PowerShell WPF (Windows Presentation Foundation) allows developers to create rich desktop applications using PowerShell to leverage graphical user interfaces alongside command-line scripting.
Add-Type -AssemblyName PresentationFramework
[System.Windows.MessageBox]::Show('Hello, World!')
Introduction to PowerShell WPF
What is WPF?
WPF, or Windows Presentation Foundation, is a powerful framework designed for building rich desktop applications on Windows. It leverages a markup language called XAML (Extensible Application Markup Language) to define user interfaces, enabling developers to separate UI design from application logic. PowerShell, often seen as a tool for automation, can also harness the capabilities of WPF to create visually appealing applications with ease.
Why Use PowerShell WPF?
Integrating WPF with PowerShell allows developers to leverage the simplicity and scripting capabilities of PowerShell while crafting sophisticated graphical user interfaces. This results in applications that are not only functional but also user-friendly. Common use cases include administrative tools, monitoring dashboards, and interactive scripts that require user input.
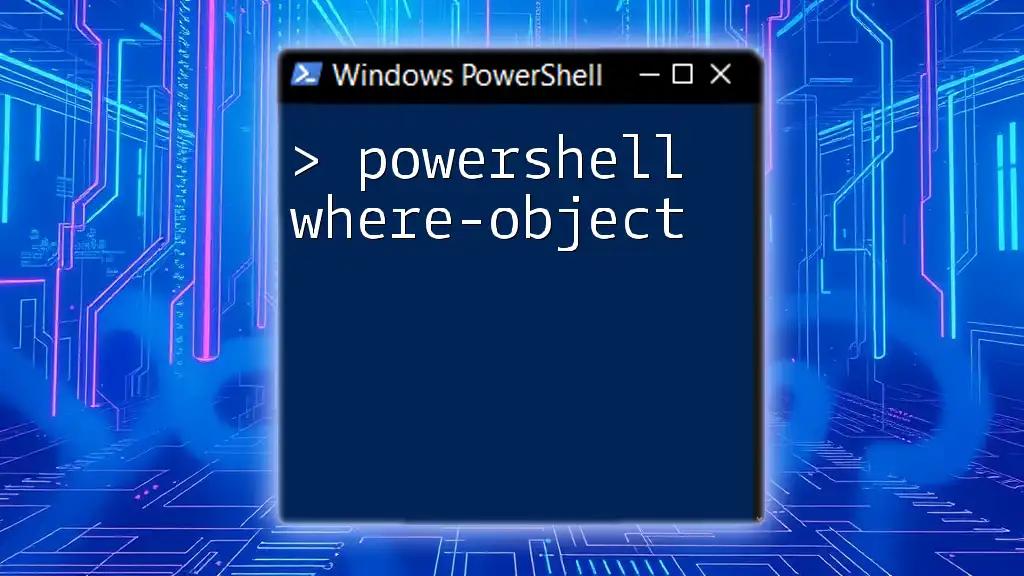
Setting Up Your Development Environment
Prerequisites for Using PowerShell WPF
Before diving into PowerShell WPF development, ensure that you have the latest version of PowerShell installed. Ideally, you should also install Visual Studio or Visual Studio Code, which provides an excellent environment for developing WPF applications.
Installing Necessary Modules
To get started, you may need to install specific modules that facilitate your use of WPF in PowerShell. Utilize the following command to install essential libraries:
Install-Module -Name WPF-Commands
This command fetches necessary components from the PowerShell Gallery so you'll be ready to create your applications.
