A PowerShell ping sweep is a method used to check the status of multiple IP addresses in a given range to determine which devices are online.
Here's a simple code snippet to perform a ping sweep:
$startIP = [System.Net.IPAddress]::Parse("192.168.1.1")
$endIP = [System.Net.IPAddress]::Parse("192.168.1.254")
$ping = Test-Connection -Count 1 -BufferSize 32 -TTL 64 -Quiet
for ($i = $startIP.Address; $i -le $endIP.Address; $i++) {
$ip = [System.Net.IPAddress]::new($i)
if ($ping -as [string] $ip) {
Write-Host "$ip is online"
}
}
What is a Ping Sweep?
A ping sweep is a network scanning technique used primarily to discover which computers or devices are active and reachable within a specified range of IP addresses. It sends ICMP echo requests (ping) to each address and listens for replies. This method is particularly valuable for network administrators, helping them verify device availability, troubleshoot connectivity issues, and maintain an accurate inventory of devices on the network.
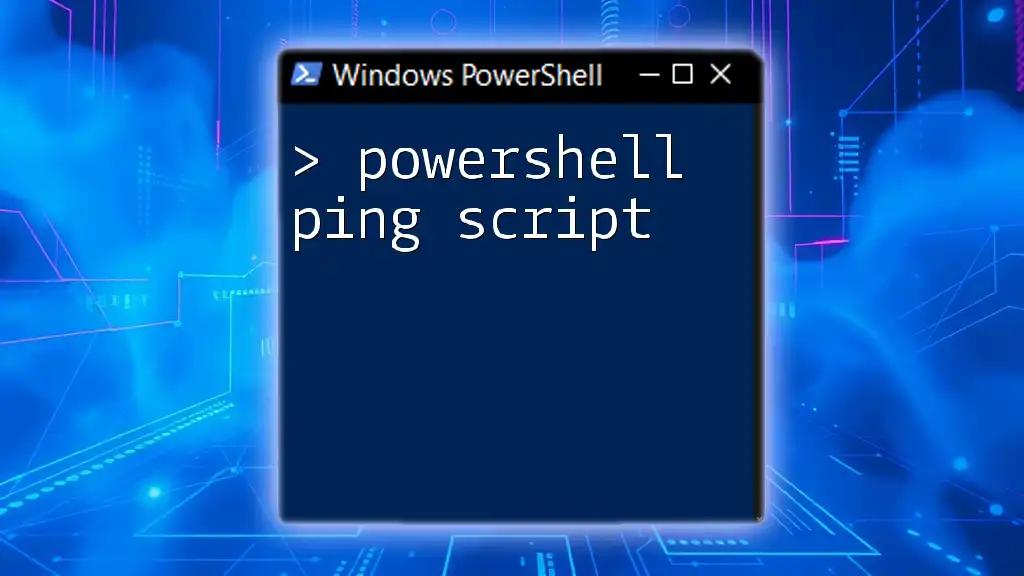
Why Use PowerShell for a Ping Sweep?
Using PowerShell for executing a ping sweep presents several advantages. First, PowerShell can handle bulk processing efficiently, thanks to its pipeline capabilities and built-in cmdlets. Additionally, it allows for easy scripting, enabling administrators to automate repetitive tasks and customize scans according to specific needs. PowerShell is not just limited to basic pings; it can also gather additional information, output results in various formats, and integrate seamlessly into larger scripts and workflows.

Preparing Your Environment
Before running any PowerShell Ping Sweep, it's essential to ensure your PowerShell environment is ready.
Setting Up PowerShell for Network Tasks
- Ensure Your PowerShell Version is Up to Date: Having the latest version of PowerShell guarantees access to all features and cmdlets, improving functionality and performance.
