The `switch` parameter in PowerShell is used to evaluate a set of conditions and execute corresponding code blocks based on matched criteria, allowing for cleaner and more organized branch logic in scripts.
switch ($dayOfWeek) {
'Monday' { Write-Host 'Start of the workweek!' }
'Friday' { Write-Host 'Almost the weekend!' }
default { Write-Host 'Just another day!' }
}
What is the Switch Parameter?
The switch parameter in PowerShell serves as a special type of parameter designed to simplify the coding of boolean options—those that are either true or false. This unique parameter type helps enhance code readability and maintainability, allowing you to write cleaner scripts without needing to explicitly specify values like `true` or `false`.
When you declare a switch parameter, its presence in a command line indicates that it is enabled, while its absence signifies it is disabled. This functionality is particularly useful for toggling features on and off without the overhead of additional conditions.
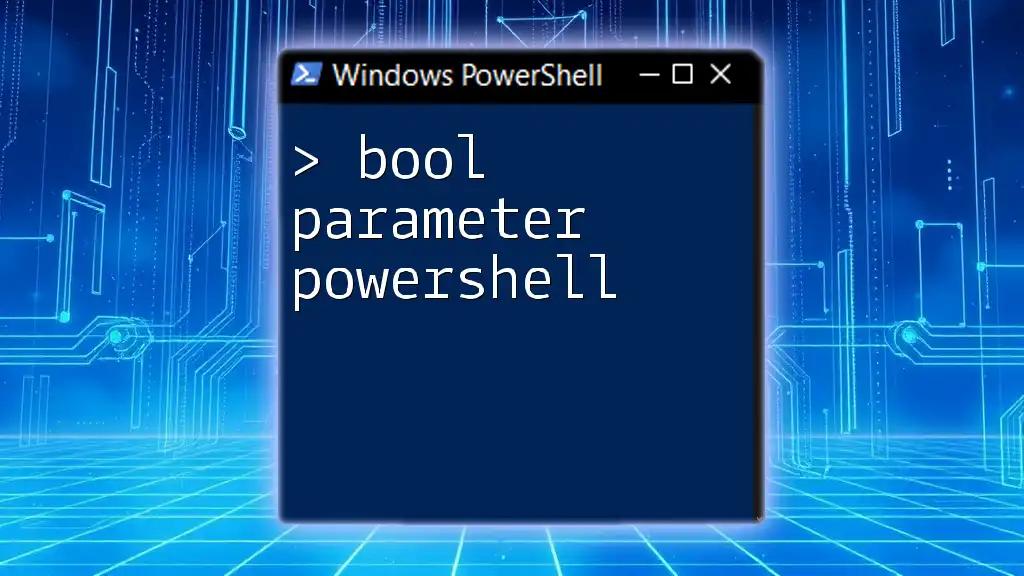
When to Use the Switch Parameter
You should consider using the switch parameter in scenarios where you need a simple binary decision in your script. This is especially useful for:
- Enhancing script readability: A switch parameter makes it instantly clear that an option can either be activated or not without cluttering the command with additional syntax.
- Controlling optional features: For example, enabling verbose logging or debugging information can be made straightforward with a switch.
Real-world Example: Toggle Logging in a Script
Imagine a scenario where you have a script that processes data and you wish to toggle logging. By using a switch parameter, you can implement logging cleanly without adding extra logic checks:
function Process-Data {
param (
[switch]$Logging
)
if ($Logging) {
Write-Host "Logging is enabled."
}
Write-Host "Processing data..."
