The `Send-MailMessage` cmdlet in PowerShell allows you to easily send emails to multiple recipients by specifying their email addresses separated by a semicolon in the `-To` parameter.
Here's a code snippet to demonstrate this:
Send-MailMessage -From "your_email@example.com" -To "recipient1@example.com;recipient2@example.com" -Subject "Test Email" -Body "This is a test email sent to multiple recipients." -SmtpServer "smtp.example.com"
Understanding the Send-MailMessage Cmdlet
What is Send-MailMessage?
The Send-MailMessage cmdlet is a powerful tool in PowerShell that allows you to send emails from within your scripts. It provides an easy way to automate email notifications, making it invaluable for system administrators, developers, and anyone who needs to keep stakeholders informed. The cmdlet uses the SMTP (Simple Mail Transfer Protocol) to send emails, enabling you to reach your recipients through various email servers.
Basic Syntax of Send-MailMessage
The syntax of the Send-MailMessage cmdlet includes several key parameters:
- `-To`: Specifies the recipients of the email. This parameter can accept a comma-separated list.
- `-From`: Indicates the sender's email address.
- `-Subject`: Sets the subject line of the email.
- `-Body`: Contains the email's content.
- `-SmtpServer`: Defines the SMTP server to handle the email sending process.
Here is a basic example showing how to use the cmdlet:
Send-MailMessage -To "recipient@example.com" -From "sender@example.com" -Subject "Test Email" -Body "This is a test email." -SmtpServer "smtp.example.com"

Sending Emails to Multiple Recipients
Overview of Multiple Recipients in Emails
When automating notifications, sending emails to multiple recipients can save time and ensure that everyone is informed. This capability is particularly useful in situations such as:
- Sending updates to a team
- Notifying stakeholders about system changes
- Alerting users about scheduled maintenance
Using Send-MailMessage to Specify Multiple Recipients
The `-To` Parameter
To send emails to multiple recipients using PowerShell's Send-MailMessage, you can specify multiple addresses in a single string, separated by commas.
Consider the following code snippet:
Send-MailMessage -To "user1@example.com,user2@example.com" -From "sender@example.com" -Subject "Test Email" -Body "This is a test email." -SmtpServer "smtp.example.com"
This command sends the email to both `user1@example.com` and `user2@example.com`, demonstrating the ease of reaching multiple recipients in one go.
Utilizing CC and BCC for Multiple Recipients
What are CC and BCC?
In addition to the `-To` parameter, PowerShell also allows you to use CC (Carbon Copy) and BCC (Blind Carbon Copy) to send emails to additional recipients. CC recipients will receive a copy of the email, and their addresses will be visible to all recipients. In contrast, BCC recipients will receive a copy of the email without their addresses being displayed.
Here is how you might implement CC and BCC in your command:
Send-MailMessage -To "user1@example.com" -Cc "user2@example.com" -Bcc "user3@example.com" -From "sender@example.com" -Subject "Test Email" -Body "This email is sent using CC and BCC." -SmtpServer "smtp.example.com"

