The "Begin", "Process", and "End" blocks in a PowerShell function allow you to define distinct phases for your code execution, enabling you to handle initialization, input processing, and cleanup effectively.
Here's a simple example of using these blocks:
function Test-Pipeline {
[CmdletBinding()]
param (
[string]$InputString
)
begin {
Write-Host "Starting process..."
}
process {
Write-Host "Processing: $InputString"
}
end {
Write-Host "Process completed."
}
}
# Example usage
Test-Pipeline -InputString "Hello, PowerShell!"
Understanding PowerShell Cmdlets
What are Cmdlets?
Cmdlets are lightweight, single-function commands used in the Windows PowerShell environment. They are integral to the operation of PowerShell, as they enable advanced task automation and configuration management. Unlike traditional command-line commands, cmdlets follow a specific naming convention, typically Verb-Noun, which makes it easier for users to understand their purpose. For example, `Get-Process` retrieves information about running processes.
Importance of Cmdlet Parameters
Cmdlets often come with parameters, which add specific functionality and customize the command's behavior. For instance, the `-Name` parameter for `Stop-Process` allows you to specify which process to terminate. Understanding how to utilize cmdlet parameters effectively can greatly enhance your PowerShell scripting capabilities, allowing for more complex operations and increases in productivity.
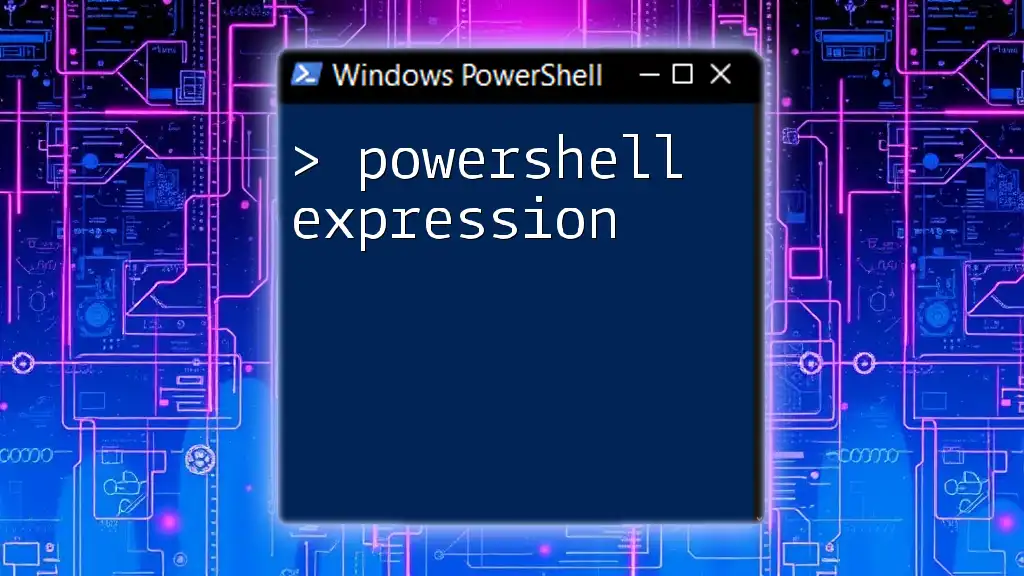
The Basics of PowerShell Functions
Why Use Functions in PowerShell?
Functions are blocks of code that perform a specific task and can be reused throughout your scripts. Utilizing functions promotes a modular approach to scripting, improving readability and maintainability. Instead of writing repetitive code, a function enables you to consolidate logic and call it multiple times with different parameters or input.
Example of a Simple PowerShell Function
Here’s a simple function that greets a user:
function Greet-User {
param (
[string]$UserName
)
Write-Host "Hello, $UserName!"
}
In this function, the param block defines the input parameter, allowing the user to pass their name when calling the function. The output, provided by Write-Host, is a friendly greeting displaying their name.
