To round a number to two decimal places in PowerShell, you can use the following command:
[math]::round($number, 2)
Replace `$number` with the value you wish to round.
Understanding Rounding in PowerShell
What is Rounding?
Rounding refers to the process of reducing the number of decimal places in a number while maintaining its approximate value. This is crucial in programming and data analysis, particularly in financial calculations, where precise numerical data is essential for reporting, budgeting, and forecasts.
Types of Rounding Methods
There are several rounding methods you can utilize in PowerShell:
- Round Half Up: This method rounds a number to the nearest value, and if the number is exactly halfway between two integers, it rounds up.
- Round Half Even: Also known as banker's rounding, this method rounds to the nearest even number when the number is exactly halfway, reducing the bias that can accumulate when adding rounded numbers.
- Truncation: This approach simply removes the decimal places without rounding, which may lead to inaccuracies but provides straightforward results.
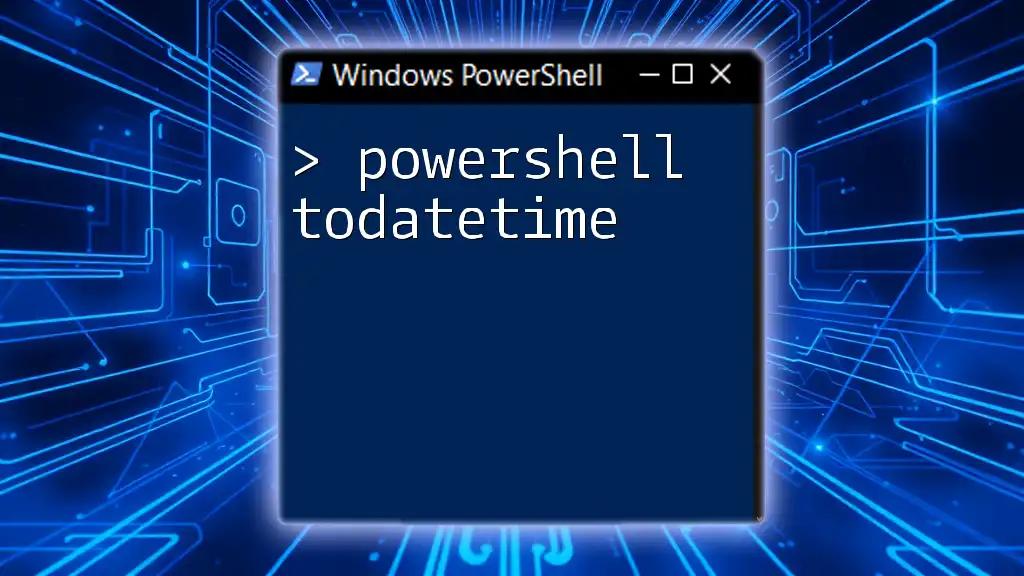
Rounding Numbers to Two Decimal Places
Using the `[math]::round()` Method
PowerShell leverages .NET's functionality for mathematical operations. The `[math]::Round()` method is one of the simplest ways to achieve rounding to two decimal places.
Syntax:
[math]::Round(value, digits)
Example: Suppose you have a decimal number and want to round it to two decimal places:
$number = 2.34567
$roundedNumber = [math]::Round($number, 2)
Write-Output $roundedNumber # Output: 2.35
In this example, PowerShell rounds the number 2.34567 to 2.35, demonstrating how effective this method is.
Custom Rounding Function
To enhance flexibility in your PowerShell scripts, you can create a custom rounding function that allows you to specify the number of decimal places.
Code Snippet:
Function Round-ToDecimal {
Param (
[decimal]$Value,
[int]$DecimalPlaces = 2
)
return [math]::Round($Value, $DecimalPlaces)
}
Usage Example: When you want to round a specific value using this function, you can invoke it like so:
Round-ToDecimal -Value 2.3489 -DecimalPlaces 2 # Output: 2.35
This function not only simplifies rounding but also provides the opportunity to round to anywhere between zero to multiple decimal places, depending on your needs.
Formatting Output for Readability
Rounding isn’t just about numeric precision; it’s also about how the information is presented. You can use PowerShell’s formatting capabilities to display rounded numbers attractively.
Using `-f` Operator
The `-f` format operator can be particularly handy for zero-padding and controlling the number of decimal places in output.
