You can pad a number with leading zeros in PowerShell by using the `-f` format specifier in a string, like this:
$number = 7
$formattedNumber = '{0:D5}' -f $number
Write-Host $formattedNumber
This will output `00007`, ensuring the number is always five digits long with leading zeros.
Understanding the Need for Leading Zeros
What are Leading Zeros?
Leading zeros are the zeros added before a number to ensure that it meets a certain length. This practice is often essential in programming and data presentation, as it keeps numerical data consistent and readable. For instance, if you have an ID system that assigns numbers in a sequence, such as 1, 2, 3, it is common to alter these values to 001, 002, 003 for uniformity, especially in databases or file systems.
Common Use Cases
Padding numbers with leading zeros is particularly useful in various scenarios:
- Formatting IDs: Ensuring that all identification numbers have the same character length enhances organization.
- Creating Structured Filenames: When generating files, such as reports or logs, applying leading zeros helps in chronological sorting.
- Database Entry Consistency: Having uniform data entries aids in ensuring accurate data retrieval and reduces potential errors.

PowerShell Basics for Padding Numbers
String Manipulation in PowerShell
In PowerShell, strings are pivotal for any text-based operation. Understanding string manipulation is vital for effectively padding numbers. A well-known method is string interpolation, which allows you to format strings dynamically at the time of execution.

