You can easily retrieve the currently logged-in user in PowerShell by using the following command:
Get-LoggedInUser = whoami
Write-Host "The currently logged-in user is: $Get-LoggedInUser"
This concise command utilizes `whoami` to display the username of the active session.
Understanding PowerShell User Cmdlets
What Are Cmdlets?
Cmdlets are lightweight commands in the PowerShell environment, designed to perform specific tasks. They follow a verb-noun naming convention that makes it easier to understand their purpose. For example, common cmdlets include `Get-Process`, `Set-Content`, and `Get-ChildItem`.
PowerShell and User Management
Managing user sessions is crucial for system administrators, especially in environments with multiple users. PowerShell provides a unique ability to retrieve information about who is currently logged into the system, making it an invaluable tool for user management.
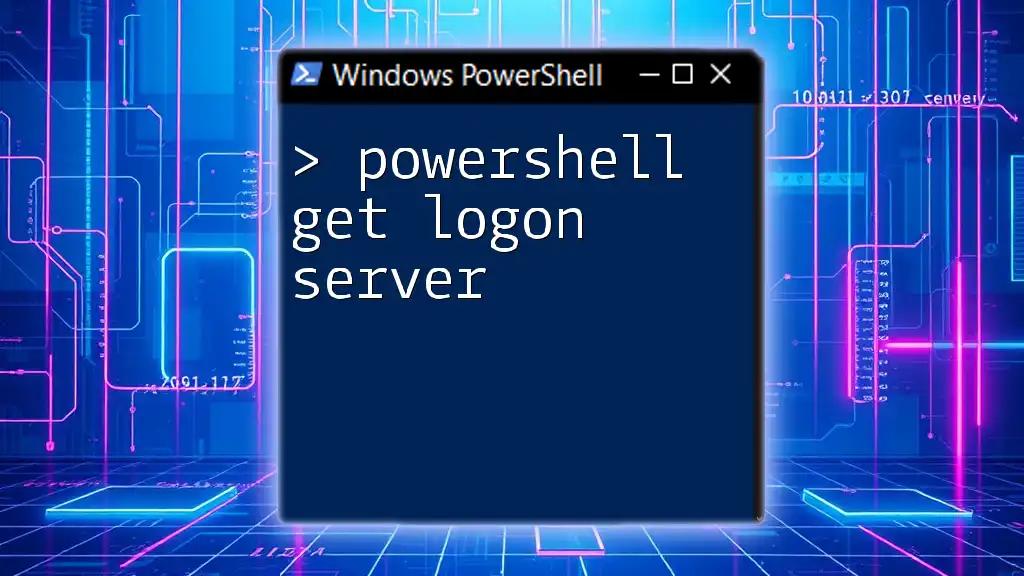
PowerShell Get Logged In User
Using Get-WmiObject
One common method to get logged in user information is through the `Get-WmiObject` cmdlet, which queries Windows Management Instrumentation (WMI) for system information. To retrieve the logged-in user, you can use the following command:
Get-WmiObject -Class Win32_ComputerSystem
This command provides a variety of system details, including the `UserName` property, which indicates the currently logged-in user. The output will resemble the following structure:
UserName : DOMAIN\Username
Using Query User
Another effective way to identify who is logged onto the machine is by using the `query user` command. This command gives you a list of all users currently logged into the system. Simply type:
query user
The output will display user names, session IDs, and their corresponding statuses, making it easy to see who is active.