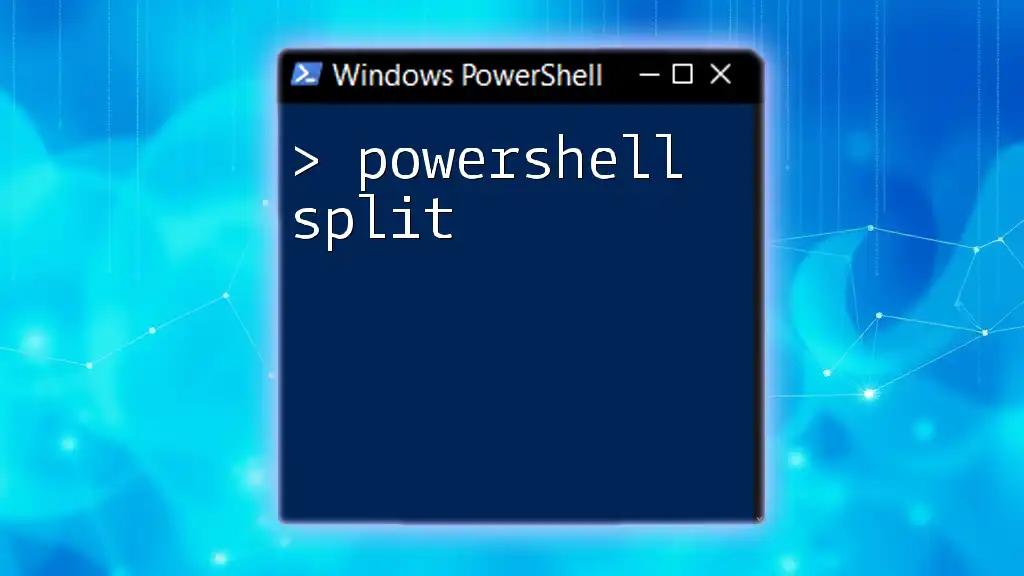
Understanding the Basics of WPF
Core Concepts of WPF
XAML is at the heart of WPF, allowing developers to define UI elements in a clear and hierarchical manner. PowerShell can interact with XAML by loading and manipulating user interfaces directly in scripts.
WPF Architecture Overview
WPF operates on a layered architecture that includes the Presentation Layer, Logical Layer, and Data Layer. The Presentation Layer is responsible for rendering the UI, while the Logical Layer handles the business logic, and the Data Layer manages data binding.
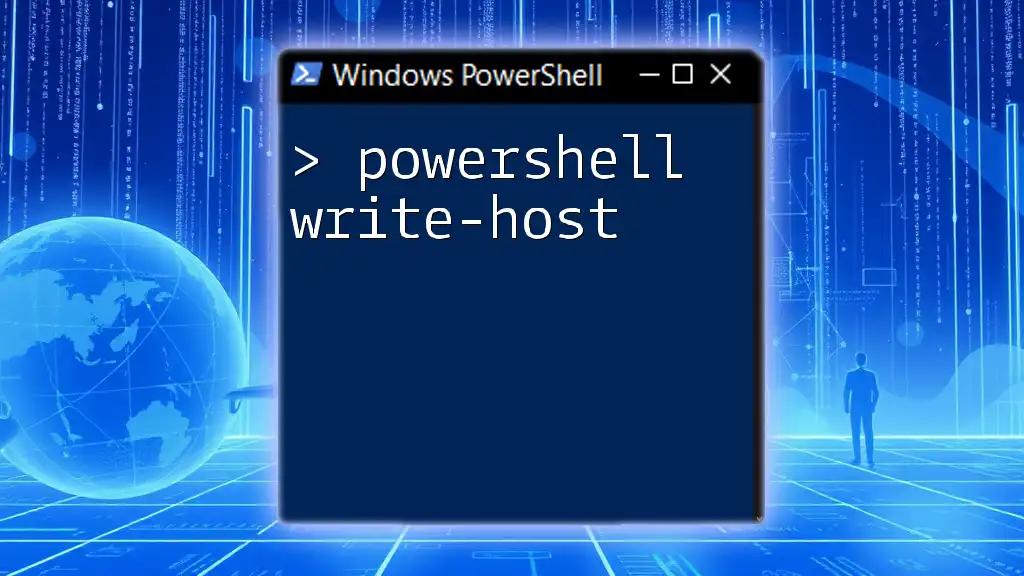
Creating Your First WPF Application with PowerShell
Getting Started with a Basic Window Form
Creating a simple WPF window can be done easily with PowerShell. Here’s how you can create a basic window:
Add-Type -AssemblyName PresentationFramework
$Window = New-Object System.Windows.Window
$Window.Title = "Hello, WPF!"
