In PowerShell, you can round down a number to the nearest whole integer using the `[math]::floor()` method.
$number = 6.78
$roundedDown = [math]::floor($number)
Write-Host "Rounded down value: $roundedDown"
Understanding Rounding in Programming
Rounding is a fundamental concept in programming that simplifies numbers to make them easier to work with. Typically, rounding is used to reduce the number of decimal places in a number, which can be important for financial calculations, data analysis, and reporting.
When it comes to rounding methods, there are various approaches:
- Rounding Up: This method rounds a number to the next highest integer.
- Rounding Down: This is the focus of our article, where numbers are rounded to the nearest lower integer.
- Rounding to Nearest: This method rounds to the nearest integer, moving up or down based on the decimal value.
The significance of rounding down lies in its applications. For example, in financial calculations, it's crucial to avoid overestimating costs or revenues, and rounding down can ensure more accurate results. Additionally, in data analysis, rounding down can help maintain consistency in results, especially when dealing with large datasets or thresholds.
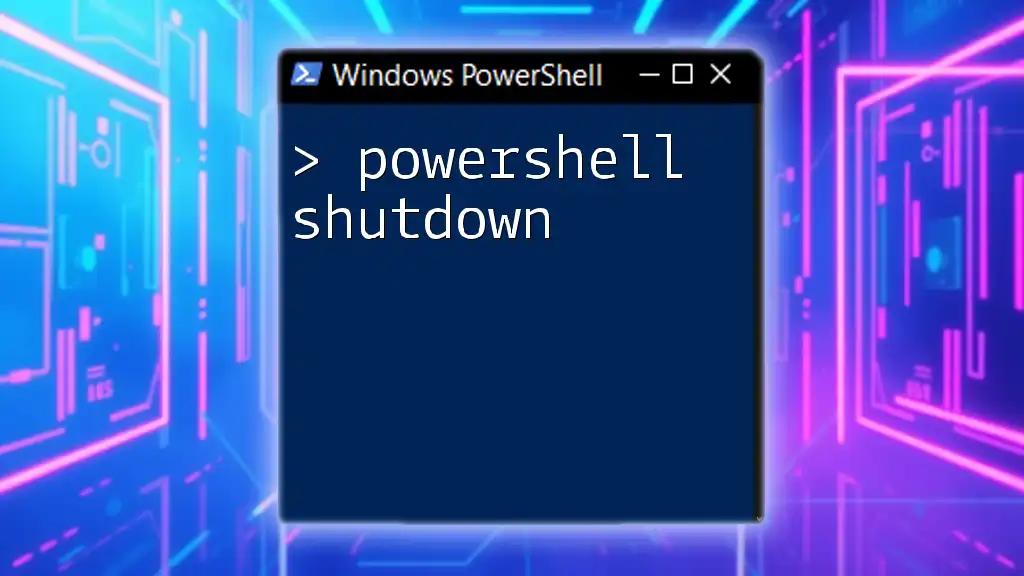
PowerShell Rounding Functions
In PowerShell, arithmetic operations and rounding are made simple through built-in functions. One of the primary methods for rounding down is using the `Floor` method from the `System.Math` class, which is a core part of the .NET framework.
Using `[math]::Floor()` for Round Down
The `Floor` method effectively truncates a decimal number to the largest integer less than or equal to the number. This is the essence of rounding down.
Example
$number = 3.7
$roundedDown = [math]::Floor($number)
Write-Output $roundedDown # Output: 3
This snippet demonstrates how a floating-point number like `3.7` gets rounded down to `3`.
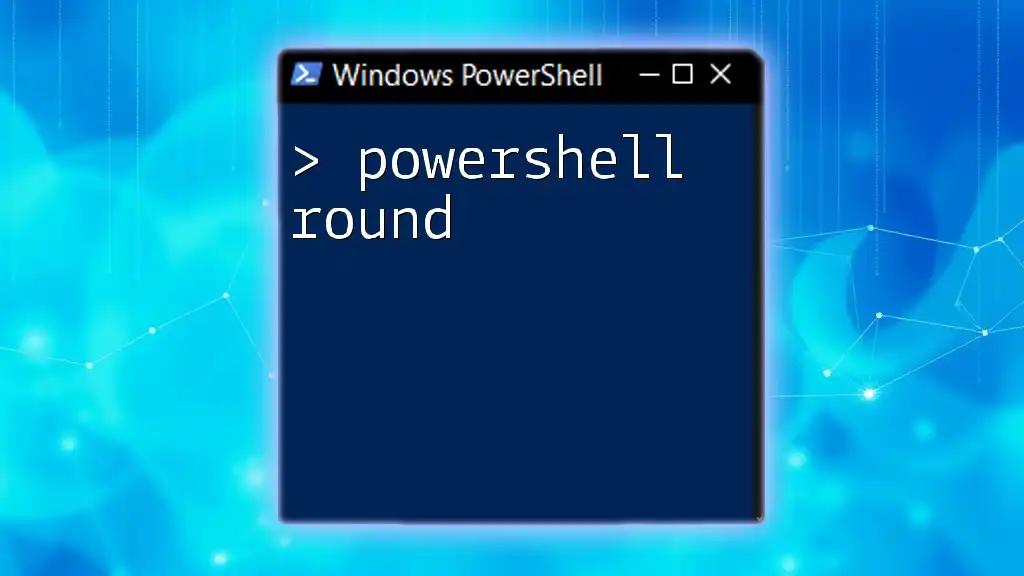
Practical Applications of Rounding Down
Rounding down has various applications in different fields. Below are some highlighted areas:
Financial Calculations
In financial applications, rounding down can help avoid inflated figures. Consider a scenario involving price calculations where a business needs to apply a discount.