- Enabling Script Execution (Execution Policy): The default execution policy may prevent scripts from running. To enable script execution, open PowerShell as an administrator and execute:
Set-ExecutionPolicy RemoteSigned
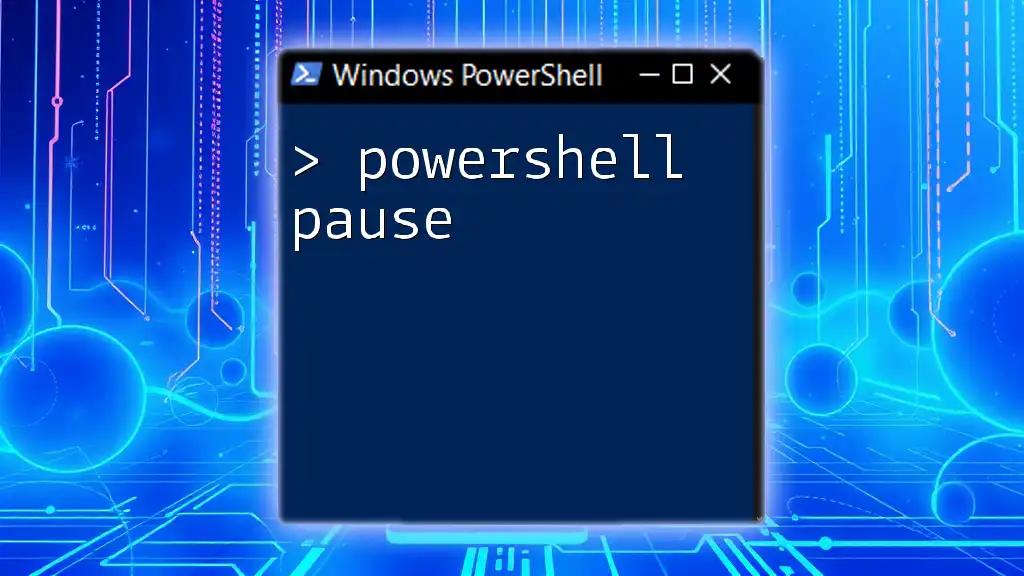
Understanding the Basics of Ping
What is Ping?
Ping is a utility that uses the Internet Control Message Protocol (ICMP) to send echo requests to a target IP address and waits for a response. This basic networking command helps diagnose connectivity issues and measure round-trip times.
Key Terminology
- Latency: The time it takes for a data packet to travel from the source to the destination and back.
- Packet Loss: The percentage of packets that are sent but do not arrive at their destination.
- Round-trip Time (RTT): The duration it takes for a packet to go from the sender to the receiver and back again.
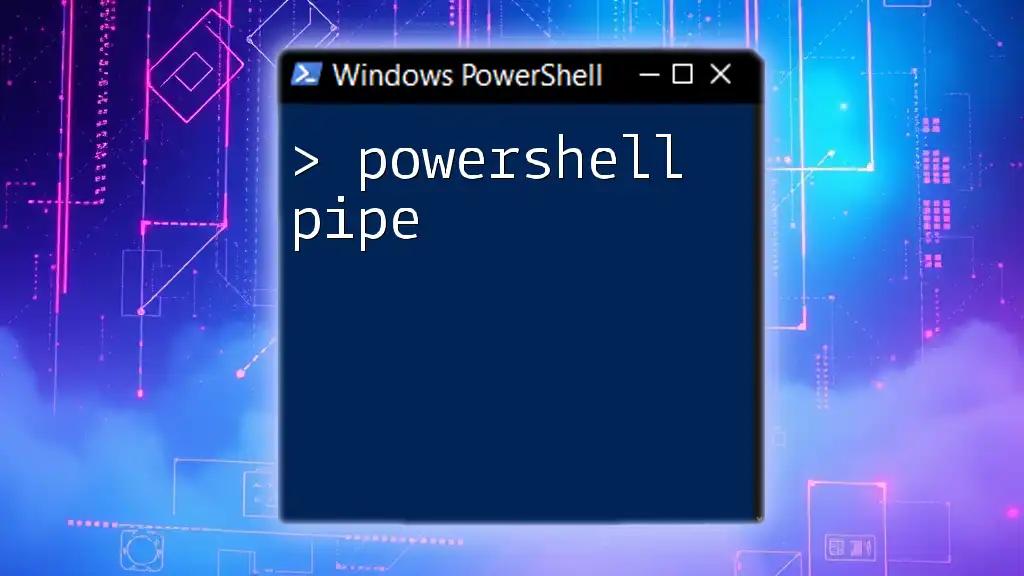
Executing a Basic Ping Command
Using the Ping Command in PowerShell
PowerShell provides a convenient cmdlet called `Test-Connection` that simplifies the pinging process. The basic syntax looks like this:
Test-Connection -ComputerName example.com -Count 4
In this example, Test-Connection attempts to ping `example.com` four times. Understanding the syntax and parameters is crucial for effective use. The key parameters include:
- `-ComputerName`: Specifies the target IP address or hostname.
- `-Count`: Defines how many echo requests to send.