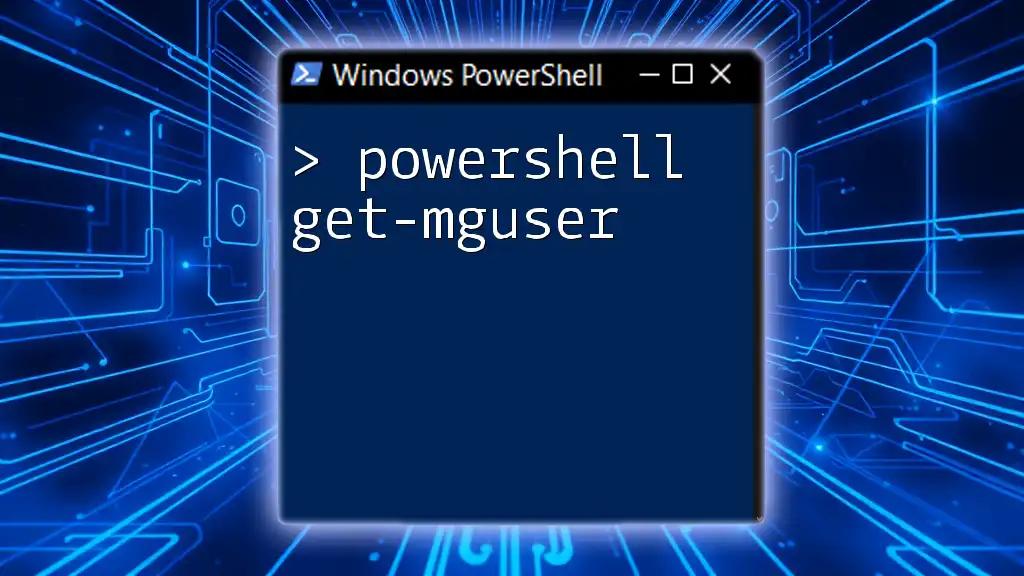
PowerShell Get Current Logged On User
How to Use Environment Variables
You can easily retrieve the current logged-in user by accessing the Windows environment variables. The command to get the user name is straightforward:
$env:USERNAME
This method works well for scripts that need the information without querying the entire system, providing a quick and efficient means to access the username.
Using [System.Security.Principal.WindowsIdentity]
For a more programmatically robust approach, consider using the .NET class `System.Security.Principal.WindowsIdentity`. It allows users to retrieve the currently logged-in user's identity with the following command:
[System.Security.Principal.WindowsIdentity]::GetCurrent().Name
This command returns the full name of the user, including the domain if applicable. Using this method ensures that you're getting the most accurate representation of the logged-in user.
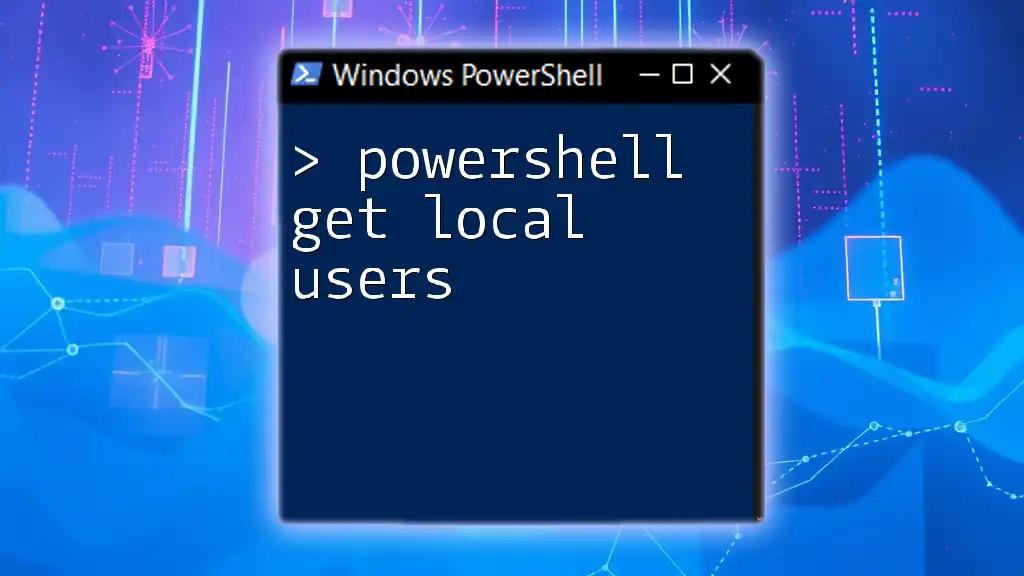
Get Logged In User PowerShell
Exploring the Get-Process Cmdlet
The `Get-Process` cmdlet can also be used to find logged-in users by associating processes with users. You can execute this command to retrieve a list of processes along with their user names:
Get-Process -IncludeUserName
This will provide output that includes the user that started each process, helping you correlate which users are currently utilizing system resources.
Filtering the Results
To refine your search and find a specific user, you can combine the `Get-Process` cmdlet with a `Where-Object` filter. For example, if you are looking for all processes running under the user "Username," the command would look like this:
Get-Process -IncludeUserName | Where-Object { $_.UserName -eq 'DOMAIN\Username' }
This command narrows down the results to just processes owned by the specified user, making it easier to monitor user activity.

