To execute a batch file using PowerShell, you can simply call the batch file's path, like this:
Start-Process "C:\Path\To\Your\Script.bat"
What is a Batch File?
A batch file is a simple text file that contains a sequence of commands that the operating system can execute in order. Typically, these files have a `.bat` or `.cmd` extension and are used to automate repetitive tasks, such as file management or launching applications. For example, a basic batch file for backing up a directory might look like this:
@echo off
xcopy C:\Source D:\Backup /E /I
In this example, the batch file copies all files from the "Source" directory to the "Backup" directory, including subdirectories.

Why Use PowerShell to Run Batch Files?
Utilizing PowerShell to execute batch files offers several advantages over conventional command prompt usage. PowerShell expands the capabilities of scripting with its advanced features such as:
- Scripting Capabilities: PowerShell provides robust scripting capabilities, allowing you to interact with the Windows operating system in ways that traditional batch files cannot.
- Integration: PowerShell scripts can easily integrate other PowerShell commands and functions, making them more flexible and powerful.
By leveraging PowerShell, users can streamline tasks and automate processes more effectively.
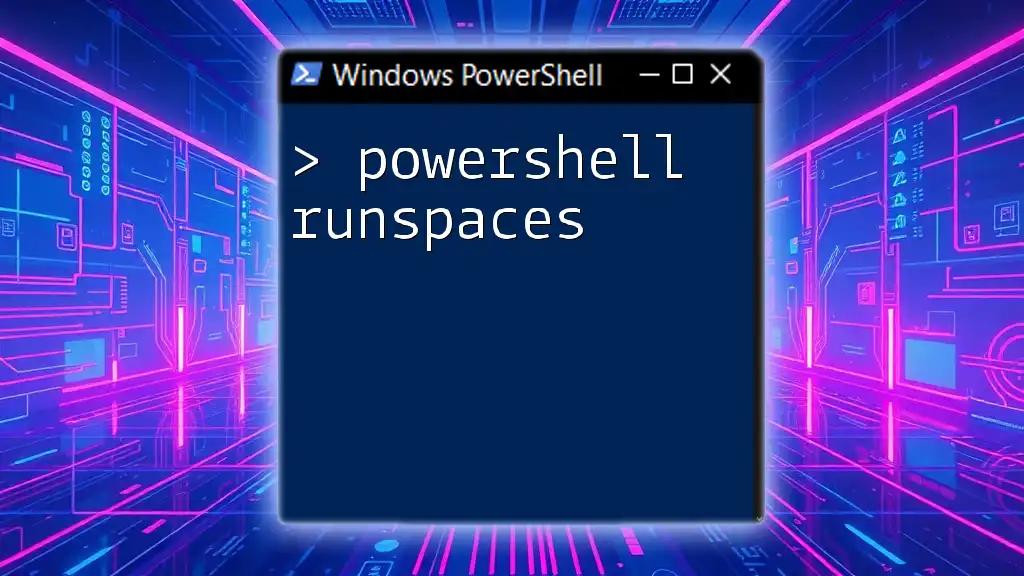
Basic Command to Run Batch Files in PowerShell
How to Run a Batch File in PowerShell
To run a batch file using PowerShell, you can utilize the call operator `&`. This operator executes a command, script, or batch file within the current PowerShell session. The syntax for launching a batch file looks like this:
& "Path\to\your\batchfile.bat"
For instance, to execute a batch file located in `C:\Scripts`, you would enter:
& "C:\Scripts\example.bat"
This simple command effectively triggers the execution of your batch file directly from within PowerShell.
