In PowerShell, special characters allow you to manipulate strings and perform specific functions, such as escaping characters or defining variable types, which can be crucial for effective scripting.
Here's a code snippet demonstrating the use of a special character (the backtick) to escape a double quote within a string:
Write-Host "He said, `"`Hello, World!`"`"
Understanding Special Characters
What are Special Characters?
Special characters are symbols that serve specific purposes in programming and scripting languages like PowerShell. They can alter the behavior of commands, denote syntax, or perform operations that otherwise wouldn't be possible without them. Understanding how to use these characters effectively is crucial for writing clean and efficient PowerShell scripts.
Common Special Characters in PowerShell
Here are some frequently used special characters in PowerShell, along with a brief overview of their functions:
- Backtick (`): The escape character in PowerShell, used to prevent special characters from being interpreted by the shell.
- Dollar Sign ($): Indicates a variable. When prefixed to a word, PowerShell treats it as a variable and evaluates it accordingly.
- Pipe (|): Connects the output of one command to the input of another, enabling powerful command chaining.
- Single Quotes (' '): Used for defining string literals without variable expansion.
- Double Quotes (" "): Allows for string interpolation, meaning variables within the quotes are evaluated and expanded.
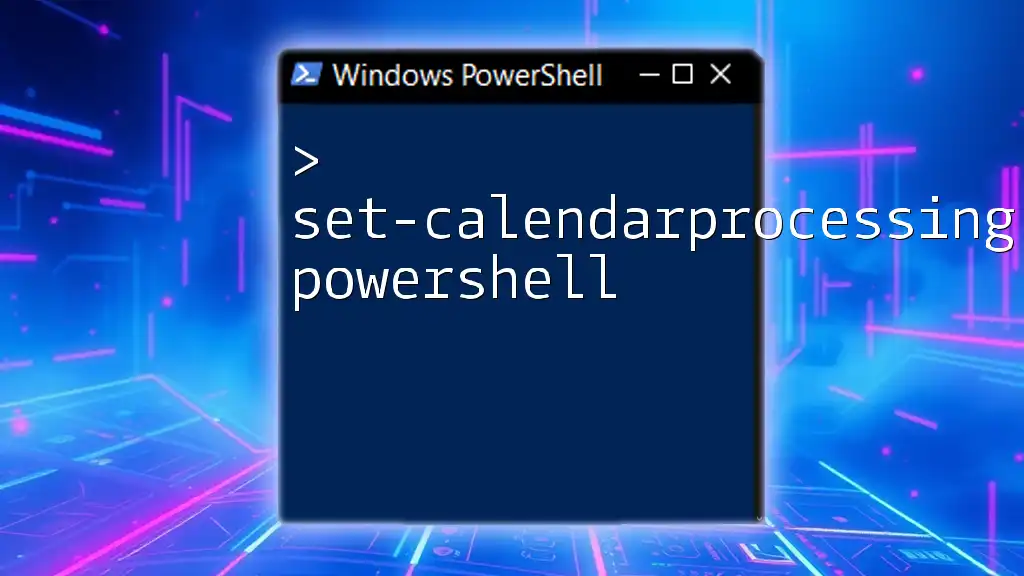
Escape Characters in PowerShell
What is an Escape Character?
An escape character allows you to indicate that the subsequent character should be treated differently from how it would normally be interpreted. In PowerShell, the backtick (`) is the escape character.
How to Use Escape Characters
You can use the backtick to escape characters that have special meaning. For instance, if you want to include a backtick in a string, you'll need to escape it using another backtick:
Write-Host "This is a backtick (`) example"
In this snippet, the string will output: This is a backtick (`) example. The backtick allows the symbol to be included in the output without triggering any special interpretation by PowerShell.
Combining Escape Characters
Using multiple escape characters in a single string is also common. For example, if you want to include both a new line and a tab in your output, you can do so like this:
$string = "This is a test of the `n new line and `t tab"
Write-Host $string
In this code, the output would display "This is a test of the" on one line, followed by "new line and" indented with a tab space.
