To quickly turn off your monitor using PowerShell, you can use the following command:
Add-Type -AssemblyName System.Windows.Forms; [System.Windows.Forms.SendKeys]::SendWait('{F15}')
This command simulates a key press that can effectively turn off your display on many systems.
Understanding Monitor Power Management
What is Monitor Power Management?
Monitor power management refers to the way computer systems control the power states of the display, including turning it on, off, or putting it into a sleep mode. Properly managing these states is essential not only for energy conservation but also for extending the lifespan of your monitor by minimizing wear.
When a monitor is turned off, it stops drawing power, which can lead to reduced electricity bills and a lower environmental footprint. The ability to turn off the monitor programmatically using PowerShell gives users flexibility and control over their system's power settings.
How does PowerShell interact with hardware?
PowerShell can interact with hardware settings through the Windows Management Instrumentation (WMI) and the .NET Framework. This integration allows PowerShell to execute commands that can change the states of hardware components. Understanding how PowerShell communicates with your system's hardware will enable you to automate many tasks related to power management efficiently.
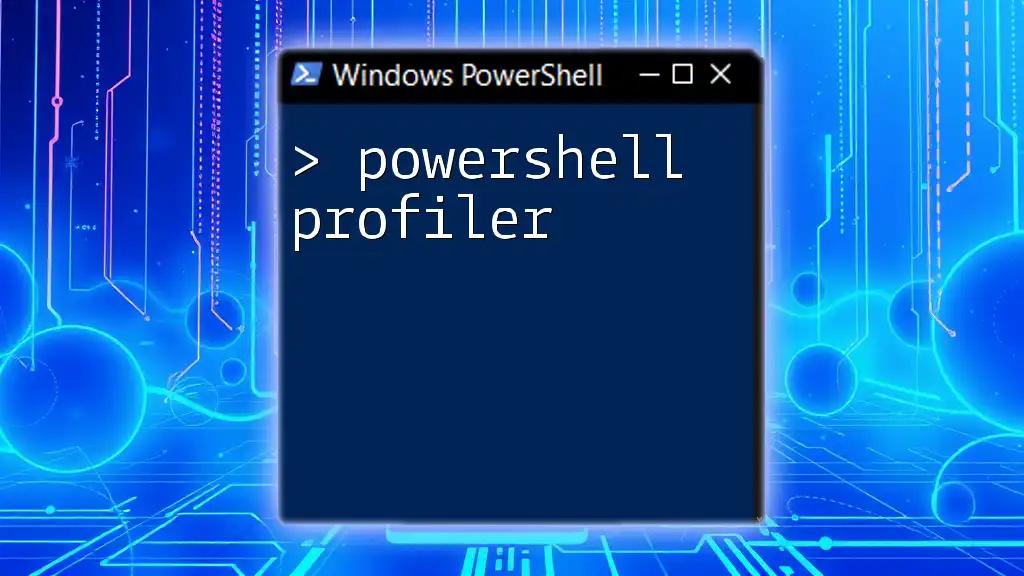
Prerequisites
System Requirements
To execute PowerShell commands effectively, you should be using a supported Windows operating system such as Windows 10 or Windows Server 2016 and above. If your system is outdated, it may not have the necessary libraries to execute certain commands.
Necessary Permissions
Some PowerShell commands, especially those interacting directly with hardware settings, will require administrative permissions. You should ensure that you are running PowerShell as an administrator to prevent any permission-related issues when trying to turn off your monitor.
