To check if a PowerShell script is running with administrator privileges, you can use the following command:
If (-Not ([Security.Principal.WindowsPrincipal] [Security.Principal.WindowsIdentity]::GetCurrent()).IsInRole([Security.Principal.WindowsBuiltInRole]::Administrator)) { Write-Host 'Not running as admin' } Else { Write-Host 'Running as admin' }
How to Check if PowerShell is Running as Admin
Using the `-eq` Operator
One of the simplest ways to check if PowerShell is running as admin is by using the `$IsElevated` property in conjunction with the `-eq` operator. This method directly assesses whether the current session has elevated privileges.
You can utilize the following code snippet:
if (-not [bool](Get-Process -Id $PID).StartInfo.Verb -eq "runas") {
Write-Host "Not running as Administrator"
} else {
Write-Host "Running as Administrator"
}
In this example, `Get-Process -Id $PID` retrieves the process associated with the current PowerShell instance. The `StartInfo.Verb` property returns the action verb, allowing you to ascertain whether it's "runas," which indicates elevated permissions. If the condition evaluates to false, it means the PowerShell session lacks administrative privileges.
Using `WindowsIdentity` Class
Another effective method for checking administrative privileges is through the `WindowsIdentity` class. This class provides access to Windows user identity and allows you to check membership in various roles.
Here’s how you can implement it:
$adminCheck = [Security.Principal.WindowsPrincipal]([Security.Principal.WindowsIdentity]::GetCurrent())
if ($adminCheck.IsInRole([Security.Principal.WindowsBuiltInRole]::Administrator)) {
Write-Host "Running as Administrator"
} else {
Write-Host "Not running as Administrator"
}
In this code, `[Security.Principal.WindowsIdentity]::GetCurrent()` retrieves the current user's identity. By wrapping it in `[Security.Principal.WindowsPrincipal]`, you can evaluate whether the user belongs to the Administrator role. This is a robust method to ensure the script operates with the necessary permissions.

Practical Examples
Creating a Simple Script to Check Elevation
It’s often useful to create a reusable script that verifies if it’s running with elevated privileges. Below is an example script that includes detailed comments to guide understanding:
# Check if the script is running with elevated privileges
if (-not [bool](Get-Process -Id $PID).StartInfo.Verb -eq "runas") {
Write-Host "This script requires Administrator privileges. Please run as Admin."
Exit
}
Write-Host "Script is running with Administrator privileges."
In this script, if the current session is not elevated, a message prompts the user to run it as an administrator, and the script exits. This proactive error management ensures users understand requirements before executing sensitive operations.
Using the Check within a Larger Script
When developing more complex scripts that depend on elevated privileges, you can seamlessly integrate the check for administrator status. Here’s a concise example of how it can fit within a larger context:
# Confirming admin rights before proceeding with file operations
if (-not [bool](Get-Process -Id $PID).StartInfo.Verb -eq "runas") {
Write-Host "Please run this script as an Administrator."
Exit
}
# Example of an operation that requires admin rights
Remove-Item -Path "C:\Path\To\Sensitive\File.txt" -Force
Write-Host "File has been successfully deleted."
In this case, if the script does not have the necessary permissions, it prompts the user accordingly before attempting to delete a sensitive file, thereby ensuring that permission errors do not occur during execution.

Common Pitfalls and Troubleshooting
Mistakes Made While Checking for Admin Rights
One common mistake when checking for admin rights is overlooking the necessity of elevated permissions until a command fails. It’s essential to proactively include these checks at the beginning of your scripts to avoid runtime errors.
How to Fix Permission Issues
If your script encounters an error due to lacking admin rights, you can resolve this by informing users to execute the script as an Administrator. This can be amended in your script by displaying a clear message indicating the requirement.

Conclusion
Checking if PowerShell is running as admin is an essential skill for anyone developing scripts that perform system-level tasks. By employing methods such as checking the `$IsElevated` property or using the `WindowsIdentity` class, you can ensure your scripts operate smoothly with the required permissions. This proactive approach not only saves time but also enhances security and functionality, allowing you to focus on the task at hand without interruptions caused by permission-related errors.

Additional Resources
For further reading, I highly recommend visiting the [official Microsoft documentation](https://docs.microsoft.com/en-us/powershell/scripting/overview?view=powershell-7.2) to deepen your understanding of PowerShell’s capabilities. Consider exploring additional learning materials like books and online courses that cater to aspiring PowerShell users and those looking to refine their scripting skills.
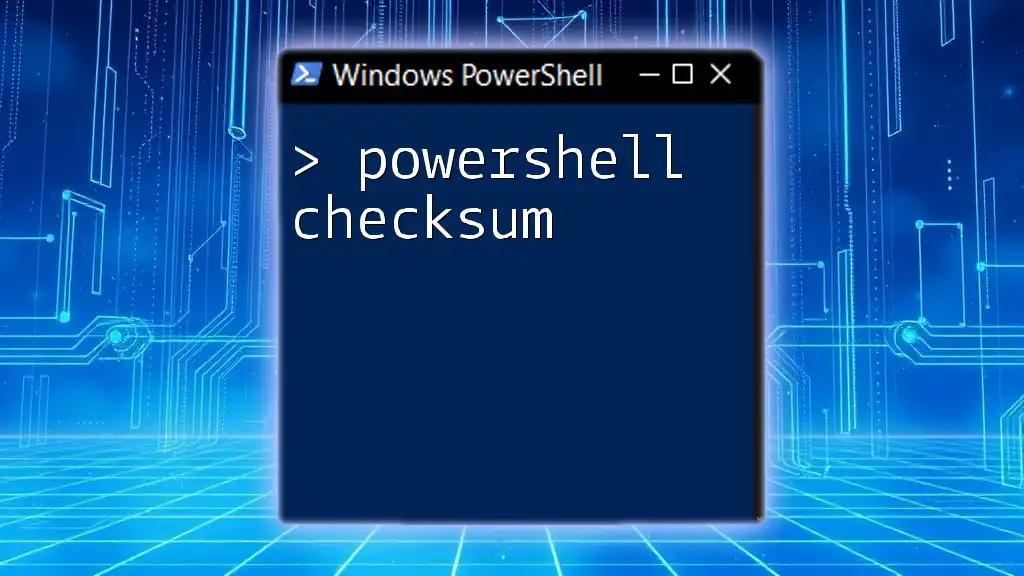
Call to Action
If you have any feedback or questions regarding PowerShell or scenarios where you’ve needed to check for administrative rights, please feel free to comment. Your experiences and insights are always welcome!