$Window.Height = 200
$Window.Width = 400
This snippet initializes a new window with a title and specified dimensions.
Adding Controls to Your Window
Controls are vital for any user interface. Let’s delve into adding common controls, for instance, a button and a label:
$Button = New-Object System.Windows.Controls.Button
$Button.Content = "Click Me"
$Button.Add_Click({
$Label.Content = "Button Clicked!"
})
$Label = New-Object System.Windows.Controls.Label
$Label.Content = "Waiting for click..."
$StackPanel = New-Object System.Windows.Controls.StackPanel
$StackPanel.Children.Add($Button)
$StackPanel.Children.Add($Label)
$Window.Content = $StackPanel
In this example, we've created a button that changes the label's content when clicked, showcasing interactive behavior.
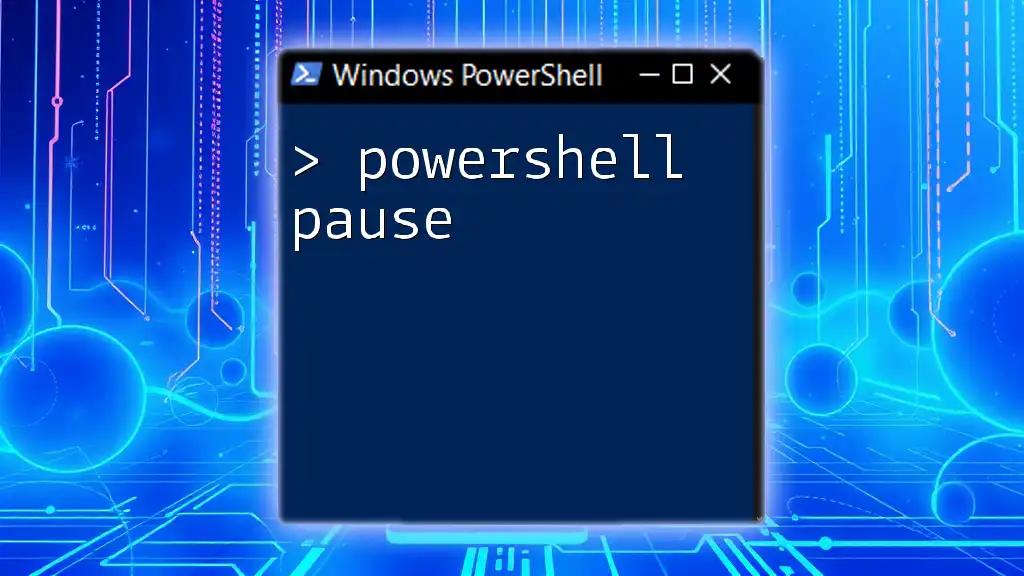
Working with Events in WPF
Understanding Event Handlers
Events in WPF are the mechanism for implementing interactivity. An event handler is a method that executes in response to an event occurring, like a mouse click or a window being loaded.
Common Events to Handle
Among the various events available, the Click event is essential. Here’s how to implement an event handler for our button:
$Button.Add_Click({
[System.Windows.MessageBox]::Show("Hello, WPF!")
})
This code snippet shows a message box when the button is clicked, demonstrating the interactive capabilities of WPF.