Finding Logged In Users
PowerShell and Active Directory
In environments where Active Directory (AD) is employed, PowerShell can query AD to check for logged-in users. You can use the following command to find users who have logged on within the last day:
Get-ADUser -Filter * | Where-Object { $_.LastLogon -gt (Get-Date).AddDays(-1) }
This command searches all Active Directory users and filters them based on their last logon date, providing a comprehensive insight into user activity across the network.
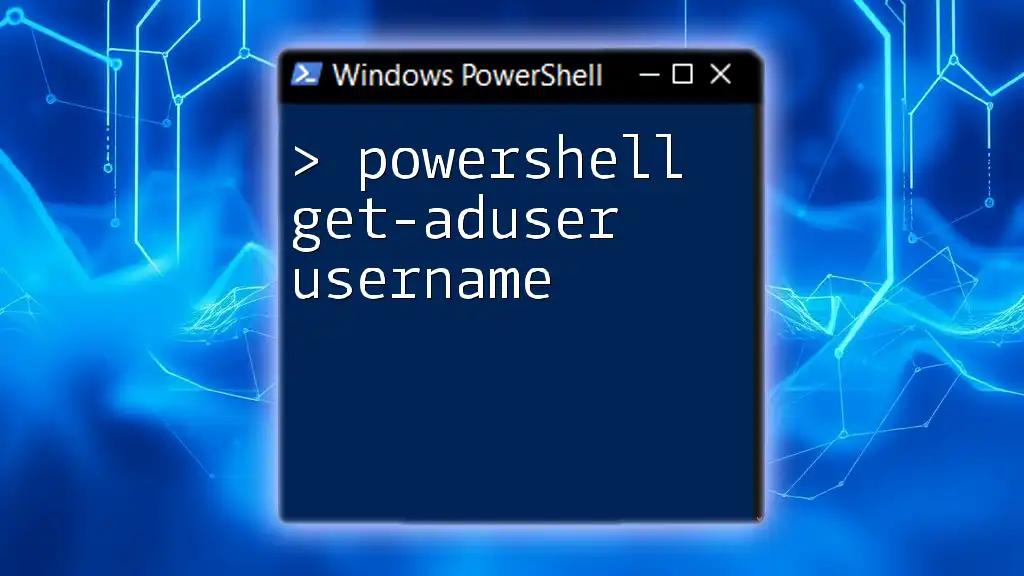
PowerShell Show Logged In Users
Utilizing Get-LoggedOnUser Function
For convenience, you can create a reusable function in PowerShell that showcases currently logged-in users. An example function might look like this:
function Get-LoggedOnUser {
$loggedUsers = query user
return $loggedUsers
}
Get-LoggedOnUser
This function encapsulates the logic to display logged-in users and allows for quick execution whenever needed.
Using Events to Track Logins
PowerShell has the ability to retrieve security event logs to track user login events. Using `Get-WinEvent`, you can fetch the relevant event IDs to find out who logged in at what time:
Get-WinEvent -LogName Security | Where-Object { $_.Id -eq 4624 }
Event ID 4624 corresponds to successful user logins, and reviewing this log can provide comprehensive insights into user access patterns.
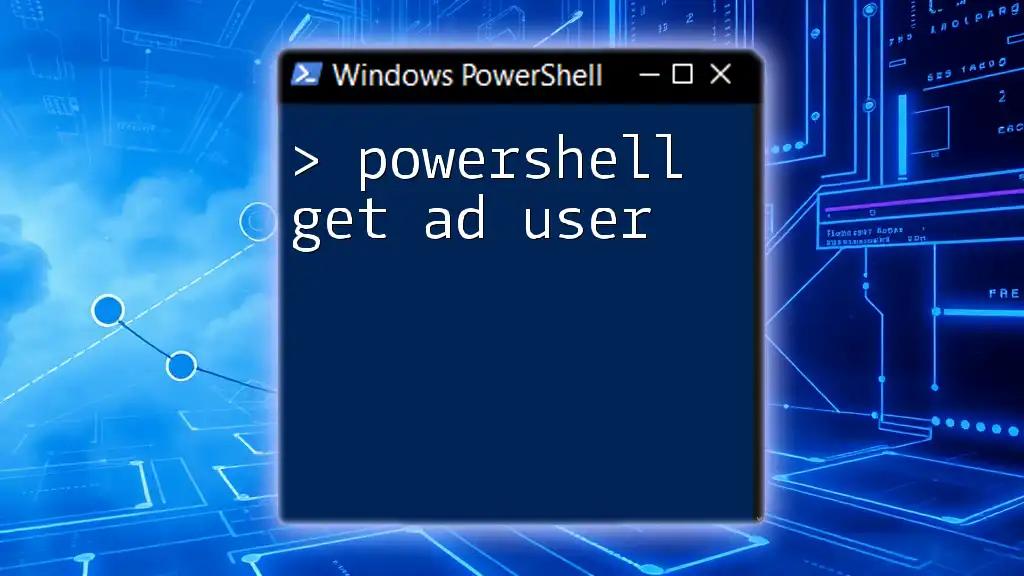
Get Currently Logged In User PowerShell
Accessing User Session Information
For a straightforward user session overview, the `quser` command can be helpful. Running this command in PowerShell provides a summary of all active sessions on the server:
quser
This command displays user names, session IDs, and their states, allowing administrators to identify active sessions at a glance.