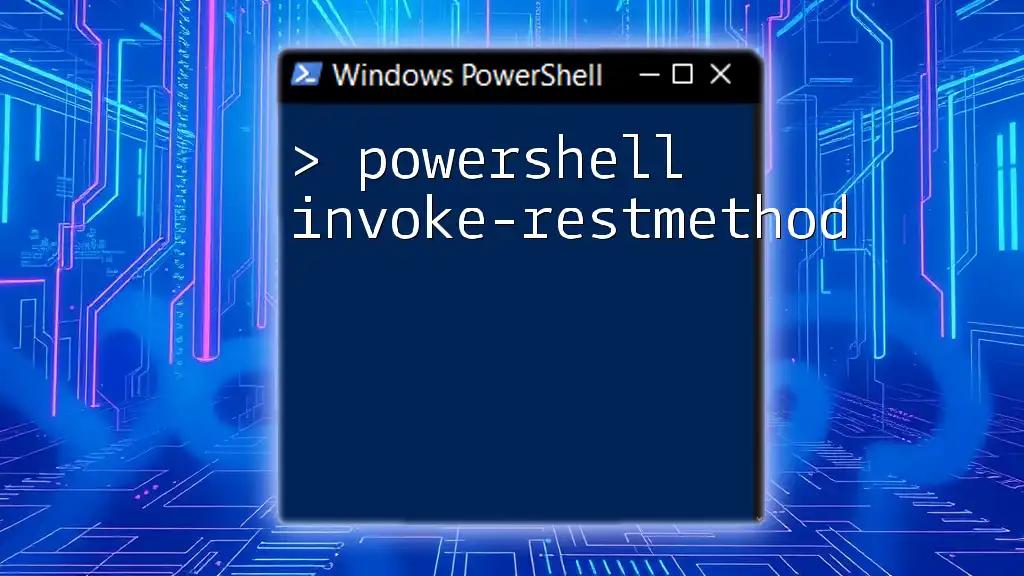
Creating a PowerShell Ping Sweep Script
The Fundamentals of a Ping Sweep Script
A PowerShell ping sweep script essentially automates the above process for a range of IP addresses. It involves looping through a specified range and executing the ping command on each IP.
Step-by-step Guide to Writing the Script
To create a ping sweep, you need to:
- Define the range of IP addresses you want to scan.
- Use a loop to iterate through each IP address.
Example Script for Ping Sweep
Here’s a simple script that performs a ping sweep across the local subnet:
$ipRange = 1..254
$network = "192.168.1."
foreach ($i in $ipRange) {
$ip = $network + $i
if (Test-Connection -ComputerName $ip -Count 1 -Quiet) {
Write-Output "$ip is online"
}
}
In this example:
- `$ipRange`: Defines the last octet of the IP addresses, ranging from 1 to 254.
- `$network`: Sets the first three octets of the IP address.
- The loop tests each IP address in the defined range, outputting whether it is online.

Enhancing the Ping Sweep with Additional Features
Collecting Additional Information
You can enhance your ping sweep by modifying the script to retrieve further details, such as response times, which provides insights into the network's performance.
Example of Adding the Response Time
Here’s how to modify the previous script to include response time information:
$response = Test-Connection -ComputerName $ip -Count 1
if ($response) {
Write-Output "$ip is online - Response Time: $($response.ResponseTime)ms"
}
This adjustment checks the response time for each reachable IP and includes it in the output.
Saving Results to a File
Another useful enhancement is saving the results of your ping sweep. This capability allows you to keep records for future reference or analysis.
Here’s how you can export results to a CSV file:
$results = @()
foreach ($i in $ipRange) {
$ip = $network + $i
if (Test-Connection -ComputerName $ip -Count 1 -Quiet) {
$results += [PSCustomObject]@{IP = $ip; Status = "Online"}
}
}
$results | Export-Csv -Path "ping_sweep_results.csv" -NoTypeInformation
In this adjusted script:
- `$results` is an array that accumulates the online results.
- Each online IP is added as a custom object.
- Finally, the results are exported to a CSV file for easy analysis.
