PowerShell is a powerful scripting language that allows users to interact with Windows Management Instrumentation (WMI) to retrieve system information and manage Windows devices through concise commands.
Here’s a code snippet that demonstrates how to query WMI for the operating system information on a local machine:
Get-WmiObject Win32_OperatingSystem | Select-Object Caption, Version, OSArchitecture
Understanding WMI
What is WMI?
Windows Management Instrumentation (WMI) is a core component of Windows operating systems that provides a standardized framework for managing and monitoring system resources. It serves as an interface through which scripts and applications can query and manipulate system settings, performance metrics, and various hardware/software configurations. The significance of WMI lies in its ability to provide administrators with a deep insight into the operating system, allowing for more effective management and troubleshooting.
The Role of WMI in PowerShell
PowerShell, a powerful scripting language and shell designed specifically for system administration, leverages WMI to facilitate the retrieval and management of system information. This integration allows users to automate tasks, gather system statistics, and modify configurations seamlessly. By utilizing WMI within PowerShell, users can streamline their administrative duties and achieve greater efficiency in system management.
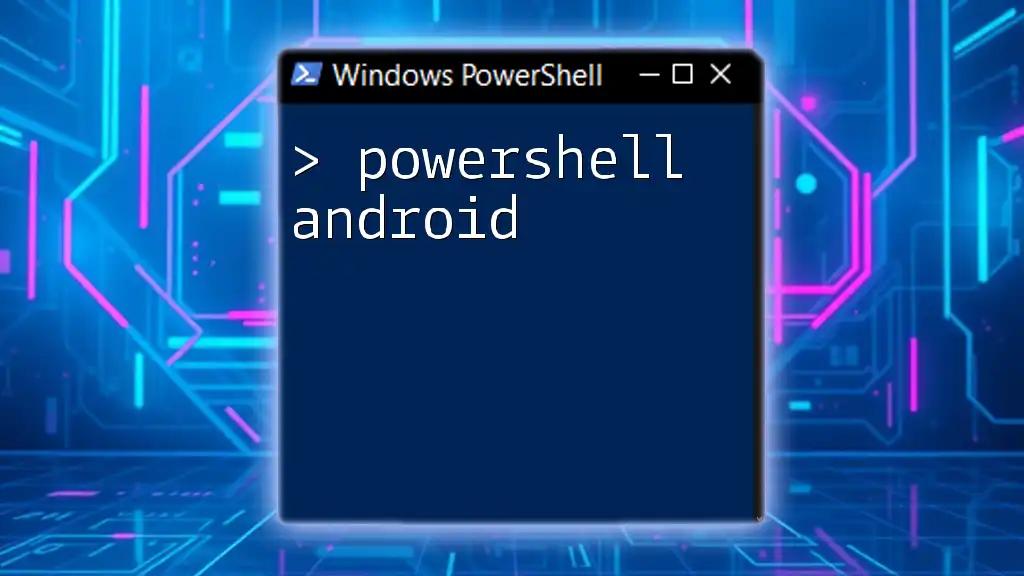
Setting Up Your Environment
Prerequisites
Before using PowerShell with WMI, ensure you have the following prerequisites:
- PowerShell Version: Make sure you are running PowerShell version 2.0 or later, as these versions contain extensive support for interacting with WMI.
- Supported Operating Systems: WMI is available across various Windows platforms, including Windows 7, 8, 10, and Windows Server editions.
Enabling WMI
In most Windows installations, WMI is enabled by default. However, it is prudent to verify this by checking the status:
- Open the Services console (`services.msc`) and locate the service named Windows Management Instrumentation.
- Ensure the Startup type is set to Automatic, and the service is currently running.
If you encounter issues with WMI, consider using the WMI Diagnosis Utility provided by Microsoft to diagnose and resolve common WMI problems.
