A PowerShell trading bot automates the buying and selling of assets on trading platforms using scripts, allowing for efficient and precise trading strategies.
# Simple PowerShell Trading Bot Example
$apiKey = "your_api_key"
$apiSecret = "your_api_secret"
$tradeAmount = 1
$market = "BTCUSD"
# Function to place a market order
function Place-MarketOrder {
param (
[string]$side # 'buy' or 'sell'
)
$url = "https://api.exchange.com/v1/order"
$body = @{
symbol = $market
side = $side
type = "market"
quantity = $tradeAmount
} | ConvertTo-Json
# Use API to place order
Invoke-RestMethod -Uri $url -Method Post -Headers @{
'API-Key' = $apiKey
'API-Secret' = $apiSecret
} -Body $body -ContentType "application/json"
}
# Example usage
Place-MarketOrder -side "buy"
Understanding Trading Bots
What is a Trading Bot?
A trading bot is a computer program that automatically executes trades on behalf of a trader based on predetermined criteria. These bots analyze market conditions and make decisions faster than human traders. There are several types of trading bots, including market-making bots and arbitrage bots, each with its own unique strategy and purpose.
Benefits of Using Trading Bots
The use of trading bots comes with multiple benefits:
- 24/7 Operation: Unlike human traders, bots can operate continuously, allowing them to seize trading opportunities at any time, day or night.
- Increased Trading Efficiency: Bots can analyze market trends and execute trades at a speed that humans cannot, ensuring maximum profit potential.
- Risk Management and Consistency: Automated trading helps in maintaining discipline and adhering to trading strategies without emotional interference.
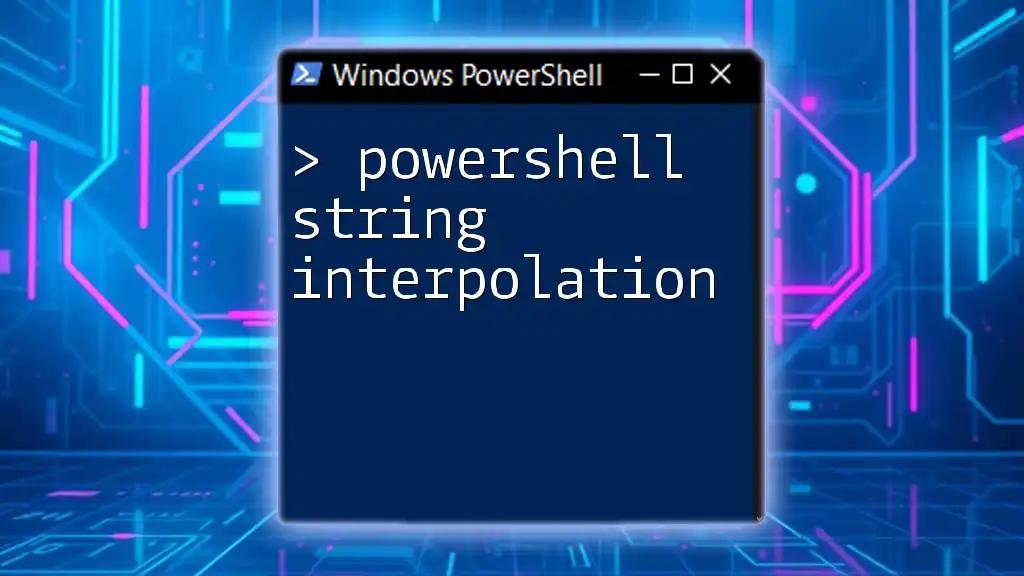
Why Use PowerShell for Trading Bots?
PowerShell Basics
PowerShell is a powerful scripting language that offers advanced capabilities for automation and system management. It is particularly useful for tasks that require data manipulation and integration with various systems. Its scripting capabilities make it an excellent choice for automating trading strategies.
Advantages of PowerShell for Trading
Using PowerShell for creating a trading bot has several advantages:
- Easy Integration with APIs: PowerShell can easily interact with RESTful APIs, making it ideal for fetching market data or executing trades through services like Binance or Kraken.
- Access to Windows-Based Systems: PowerShell is natively available on Windows systems, allowing seamless execution of scripts without the need for additional installations.
