To install an MSI package remotely using PowerShell, you can utilize the `Invoke-Command` cmdlet along with `msiexec` to execute the installation on a remote machine. Here's a code snippet demonstrating how to do this:
Invoke-Command -ComputerName "RemotePC" -ScriptBlock { Start-Process msiexec.exe -ArgumentList '/i "C:\Path\To\YourInstaller.msi" /quiet /norestart' -Wait }
This command remotely installs the specified MSI file in silent mode, ensuring minimal disruption.
Understanding MSI Files
What is an MSI File?
An MSI (Microsoft Installer) file is a package format used by Windows for the installation, maintenance, and removal of software. It provides an efficient way to deploy applications, and it contains all the necessary information for installing a program, including files, installation paths, and registry entries.
Benefits of Using MSI Files
Using MSI files provides several advantages:
- Automation of Installations: MSI files enable you to automate software deployment processes, minimizing manual intervention.
- Consistency Across Systems: By using standardized packages, you ensure that software installations are uniform, which reduces discrepancies in configurations across different machines.
- Ease of Distribution: MSI files can be easily shared over networks or deployed through tools like System Center or Group Policy, making them perfect for environments with multiple machines.
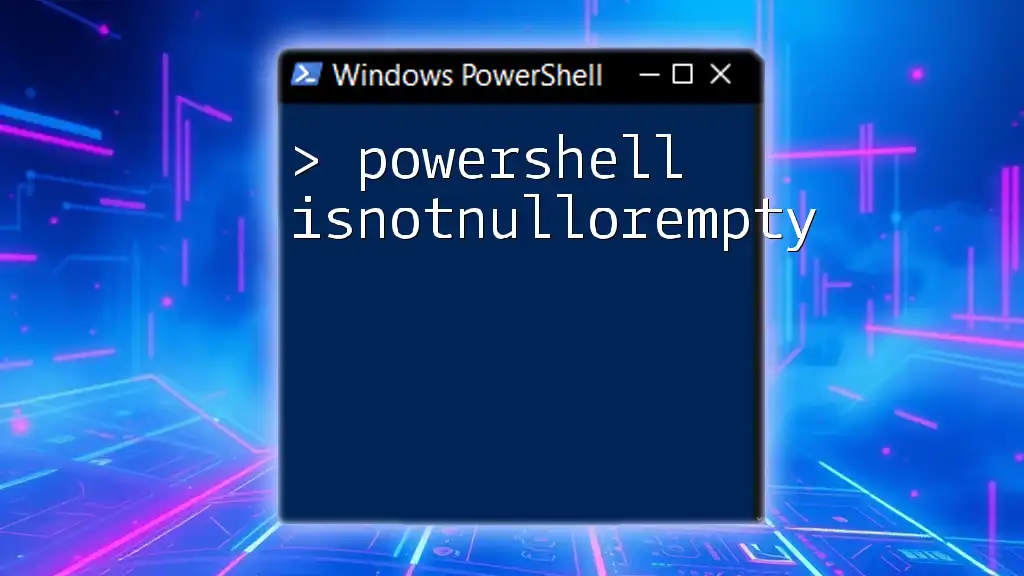
Prerequisites for Remote Installation
System Requirements
Before you start installing MSI files remotely, be sure to check that your systems meet the following requirements:
- Windows Version: Ensure that the remote machines are running compatible versions of Windows that support PowerShell Remoting.
- PowerShell Version: Verify that the target machines have an appropriate version of PowerShell installed; ideally, use PowerShell 5.1 or newer.
Necessary Permissions
To perform remote installations, you must have the necessary user account privileges:
- User Accounts and Group Permissions: Your user account needs sufficient permissions on the remote machine. It's generally advisable to operate with an administrative account to avoid permission issues.
- Running as an Administrator: Always execute PowerShell as an administrator to ensure access to all commands and features.
Network Considerations
Connectivity plays a crucial role in remote installations:
- Network Connectivity Requirements: Ensure that you have stable network connectivity to all remote machines.