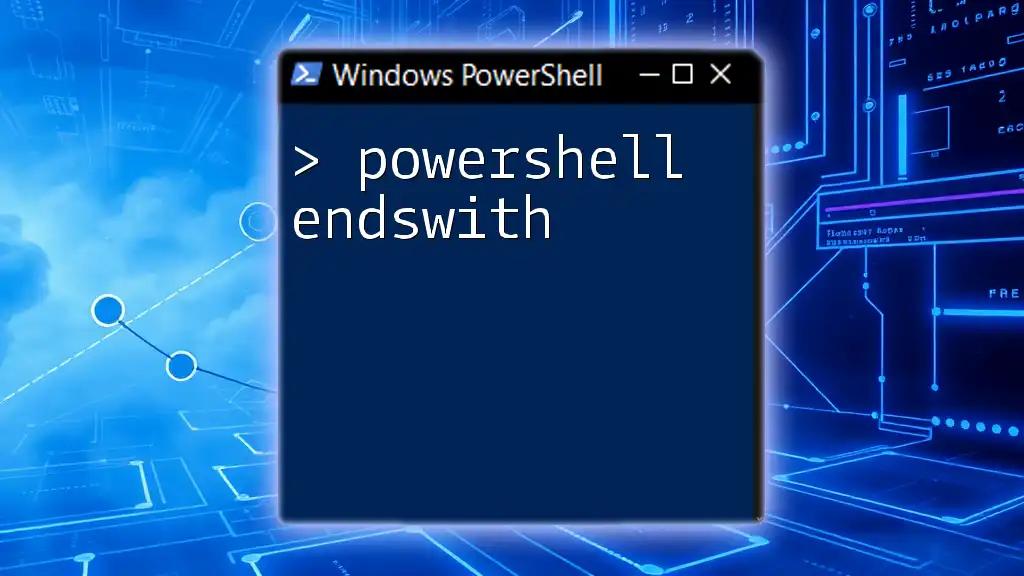
PowerShell Cmdlets for WMI
Overview of Get-WmiObject and Get-CimInstance
Two primary cmdlets facilitate WMI access in PowerShell: Get-WmiObject and Get-CimInstance. While both serve similar purposes, they have distinct differences regarding their usage and performance.
- Get-WmiObject: This cmdlet works with WMI using DCOM, which can introduce latency in remote management scenarios.
- Get-CimInstance: Introduced with PowerShell 3.0, this cmdlet interacts with WMI over WinRM (Windows Remote Management), offering better performance and compatibility with non-Windows systems.
Basic Syntax for WMI Queries
When querying WMI, it’s vital to understand the basic syntax:
Get-WmiObject -Class <ClassName> -Namespace <Namespace>
For example, to obtain information about the operating system, you might use:
Get-WmiObject -Class Win32_OperatingSystem
This query retrieves an object representing the current operating system, granting access to various properties such as `Version`, `BuildNumber`, and `OSArchitecture`.

Performing Common WMI Queries
System Information Queries
Retrieving essential system information is a fundamental task in system administration. To obtain detailed information about your computer's specifications, run the following command:
Get-WmiObject -Class Win32_ComputerSystem
This command provides critical data, including the computer's model, manufacturer, total physical memory, and number of logical processors.
Process Management Queries
To list all currently running processes, use:
Get-WmiObject -Class Win32_Process
This command returns a plethora of information about each process, including the `ProcessId`, `Name`, and `Handle`. This information is invaluable when diagnosing performance issues or terminating rogue processes.
Hardware Information Queries
To gain insights into the hardware installed on your system, such as hard drives, execute the following command:
Get-WmiObject -Class Win32_DiskDrive
This command reveals details such as `Model`, `Size`, and `Partitions` for each physical disk, enabling effective hardware management.
