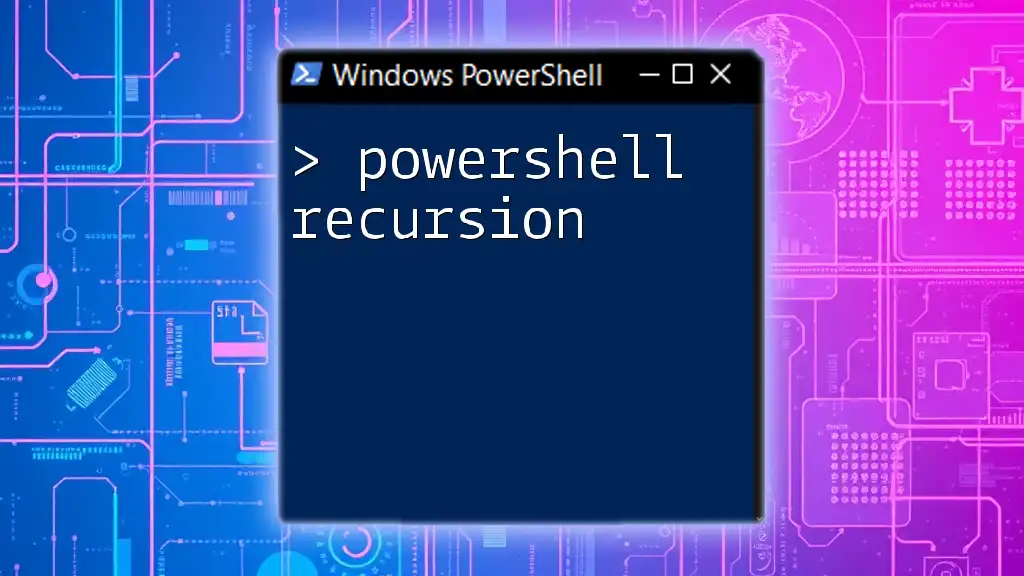
How to Check Installed TLS Versions with PowerShell
System Requirements
Before diving into the commands, ensure that you have appropriate permissions to run PowerShell. Additionally, verify the version of PowerShell you are running. You can do this by executing:
$PSVersionTable.PSVersion
Using the .NET Framework to Check TLS Versions
Accessing .NET Framework Classes
PowerShell leverages the .NET Framework to access different classes and properties. For checking TLS versions, you will primarily use the `System.Net.ServicePointManager` class.
To retrieve the current security protocol settings, you can execute:
[Net.ServicePointManager]::SecurityProtocol
Checking Enabled Security Protocols
To understand which TLS versions are currently enabled on your system, use the following code snippet:
$currentProtocol = [Net.ServicePointManager]::SecurityProtocol
if ($currentProtocol -band [Net.SecurityProtocolType]::Tls12) {
Write-Output "TLS 1.2 is enabled."
}
This code checks and outputs if TLS 1.2 is enabled, allowing you to quickly determine your current security posture.
Using Registry to Check TLS Versions
Accessing TLS Settings in the Registry
Windows stores TLS settings in the registry, where you can manually verify which protocols are enabled. The relevant keys can be found at:
HKEY_LOCAL_MACHINE\SYSTEM\CurrentControlSet\Control\SecurityProviders\SCHANNEL\Protocols
Here, you can navigate through the protocols to examine their respective settings.
PowerShell Script to Query the Registry
You can use PowerShell to query the registry and fetch enabled TLS versions with the following command:
Get-ItemProperty -Path "HKLM:\SYSTEM\CurrentControlSet\Control\SecurityProviders\SCHANNEL\Protocols\TLS 1.2\Client" | Select-Object -Property Enabled, Disabled
This command retrieves the `Enabled` and `Disabled` properties of the TLS 1.2 client setting, providing clear insight into your current configurations.