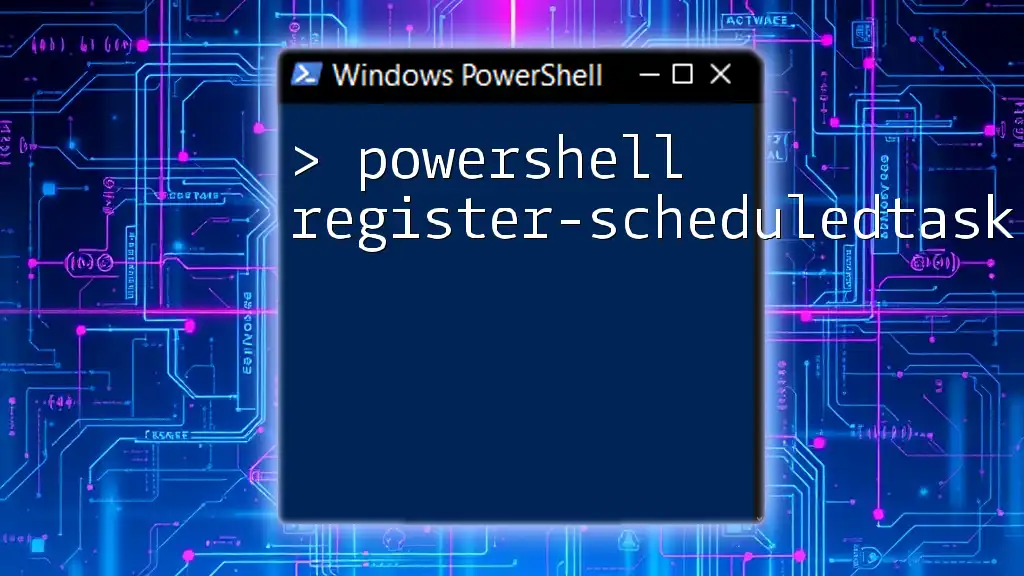
The Three Block Structure in PowerShell
What are Begin, Process, and End Blocks?
When creating functions in PowerShell, understanding the begin, process, and end blocks is essential for structuring your code efficiently.
Begin Block: This block runs once at the start of the function, making it ideal for initializing variables or setting up resources that will not change during the function execution. It prepares everything needed for the actual processing.
Process Block: This block is executed once for each input object that the function receives. It contains the main logic of the function and is where you perform operations on the items you are processing, making it critical to your script's functionality.
End Block: This block runs once after all input objects have been processed. Typically, it is used for cleanup actions, such as closing files, releasing resources, or displaying summaries.
Example of a Complete PowerShell Function with All Blocks
Below is an example of a function that employs all three blocks, reading a list of names and greeting each user:
function Greet-Users {
param (
[string[]]$UserNames
)
begin {
Write-Host "Preparing to greet users..."
}
process {
foreach ($UserName in $UserNames) {
Write-Host "Hello, $UserName!"
}
}
end {
Write-Host "All users have been greeted."
}
}
Explanation of the Code
- Begin Block: Indicates the start of the greeting process by outputting a preparatory message.
- Process Block: Iterates through each name provided in the `$UserNames` array, greeting users one by one.
- End Block: Concludes the operation with a summary message indicating that all users have been greeted.
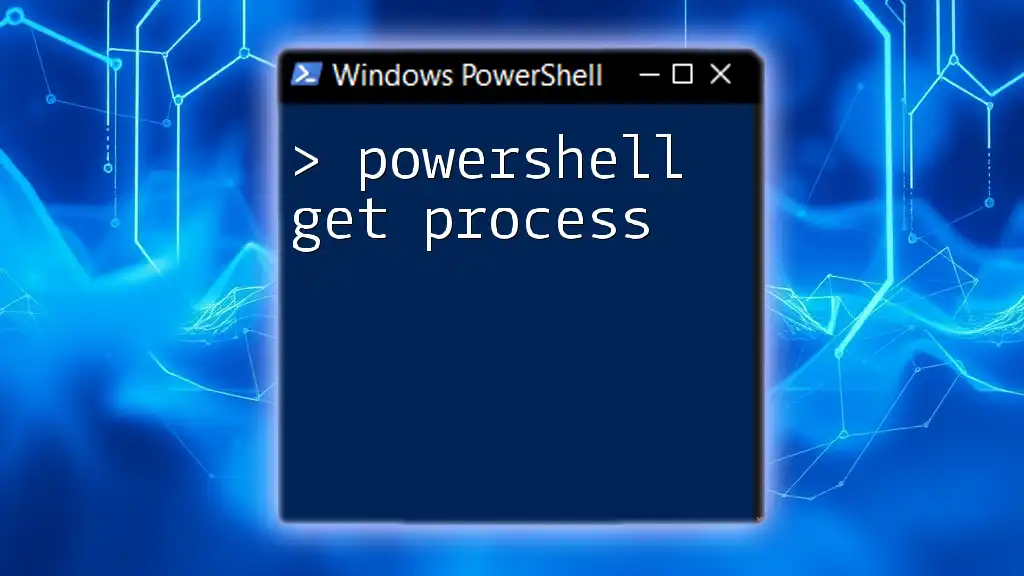
Practical Applications of Begin, Process, and End
Batch Processing with PowerShell
Batch processing is fundamental for automating repetitive tasks. For example, if you need to process a list of files, using the three blocks allows you to manage setup, processing, and cleanup efficiently.