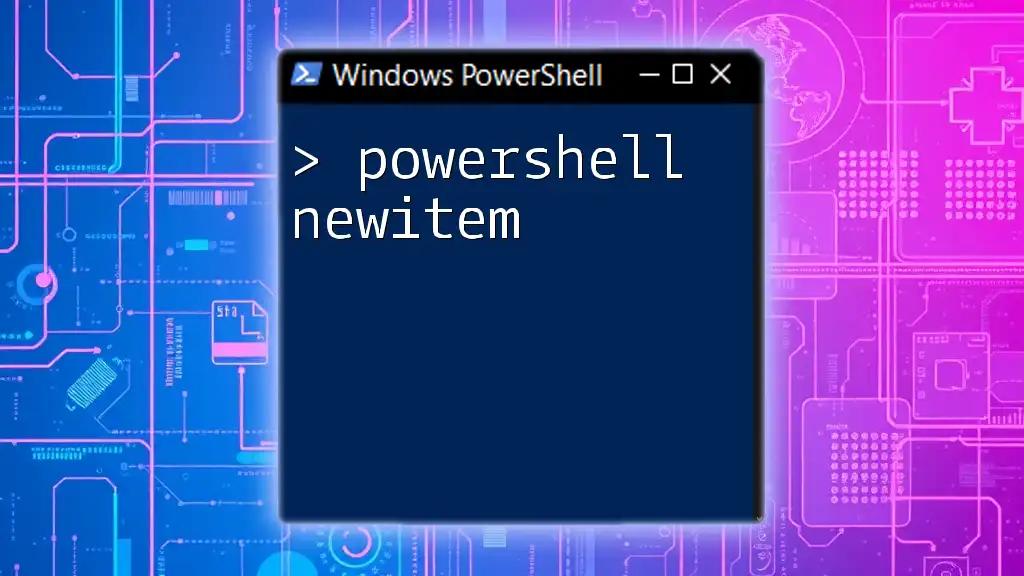
Working with WMI Filters
Creating WMI Queries with Filters
The `-Filter` parameter allows for more targeted WMI queries, improving efficiency. For example, to query a specific process by name, use:
Get-WmiObject -Class Win32_Process -Filter "Name = 'notepad.exe'"
This command returns information only about instances of Notepad that are currently running, significantly reducing unnecessary data output.
Handling Multiple Filters
To execute queries with multiple conditions, you can employ `AND` and `OR` operators. For instance, the following command retrieves information about both Notepad and Calculator processes:
Get-WmiObject -Class Win32_Process -Filter "Name = 'notepad.exe' OR Name = 'calc.exe'"
This flexible querying capability enables streamlined maintenance of system resources.
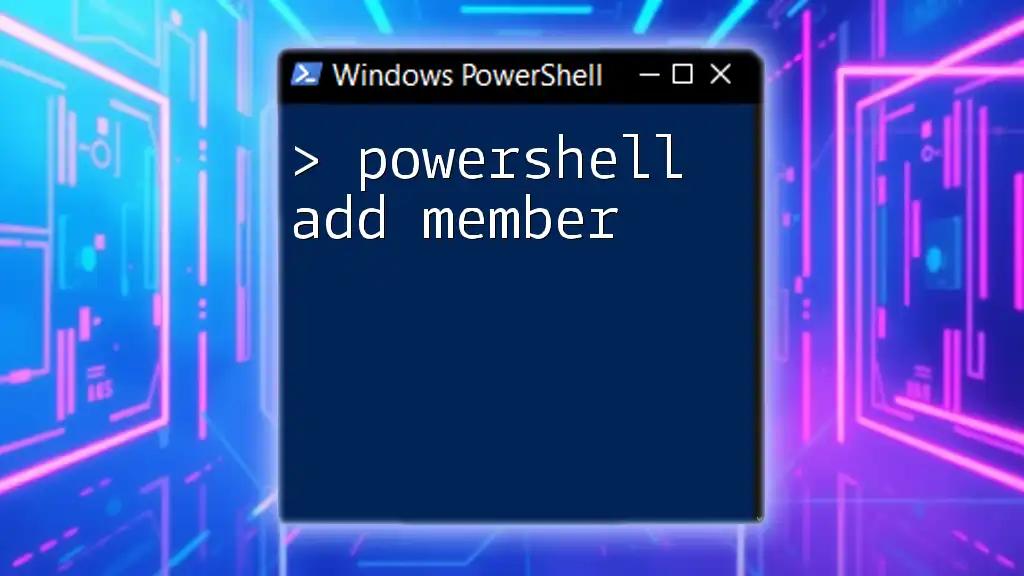
Advanced WMI Techniques
Writing Custom WMI Queries
To customize the output of WMI queries, utilize Select-Object to specify particular properties. For example, retrieving specific drive information can be done using:
Get-WmiObject -Class Win32_LogicalDisk | Select-Object DeviceID, FreeSpace, Size
This command focuses on only the most relevant properties, making the output easier to read.
