You can easily check the connectivity status of a list of computers using PowerShell's `Test-Connection` cmdlet in combination with a loop.
Here’s a simple code snippet to ping a list of computers:
$computers = @("Computer1", "Computer2", "Computer3")
foreach ($computer in $computers) {
Test-Connection -ComputerName $computer -Count 1 -ErrorAction SilentlyContinue |
Select-Object Address, ResponseTime, Status
}
Understanding the `ping` Command in PowerShell
What is the `ping` Command?
The `ping` command is a powerful network diagnostic tool used to check the connectivity between your computer and another device on the network. In PowerShell, this command is accessible through the `Test-Connection` cmdlet. Ping works by sending Internet Control Message Protocol (ICMP) echo request messages to the specified computer and waiting for a reply. If the target computer is reachable, you will receive a series of replies that indicate the response time and packet loss.
How `ping` Works
When you issue a ping command, the sending computer transmits small packets of data, called ICMP packets, to the target device. The target device, if reachable, sends back a reply. This process helps you determine whether the network is functioning correctly, as well as the responsiveness of the device.
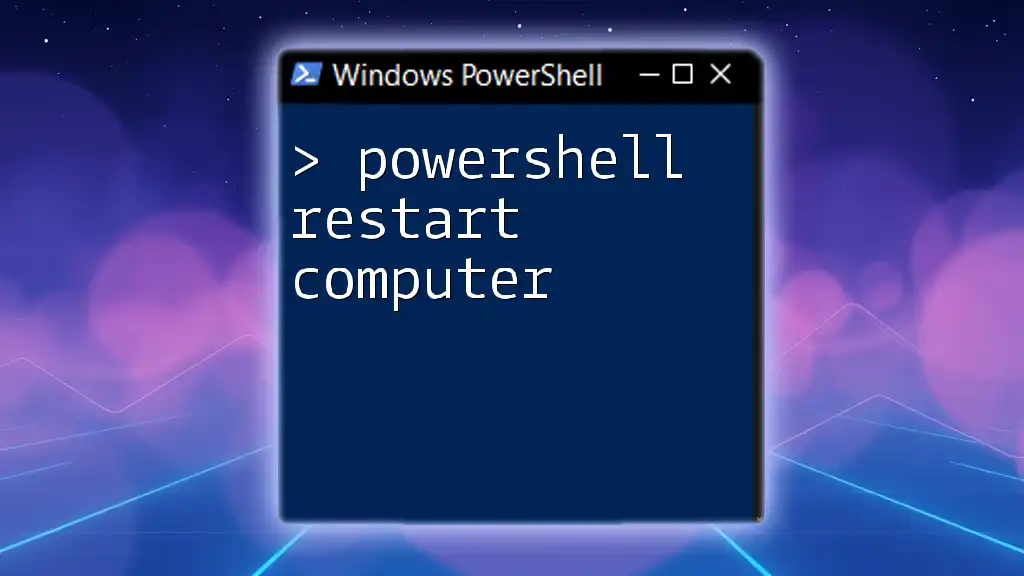
Setting Up Your Environment for PowerShell
Opening PowerShell
To start working with PowerShell, you need to open the PowerShell interface. You can do this by:
- Pressing Windows + X and selecting “Windows PowerShell” or “Windows PowerShell (Admin)” if you need elevated permissions.
- Searching for "PowerShell" in the Start Menu and selecting it.
Make sure you are aware of which version of PowerShell you are using—Windows PowerShell is the traditional version, while PowerShell Core offers cross-platform compatibility and a range of additional features.
Permissions and Required Environments
Sometimes, to execute certain commands, especially those involving scripts, you may require elevated privileges. Launching PowerShell as an Administrator provides the necessary permissions to run all commands, particularly when interacting with system properties or network resources.