}
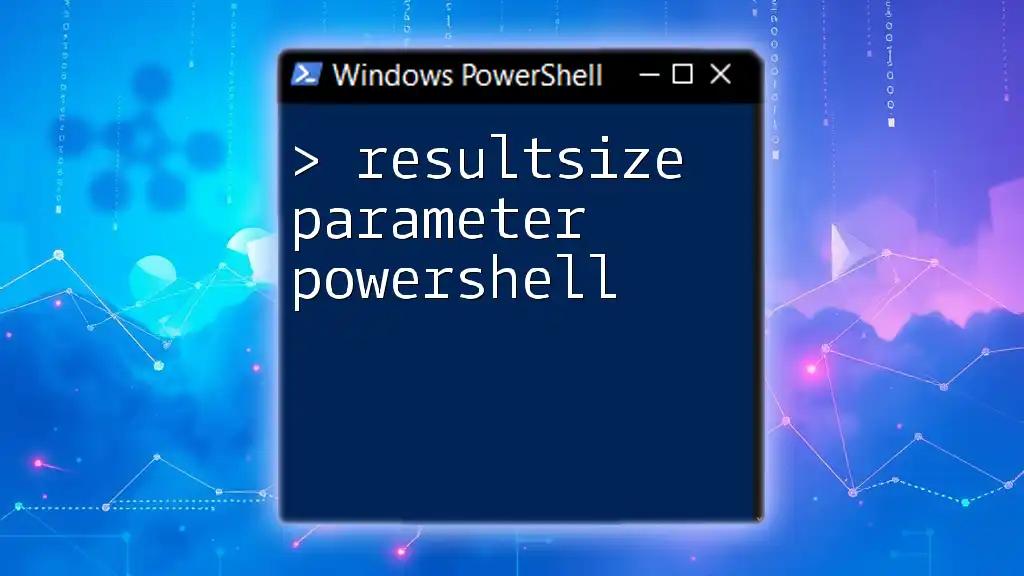
Syntax of the Switch Parameter
The syntax for defining a switch parameter in a function is straightforward. It typically looks like this:
param (
[switch]$MySwitch
)
Breakdown of the Syntax
- Param Block: The `param` keyword allows you to define parameters that the script or function can accept.
- Switch Type: The `[switch]` type indicates that this parameter will only convey a true or false state depending upon its presence or absence.
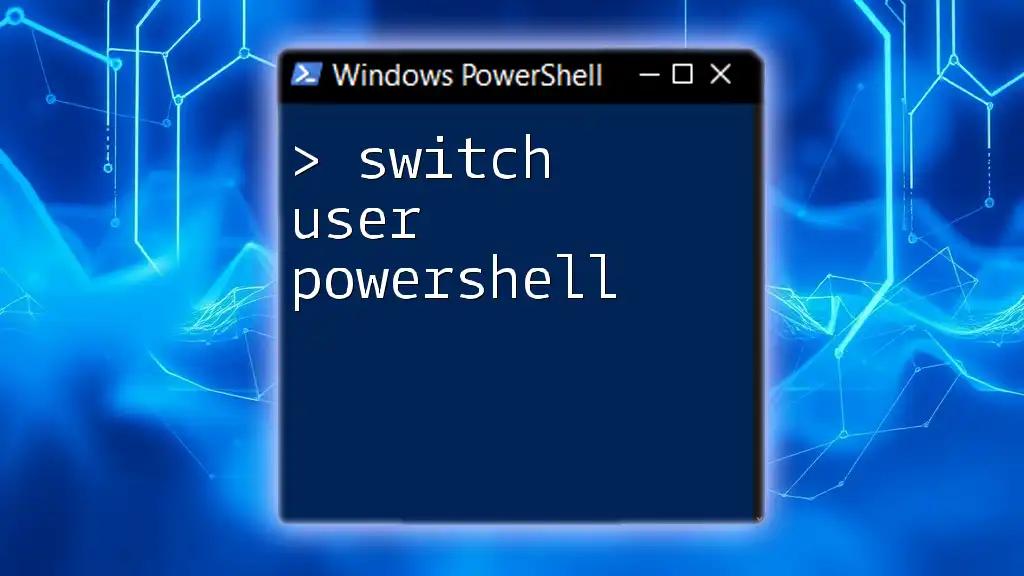
Creating and Using Switch Parameters in Functions
Basic Function Example
Let’s look at a simple function to illustrate how a switch parameter can be utilized:
function Test-Switch {
param (
[switch]$Verbose
)
if ($Verbose) {
Write-Host "Verbose mode is ON"
}
Write-Host "Executing function"
}
Explanation of the Example
In this function, the Verbose switch allows users to get additional output. When the function `Test-Switch` is called with the `-Verbose` switch, the function prints "Verbose mode is ON", providing useful information while executing.
