Unlock your tech potential with our "PowerShell Zero to Hero" program, designed to transform beginners into proficient PowerShell users through quick, practical lessons.
Write-Host 'Hello, World!'
Getting Started with PowerShell
Installing PowerShell
To begin your journey from PowerShell Zero to Hero, you'll first need to install PowerShell on your system. PowerShell is available on multiple platforms, including Windows, macOS, and Linux. It's essential to differentiate between Windows PowerShell and PowerShell Core. The latter is cross-platform, allowing for more versatility in diverse environments.
- For Windows users, PowerShell comes pre-installed. For others, installation can be performed by downloading the installer from the official [PowerShell GitHub page](https://github.com/PowerShell/PowerShell).
- For macOS and Linux, you can install PowerShell via package managers such as Homebrew for macOS or APT for Ubuntu.
Launching PowerShell
Once installed, you can launch PowerShell in several ways, including:
- Windows Terminal: A modern terminal application that supports multiple shells.
- PowerShell ISE: This Integrated Scripting Environment provides a user-friendly interface for scripting.
- Visual Studio Code: A powerful code editor with PowerShell extensions for enhanced functionality.
Understanding the PowerShell Environment
Understanding the difference between CLI and ISE is crucial for effective usage. The command prompt in CLI allows for quick commands, while ISE provides better error handling and debugging capabilities.
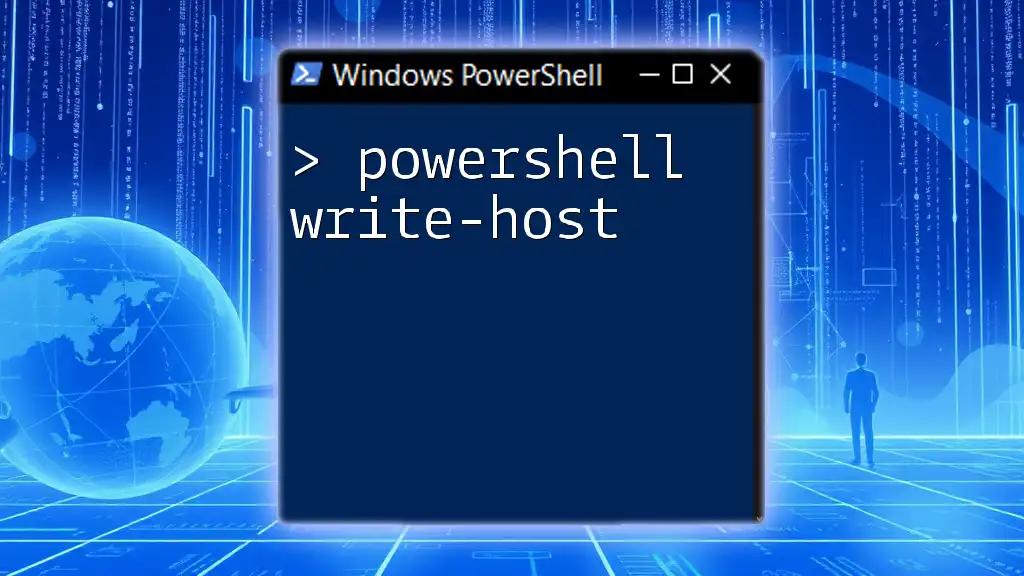
The Basics of PowerShell Commands
Command Structure
PowerShell commands, also known as cmdlets, adhere to a specific syntax: verb-noun. Understanding this structure is foundational for mastering PowerShell.
For instance, the command:
Get-Process -Name "powershell"
Here, `Get` is the verb, and `Process` is the noun. The command fetches the process information for PowerShell specifically. Getting familiar with this format will allow you to quickly understand and construct your own commands.
Common Cmdlets
Here are a few essential cmdlets you'll encounter frequently:
- `Get-Help`: This command provides assistance on how to use other cmdlets. For example, running `Get-Help Get-Process` will give you detailed information about the `Get-Process` cmdlet.
- `Get-Command`: This retrieves all available commands in your session, which can help you discover new cmdlets.
- `Get-Service`: Returns the status of services on your system.