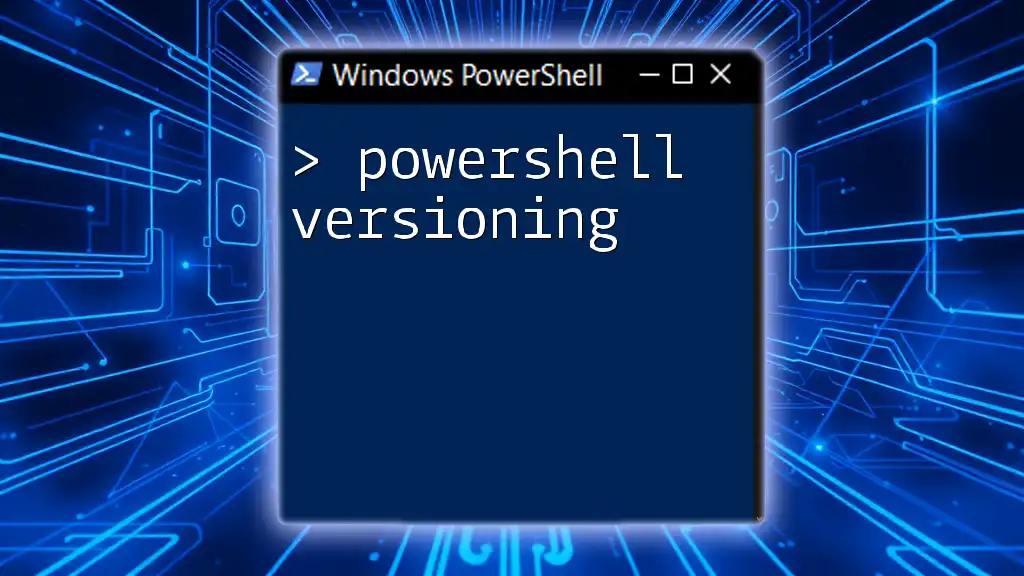
Updating TLS Settings Using PowerShell
Enabling or Disabling TLS Versions
Regular updates to your security protocols are critical in maintaining a secure environment. If you identify that certain TLS versions are disabled and need to be enabled, using PowerShell can simplify this process.
To enable TLS 1.2, for example, you can run:
Set-ItemProperty -Path "HKLM:\SYSTEM\CurrentControlSet\Control\SecurityProviders\SCHANNEL\Protocols\TLS 1.2\Client" -Name "Enabled" -Value 1
This command modifies the registry to allow the use of TLS 1.2 for clients.
Restarting the System
After making changes to TLS settings, a restart is often necessary for the changes to take effect. You can initiate a system restart using:
Restart-Computer -Force
This ensures that all applications and services load with the updated protocol settings.
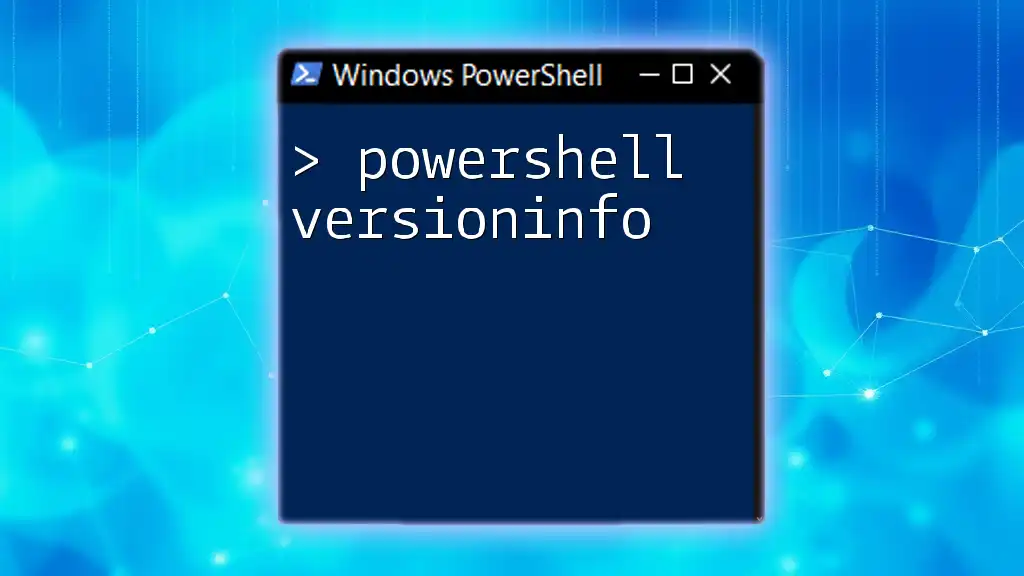
Common Issues and Troubleshooting
Problems with Checking or Updating TLS Versions
While executing PowerShell commands, you might encounter unexpected errors. Common issues often revolve around permissions. Ensure that you are running PowerShell as an administrator to avoid permission-related errors that might prevent you from accessing necessary properties.