Example:
$number = 2.5
$formattedNumber = "{0:N2}" -f $number
Write-Output $formattedNumber # Output: 2.50
Here, the output is formatted to show two decimal places, even if the original number is a whole number.
Using `ToString()` Method
Another way to format numbers is through the `ToString()` method, which allows for more granular control over how you represent numbers.
Code Snippet:
$number = 2.45678
$formattedString = $number.ToString("N2")
Write-Output $formattedString # Output: 2.46
This method rounds the number properly and formats it, ensuring clarity in presentation.
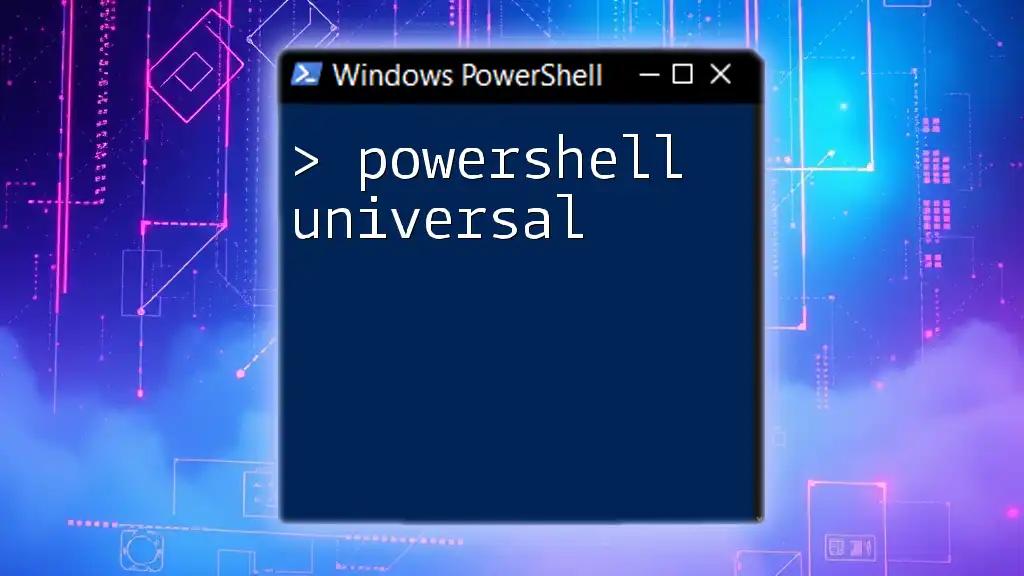
Rounding in Arrays and Collections
Applying Rounding to Each Element
When dealing with an array of numbers, it’s efficient to round them all in one go. PowerShell provides several tools to iterate through collections seamlessly.
Code Snippet:
$numbers = 1.2345, 2.3456, 3.4567
$roundedNumbers = $numbers | ForEach-Object { [math]::Round($_, 2) }
Write-Output $roundedNumbers # Output: 1.23, 2.35, 3.46
In this example, each number in the array is individually rounded to two decimal places using the `ForEach-Object` cmdlet.
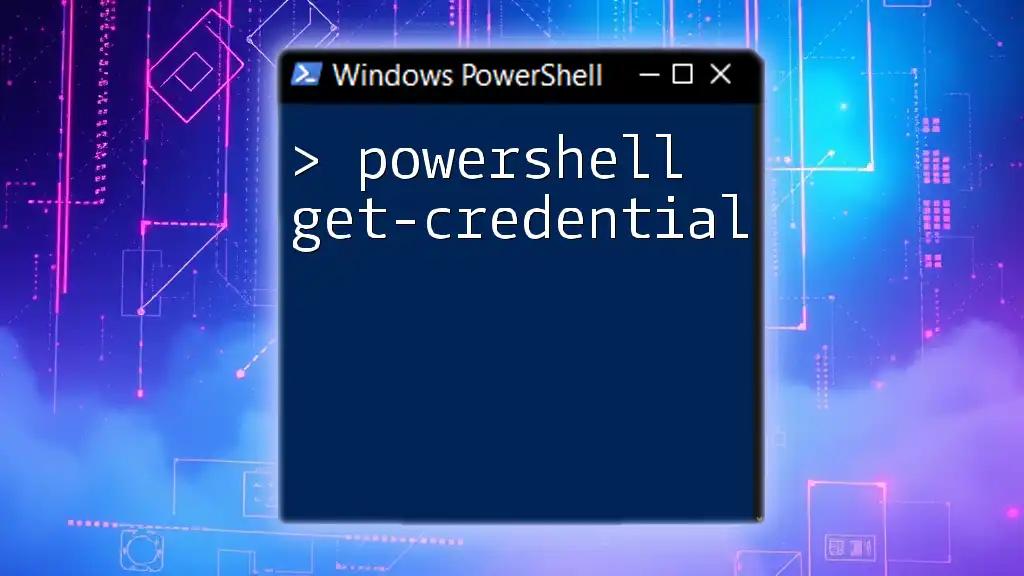
Handling Edge Cases
Rounding Negative Numbers
Rounding works consistently across positive and negative values, but it’s essential to understand how this applies when rounding negative numbers.