Error Handling in WMI Queries
It's essential to implement error handling, particularly in scripts that invoke WMI queries. A structured approach using Try/Catch blocks enhances reliability. For instance:
try {
Get-WmiObject -Class Win32_NonExistentClass
} catch {
Write-Host "Error occurred: $_"
}
This example captures any errors from the WMI query and outputs a helpful message, allowing users to troubleshoot issues promptly.
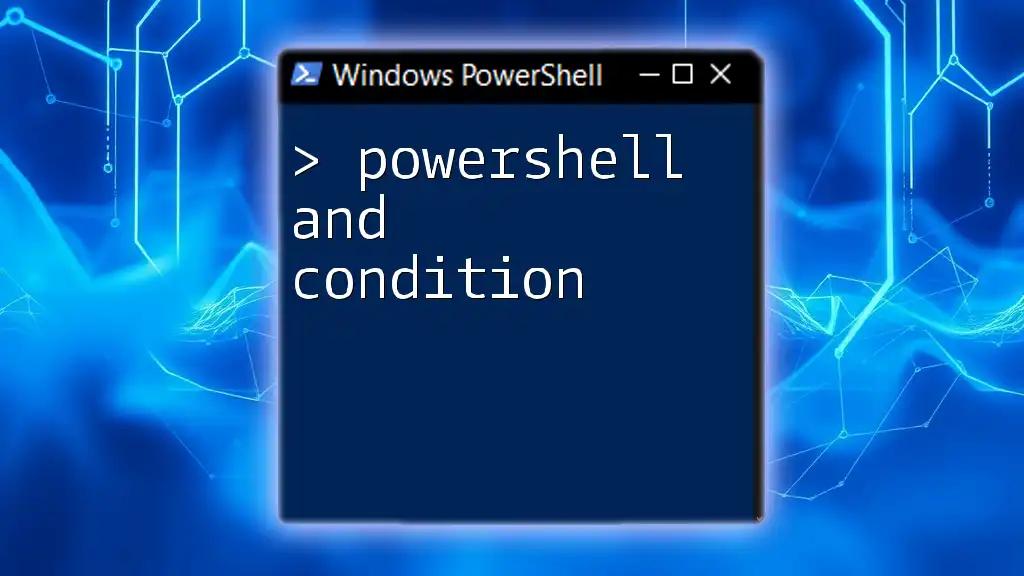
Automating WMI Queries
Creating PowerShell Scripts
To streamline repetitive tasks, consider creating PowerShell scripts for WMI queries. A simple template might look as follows:
# Define the output file
$outputFile = "C:\Reports\WMIReport.txt"
# Query and export results
Get-WmiObject -Class Win32_OperatingSystem | Out-File $outputFile
This script not only queries the operating system information but also exports the data to a text file for later review.
Scheduling WMI Tasks
To ensure consistent monitoring or reporting, use Task Scheduler to run your PowerShell scripts at defined intervals. By creating a scheduled task and pointing it to your PowerShell script, you can automate WMI queries without any manual intervention.