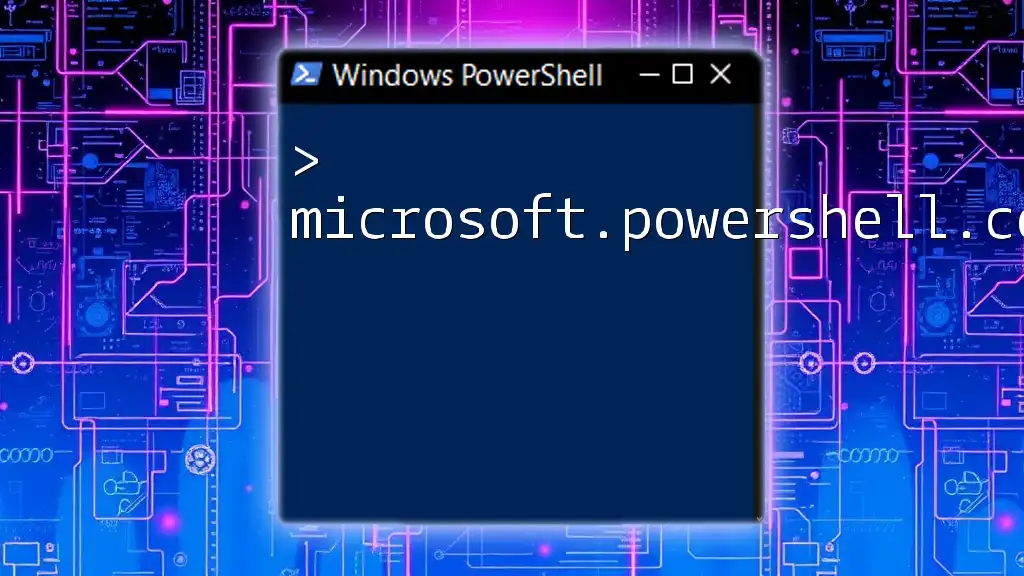
Quoting Strings in PowerShell
Single Quotes vs Double Quotes
Understanding the difference between single and double quotes is fundamental in PowerShell scripting.
Single quotes represent a string literally. This means that any variable or escape sequence within single quotes will not be interpreted. For example:
$singleQuoted = 'Single quotes do not expand $variables'
This will output the string exactly as it appears, without expanding `$variables`.
In contrast, double quotes allow for string expansion. Any variables within double quotes are evaluated:
$number = 10
$doubleQuoted = "Double quotes expand the number: $number"
Write-Host $doubleQuoted
Here, the output will be "Double quotes expand the number: 10".
Heredoc and Now-String
Heredocs and Now-Strings are useful for working with multiline strings. A Heredoc starts and ends with `@"` and `@"` respectively, while a Now-String starts and ends with `@'` and `@'` respectively. Here's how they work:
$hereDoc = @"
This is a heredoc example
It can span multiple lines
"@
Write-Host $hereDoc
This produces a multiline output. In contrast, a Now-String can encapsulate any content without the need for escape characters, as seen in the following:
$nowString = @'
This is a Now-String example including a $variable which won't expand.
'@
Write-Host $nowString
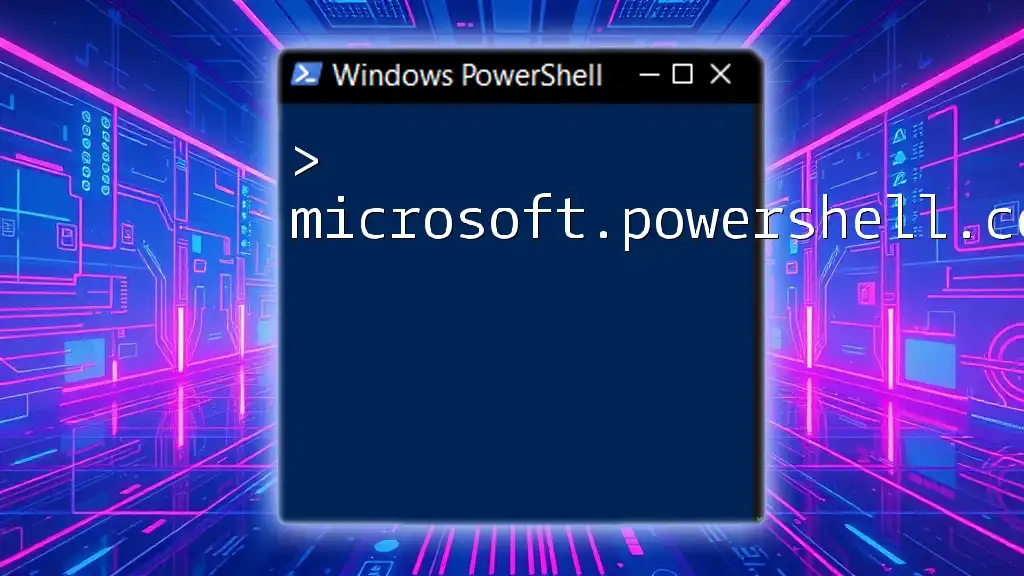
The Importance of the Dollar Sign ($)
Understanding Variables
In PowerShell, any value that you want to store for reuse is defined as a variable, which is always prefixed by a dollar sign ($). When the shell sees `$`, it expects a variable name to follow.