Example:
$negativeNumber = -2.34567
$roundedNegative = [math]::Round($negativeNumber, 2)
Write-Output $roundedNegative # Output: -2.35
Notably, the rounded result remains logically consistent, mirroring how the rounding would proceed with positive numbers.
Rounding Zero and Whole Numbers
Rounding behaves similarly with zero and whole numbers, providing an opportunity to maintain format consistency.
Example:
$zero = 0
$roundedZero = [math]::Round($zero, 2)
Write-Output $roundedZero # Output: 0.00
For whole numbers:
$wholeNumber = 5
$roundedWhole = [math]::Round($wholeNumber, 2)
Write-Output $roundedWhole # Output: 5.00
Both examples ensure that even unmodified numbers display a consistent format when rounded.
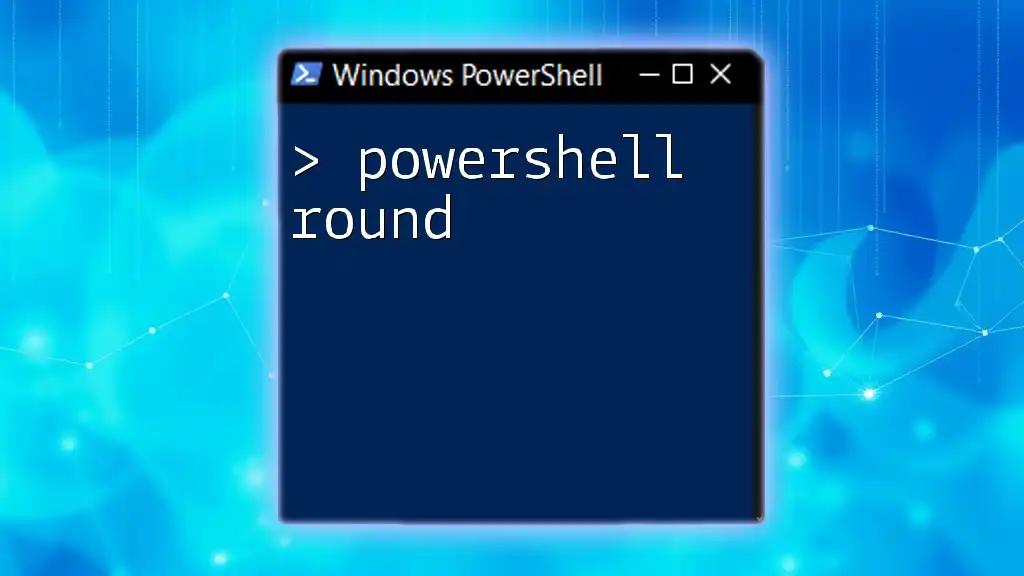
Conclusion
Mastering the ability to PowerShell round to 2 decimal places is invaluable for developers, data analysts, and anyone working with numbers in PowerShell. Whether through built-in methods or custom functions, rounding preserves data integrity and presents information in a user-friendly manner.
With the techniques outlined in this guide, you're equipped with the knowledge to effectively manage rounding in your PowerShell scripts. Embrace the practice by trying out these examples and discovering how they can improve your coding workflow.