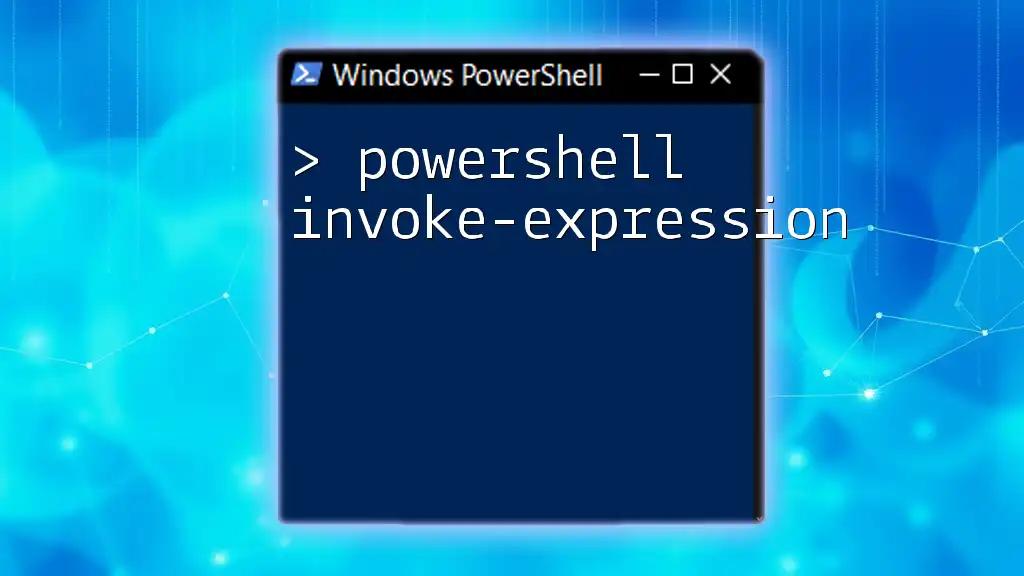
Troubleshooting Common Issues
Common Errors During Ping Sweeps
There can be various reasons why some IP addresses don’t respond:
- The device is powered off or disconnected.
- Firewalls may block ICMP requests.
- The IP address may not be in use or configured incorrectly.
Improving Ping Sweep Accuracy
To minimize false positives and improve accuracy:
- Ensure firewalls on the target devices are configured to allow ICMP traffic.
- Consider implementing longer timeout intervals to account for latency.

Comparing PowerShell Ping Sweeps to Other Methods
Traditional Ping Tools vs. PowerShell
While traditional ping tools are effective for simple operations, they lack the versatility and automation potential of PowerShell. PowerShell allows for script customization, output formatting, and integration with other tasks, making it a more robust solution.
When to Use PowerShell for Networking Tasks
Choose PowerShell for ping sweeps when:
- You need to scan multiple subnets or ranges.
- You want to collect additional metrics or automate repetitive tasks.
- You require organized reporting of your findings.
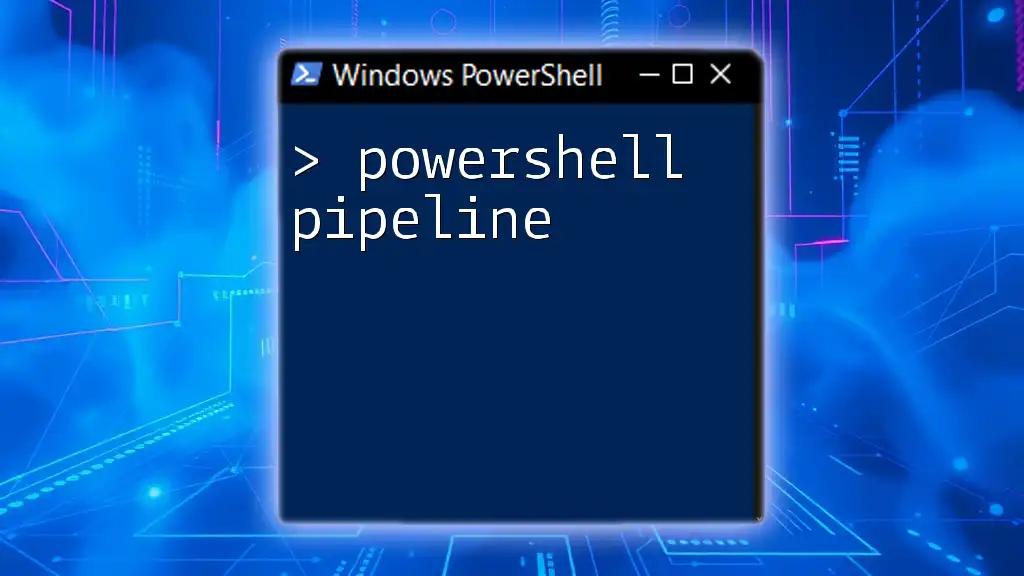
Conclusion
In this guide, we explored the essential concept of a PowerShell ping sweep, from its definition to practical implementations. By utilizing PowerShell's powerful capabilities, you can streamline network diagnostics, enhance your troubleshooting capabilities, and maintain a comprehensive view of your network's health.

Additional Resources
For those looking to deepen their PowerShell knowledge, consider visiting the official [PowerShell Documentation](https://docs.microsoft.com/en-us/powershell/) and exploring online communities. Books and courses focused on PowerShell scripting are also excellent resources for advancing your skills in this area.