$number = 10
Write-Host "The number is $number"
This will evaluate and display: "The number is 10". Understanding the role of the dollar sign is essential for effective data manipulation.
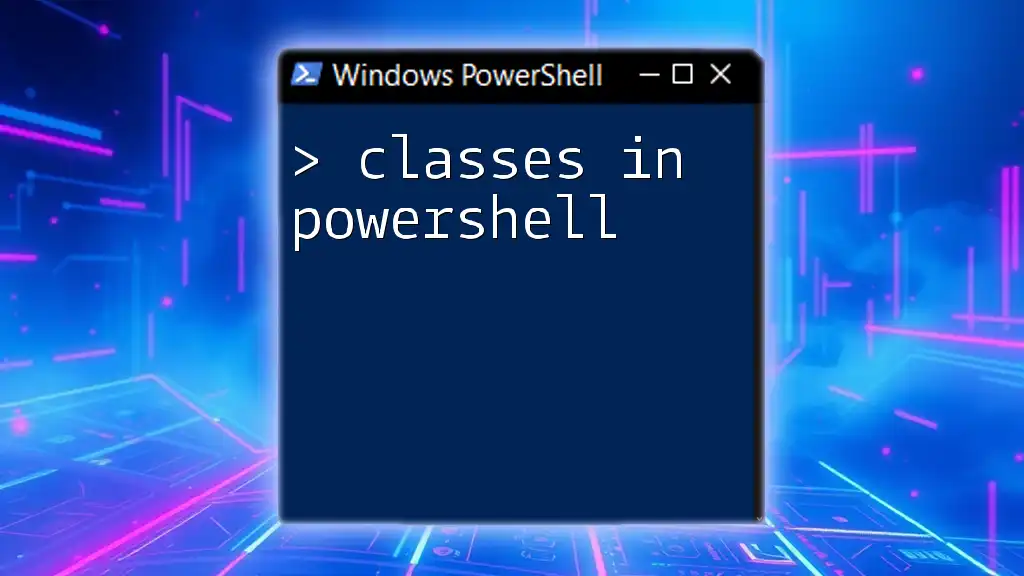
Pipes (|) and Command Chaining
How Pipes Work
Pipes provide a mechanism for chaining commands by passing the output of one command as the input to another. This powerful feature enhances the capability of scripting and enables significantly more concise command expressions.
For example, you can retrieve all running processes and filter them:
Get-Process | Where-Object {$_.CPU -gt 1}
In this case, the `Get-Process` command lists all processes, and `Where-Object` filters those processes based on CPU usage greater than 1.