- Enhanced Data Handling and Reporting: PowerShell provides robust data handling capabilities, enabling traders to manage and analyze their data effectively.

Setting Up Your Trading Environment
Required Tools and Software
Before you start coding your PowerShell trading bot, ensure that you have:
- PowerShell installed: Newer versions come pre-installed with Windows. You can check by typing `powershell` in your command prompt.
- Required libraries or modules like `Invoke-WebRequest` for making API calls and `JSON` for handling data formats. Both are built into PowerShell.
Choosing a Trading Platform
Select a trading platform with a solid API. Popular choices include Binance and Kraken, both of which provide developer-friendly APIs. Make sure to set up your API keys for secure access to your trading account.

Designing a Basic Trading Bot
Defining Your Strategy
Before you start coding, define your trading strategy. Consider what type of market conditions you will target and what specific criteria will trigger trades. Common strategies include trend following and arbitrage, which can be tailored to suit your risk tolerance and investment goals.
Writing Your First PowerShell Script
Basic Structure of a Trading Bot Script
Start by creating the basic structure of your bot. This forms the foundation for the logic you'll implement.
# Sample Script Structure
$apiKey = "your_api_key"
$apiSecret = "your_api_secret"
$baseUrl = "https://api.yourtradingplatform.com"
In this snippet, replace `your_api_key` and `your_api_secret` with your actual API credentials. The `$baseUrl` will be used for API calls.
Connecting to an API
To interact with your chosen trading platform, you'll need to make API calls. Using PowerShell’s `Invoke-RestMethod`, you can seamlessly fetch data.
$response = Invoke-RestMethod -Uri "$baseUrl/endpoint" -Method Get -Headers @{ "API-Key" = $apiKey }
This example demonstrates how to retrieve data from the trading platform’s API endpoint while including your API key for authentication.
Analyzing Market Data
Fetching price data is essential for making informed trading decisions. To retrieve market prices, use the following snippet:
$priceData = Invoke-RestMethod -Uri "$baseUrl/ticker" -Method Get
This command pulls the latest ticker data, which can be used to make buy or sell decisions based on your trading strategy.

Implementing Trade Logic
Executing Trade Orders
Your trading bot will need to execute trades based on the defined strategy. Here is how to create functions for buying and selling:
function Buy {
param (
[string]$symbol,
[decimal]$amount
)
# Buy Order Logic
}
function Sell {
param (
[string]$symbol,
[decimal]$amount
)
# Sell Order Logic
}
These functions will serve as the core methods to execute buy and sell orders. You will later need to implement the logic inside these functions based on your strategy.
Managing Trades
Risk management is crucial for preserving capital. Implement stop-loss and take-profit conditions within your trading logic. Keep track of open positions, adjusting them based on real-time market data to mitigate risk effectively.
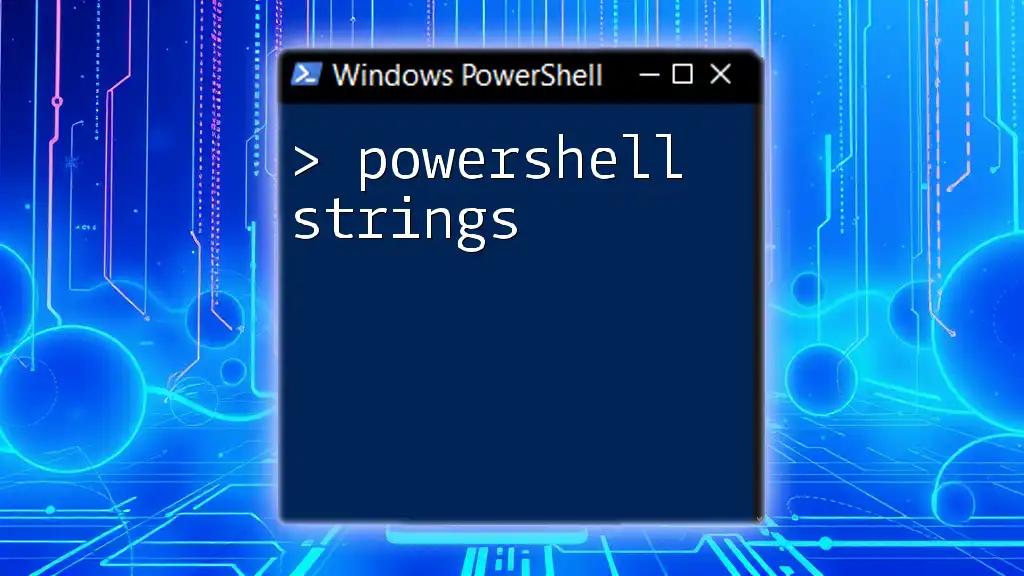
Testing Your Trading Bot
Backtesting Strategies
Before deploying your trading bot, backtest the trading strategy against historical data. This will give you insights into how your bot might perform in the current market conditions. PowerShell can help you analyze past performance using historical price data.