Cross-Checking with Services
Sometimes user sessions can be validated by checking associated services. Use the following command to list running services, which can aid in cross-referencing user activity:
Get-Service | Where-Object { $_.Status -eq 'Running' }
Managing user services can help ensure resources are being utilized efficiently.
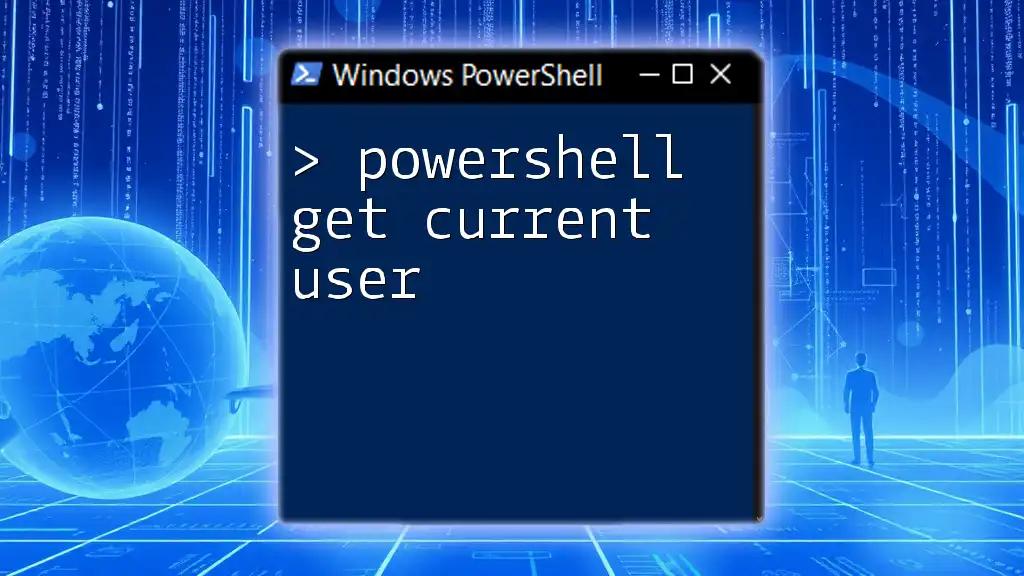
Advanced PowerShell Techniques
Creating Scripts for Automation
For those looking to automate user monitoring further, writing a script to periodically check for logged-in users can be beneficial. Consider this basic template for scripting:
while ($true) {
Get-WmiObject -Class Win32_ComputerSystem | Select-Object UserName
Start-Sleep -Seconds 60
}
This script will continually check and output the currently logged-in user every minute until you stop it.
Error Handling and Debugging
When executing commands that retrieve logged-in user information, you may encounter errors or unexpected output. Implementing error handling techniques can help catch issues early. Use `Try-Catch` blocks to manage exceptions gracefully:
try {
Get-WmiObject -Class Win32_ComputerSystem
} catch {
Write-Host "An error occurred: $_"
}
By adopting good error handling practices, you not only protect your scripts from crashing but also provide clear feedback on what went wrong.

Conclusion
Summarizing the essential PowerShell commands outlined above, it’s clear that retrieving logged-in user information is both crucial and accessible through various methods. Whether you are using WMI, querying Active Directory, or accessing event logs, PowerShell provides a robust toolkit for monitoring user sessions efficiently. For learners eager to delve deeper, numerous resources are available to enhance their PowerShell proficiency and user management skills.