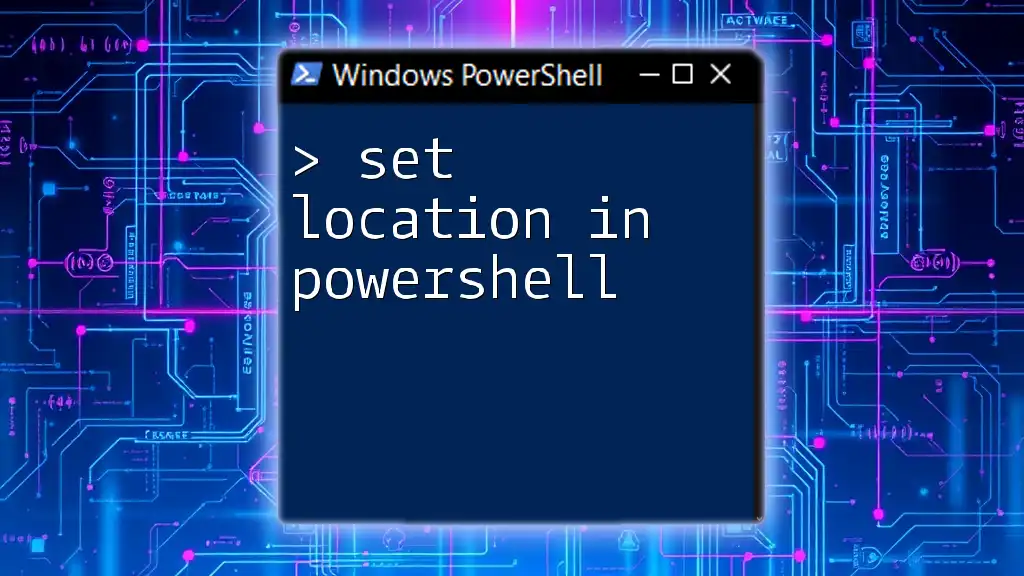
Control Characters
Introduction to Control Characters
Control characters can perform specific functions in a console environment, affecting how commands are inputted or executed.
Common Control Characters and Their Uses
- Ctrl + C: Used to interrupt and stop a running command.
- Ctrl + Z: Sends an end-of-file (EOF) signal in the console.
Understanding these control characters can significantly improve command-line efficiency.
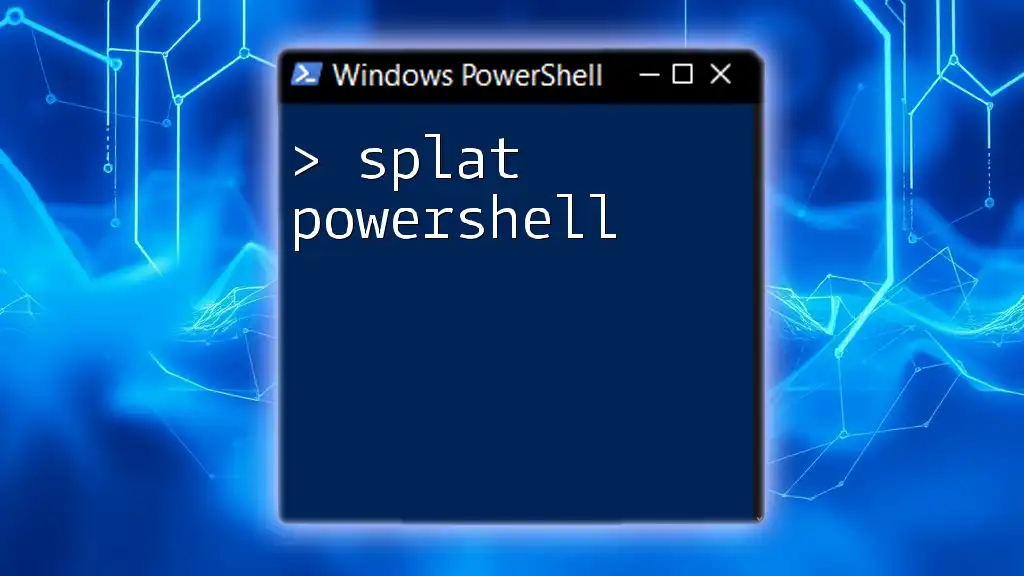
Special Characters in Strings
Comma (,), Semicolon (;), and Other Delimiters
Delimiters serve to separate items within commands and structures. For example, when creating an array, commas are used:
$array = "Value1", "Value2", "Value3"
In this example, the comma separates the values, allowing PowerShell to recognize them as individual elements of an array.