Example
$price = 19.99
$discountedPrice = 15.50
$finalPrice = [math]::Floor($price - $discountedPrice)
Write-Output $finalPrice # Output: 4
In this case, the final price is calculated by deducting the discounted price from the original price and then rounding down to avoid presenting a misleading figure.
Data Analysis and Reporting
In data analysis, especially when preparing datasets for reports, rounding down can help in maintaining uniformity in the presentation of results.
Example
$dataSet = @(2.4, 3.8, 5.1)
$roundedDataSet = $dataSet | ForEach-Object { [math]::Floor($_) }
Write-Output $roundedDataSet # Output: 2, 3, 5
In this snippet, a set of decimal numbers is processed, each being rounded down to provide a clearer representation of the data.

Using Custom Functions for Rounding Down
Creating a custom function can further simplify the process of rounding down. This encapsulation allows you to reuse the logic effortlessly throughout your scripts.
Creating a Custom Round Down Function
The following function illustrates how you can set up a simple round down utility in PowerShell:
function Round-Down {
param (
[double]$inputNumber
)
return [math]::Floor($inputNumber)
}
# Usage
$result = Round-Down 4.6
Write-Output $result # Output: 4
In this example, we define a function called `Round-Down` that takes a floating-point number and returns its rounded-down value. When we call this function with `4.6`, the output will be `4`.
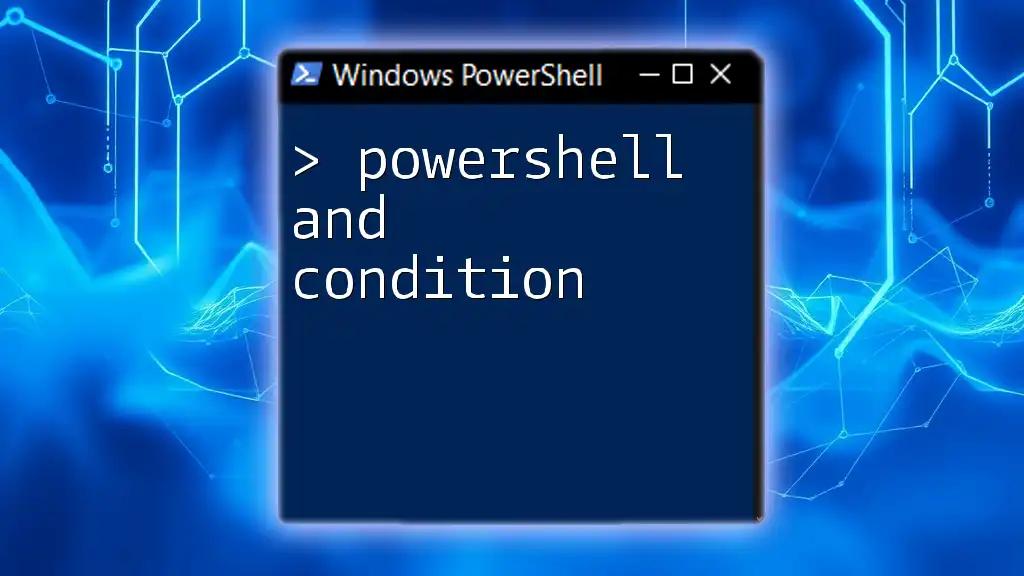
Common Mistakes When Rounding Down
While rounding down is straightforward, it’s important to be aware of common mistakes:
Data Type Issues
One common pitfall relates to data types. PowerShell can handle both integers and floating-point numbers, but incorrectly assuming a number’s type can lead to unexpected results. Ensure the input is indeed a number before processing. For instance, if a variable is treated as a string, the try to round will lead to errors.
Handling Special Cases
Rounding negative numbers can also be confusing. For example, using `[math]::Floor()` on `-2.3` will yield `-3`, which may not be intuitive for all users. Always consider how your rounding logic impacts negative values.