Verifying Changes
After updating TLS settings, it's crucial to verify that the changes were applied successfully. Re-running the checks discussed previously can help confirm that the enabling/disabling of TLS versions has occurred as intended.
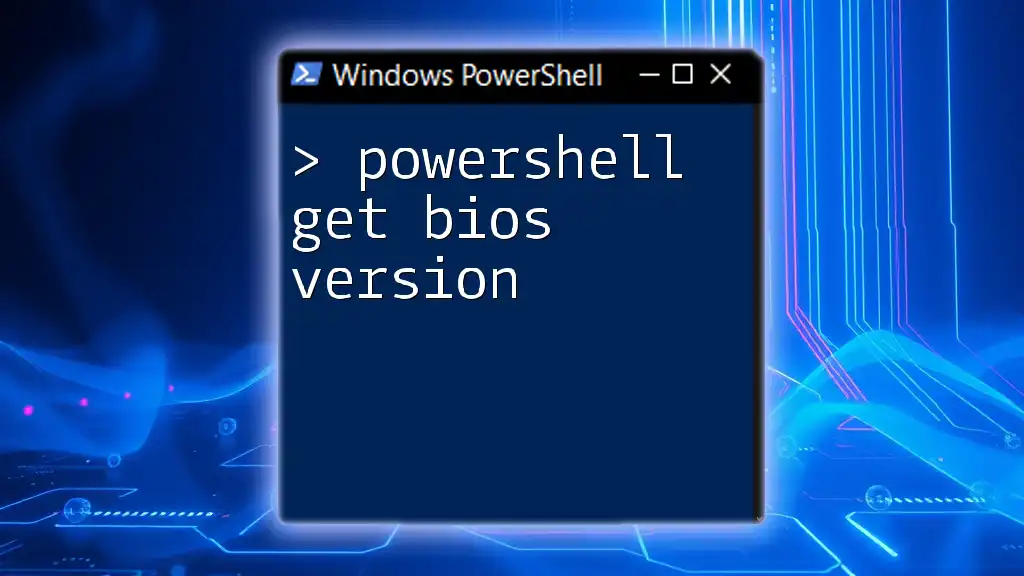
Conclusion
Checking and updating TLS versions is vital for securing network communications and protecting sensitive information. By utilizing PowerShell, you can efficiently audit and modify your TLS settings, ensuring your systems are always in line with best security practices.
Regularly monitor and maintain your TLS settings to keep up with ever-changing security landscapes. As always, feel free to reach out with questions or to share experiences regarding your journey with PowerShell and TLS version management!