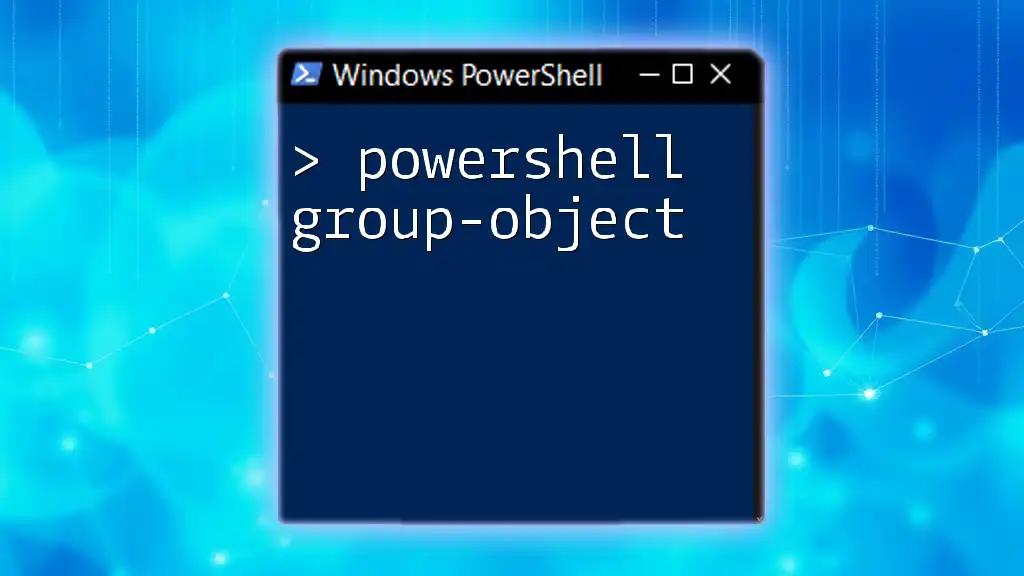
Additional Resources
For further learning, consider exploring PowerShell's official documentation or delving into online courses focused on mastering PowerShell scripting.
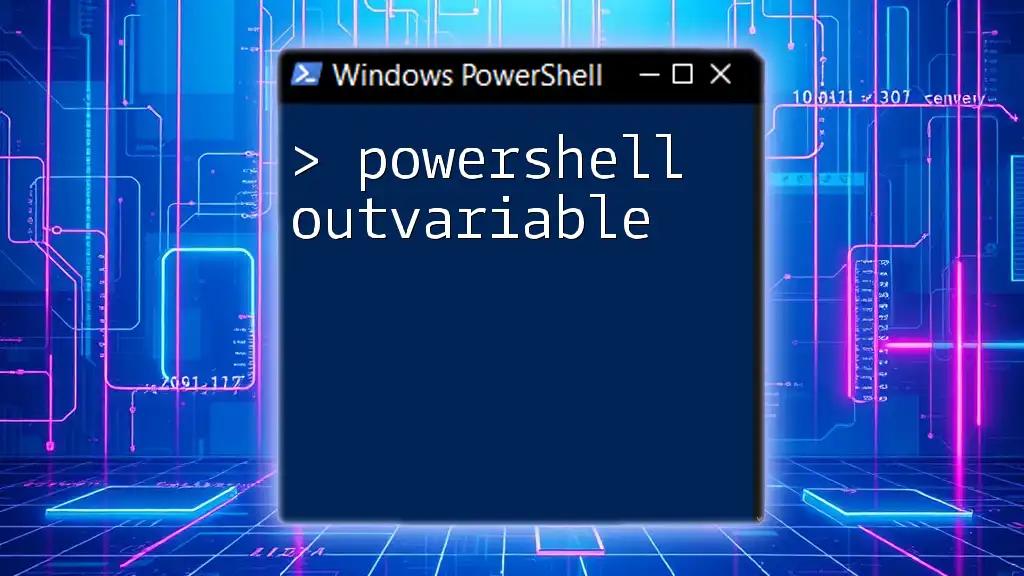
FAQ Section
Common Questions About Rounding in PowerShell
What happens if I round a number with more than two decimal places?
When a number with more than two decimal places is rounded, PowerShell will trim or round the excess decimals according to the specified method (e.g., round half up, round half even).
Can I round to a different number of decimal places?
Yes! By using the `[math]::Round()` method or your custom function, you can easily specify the desired number of decimal places to round to.
What are some common pitfalls to avoid?
Be mindful of potential errors when rounding negative numbers or very large floating-point numbers, as precision may vary due to floating-point representation in computers.