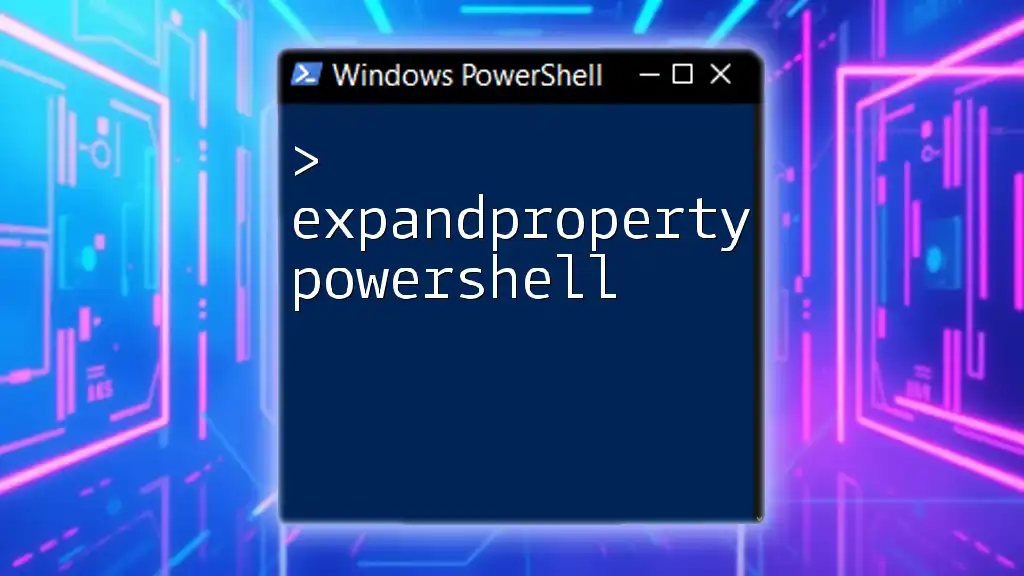
Summary
In this comprehensive guide, we've explored the various special characters in PowerShell, their uses, and their importance in scripting. By mastering these special characters, you will significantly enhance your PowerShell scripting capabilities.
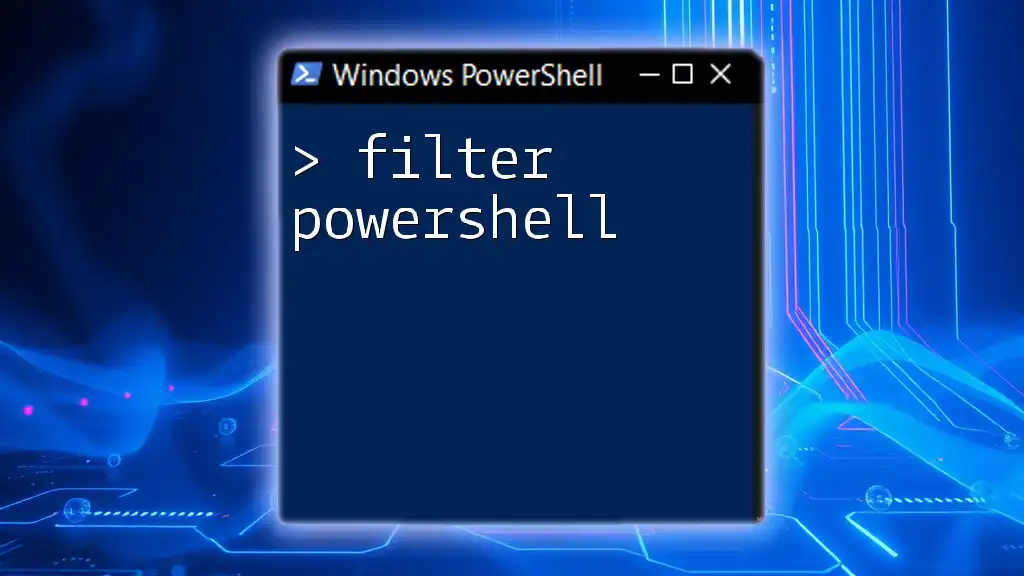
Additional Resources
To further your understanding, consider exploring official PowerShell documentation or engaging with online forums dedicated to PowerShell scripting. Expanding your resources can provide different perspectives and techniques that refine your command line skills.