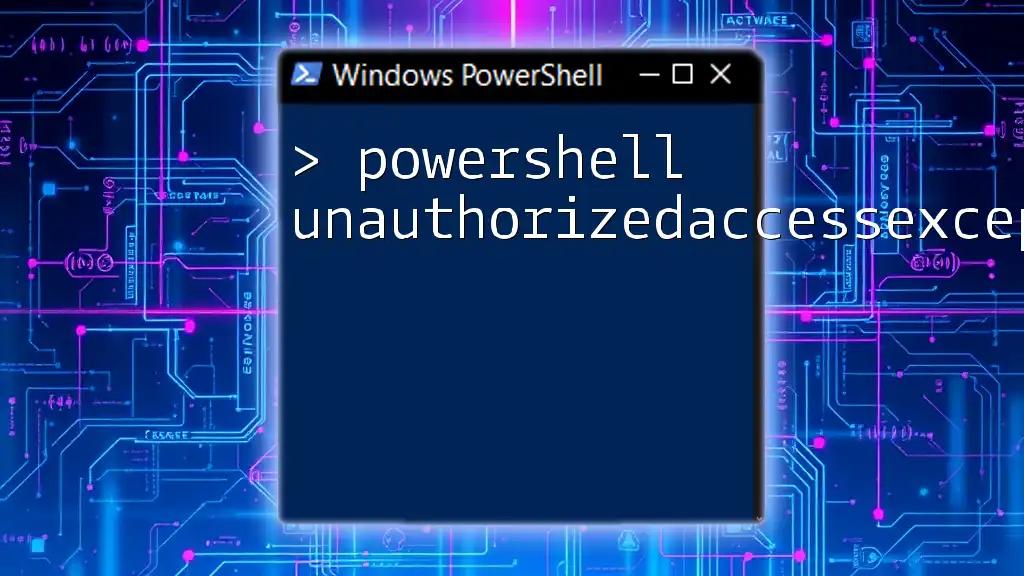
Executing Batch Files with PowerShell
PowerShell Exec Bat File
In addition to the call operator, you can also execute batch files using the `Start-Process` cmdlet. This cmdlet starts a process, such as running an executable or launching a script.
The syntax is as follows:
Start-Process "C:\Scripts\example.bat"
This method is particularly useful if you want to run the batch file asynchronously, allowing for more complex automation workflows.
Using the Call Operator
PowerShell Call Batch File
As mentioned earlier, using the call operator (`&`) is one of the simplest ways to run a batch file. The advantage of this approach is that it executes the batch file in the current scope, allowing access to any variables that may be set within that session. For instance:
& "C:\Scripts\example.bat"
This direct execution ensures that any environment settings or variables needed for the batch operations are respected.
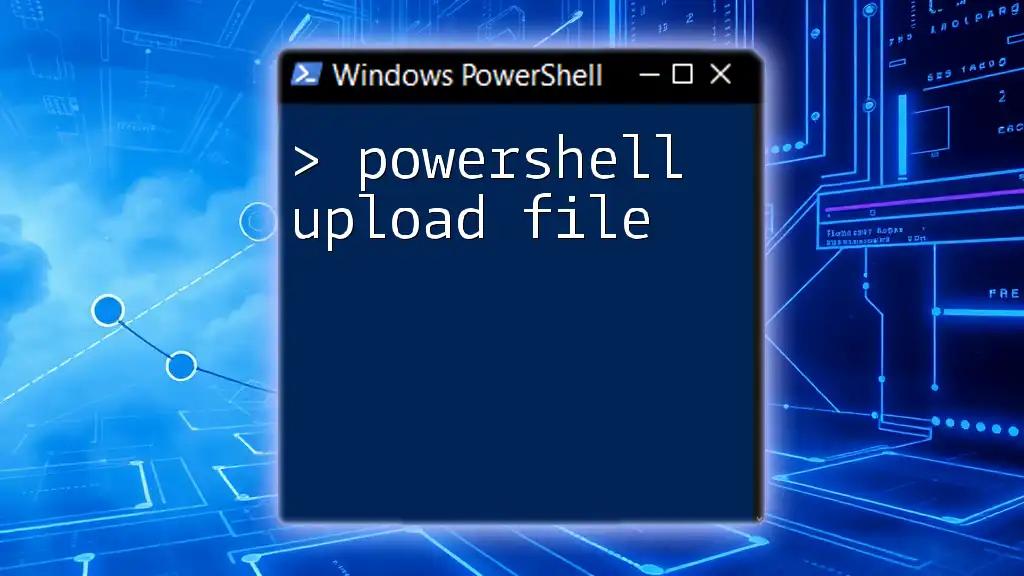
Running Batch Files with Arguments
Passing Arguments to a Batch File
Batch files can also accept parameters. This capability is invaluable when running scripts with variable inputs. To pass arguments through PowerShell, simply append them after the batch file's path. For example:
& "C:\Scripts\example.bat" "argument1" "argument2"
Inside your batch file, you can reference these arguments using `%1`, `%2`, etc. This allows for dynamic behavior dependent on user input.