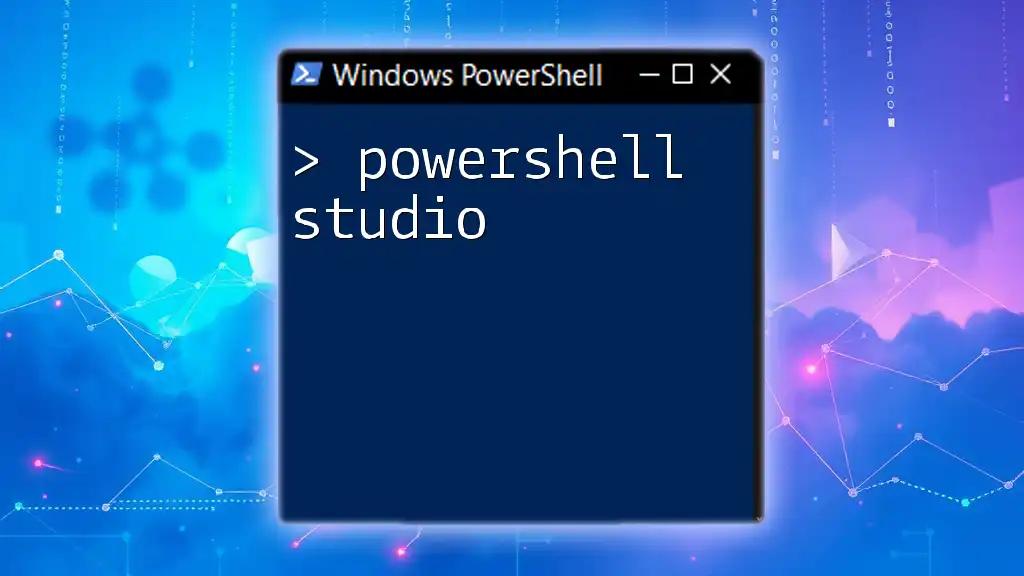
Conclusion
In conclusion, using PowerShell round down techniques can significantly enhance your scripting capabilities. Whether it's in financial calculations, data analysis, or creating reusable functions, understanding the `Floor` method and how to implement your custom logic is invaluable.
Taking the time to master these techniques will lead to more accurate outcomes and greater efficiency in your PowerShell scripts.
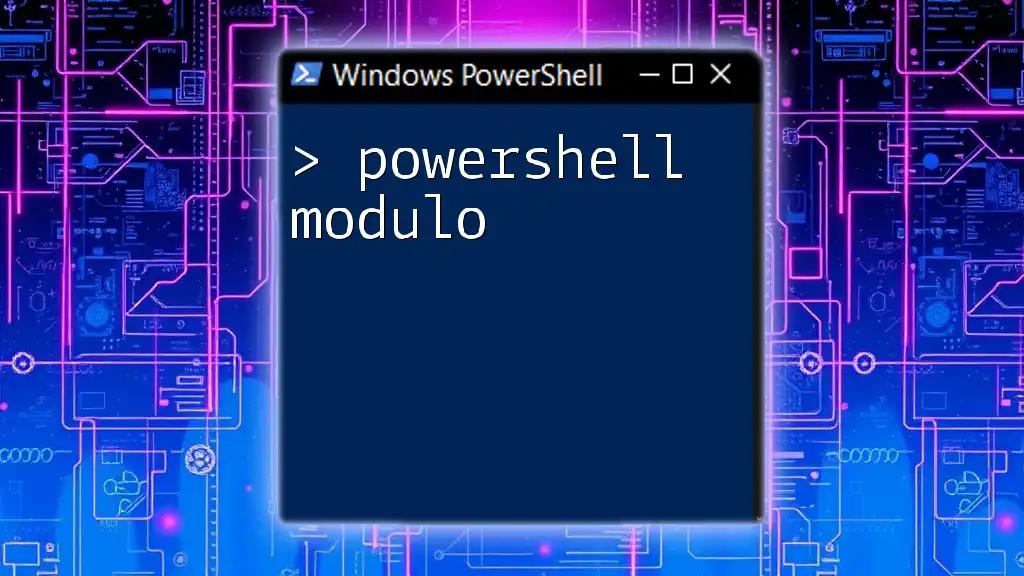
Additional Resources
For more insights into PowerShell and its capabilities, explore official Microsoft documentation and consider engaging with online PowerShell communities such as PowerShell.org and Stack Overflow for support and continuous learning.

Call to Action
If you are eager to learn more about PowerShell commands or want to enhance your skills, consider joining our community or signing up for our upcoming workshops. We look forward to guiding you through the world of PowerShell and helping you become a proficient user!