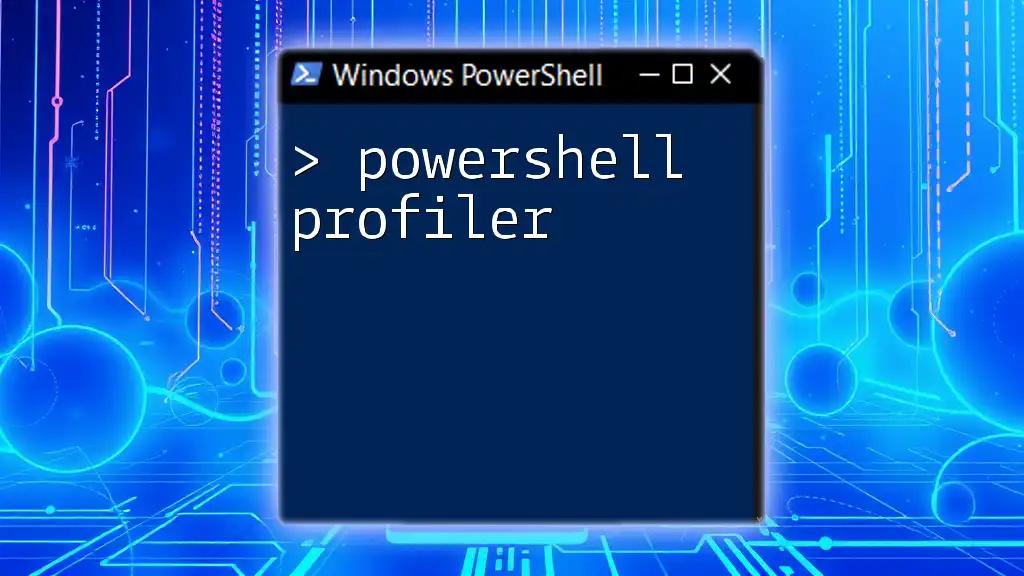
Using Variables and Data Types
Creating and Using Variables
One essential aspect of programming in PowerShell is the ability to create and utilize variables. You declare variables using a dollar sign `$`, followed by the variable name.
$greeting = "Hello, PowerShell!"
This command assigns the string "Hello, PowerShell!" to the variable `$greeting`. To display the content of the variable, simply use:
Write-Host $greeting
Understanding Data Types
PowerShell supports various data types, including strings, integers, arrays, and hashtables. Understanding these types will make your scripts more efficient.
For instance, declaring and using an array is straightforward:
$fruits = @("Apple", "Banana", "Cherry")
You can access individual elements by their index, which is zero-based:
$fruits[0] # This will return "Apple"
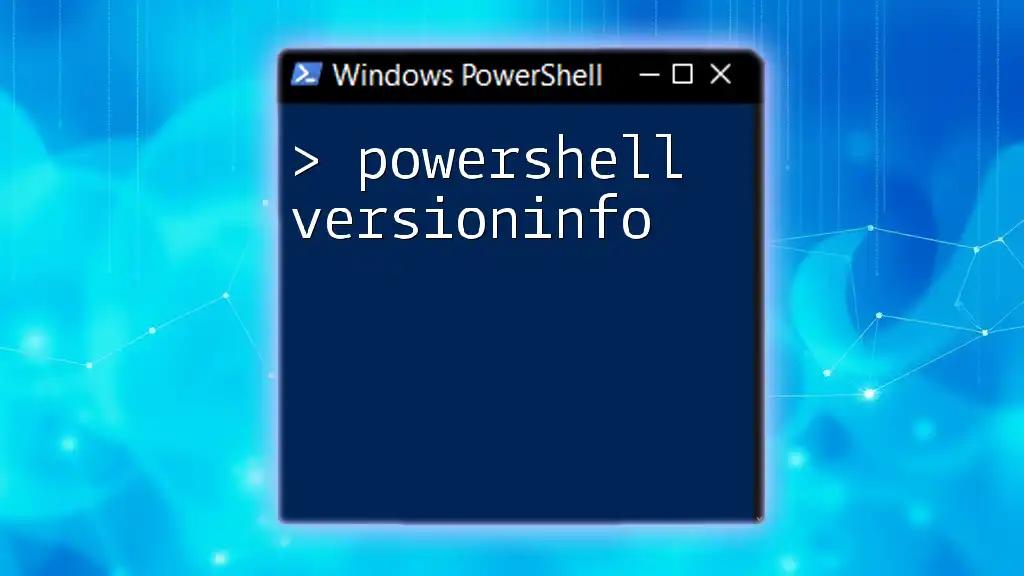
Control Flow in PowerShell
Conditional Statements
Conditional statements allow your scripts to make decisions based on specific conditions, using keywords like `if`, `else`, and `switch`.