- Firewall Settings Adjustment: Adjust any firewalls or security settings that might block PowerShell Remoting traffic. The required ports (typically TCP 5985 for HTTP and TCP 5986 for HTTPS) must be open.
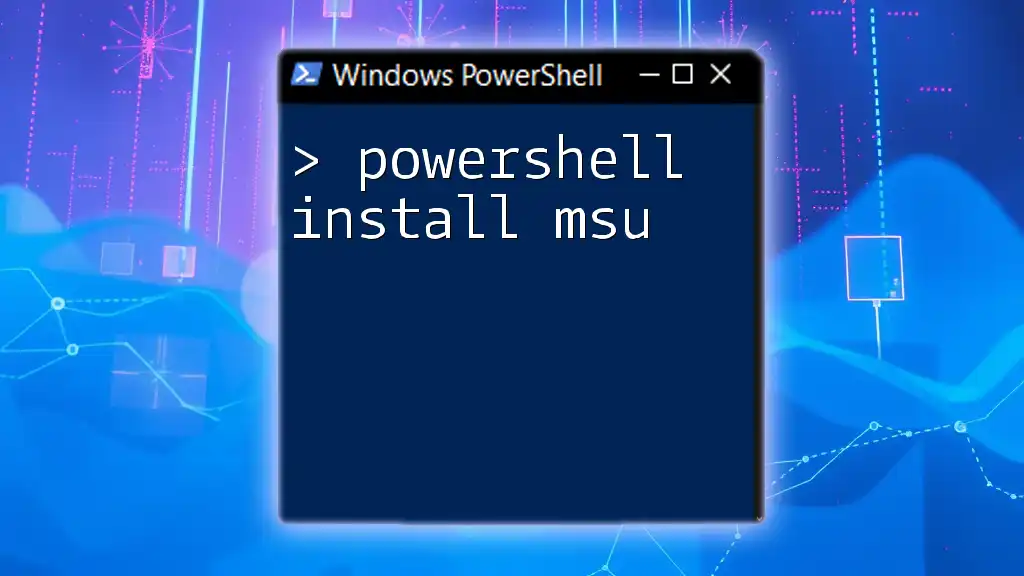
Setting Up PowerShell for Remote Use
Enabling PowerShell Remoting
To enable PowerShell Remoting, execute the following command on the remote machine:
Enable-PSRemoting -Force
This command sets up the WinRM service and configures the necessary firewall rules to allow remote connections.
Configuring Trusted Hosts
In certain environments, particularly those that do not operate in a domain, you may have to configure trusted hosts:
Set-Item WSMan:\localhost\Client\TrustedHosts -Value "RemotePC" -Concatenate
Replace "RemotePC" with the hostname or IP address of the target machines. This step allows your local machine to communicate securely with the specified remote machine.
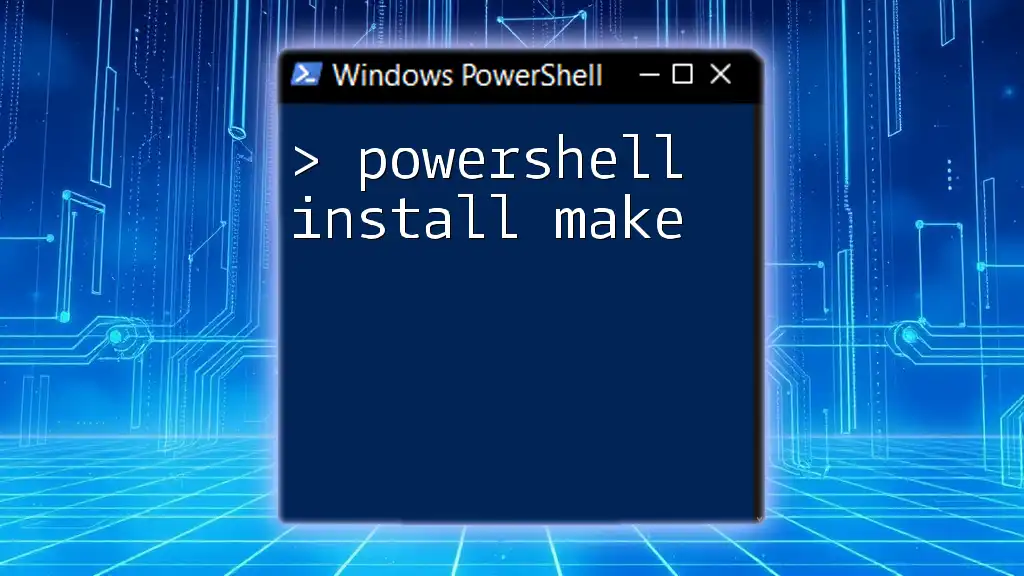
PowerShell Command to Install MSI Remotely
Basic Syntax for Remote MSI Installation
To install MSI packages remotely using PowerShell, you can use the `Invoke-Command` cmdlet. Here is a general structure of the command:
Invoke-Command -ComputerName "RemotePC" -ScriptBlock {