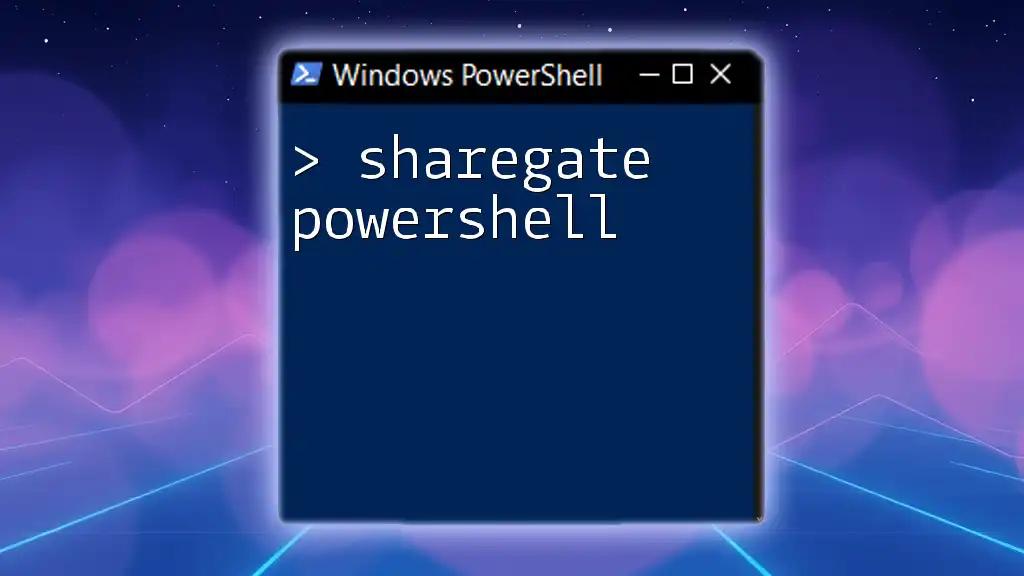
Common Use Cases for Switch Parameters
Logging or Verbose Modes
One of the most common use cases for the switch parameter is enabling or disabling logging in scripts. By declaring a logging switch, users can turn logging on or off, which keeps the output clean when unnecessary.
function Start-Process {
param (
[switch]$Log
)
if ($Log) {
Write-Host "Logging is enabled..."
}
}
Error Handling
Switch parameters can also facilitate error management. By allowing scripts to run in a silent mode or a verbose mode for error reporting based on whether a switch is set, users can easily trace issues when they arise.
Conditional Execution
You can utilize switch parameters to control the execution of various code paths based on conditions. For instance, consider a script that interacts with external services where some operations should only execute when certain configurations are toggled.
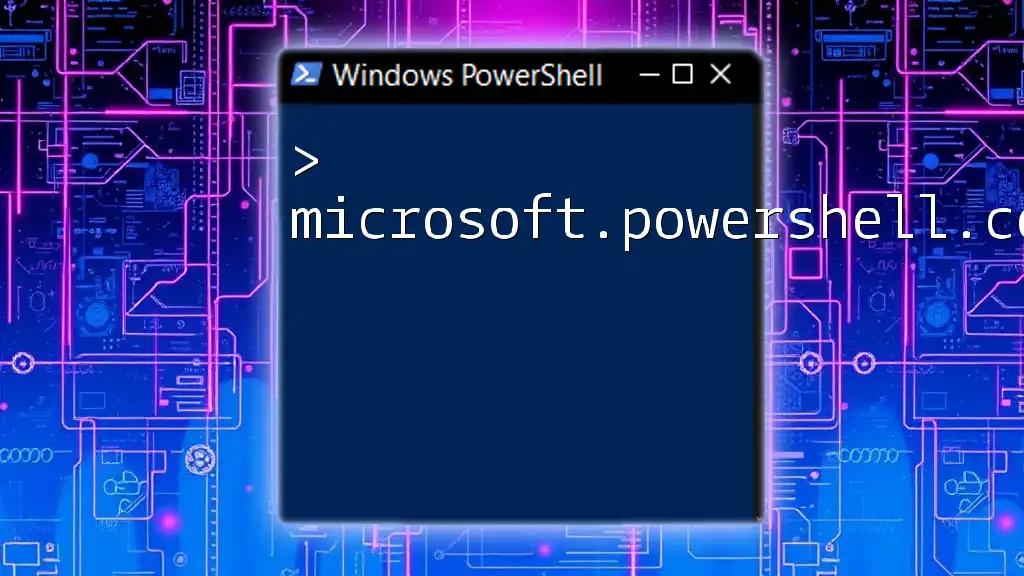
Best Practices for Using Switch Parameters
Naming Conventions
Clear and intuitive naming is crucial. Switch parameter names should convey their purpose. For instance, using `-Verbose` clearly indicates that it pertains to verbose output, while `-Debug` should suggest debugging behavior.
Commenting and Documentation
Proper documentation and comments around functions that utilize switch parameters are essential. Comments help both you and others understand what the switch does and how it affects the function's behavior.