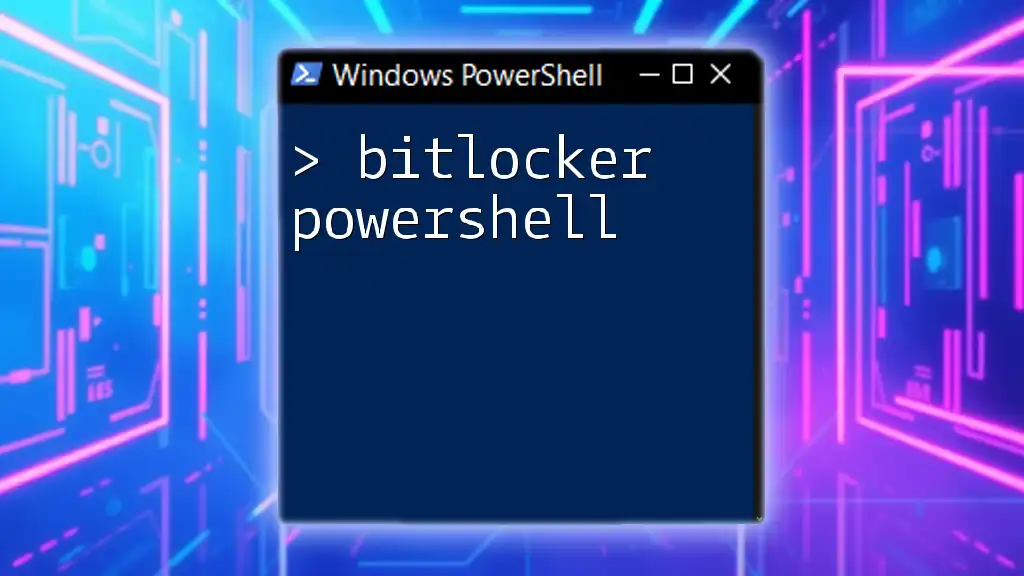
Conclusion
Understanding and mastering special characters in PowerShell is foundational for effective scripting. As you practice utilizing these characters, you'll find that your ability to write clean, efficient scripts improves dramatically. Don't hesitate to experiment with these examples and develop your command-line skills through hands-on practice.
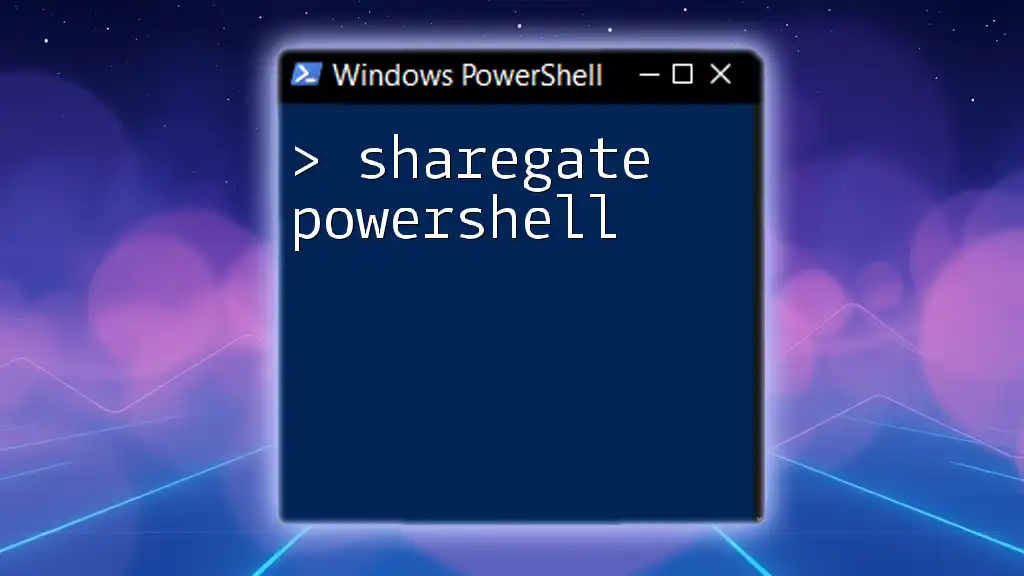
Call to Action
If you found this guide helpful, subscribe to our blog for more insightful articles on PowerShell and other scripting languages, and stay tuned for upcoming posts that will deepen your knowledge!