Start-Process -FilePath "C:\Path\To\YourInstaller.msi" -ArgumentList "/quiet", "/norestart" -Wait
}
Example Command
Here’s an example demonstrating the usage of the command:
Invoke-Command -ComputerName "RemotePC" -ScriptBlock {
Start-Process -FilePath "C:\Installers\MyApplication.msi" -ArgumentList "/quiet", "/norestart" -Wait
}
In this example, `"RemotePC"` is the target machine, and the MSI file located at `C:\Installers\MyApplication.msi` is installed silently without a restart.
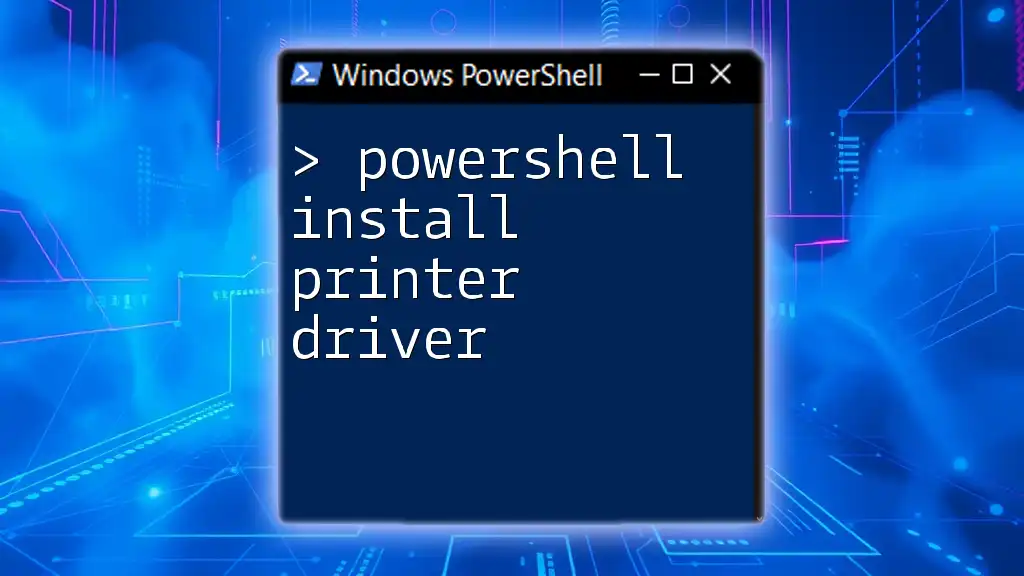
Creating a PowerShell Script to Install MSI Silently and Remotely
Writing the Script
Crafting a PowerShell script simplifies installing MSI files on multiple machines. Include silent installation flags to avoid user interaction during the process.
Example Script
Here’s a simple script that installs an MSI file on multiple remote machines:
$remoteComputers = "PC1", "PC2", "PC3"
$msiPath = "\\NetworkShare\YourInstaller.msi"
foreach ($computer in $remoteComputers) {
Invoke-Command -ComputerName $computer -ScriptBlock {
param($msi)
Start-Process -FilePath $msi -ArgumentList "/quiet", "/norestart" -Wait
} -ArgumentList $msiPath
}
This script declares a list of remote computers and specifies the network path to the MSI file. It then loops through each computer and executes the installation.