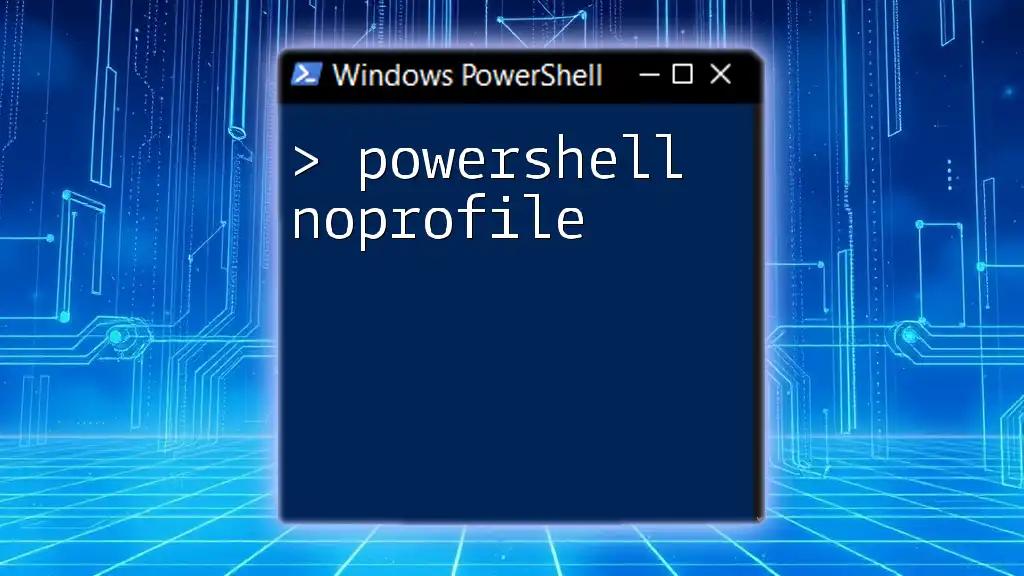
Working with Different Directories
Run Batch File from Different Locations
PowerShell allows for easy navigation between directories, making it straightforward to execute a batch file from various locations. You can change the directory context using `Set-Location` (alias `cd`). Here’s how:
Set-Location "C:\Scripts"
& ".\example.bat"
This method changes the working directory to `C:\Scripts` and then runs `example.bat`.
Using Full Paths
In many cases, using a full path is the simplest and most error-free approach. It ensures that PowerShell knows exactly which file you intend to execute. For instance:
& "C:\Scripts\example.bat"
By providing the complete path, you eliminate any ambiguity regarding the location of your batch file.
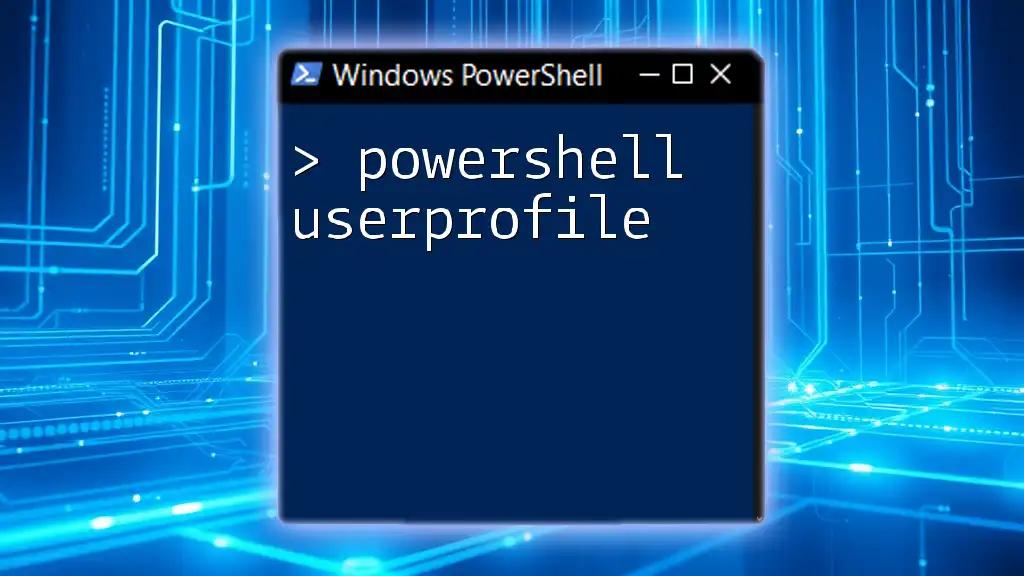
Automating Batch File Execution in PowerShell Scripts
Run Batch File in PowerShell Script
Integrating batch files into PowerShell scripts enhances automation capabilities. To incorporate a batch file execution within a PowerShell script, you could write:
# Sample script
$batFilePath = "C:\Scripts\example.bat"
& $batFilePath
By doing so, you can create more complex PowerShell scripts that combine batch file functionality with PowerShell cmdlets.