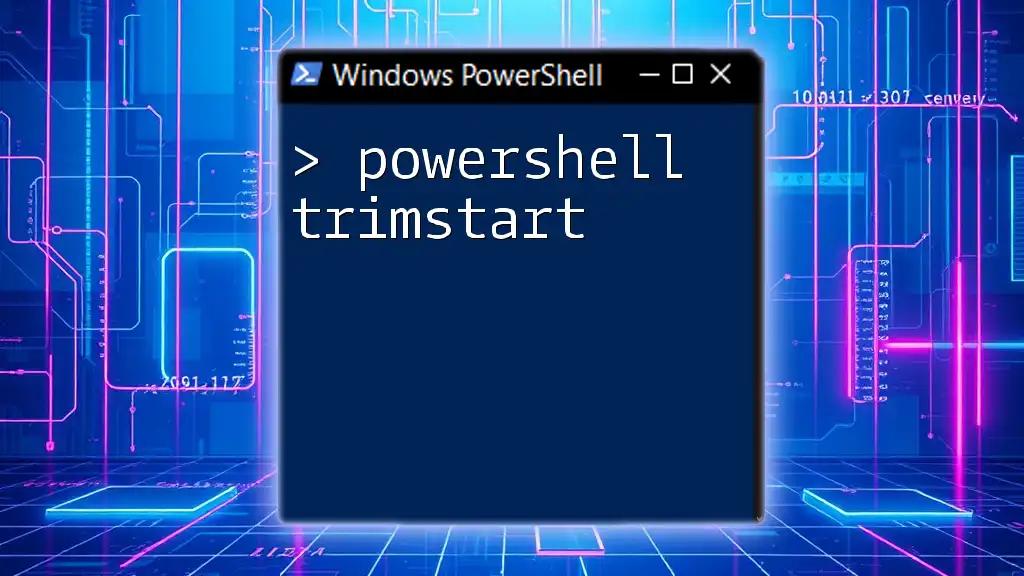
Turning Off the Monitor with PowerShell
Basic Command to Turn Off the Monitor
Here's a straightforward method to turn off your monitor using PowerShell:
Add-Type -AssemblyName System.Windows.Forms
[System.Windows.Forms.SendKeys]::SendWait('{F2}')
This code snippet utilizes the `System.Windows.Forms` assembly to send a key event that mimics the action of pressing the "F2" key, which typically would turn off the monitor. It effectively simulates user input to achieve the desired outcome.
Alternative Method Using `DisplaySwitch.exe`
If the above method does not work, an alternative approach is to use the built-in `DisplaySwitch.exe`. You can use the following command:
Start-Process DisplaySwitch.exe /off
`DisplaySwitch.exe` is a tool that controls display settings for dual monitors, but it can also be leveraged to turn off the primary display.
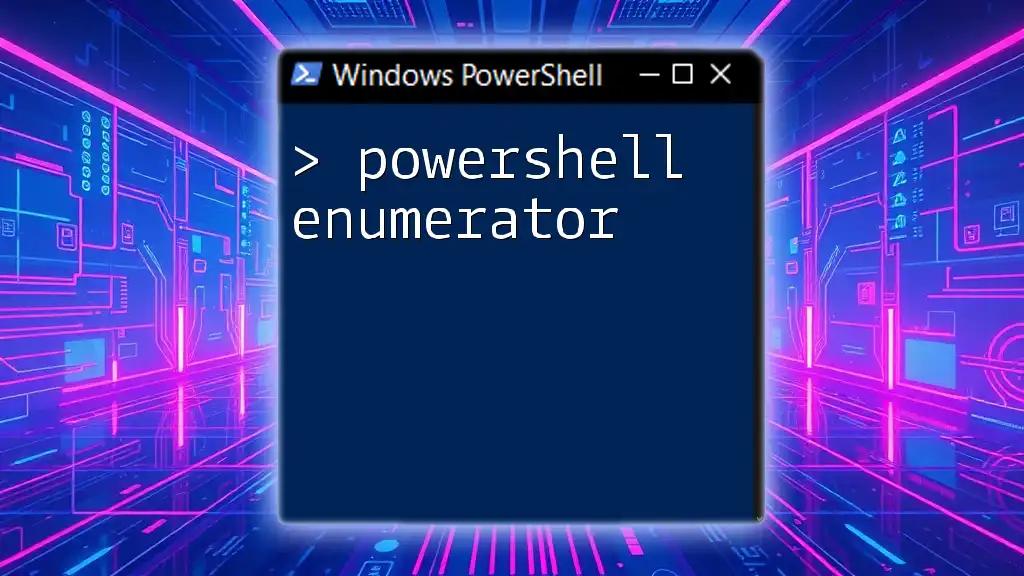
Troubleshooting Common Issues
PowerShell Errors and Solutions
Should you experience errors when running the commands, check whether you have the latest version of PowerShell installed on your system. Ensure you're running the command as an administrator. If you encounter syntax errors, double-check your typing for any missed characters or extra spaces.
Monitor Does Not Turn Off
In some cases, despite executing the correct commands, your monitor may refuse to power down. This could be due to several reasons, including hardware compatibility settings or active processes keeping it awake. You can check your Windows power settings under "Control Panel" > "Hardware and Sound" > "Power Options" for any configurations that may be preventing the monitor from turning off.