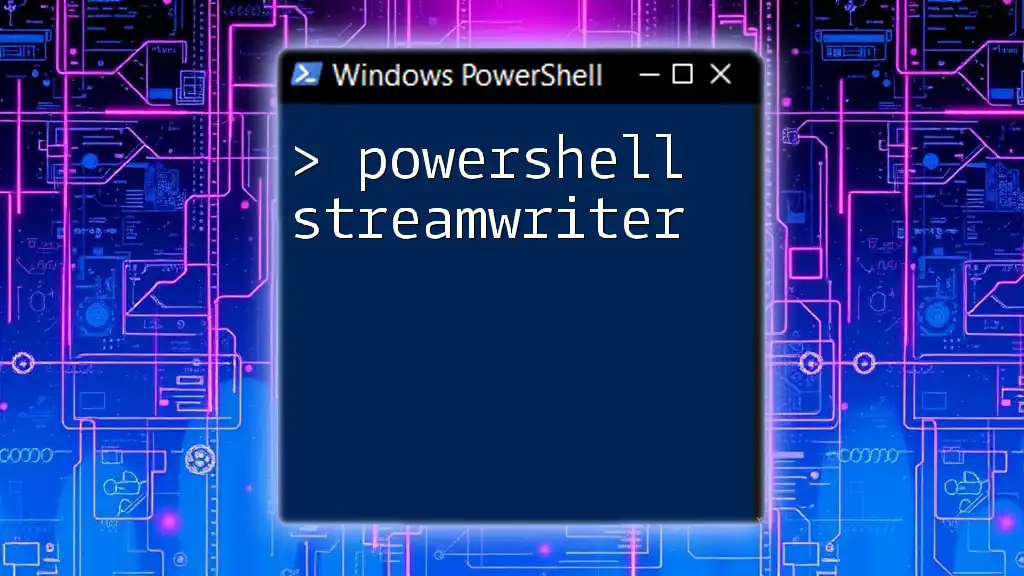
Error Handling While Installing MSI Remotely
Common Issues and Resolutions
When installing MSI files remotely, you may encounter a variety of issues:
- Connectivity Issues: Ensure that the mentioned hosts are reachable. You can test connectivity using the `Test-Connection` command.
- Permissions Errors: If you receive permission errors, verify that your user account has administrative rights on the remote systems.
Implementing Error Handling in Your Script
For a more robust installation script, implement error handling using `try-catch` blocks:
try {
Start-Process -FilePath $msi -ArgumentList "/quiet", "/norestart" -Wait
}
catch {
Write-Host "Error during installation: $_"
}
This structure helps to capture any errors during the execution and allows you to output detailed information.
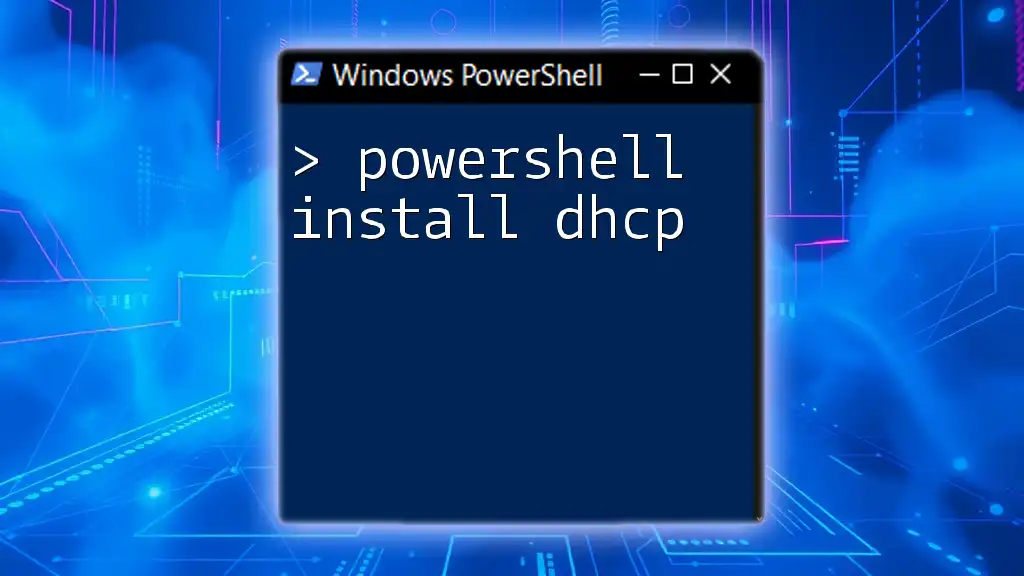
Best Practices for Remote MSI Installation
Testing Before Full Deployment
Before deploying MSI installations broadly, always test your script on a single machine. This helps identify potential issues without affecting multiple users.