For example:
if ($greeting -eq "Hello, PowerShell!") {
Write-Host "You are welcome!"
} else {
Write-Host "Greetings not recognized."
}
This code checks if the variable `$greeting` matches a specific string and outputs a corresponding message.
Loops
Loops are vital for executing repetitive tasks efficiently. There are several types: `for`, `foreach`, `while`, and `do-while`.
Here’s an example of a `foreach` loop, which iterates over each item in an array:
foreach ($fruit in $fruits) {
Write-Host $fruit
}
This will print each fruit on a new line.

Working with Files and Directories
File System Navigation
Navigating the file system is a critical skill in PowerShell. You can list files and directories using:
Get-ChildItem -Path C:\Users\YourUsername\Documents
This command will display all items within the specified directory.
Reading and Writing Files
PowerShell allows efficient file management with cmdlets like `Get-Content` for reading files and `Set-Content` for writing to files. For instance, to read a text file, you can use:
Get-Content -Path "C:\example.txt"
This command fetches and displays the contents of `example.txt`.

Scripting in PowerShell
Creating Your First Script
Creating a PowerShell script is as easy as writing a series of commands into a `.ps1` file using any text editor. Here’s an example of a simple script that defines a greeting:
Write-Host "Welcome to your PowerShell journey!"
Running Scripts and Execution Policies
PowerShell has built-in security mechanisms to safeguard against untrusted scripts. Before running your script, you may need to adjust the execution policy:
Set-ExecutionPolicy RemoteSigned
This command allows scripts created locally to run while enforcing safety checks on downloaded scripts.
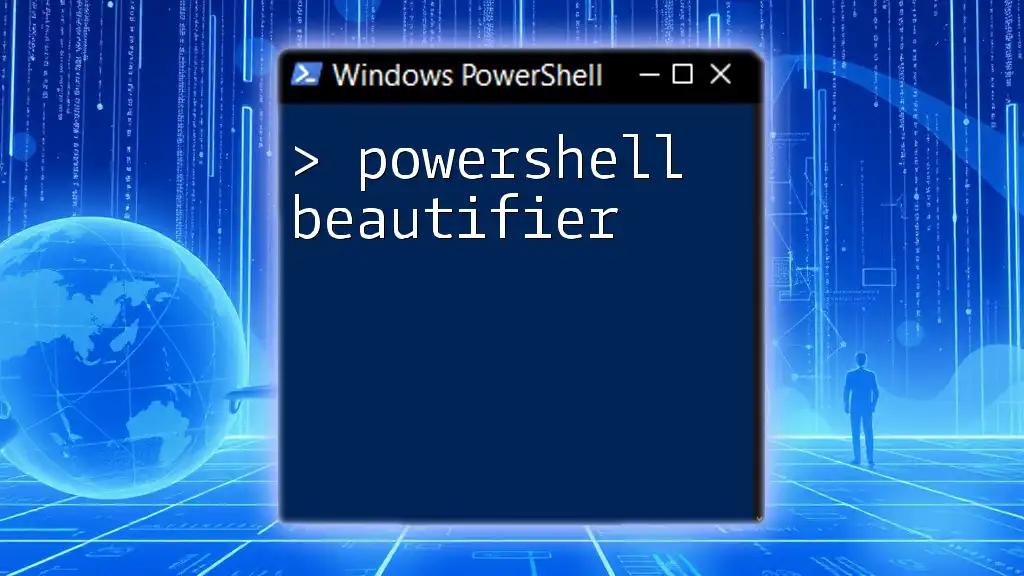
Advanced PowerShell Techniques
Functions
Functions are named blocks of code designed to perform a specific task, promoting code reusability. Here’s how to create a simple function:
function Get-Greeting {
param ($name)
return "Hello, $name!"