Additionally, you may need to adjust the script execution policy to allow scripts to run. You can do this by entering the following command:
Set-ExecutionPolicy RemoteSigned -Scope CurrentUser
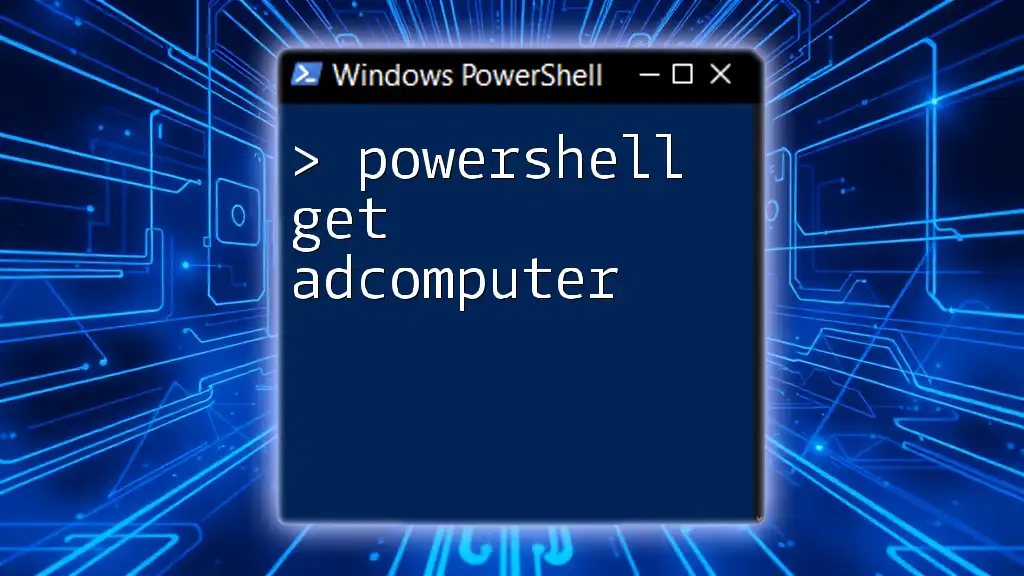
Creating a List of Computers
Format of Computer List
When working with a list of computers in PowerShell, it's essential to have this list in a manageable format. The list can be stored directly in your script as an array, read from a text file, or built using a CSV file.
Example: Creating a Simple Computer List
You can define a straightforward list of computers as an array in your PowerShell script:
$computers = "PC1", "PC2", "PC3"
This creates an array named `$computers` with three entries, making it easy to loop through them later.
Loading a Computer List from a File
If you have a long list of computers, it’s often more practical to keep these in a separate text file. You can easily read the contents of the file into your PowerShell variable using the following command:
$computers = Get-Content -Path "C:\path\to\your\computers.txt"
This command will read each line from the specified file into an array, ensuring that each entry corresponds to a computer name.
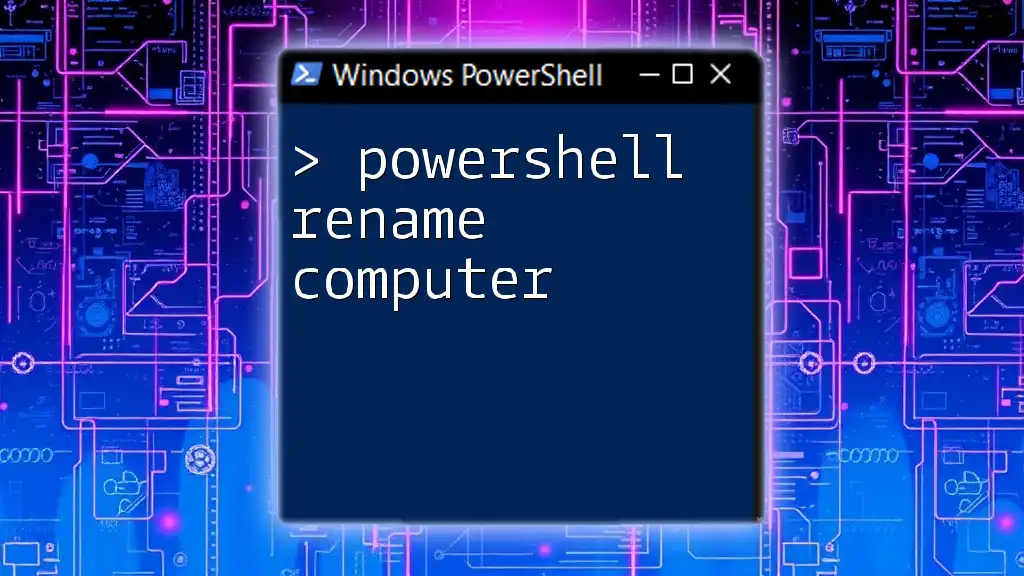
Using PowerShell to Ping a List of Computers
Basic Ping Command Syntax
To ping a single computer, the command is quite straightforward. Here’s how you can use the `Test-Connection` cmdlet:
Test-Connection -ComputerName $computer -Count 2
In this command, `$computer` is a placeholder for the variable that contains the computer name you want to ping, and `-Count` specifies how many echo requests you want to send.