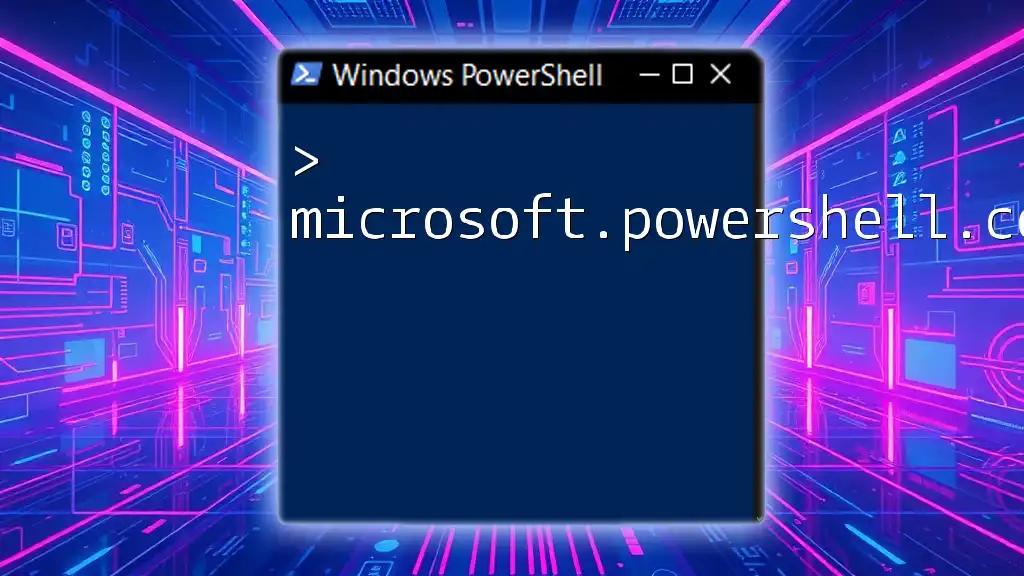
Troubleshooting Common Issues
Misunderstanding Switch Defaults
A common pitfall with switch parameters is underestimating their default behavior. If not set, the switch defaults to `false`. Ensure that logic checks in scripts explicitly account for this to avoid unexpected behavior.
Logic Errors in Scripts Using Switch Parameters
When using switch parameters, ensure the condition checks are accurate. Misunderstandings can lead to logic errors that may cause commands to run or skip unexpectedly. Always validate switch conditions carefully.
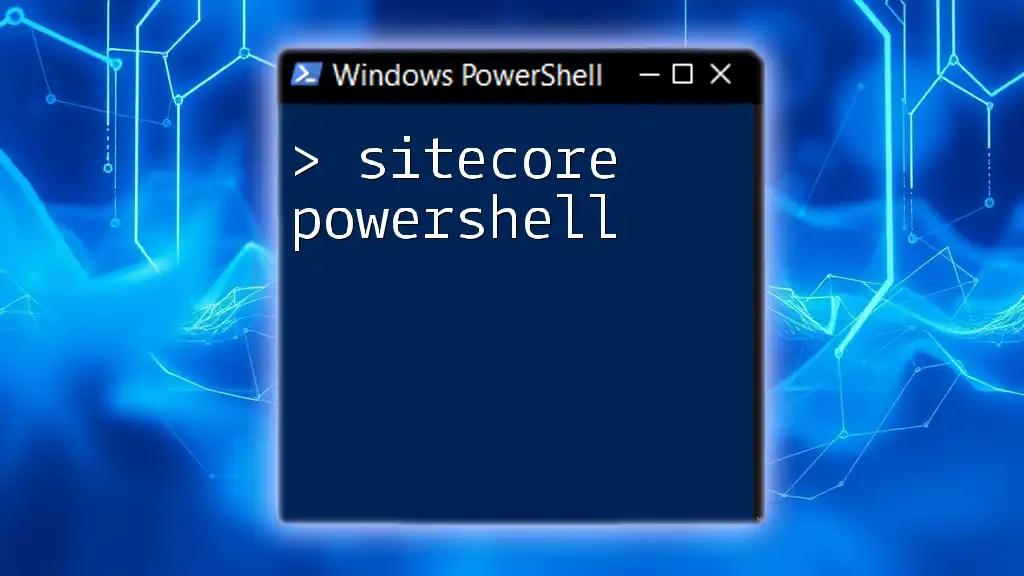
Advanced Strategies with Switch Parameters
Combining Switch Parameters
You can use multiple switch parameters within a single function to enable various features. This allows for a more flexible command interface. Example:
function Advanced-Features {
param (
[switch]$Verbose,
[switch]$Log
)
if ($Verbose) {
Write-Host "Verbose mode is ON"
}
if ($Log) {
Write-Host "Logging is enabled."