Paper Trading
Engaging in paper trading allows you to simulate real trades without financial risk. Implement a simulation mode within your PowerShell script, where you can still run your logic but without making actual trades.
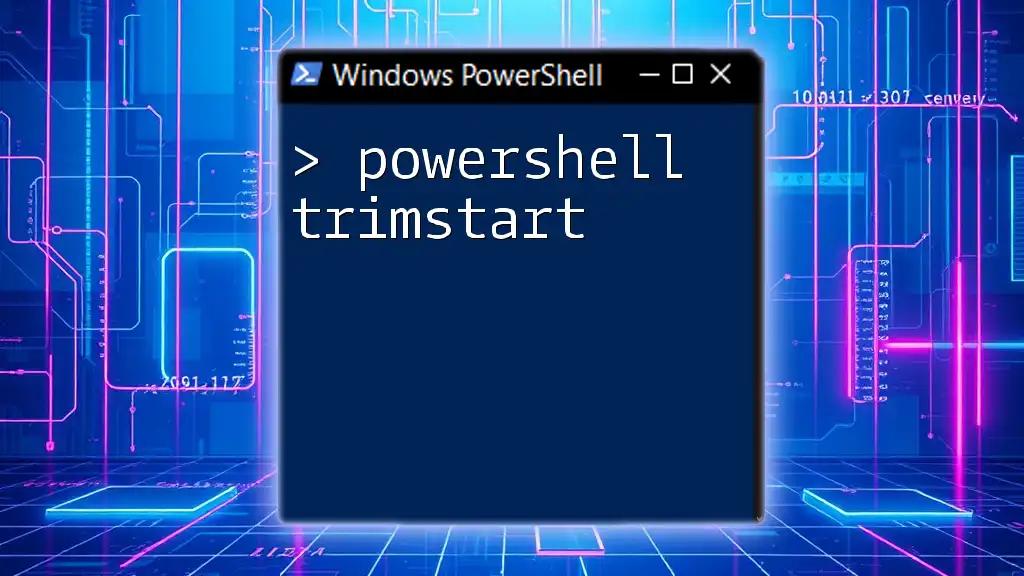
Deploying Your Trading Bot
Running the Bot
When you’re ready to deploy, run the bot on your local machine. To automate its execution, consider using the Windows Task Scheduler to have PowerShell execute your script at regular intervals.
Monitoring Performance
Logging trades and performance metrics is essential for evaluating your bot's effectiveness. Use the following code snippet to log trades:
# Sample Logging Code
Add-Content -Path "trades.log" -Value "Trade data here"
This will create a log file where you can record important trade information for later analysis.