Error Handling While Running Batch Files
How PowerShell Handles Errors
Error handling in PowerShell is essential for robust scripts. You can leverage the `try` and `catch` blocks to capture issues when executing a batch file. For example:
try {
& "C:\Scripts\example.bat"
} catch {
Write-Host "An error occurred: $_"
}
This construct allows you to respond appropriately to any errors that occur, improving the overall stability of your automation processes.

Best Practices for Running Batch Files in PowerShell
To optimize the process of running batch files via PowerShell, consider these best practices:
- Ensure Proper Path: Always specify the correct file path to avoid "file not found" errors.
- Check Permissions: Ensure that the necessary permissions are in place, particularly when automating tasks that modify files or system settings.
- Test Scripts: Before fully automating batch files, test them in a controlled environment to catch any errors or unexpected behavior.

Conclusion
In this article, we explored the essential methods and best practices for efficiently using PowerShell to run batch files. Whether you use direct execution, the call operator, or `Start-Process`, PowerShell provides a flexible environment for enhancing your automation capabilities. As you continue your journey with PowerShell, experimenting with batch files will enable you to streamline countless tasks and improve your productivity. To further your knowledge, consider subscribing for more tips and tricks about PowerShell and automation.
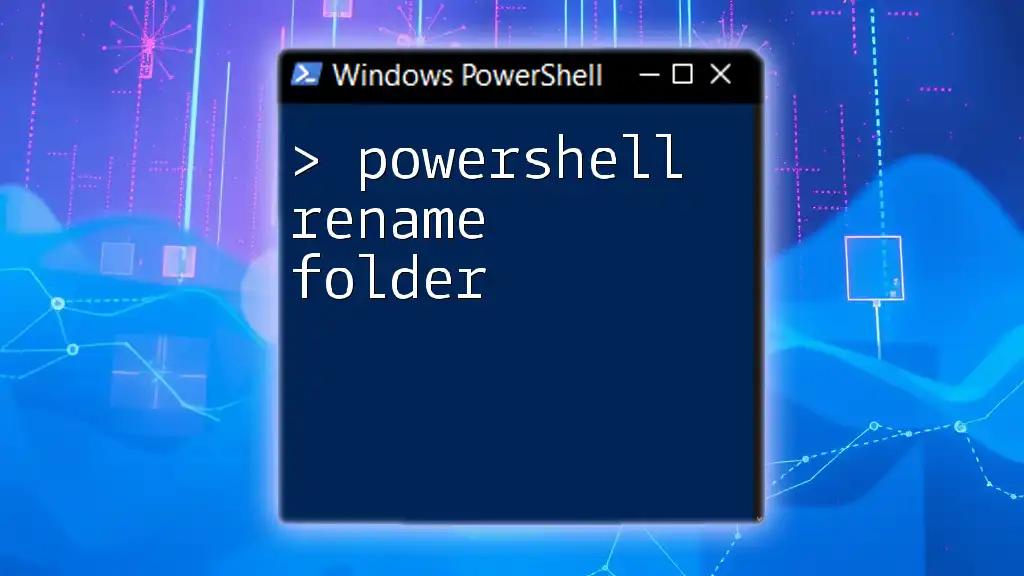
Additional Resources
For further exploration, check out the following resources:
- PowerShell Documentation
- Recommended tools for creating batch files
- Additional reading materials covering PowerShell scripting techniques and best practices.