}
}
Switch Parameter and Pipeline Integration
The switch parameters can also work beautifully within PowerShell pipelines. This adds even greater dynamism to your scripts, allowing you to pass options alongside objects through the pipeline. Code snippets can demonstrate this functionality effortlessly.
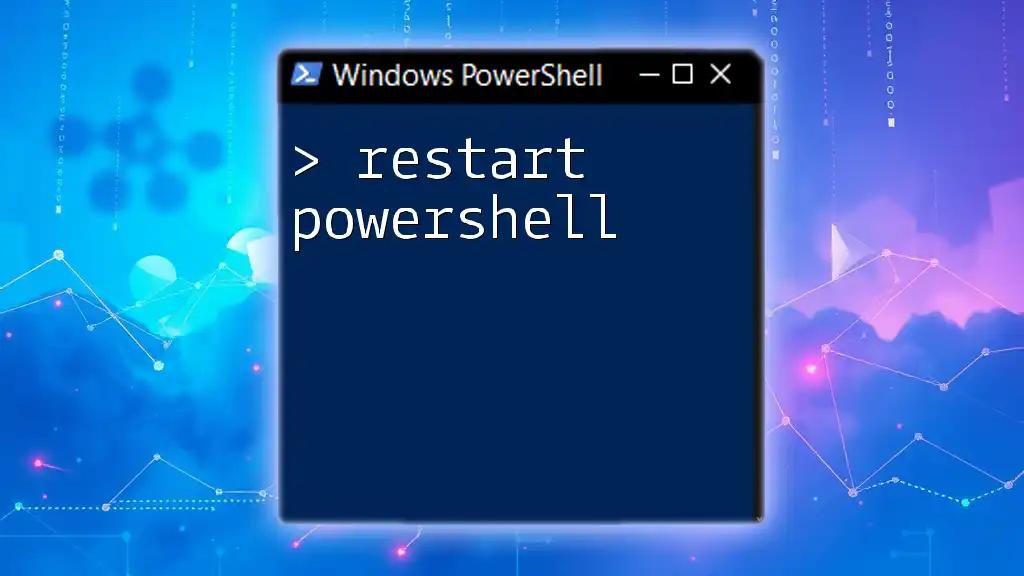
Conclusion
Understanding the switch parameter in PowerShell is essential for writing effective, readable, and maintainable scripts. By utilizing switch parameters, you can simplify decision-making processes in your code, making it easier for others (and yourself) to understand and modify later on.
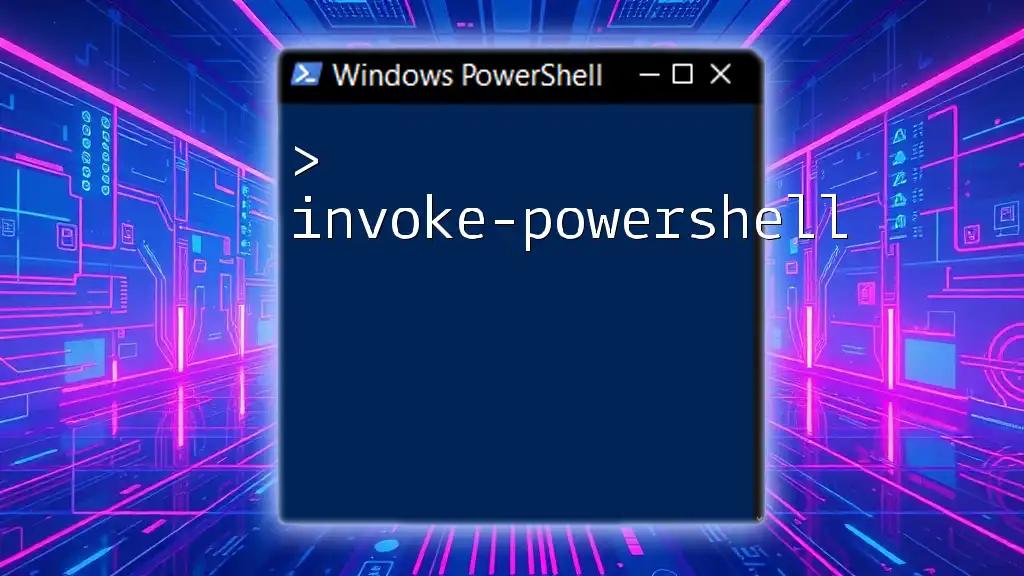
Call to Action
Ready to learn more about PowerShell and take your scripting skills to the next level? Explore more PowerShell tutorials on our website, and don't forget to sign up for our newsletter for the latest updates on upcoming learning materials!