Looping Through the List of Computers
To ping each computer in your list, you can use a `foreach` loop. This allows you to iterate over each entry in the array and run the ping command for each:
foreach ($computer in $computers) {
Test-Connection -ComputerName $computer -Count 2
}
With this loop, PowerShell will go through each computer in `$computers` and ping them one after another.
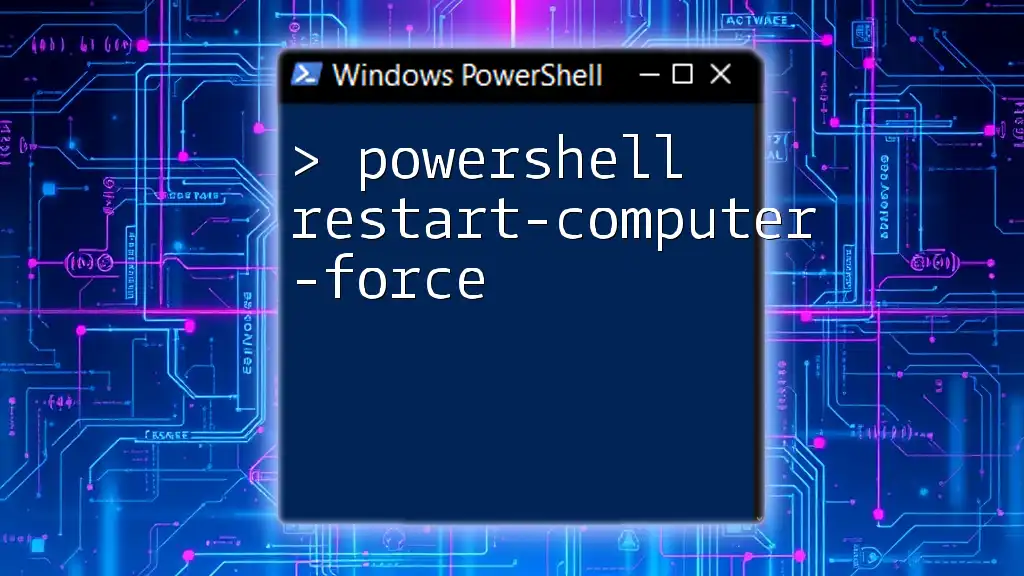
Advanced Ping Options
Customizing the Ping Command
The `Test-Connection` cmdlet offers various parameters to customize your ping tests. You can adjust the number of requests sent, the delay between requests, and even set a Time To Live (TTL) value. For example:
Test-Connection -ComputerName $computer -Count 5 -Delay 1 -TTL 128
In this command, `-Delay` specifies a one-second delay between each ping, and `-TTL` sets the maximum number of hops for the packet.
Handling Exceptions and Errors
While conducting network checks, errors may arise if the target device is unreachable. To handle these errors gracefully, you can use `try` and `catch` blocks to manage exceptions:
try {
Test-Connection -ComputerName $computer -Count 2 -ErrorAction Stop
} catch {
Write-Host "$computer is not reachable"
}
This encapsulates your ping command within a `try` block, and if an error occurs, it will be caught in the `catch` block, allowing you to output a user-friendly message indicating the unreachable computer.
