To send an email with an attachment using PowerShell, you can utilize the `Send-MailMessage` cmdlet as shown in the code snippet below:
Send-MailMessage -From "you@example.com" -To "recipient@example.com" -Subject "Subject Here" -Body "Email body here" -SmtpServer "smtp.example.com" -Attachments "C:\path\to\your\file.txt"
Understanding PowerShell Email Functionality
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed specifically for system administration and automation tasks. It allows administrators to manage the system and process automation effectively, using command-line and scripting capabilities. PowerShell integrates with .NET, enabling the use of .NET methods and classes seamlessly.
Email Sending Capabilities of PowerShell
One of PowerShell's many capabilities is its ability to send emails directly from scripts. This can be particularly useful for automated notifications, alerts, or reports. The `Send-MailMessage` cmdlet is the main tool we will use to send emails. It leverages the Simple Mail Transfer Protocol (SMTP) for the sending process, which is a standard protocol used for sending emails across networks. Understanding SMTP settings is crucial for sending emails successfully from your PowerShell scripts.
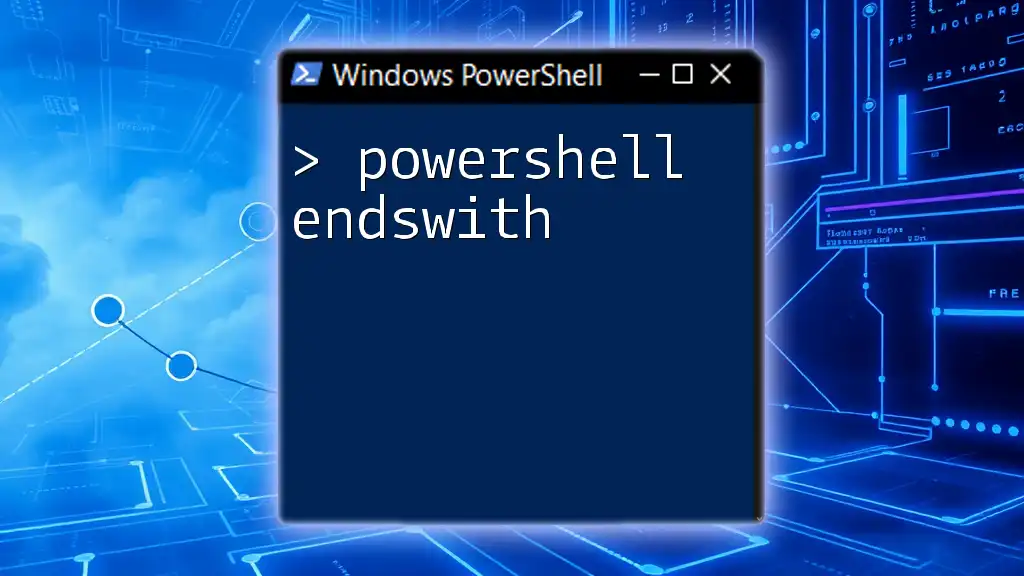
Prerequisites for Sending Email
PowerShell Version Requirements
Before diving into scripting, ensure your PowerShell version meets the minimum requirements. PowerShell 2.0 and above support the `Send-MailMessage` cmdlet. You can check your PowerShell version by running:
$PSVersionTable.PSVersion
SMTP Server Setup
To send emails, you must configure an SMTP server, which acts as a mail relay. Common SMTP servers include Gmail, Microsoft Exchange, and your own organization’s mail server. Each SMTP service has specific configurations essential for successful email dispatch. Take note of:
- SMTP server address: e.g., `smtp.gmail.com` for Gmail.
- Port number: Commonly, ports 25, 587 (TLS), or 465 (SSL) are used for SMTP.
- Authentication details: Some SMTP servers require authentication to send emails.
Make sure your email client settings (like those found in Outlook or any other mail client) are configured correctly to use the SMTP server, as you will need similar details in your script.