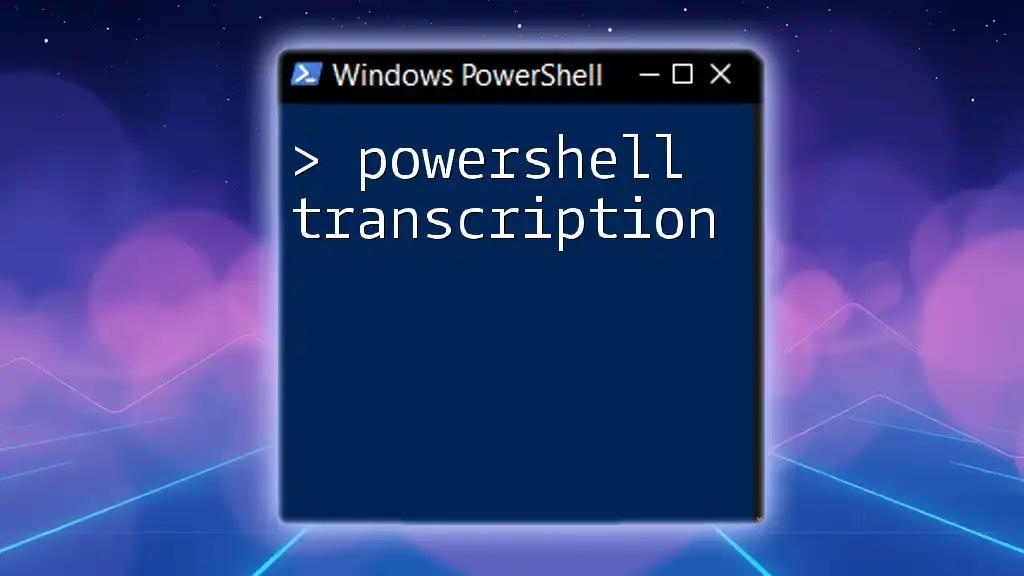
Troubleshooting Common Issues
Debugging Your PowerShell Scripts
Debugging is crucial to ensuring that your bot runs as intended. Use `Write-Host` or `Write-Output` statements throughout your script to monitor variables and flow. Common issues include API connection errors and incorrect trade logic, so pay special attention to these areas.
Optimizing Performance
To enhance your trading bot's performance, focus on optimizing your code. Ensure that API calls are as efficient as possible and that data handling is streamlined. Reduce unnecessary computations and utilize PowerShell's native functions for better resource management.
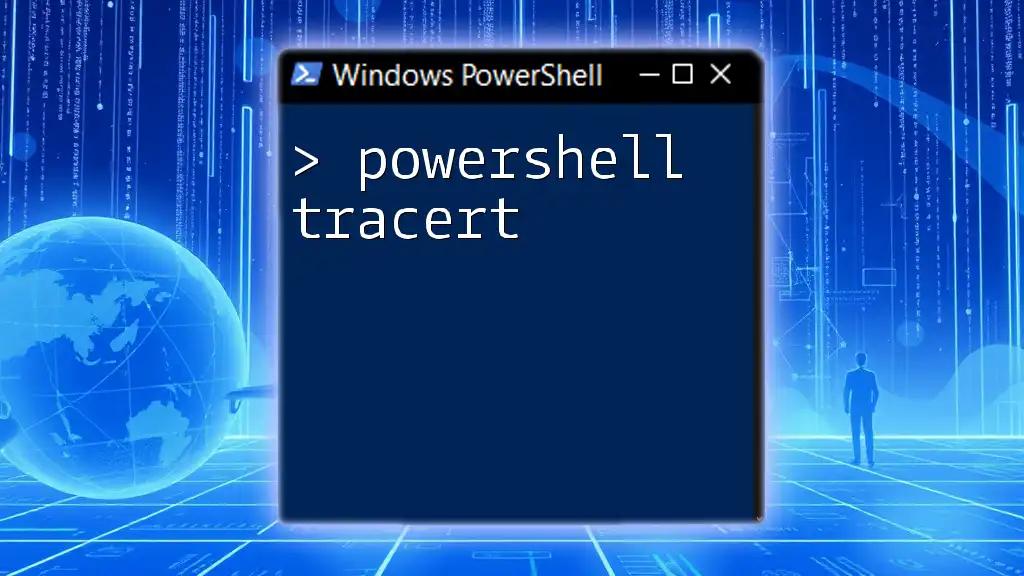
Future Enhancements
Adding Advanced Features
Once you have a functional bot, consider incorporating advanced features like machine learning for predictive analytics or portfolio optimization techniques to enhance performance further.
Keeping Up with Market Changes
The financial market is ever-evolving; staying informed is essential. Regularly review your bot's performance and be ready to adapt its trading strategies to new market conditions or changes imposed by trading platforms.

Conclusion
Creating a PowerShell trading bot is an exciting journey into the world of automated trading. By understanding the fundamentals of trading bots and combining them with the powerful capabilities of PowerShell, you can develop an effective trading strategy that operates independently of your direct input. Continuous learning, testing, and adaptation are key to maximizing the potential of your PowerShell trading bot.