Methods to Pad Numbers with Leading Zeros in PowerShell
Using String Format Method
The String Format Method is a robust way to add leading zeros to a number. It uses a specific syntax for formatting strings, where `"{0:D3}"` indicates that the number should be formatted to a minimum width of three digits, padding with zeros as necessary.
Here’s how it works:
$number = 5
$paddedNumber = "{0:D3}" -f $number
Write-Output $paddedNumber # Output: 005
In this example, the number 5 is transformed into 005, creating a three-digit display.
Using the PadLeft Method
An alternative and equally effective method is the `PadLeft` function. This method is particularly handy for strings and allows for padding from the left side.
To utilize it, you simply define the total length and the character to pad with. Here’s an example:
$number = "42"
$paddedNumber = $number.PadLeft(5, '0')
Write-Output $paddedNumber # Output: 00042
In this case, the string 42 is padded with three leading zeros, resulting in a total length of five characters.
Custom Function for Padding
Another approach is to create a reusable function that pads numbers based on user-specified parameters, enhancing flexibility in your scripts. Here’s a straightforward example:
function Pad-Number {
param (
[int]$number,
[int]$length
)
return "{0:D$length}" -f $number
}
Pad-Number -number 7 -length 4 # Output: 0007
This function, `Pad-Number`, can take any integer and a desired length, providing a consistent way to format numbers across your scripts.
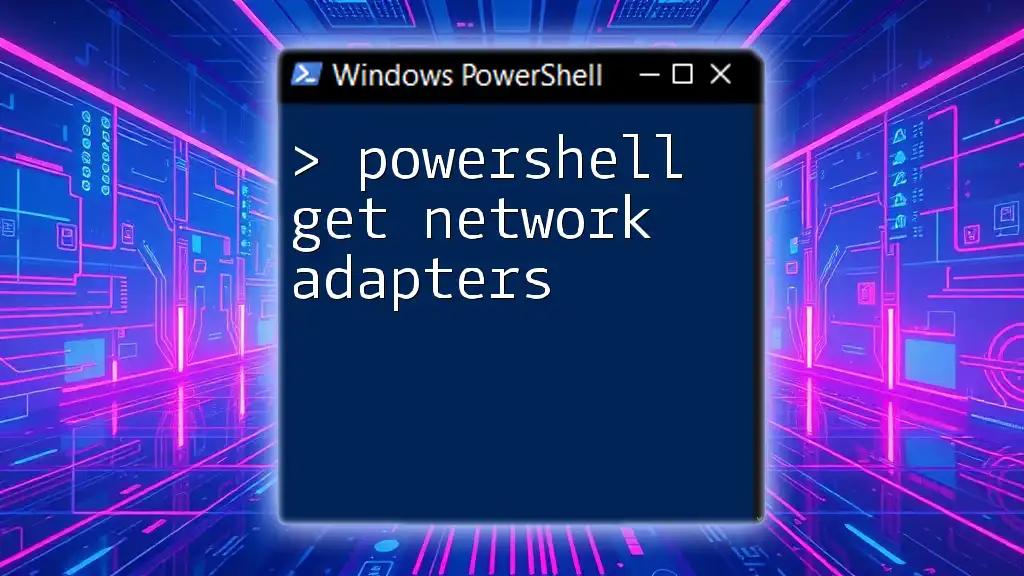
Advanced Techniques for Padding in PowerShell
Padding Numbers in Collections
PowerShell makes it easy to apply padding to multiple numbers at once using loops. For example, if you wanted to pad a range of numbers from 1 to 10, you could implement a loop alongside string formatting:
$numbers = 1..10
$paddedNumbers = foreach ($n in $numbers) { "{0:D2}" -f $n }
Write-Output $paddedNumbers # Output: 01, 02, 03, ...
Using `foreach`, each number in the array is iterated over, padded with leading zeros, and collected into a new array.
Formatting Date and Time with Leading Zeros
In many scenarios, especially in logging and reporting, formatting dates and times correctly is essential. You can use similar string formatting techniques to ensure components like hours and minutes are consistently two digits.
For instance:
$date = Get-Date
$formattedDate = "{0:yyyy-MM-dd HH:mm:ss}" -f $date
Write-Output $formattedDate # Output: 2023-10-01 12:01:59
In this command, the date output is formatted to ensure that both the date and time use leading zeros where appropriate, offering a consistent view.
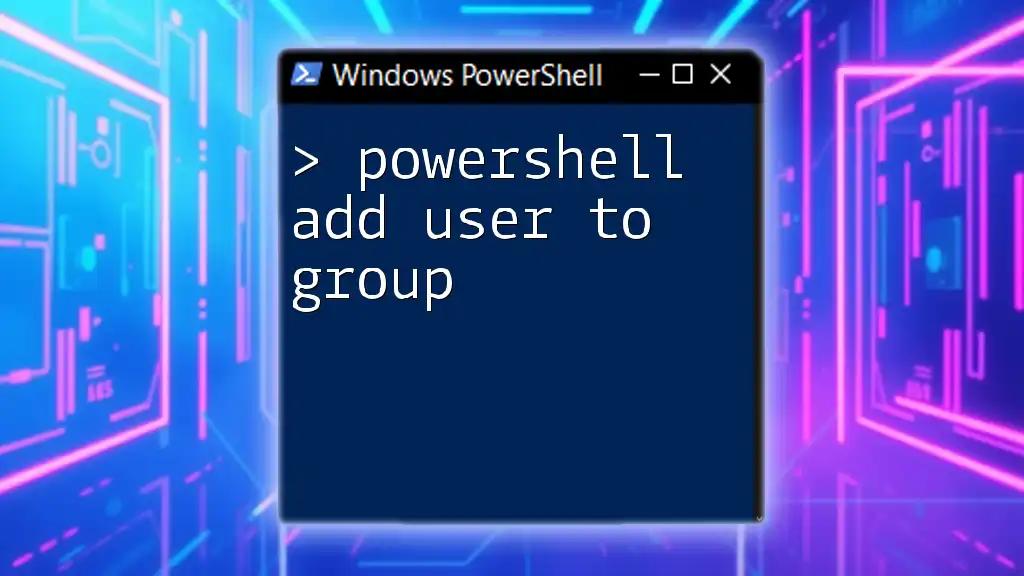
Error Handling and Best Practices
Common Errors to Avoid
When padding numbers, it's crucial to handle cases where padding may break your logic. Be mindful of potential pitfalls such as:
- Negative Numbers: Padding may yield unexpected results.
- Overly Large Values: Ensure that the padding logic does not truncate critical data.
Best Practices
To enhance efficiency and readability:
- Maintain Consistency: Always choose a padding size that fits your project’s needs to avoid inconsistencies.
- Reusability of Functions: Create functions like `Pad-Number` to streamline workflows across various scripts.
- Documentation and Comments: Always comment on your code to provide clarity, especially in areas where padding logic is applied.

Conclusion
By mastering the techniques for PowerShell pad number with leading zeros, you can greatly improve the readability and organization of your numerical data in scripts. Whether you’re generating filenames, formatting IDs, or preparing logs, these methods will ensure you maintain a high standard of data presentation.

Additional Resources
For further learning, consider exploring the official PowerShell documentation and engaging with community forums. Resources, tutorials, and courses will help solidify your understanding and application of these concepts as you develop your PowerShell skills.