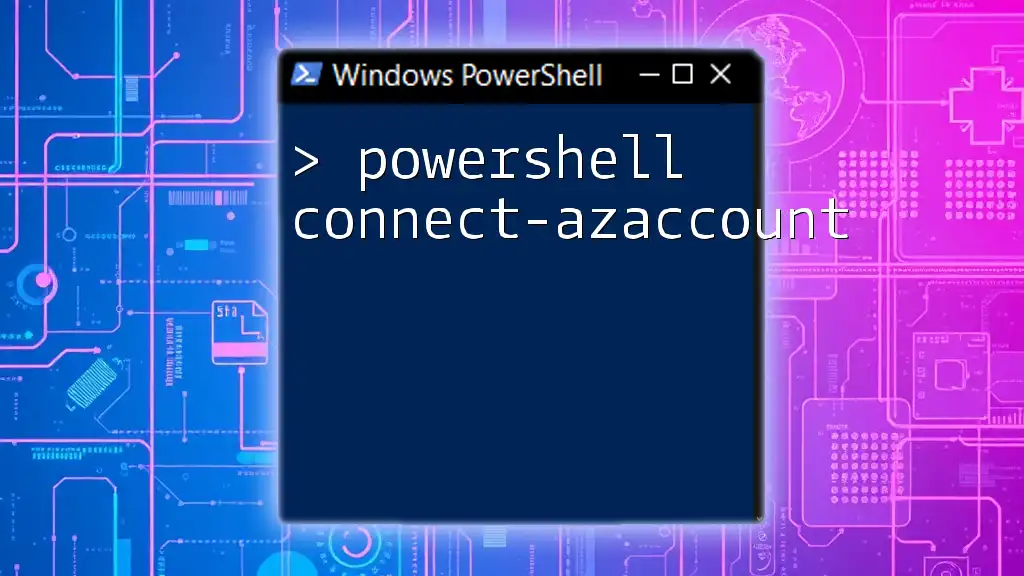
Crafting Your PowerShell Script
Basic Structure of a PowerShell Email Script
Understanding the fundamental parameters for the `Send-MailMessage` cmdlet is crucial. These parameters include:
- To: Recipient's email address.
- From: Sender's email address.
- Subject: Subject line of the email.
- Body: Content of the email.
Here is a basic example of a PowerShell email script without attachments:
$SmtpServer = "smtp.yourserver.com"
$From = "your_email@example.com"
$To = "recipient@example.com"
$Subject = "Test Email"
$Body = "This is a test email."
Send-MailMessage -SmtpServer $SmtpServer -From $From -To $To -Subject $Subject -Body $Body
This simple script connects to your SMTP server and sends an email. Make sure to replace the placeholders with your actual SMTP server information and email addresses.
Adding Attachments
How to Add an Attachment in PowerShell
Adding attachments to your emails is straightforward with PowerShell. You can include files in your email using the `-Attachments` parameter. Below is a code snippet illustrating how to send an email with an attachment:
$AttachmentPath = "C:\path\to\your\file.txt"
Send-MailMessage -SmtpServer $SmtpServer -From $From -To $To -Subject $Subject -Body $Body -Attachments $AttachmentPath
This script not only sends a basic email but also includes a file located at the specified path. Ensure that the file exists and that PowerShell has sufficient permissions to access it.
Crafting HTML Emails with Attachments
Sending HTML formatted emails can provide a more polished look. You can set the email body as HTML using the `-BodyAsHtml` parameter. Here’s how you can send an HTML email while including an attachment:
$Body = "<h1>This is a test email</h1><p>Your attached file is included below.</p>"
Send-MailMessage -SmtpServer $SmtpServer -From $From -To $To -Subject $Subject -Body $Body -BodyAsHtml -Attachments $AttachmentPath
In this example, the body of the email contains HTML content, enhancing its appearance. Make sure the recipient's email client supports HTML to display the email correctly.