Logging and Monitoring Installation Success
Keeping track of your installations is vital. You can log installation success and errors with a simple command:
Start-Process -FilePath $msi -ArgumentList "/quiet", "/norestart" -Wait -RedirectStandardOutput "C:\Logs\InstallLog.txt"
This command redirects the output to a log file, allowing for easier troubleshooting later.
Regular Updates and Maintenance
Keep your MSI packages updated. Periodically review installation success rates and refine your deployment strategies based on these metrics to improve your overall process efficiency.
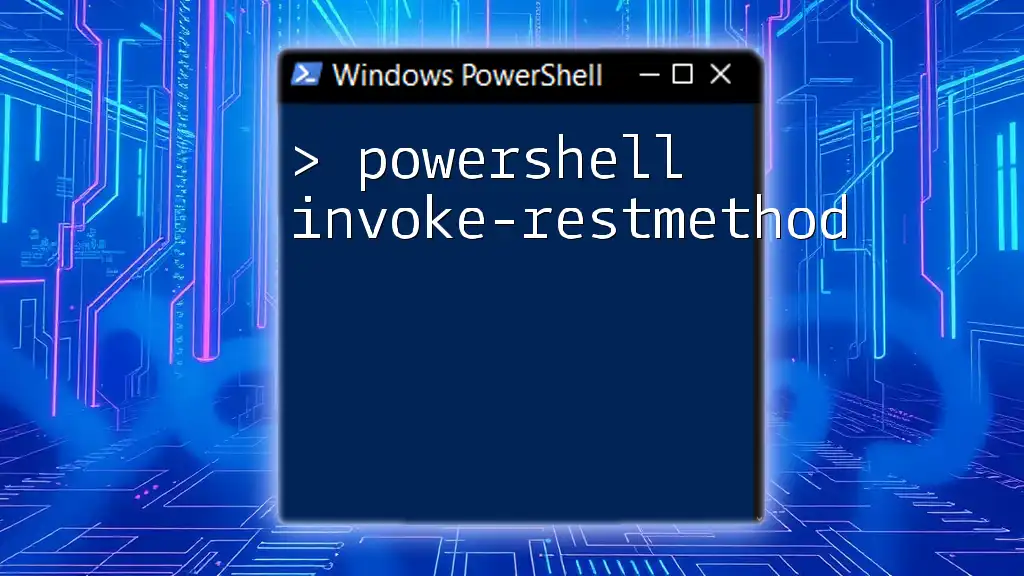
Conclusion
PowerShell provides a powerful framework for installing MSI files remotely, streamlining the deployment process in professional environments. By leveraging scripts for silent installations and handling errors efficiently, IT professionals can ensure a smooth software deployment experience.