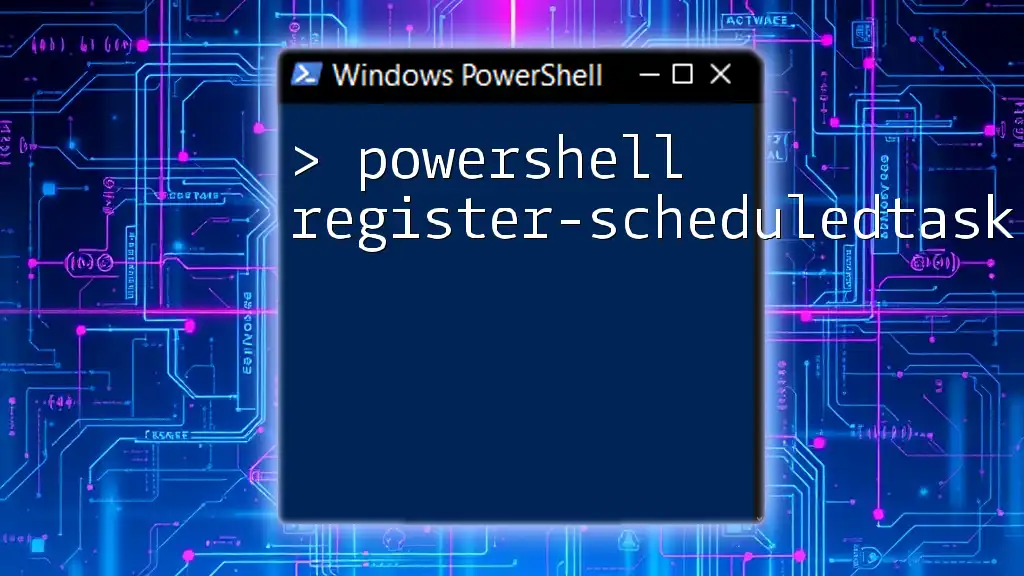
Outputting Results
Displaying Results in Console
Upon executing the ping commands, you will likely want to see the results in real-time. By default, `Test-Connection` will output the results to the console. You can also use `Write-Host` for custom output messages, such as indicating a successful ping.
Exporting Results to a File
If you require a permanent record of your network checks, you can easily export the results to a text or CSV file. This is particularly useful for documentation and reporting purposes:
Test-Connection -ComputerName $computer -Count 2 | Export-Csv -Path "C:\path\to\output.csv" -NoTypeInformation
This command will compile the results of your ping checks into a CSV format, which can then be opened in programs like Microsoft Excel for deeper analysis.
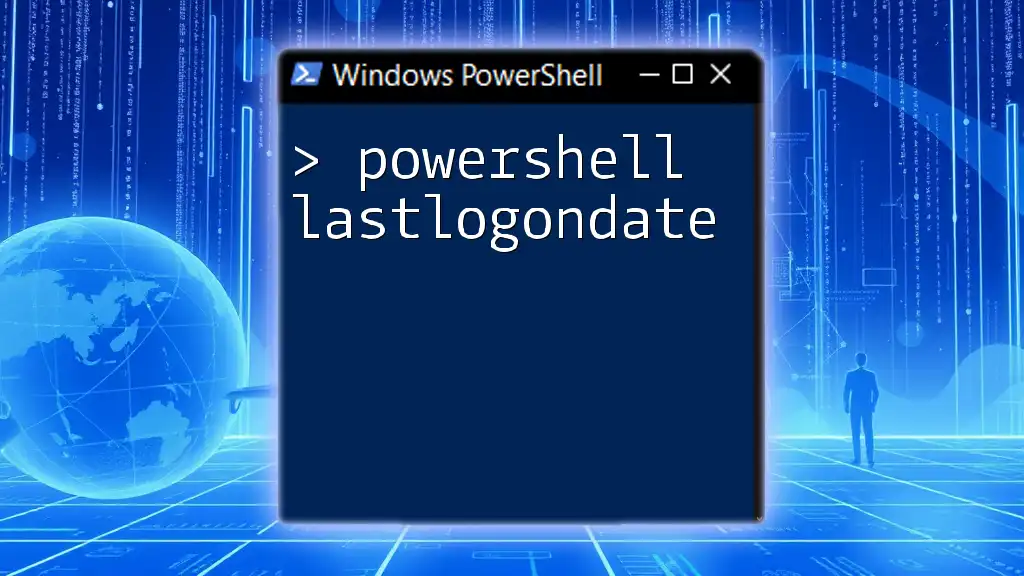
Practical Applications of a PowerShell Ping Script
Network Monitoring
Using PowerShell to ping a list of computers can help network administrators monitor the health and connectivity of critical devices. By running these scripts periodically, they can receive immediate feedback on network status.
Troubleshooting Connectivity Issues
When users report connectivity problems, having a ping test ready can assist in diagnosing problems rapidly. By executing the PowerShell ping commands, administrators can easily identify which computers are down and require further investigation.
Automating Routine Checks
For businesses that need to ensure constant network availability, automating the ping checks using Windows Task Scheduler can be an effective strategy. By scheduling a script that runs at regular intervals, administrators can be alerted to issues before they escalate.