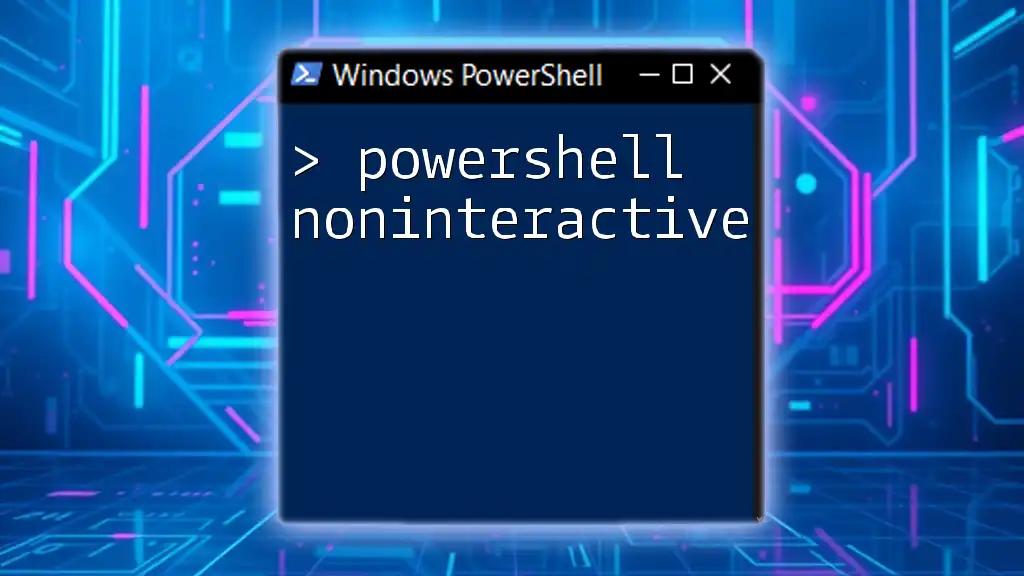
Scheduling Monitor Off Command
Using Task Scheduler to Automate Monitor Turn Off
Automating the process to turn off the monitor can enhance user experience significantly. You can create a scheduled task that allows your monitor to turn off at designated times. Here's a step-by-step guide on how to do this:
- Open Task Scheduler: Search for "Task Scheduler" in the Windows search bar and open it.
- Create a New Task: Click on "Create Basic Task" in the right panel.
- Set the Name and Trigger: Give your task a name and specify when you want it to trigger (for example, daily at 9 p.m.).
Here’s the PowerShell command to execute in the "Action" part of your task:
$action = New-ScheduledTaskAction -Execute 'powershell.exe' -Argument 'Start-Process DisplaySwitch.exe /off'
$trigger = New-ScheduledTaskTrigger -At 9pm -Daily
Register-ScheduledTask -Action $action -Trigger $trigger -Id 'TurnOffMonitor' -User 'SYSTEM'
This script creates a scheduled task that sends a command to turn off the monitor every day at 9 p.m.
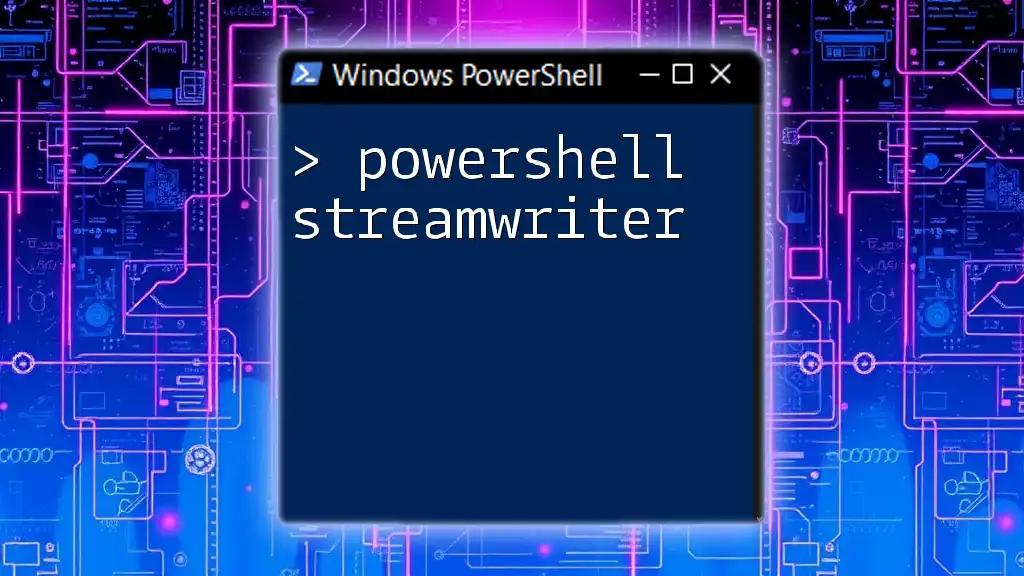
Conclusion
Incorporating PowerShell into your daily routine to manage monitor power settings can save energy and extend the life of your display. By mastering how to programmatically turn off your monitor, you gain not only convenience but also the opportunity to automate tasks effectively.