}
You can then call your function with an argument:
Get-Greeting "John"
Modules and Snap-ins
Modules are packages of cmdlets, functions, and workflows that extend PowerShell's capabilities. To use a module, you must first import it:
Import-Module ActiveDirectory
This example imports the `ActiveDirectory` module, allowing you to use its cmdlets.
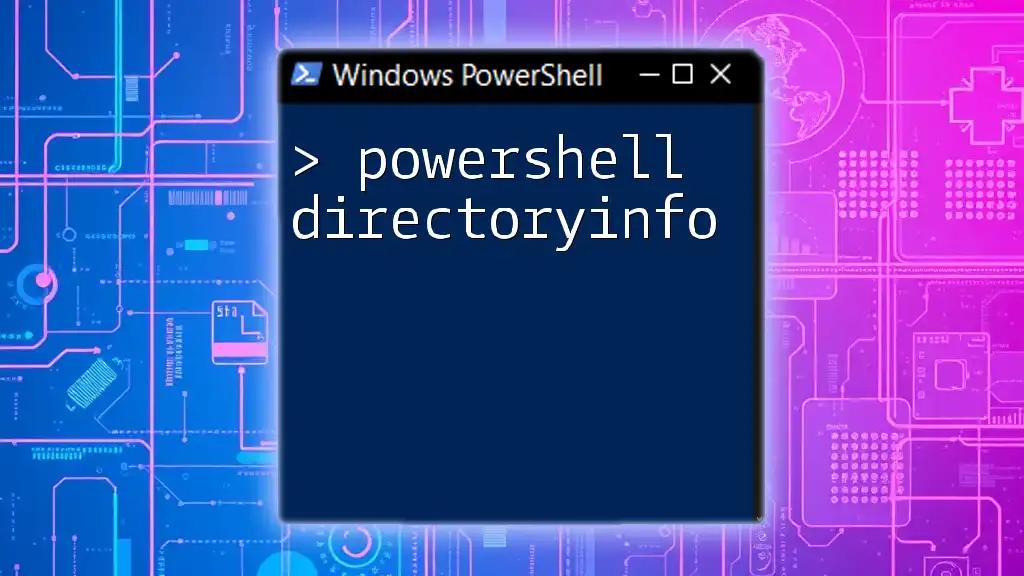
Automating Tasks with PowerShell
Using Scheduled Tasks
PowerShell makes automation seamless through scheduled tasks. You can create a task that runs a script at specified intervals:
$action = New-ScheduledTaskAction -Execute "PowerShell.exe" -Argument "-File C:\path\to\script.ps1"
This command sets up a task that executes your PowerShell script at defined times.
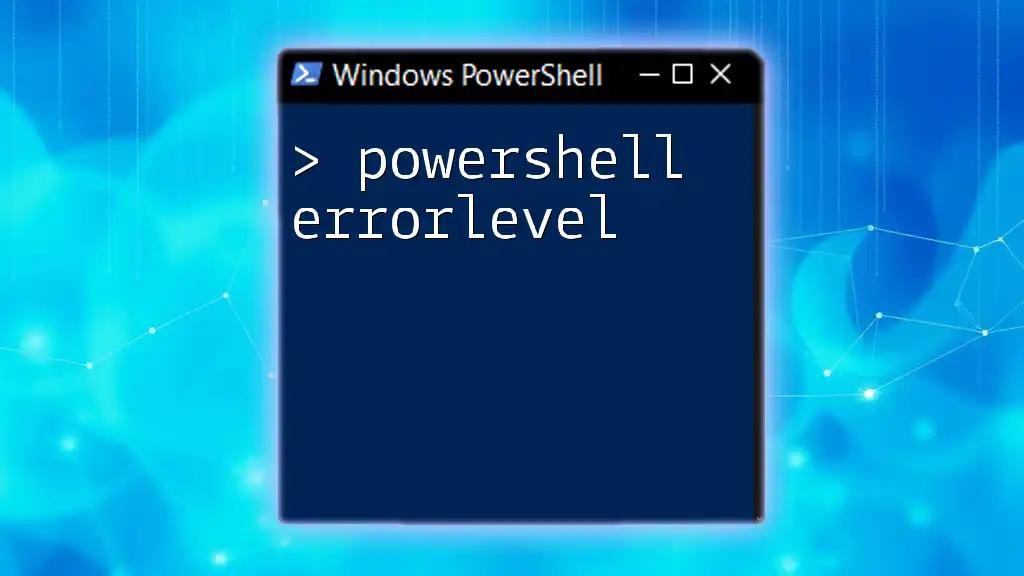
Troubleshooting and Debugging
Common Errors and Solutions
As you write PowerShell scripts, errors are inevitable. Familiarize yourself with common error messages and how to resolve them. For instance, a path not found error typically arises from incorrect file or directory paths.