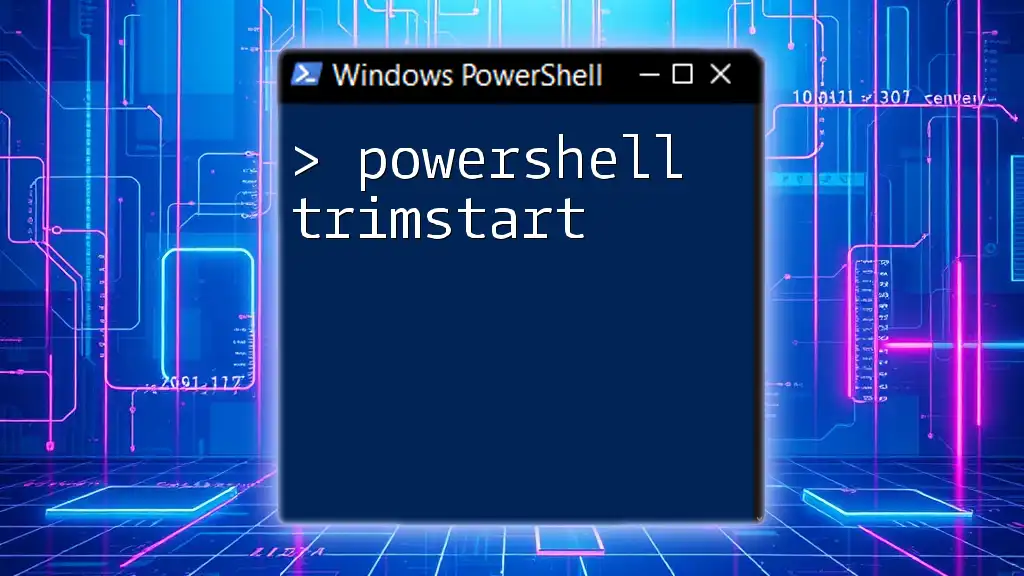
Call to Action
For more PowerShell tips and scripts, consider subscribing to our updates. Join our community to share experiences and learn more about effective PowerShell practices!
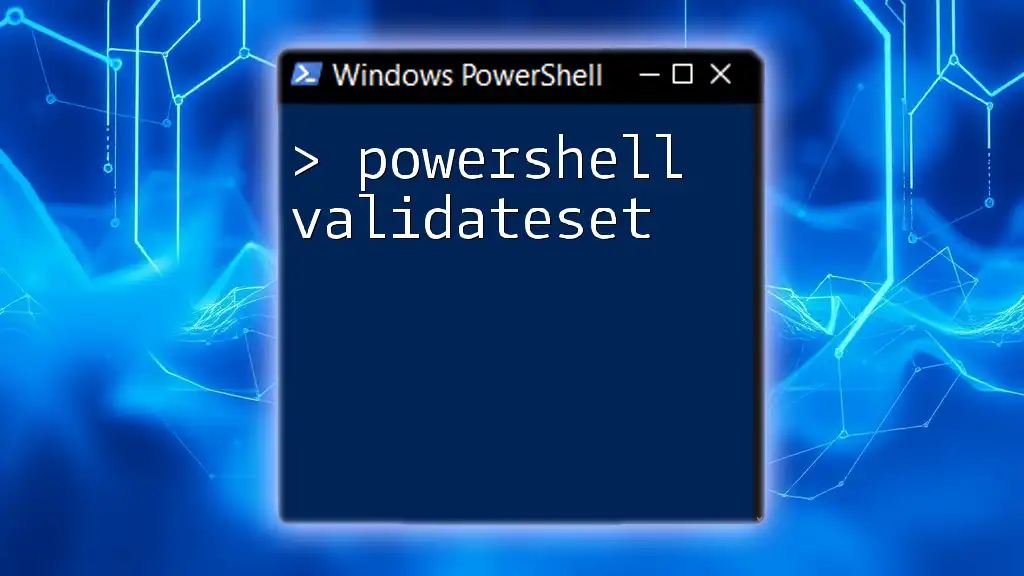
Additional Resources
For further insights into PowerShell and software deployment, explore the [official Microsoft documentation on PowerShell](https://docs.microsoft.com/en-us/powershell/). Additional reading will enhance your understanding of MSI management and PowerShell scripting.
FAQs
-
What are the requirements for using PowerShell for MSI installations? Ensure you have the appropriate permissions and network connectivity to the target systems before attempting any installations.
-
What are common errors when installing MSI files remotely? Connectivity issues and permissions errors are typical challenges; both can be remedied with proper configuration and user rights adjustments.
By adhering to this guide, you'll be well-equipped to execute remote MSI installations using PowerShell effectively. Happy scripting!