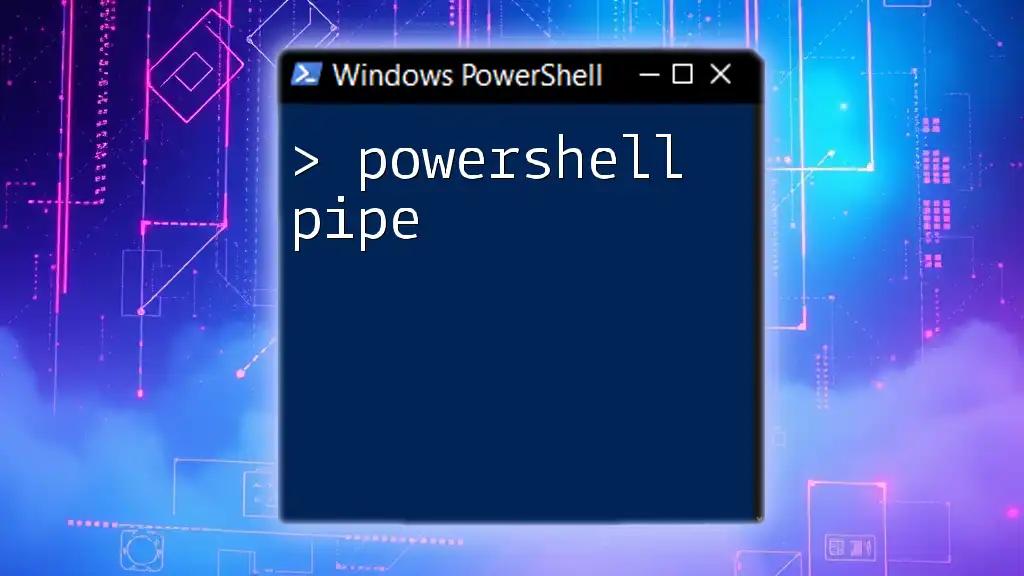
Data Binding in WPF
What is Data Binding?
Data binding allows you to associate UI elements with data sources, enabling real-time updates and dynamic content in your applications. This is particularly useful in scenarios where user input must be displayed or stored.
Implementing Data Binding in PowerShell
You can bind controls like `TextBox` to variables. Here’s how:
$TextBox = New-Object System.Windows.Controls.TextBox
$TextBox.Text = "Initial Value"
Using Observable Collections for Dynamic Data
Observable Collections provide a way to dynamically update data in the UI. Here’s an example where we bind a list to a WPF control:
$Collection = [System.Collections.ObjectModel.ObservableCollection[string]]::new()
$Collection.Add("Item 1")
$Collection.Add("Item 2")
When items are added or removed from the collection, the UI reflects those changes automatically.

Advanced WPF Features
Styles and Templates
WPF allows for the customization of UI elements using styles and templates. Styles can change visual appearance without altering functionality. For instance, you could apply a uniform color scheme to all buttons in an application.
Resource Dictionaries
Resource dictionaries enable centralized resource management for your styles and templates. This means you can define a set of styles in one place and apply them throughout your application, promoting consistency.
Using Custom Controls
Creating custom controls aids in reusing UI components across different applications. User controls can encapsulate complex logic and UI into manageable pieces. For instance, you could wrap a commonly used form into a user control to streamline development.

Debugging and Troubleshooting WPF Applications
Common Issues in WPF PowerShell Applications
As with any development process, creating WPF applications may lead to issues like unresponsive UI, binding errors, or unexpected behavior. Identifying the problem early can save time.
Using Debugging Tools
PowerShell provides built-in tools to assist in debugging. Using try-catch statements allows for graceful error handling:
try {
# Code that may fail
} catch {
Write-Host "An error occurred: $_"
}
Utilizing such error handling ensures your application can manage exceptions effectively, informing the user instead of crashing.