Consider a scenario where you need to resize images in a directory:
function Resize-Images {
param (
[string[]]$ImagePaths
)
begin {
Write-Host "Starting to resize images..."
}
process {
foreach ($ImagePath in $ImagePaths) {
# Assuming a cmdlet Resize-Image that resizes the images
Resize-Image -Path $ImagePath -NewSize 800x600
}
}
end {
Write-Host "All images have been resized."
}
}
Handling Complex Data Types
PowerShell’s flexibility allows handling complex data types efficiently. For example, you can create a function that accepts an array of objects and performs operations based on their properties.
Example of a Function for Processing Objects
function Process-Users {
param (
[PSCustomObject[]]$Users
)
begin {
Write-Host "Processing user data..."
}
process {
foreach ($User in $Users) {
Write-Host "User: $($User.Name), Age: $($User.Age)"
}
}
end {
Write-Host "Processed all user data."
}
}
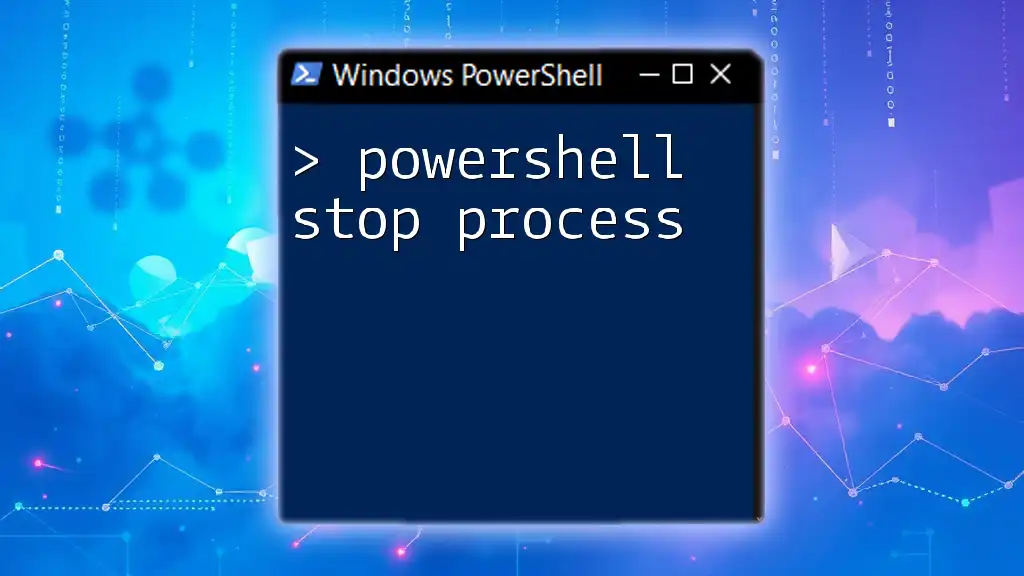
Performance Optimization Strategies
Optimizing PowerShell scripts is essential for better performance, especially when dealing with large datasets. Consider these strategies:
- Choose the Right Blocks: Use the begin block for one-time setup tasks, ensuring the process block is solely focused on the main logic.
- Limit Pipeline Input Sensibly: Instead of processing one item at a time, batch your input or use streaming to manage memory efficiently.
- Avoid Unnecessary Computations: Evaluate if calculations in the process block can be moved to the begin block.