Advanced Techniques for Sending Emails
Constructing Recipient Lists
Using Arrays for Multiple Recipients
For more dynamic scenarios, you can create an array that holds all your recipient email addresses, enabling you to easily manage lists of recipients. This method is particularly useful when your recipient list varies based on conditions or external inputs.
Here’s how you can do it using an array:
$recipients = "user1@example.com", "user2@example.com", "user3@example.com"
Send-MailMessage -To $recipients -From "sender@example.com" -Subject "Advanced Email" -Body "Email sent to multiple recipients" -SmtpServer "smtp.example.com"
Reading Email Addresses from a File
Use Case for File Inputs
When sending emails to a large number of recipients, maintaining lists directly in your script can become cumbersome. Instead, you can store email addresses in an external file, such as a CSV, and read them in your PowerShell script.
Example: If you have a CSV file named `Recipients.csv` containing a list of email addresses, you can read them as follows:
$recipients = Import-Csv -Path "C:\Path\To\Recipients.csv"
Send-MailMessage -To ($recipients.Email) -From "sender@example.com" -Subject "Email to List" -Body "Body of the email" -SmtpServer "smtp.example.com"
This approach streamlines the management of recipient lists and enhances your script's flexibility.
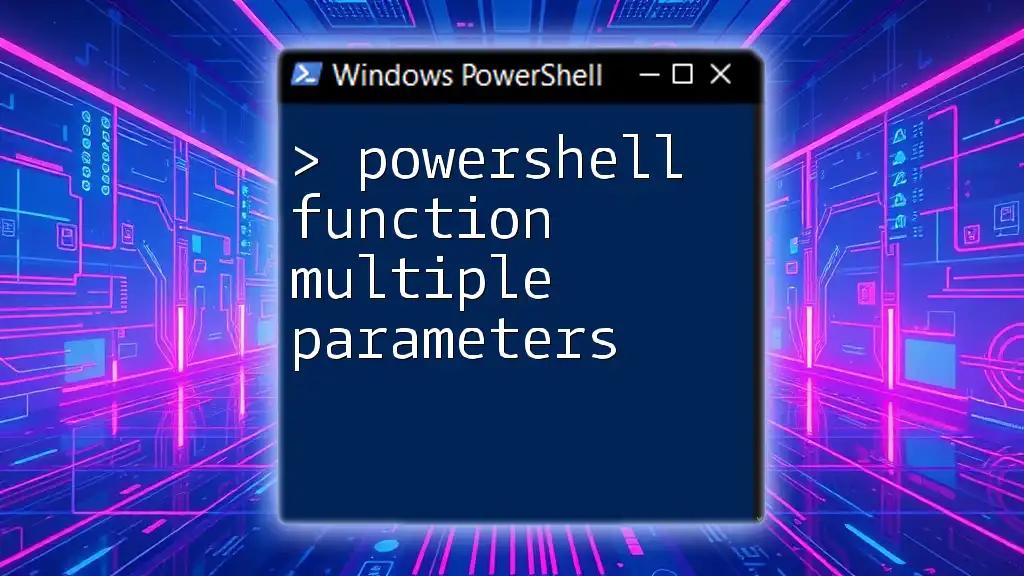
Error Handling and Troubleshooting
Common Issues When Sending to Multiple Recipients
When sending emails to multiple recipients, there are several common issues that may arise. These include:
- Invalid email addresses resulting in failure to send
- SMTP errors due to incorrect server configurations
- Delivery issues, such as bounce-back emails
Checking SMTP Server Settings
Verifying SMTP configurations
To troubleshoot sending issues, ensure that your SMTP settings are correct. You can test the connection to your SMTP server by using a simple test command. If you encounter problems, double-check your `-SmtpServer` address and any required credentials for authentication.

Best Practices for Sending Emails with PowerShell
Formatting Email Body
Taking the time to format your email body improves readability and enhances your message clarity. Consider using HTML to create visually appealing emails. This can capture attention and ensure key points are communicated effectively.
Here's an example of sending an HTML formatted email:
Send-MailMessage -To "user@example.com" -From "sender@example.com" -Subject "HTML Email" -Body "<h1>Hello</h1><p>This is an HTML email.</p>" -BodyAsHtml $true -SmtpServer "smtp.example.com"
Scheduling Email Alerts
Another valuable use of the Send-MailMessage cmdlet is to create automated email alerts triggered by specific events. By combining this cmdlet with Windows Task Scheduler, you can set up regular updates or alerts, further enhancing automation capabilities.

Conclusion
The PowerShell Send-MailMessage cmdlet provides a robust method for sending emails to multiple recipients quickly and efficiently. By employing the techniques covered in this article, you can enhance your automation scripts and ensure your communication flows smoothly within your organization. Embrace the power of PowerShell to manage your email notifications and become more efficient in your workflows. Consider joining our program to explore more concise and practical PowerShell tutorials!