Using `Try`, `Catch`, and `Finally`
Effective error handling can save you a lot of headaches. PowerShell provides `try`, `catch`, and `finally` blocks for error handling. Here’s how it works:
try {
Get-Content "C:\nonexistentfile.txt"
} catch {
Write-Host "Error: $_"
}
In this example, if the specified file does not exist, the error is caught and a message is displayed.

Resources for Further Learning
After completing the initial stages of your PowerShell learning, you might want to explore deeper. Online communities such as Reddit, PowerShell-focused forums, and social media groups provide platforms to ask questions and share knowledge.
Additionally, several books and online courses can assist in elevating your skills from PowerShell Zero to Hero. Look for highly-rated resources and tutorials to broaden your understanding.
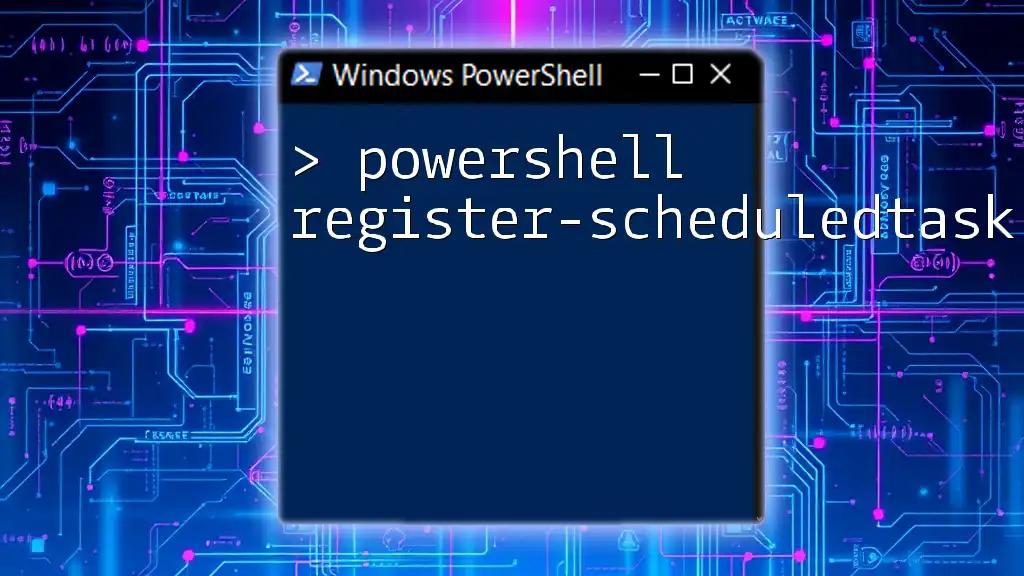
Conclusion
Throughout this guide, we've journeyed from foundational concepts to advanced techniques in PowerShell. Don’t underestimate the importance of hands-on practice; engage with real-world scenarios to solidify your knowledge.
As you continue to explore and experiment with PowerShell, remember that the path to becoming a PowerShell hero is marked by persistence and continual learning. Consider enrolling in specialized training programs to further enhance your skills and achieve your PowerShell Zero to Hero transformation.

Appendices
Cheat Sheet of Common Cmdlets: A quick reference guide to frequently used PowerShell commands can streamline your workflow and increase efficiency.
Glossary of PowerShell Terms: Familiarizing yourself with key terms will enhance your understanding and communication regarding PowerShell.