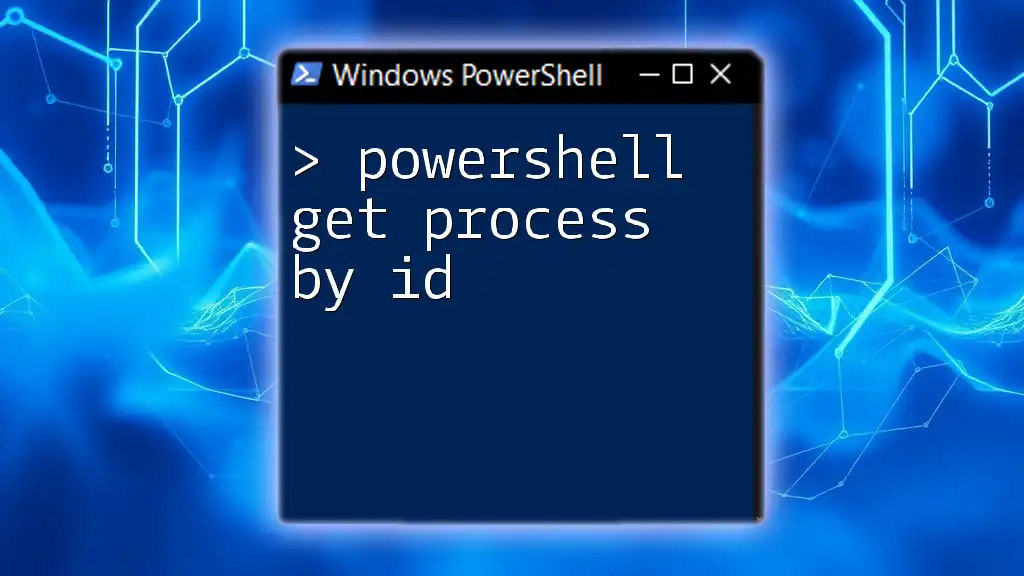
Advanced Techniques
Using Pipelining with Begin, Process, and End
Pipelining allows you to pass the output of one command directly into another command as input. This can be incredibly efficient in a function that utilizes the three blocks.
For instance, using `Get-Process` piped into your custom function to filter running processes.
Get-Process | Resize-Images
In such cases, the process block will handle each process flushed from the pipeline, optimizing operations in real-time.
Error Handling in PowerShell Scripts
Effective error handling is vital for robust scripting. Introducing try-catch statements within the blocks can help manage unexpected errors gracefully.
process {
try {
# Attempt to access resources
}
catch {
Write-Host "An error occurred: $_"
}
}
By implementing error handling, you ensure that your script behaves predictably even when faced with problems.
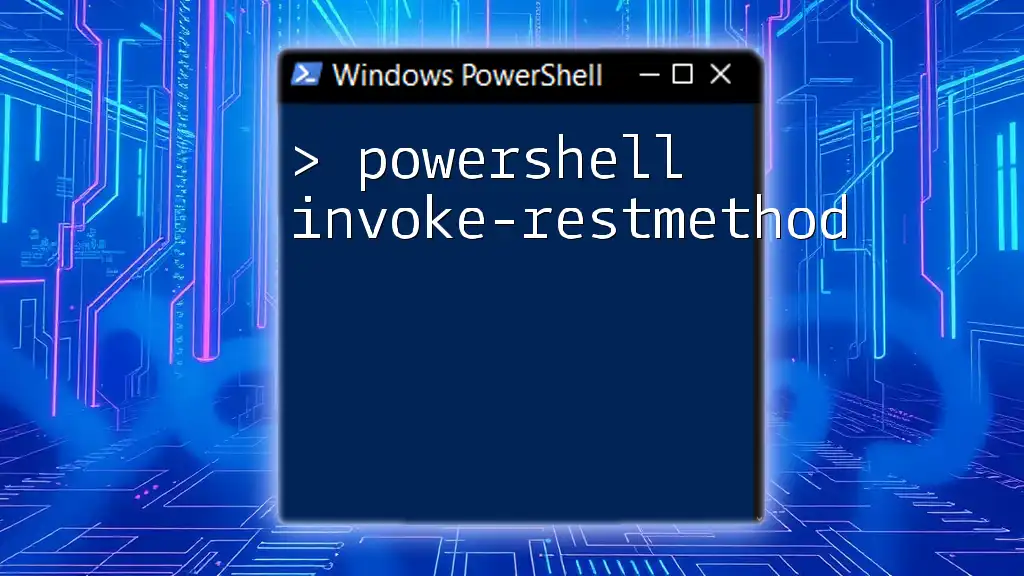
Conclusion
Utilizing PowerShell begin process end constructs is invaluable for writing organized, efficient, and manageable scripts. As you grow more comfortable with these structures, practice writing your functions that apply this methodology. Engaging with communities and exploration of advanced techniques will further enhance your PowerShell capabilities.