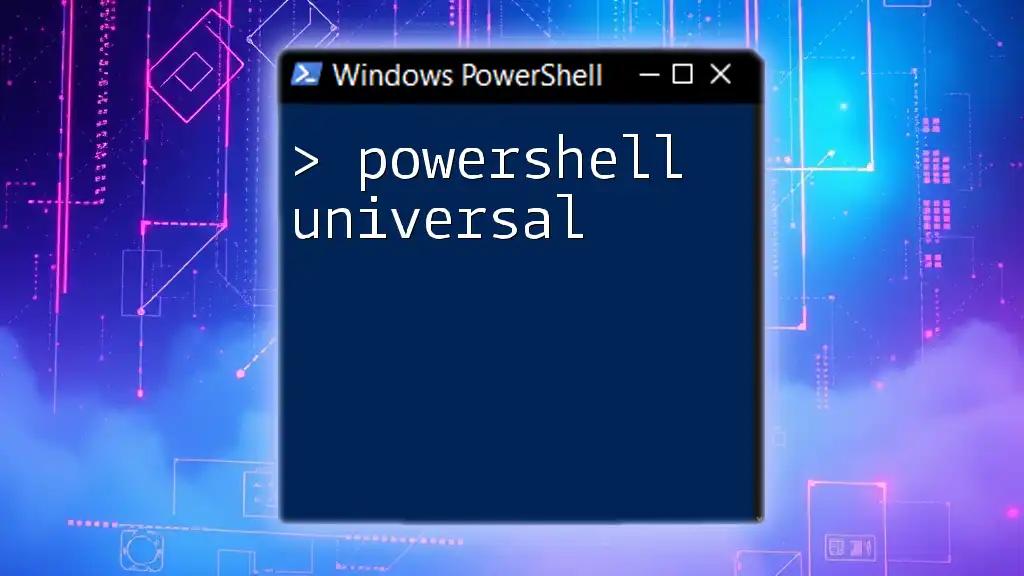
Additional Resources
For those eager to expand their PowerShell knowledge, the following resources can be invaluable:
- Official PowerShell documentation on Microsoft’s website.
- Community forums and groups dedicated to PowerShell for real-time discussions and troubleshooting.
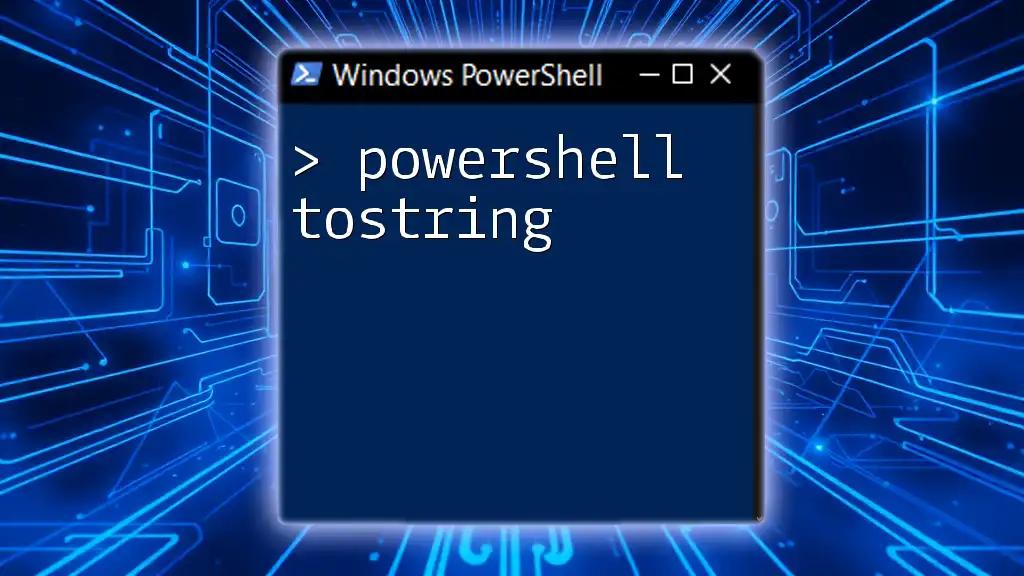
FAQs
Can I turn off multiple monitors?
While the commands provided primarily focus on turning off a single monitor, you can adapt them for multi-monitor setups by executing the command for each display.
Will turning off my monitor affect my applications running?
No, turning off the monitor using PowerShell will not affect any applications or processes running in the background. Your system remains active and functional while the display is powered down.

Call to Action
If you found this guide helpful, be sure to subscribe for more PowerShell tips and tutorials, and don't hesitate to leave comments with questions or suggestions for future topics you'd like to learn about!