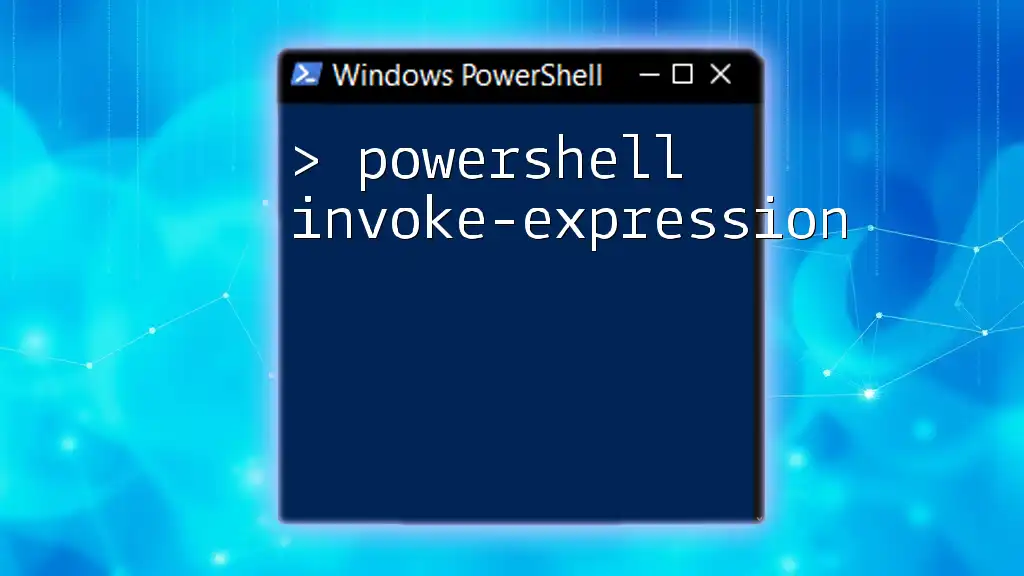
Additional Resources
Recommended Reading
For further reading, consider exploring the official Microsoft documentation on TLS and PowerShell. Staying informed about cybersecurity best practices is essential for any IT professional.
Contact Information
If you require additional help or training, do not hesitate to get in touch. We're here to assist you on your journey to mastering PowerShell!
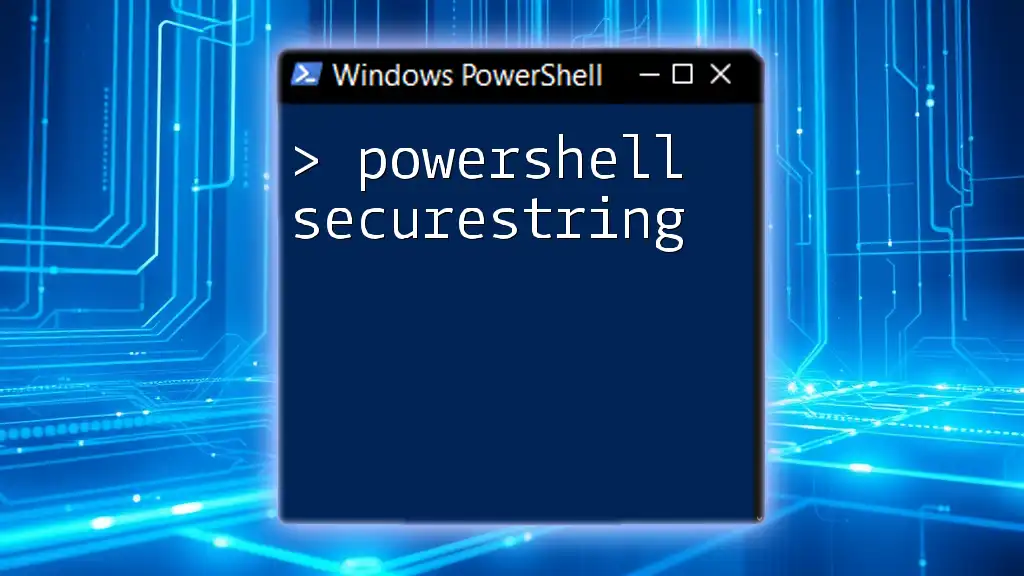
Call to Action
For more insightful PowerShell tips and training resources, subscribe to our newsletter today. Additionally, we offer a free guide on PowerShell basics when you sign up!