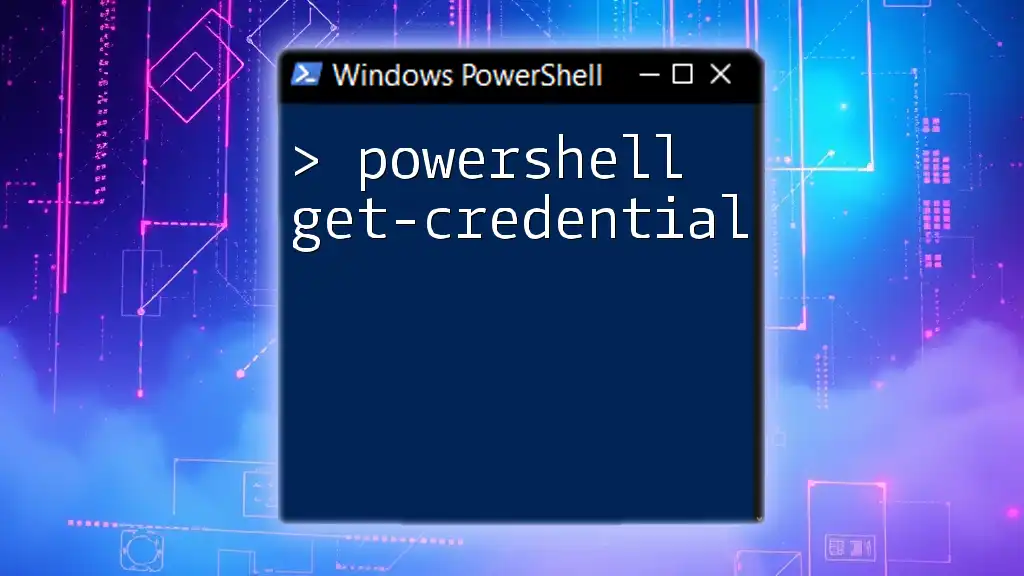
Additional Resources
Recommended Books and Tutorials
Consider exploring books and online resources like "Learn Windows PowerShell in a Month of Lunches" and instructional platforms such as Pluralsight or EdX, which offer comprehensive courses on PowerShell.
Community and Forums
Engaging with communities such as PowerShell.org or the PowerShell subreddit can provide insights and assistance in your learning journey.
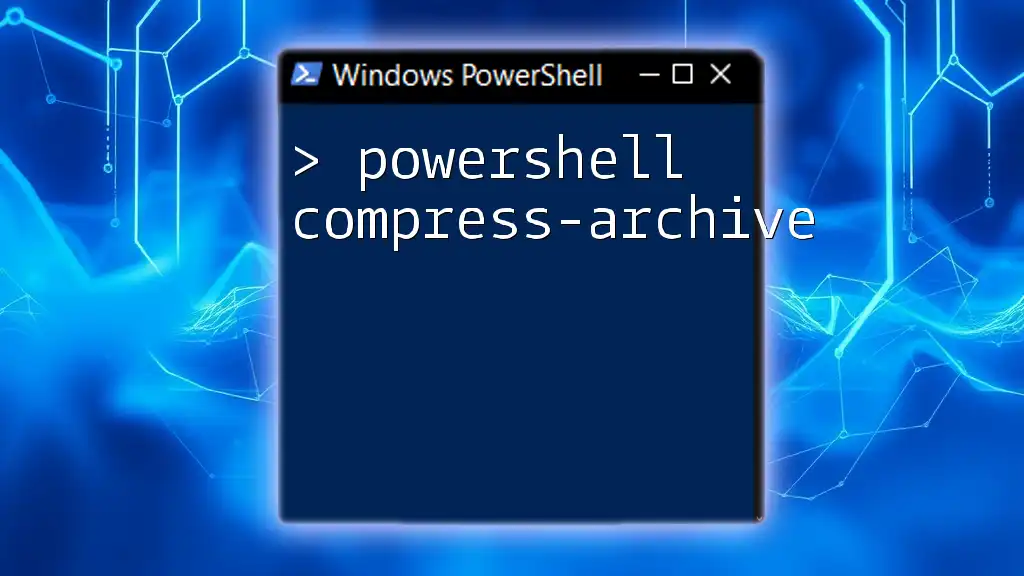
Call to Action
Join our training programs to deepen your understanding of PowerShell! Sign up for our workshops and receive the latest learning materials to advance your scripting skills.