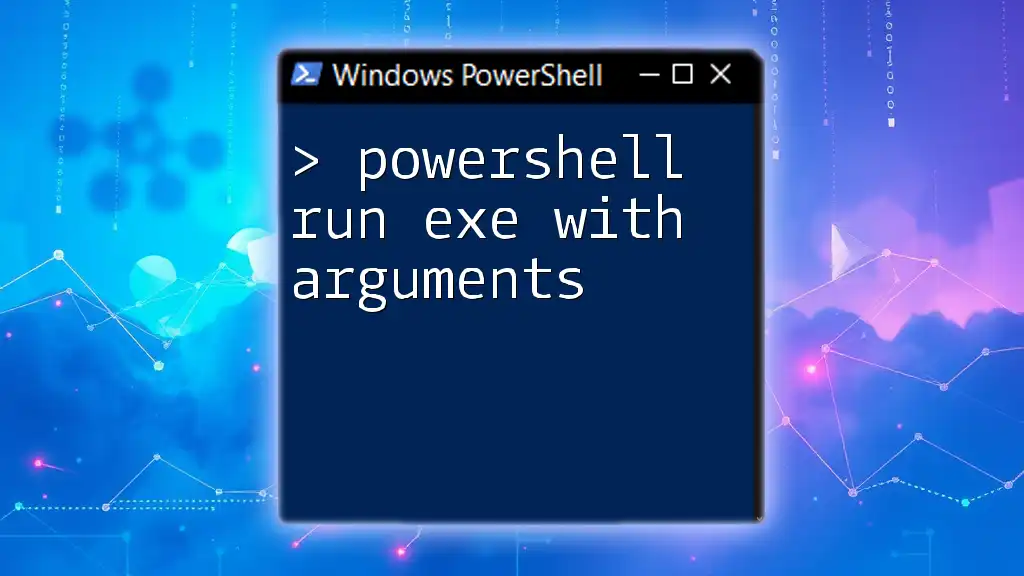
Error Handling and Troubleshooting
Common Errors and Their Solutions
When sending emails via PowerShell, you may encounter several common issues:
- SMTP Authentication Errors: Ensure your SMTP server credentials are correct and that the server allows sending from your email address.
- Network Issues: Check firewall settings or network configurations that might block SMTP traffic.
- File Not Found Errors: If your attachment path is incorrect or the file does not exist, you will get errors.
Debugging Tips for PowerShell Email Scripts
If problems arise when attempting to send an email, debugging is essential. Enabling verbose logging can assist in identifying issues. Use the `-Verbose` switch in your commands for detailed information. Additionally, reviewing SMTP server logs can provide insight into why an email may not have been sent.
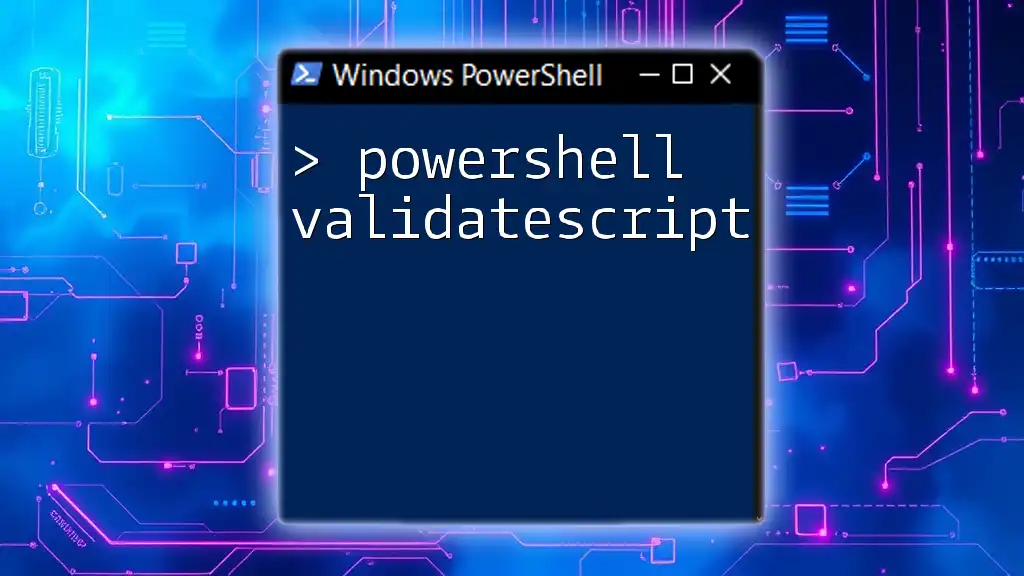
Best Practices for Sending Emails with PowerShell
Security Considerations
When dealing with emails and sensitive information, security is paramount. Always use secure connections by specifying SSL/TLS parameters when configuring your SMTP settings. This prevents your credentials and email data from being exposed.
It's also critical to sanitize email content to protect against injection attacks. Always ensure that the content you send is from a trusted source and appropriately cleansed of malicious input.
Automating Email Sending
You can automate your PowerShell email scripts with Windows Task Scheduler. Scheduling scripts can help streamline tasks like sending daily reports or alerts at specific intervals.