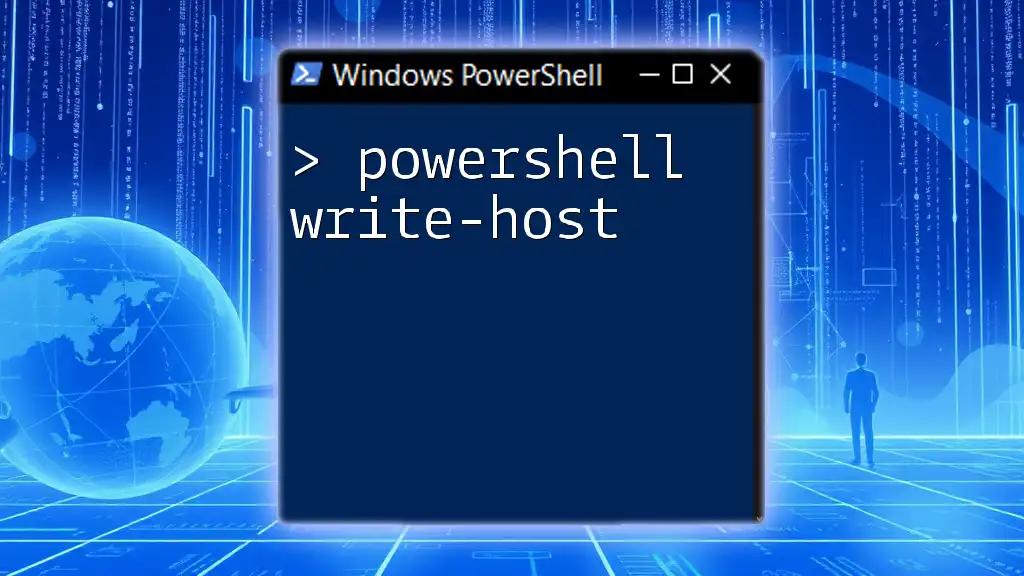
Best Practices for Using WMI in PowerShell
Performance Considerations
When working with WMI, it's vital to focus on performance. Optimize your queries by:
- Limiting the number of properties requested to only those necessary.
- Utilizing WMI filters effectively to reduce the scope of returned data.
- Avoiding unnecessary loops or repetitive queries.
Security Implications
Understanding WMI security permissions is critical, especially in multi-user environments. Ensure that only authorized users can run scripts that interact with WMI. Use PowerShell's built-in security features to safeguard sensitive information and maintain compliance with organizational security policies.
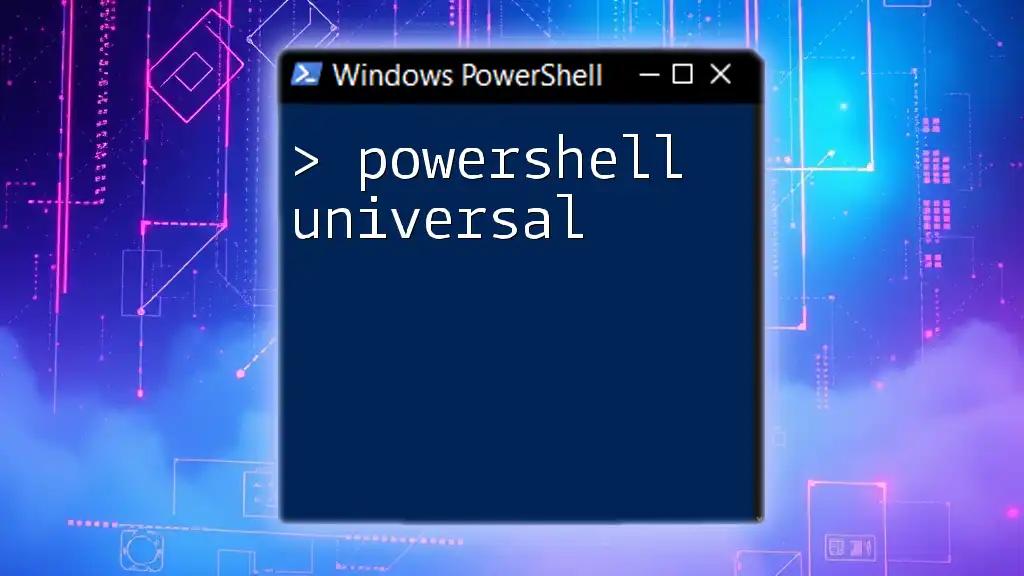
Conclusion
Recap of WMI and PowerShell Benefits
By effectively utilizing PowerShell and WMI, administrators can automate complex tasks, gather vital system data, and manage hardware/software settings more efficiently. This powerful combination enhances productivity and provides a robust framework for system management.
Next Steps
Now that you’ve grasped the essentials of PowerShell and WMI, practice the commands and techniques outlined above. As you become more familiar, explore additional resources and learning materials to further master this essential administrative skill set.