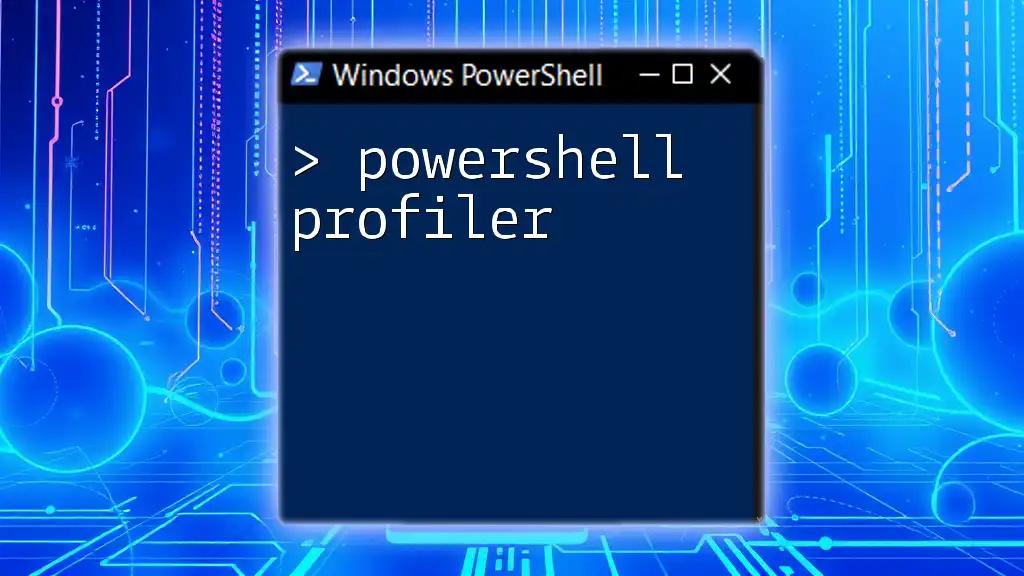
Deploying WPF Applications
Packaging Your Application for Distribution
When your WPF application is complete, packaging it for distribution can be accomplished through various techniques such as creating a standalone executable. This enables users to run your application without needing to interact with PowerShell directly.
Best Practices for Deployment
Ensure that you document your application’s dependencies and system requirements before distribution. This will make it easier for users to install and run your application.
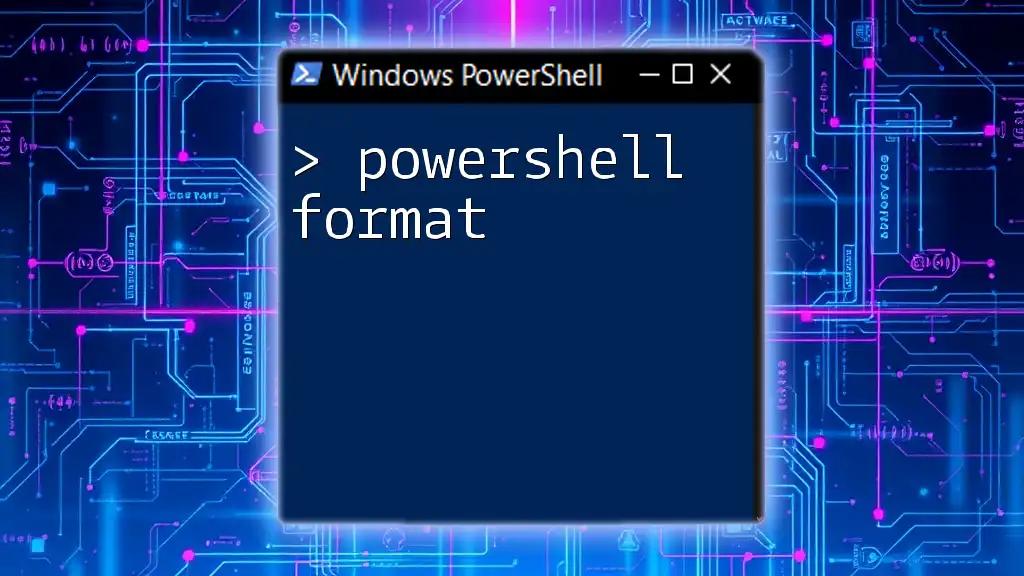
Conclusion
Incorporating PowerShell WPF into your development toolkit empowers you to create sophisticated, user-friendly applications with relative ease. With a rich framework at your disposal and the ability to integrate powerful scripts, the possibilities are virtually limitless. Explore these concepts further, delve into additional resources, and cultivate your skills to build impactful applications using PowerShell WPF.