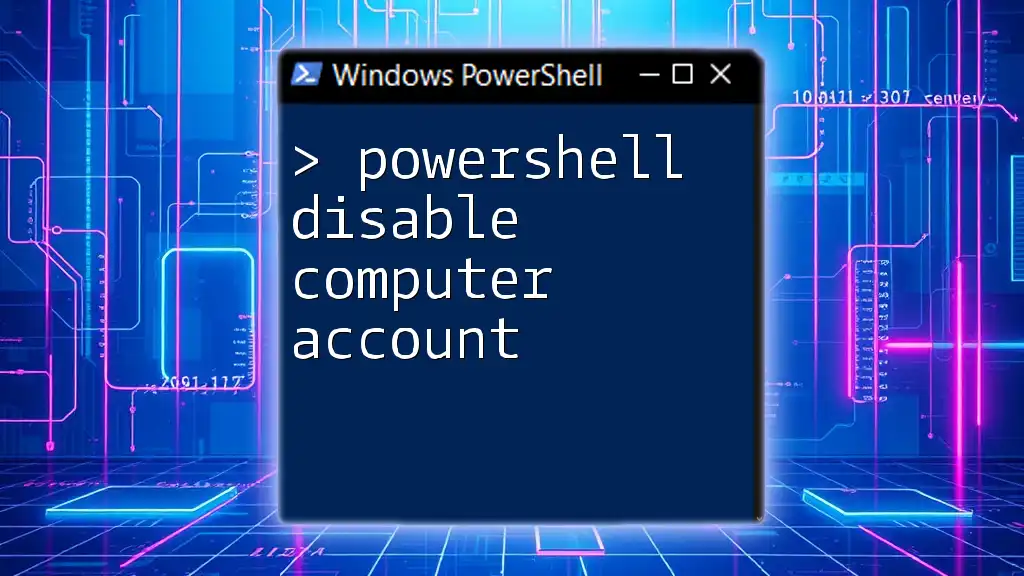
Conclusion
By leveraging the capabilities of PowerShell to ping a list of computers, you can significantly enhance your network management and troubleshooting processes. This guide has outlined the essential commands, examples, and error handling techniques necessary for efficiently executing ping tests. As you become more comfortable with PowerShell, feel free to explore additional cmdlets and expand your scripts to better fit your unique networking needs.
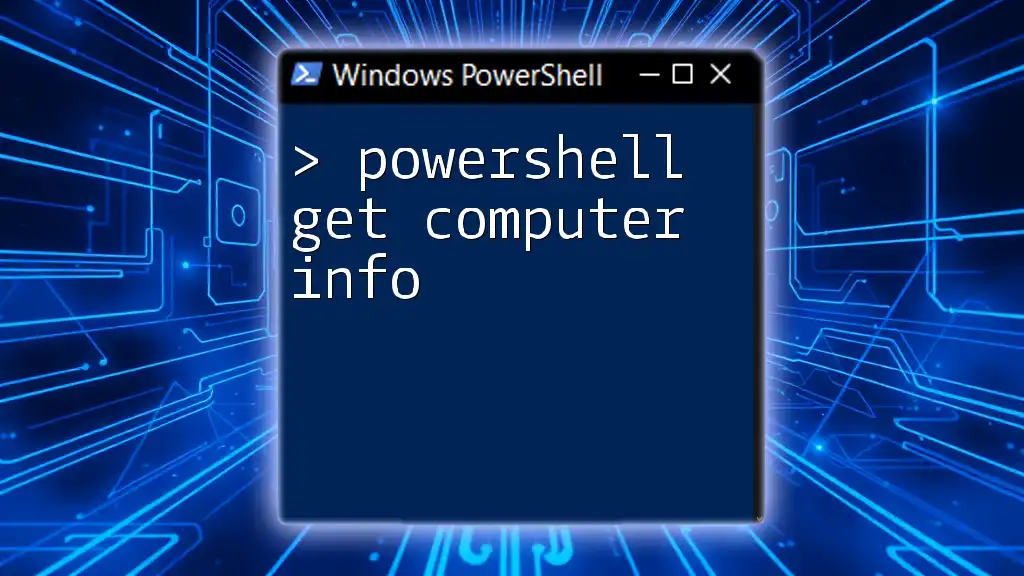
Additional Resources
References and Documentation
For an in-depth understanding of the PowerShell `Test-Connection` cmdlet and other networking commands, refer to the official Microsoft documentation.
Tutorials and Courses
If you're looking to expand your PowerShell knowledge, consider exploring online tutorials and courses dedicated to scripting and automation.