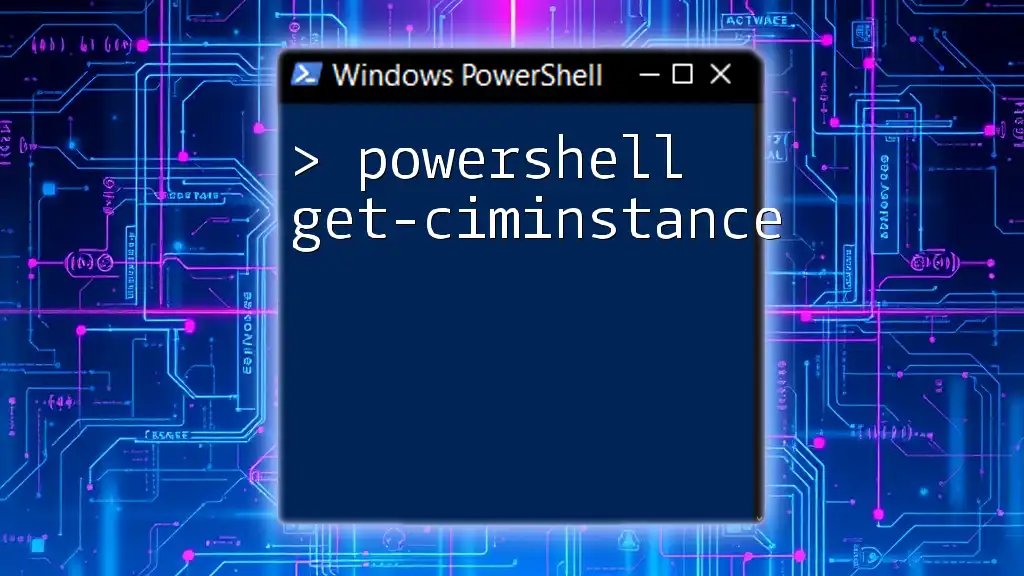
Conclusion
In this guide, we have covered how to use PowerShell to send emails with attachments effectively. By following the explained steps and utilizing provided examples, you can automate your email notifications and improve communication processes.
Explore and experiment with your PowerShell scripts to unlock the full potential of automation in your workflows. Embrace the convenience and efficiency that comes with mastering the PowerShell send email with attachment functionality!
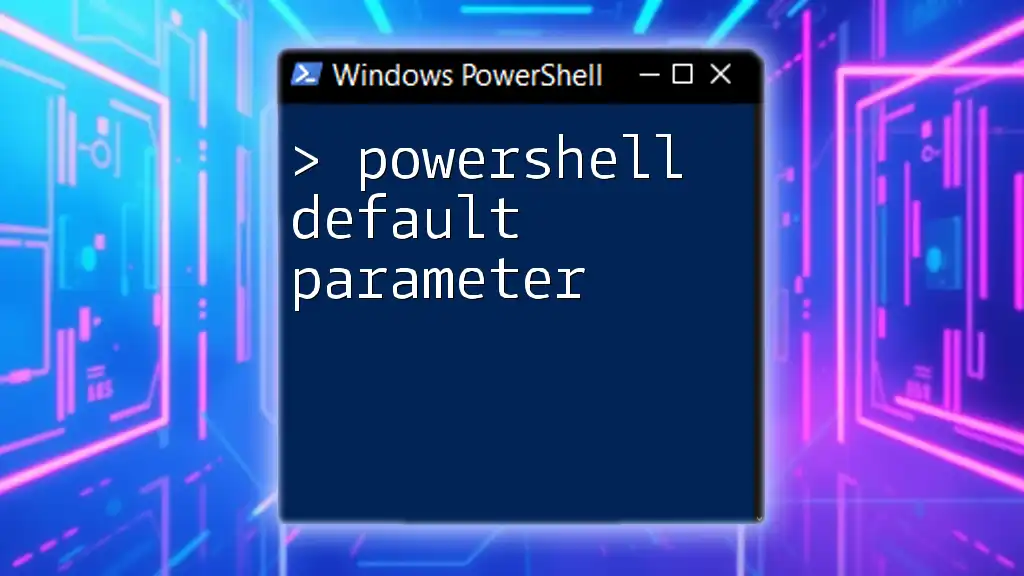
Additional Resources
For further mastery, consider exploring PowerShell community forums, blogs, and documentation resources. Engaging with the community can provide insights and support as you develop your PowerShell skills.