To add a new column to a CSV file in PowerShell, you can import the CSV, add the column data, and then export it back to a new CSV file using the following code snippet:
Import-Csv 'input.csv' | ForEach-Object { $_ | Add-Member -MemberType NoteProperty -Name 'NewColumn' -Value 'YourValue' } | Export-Csv 'output.csv' -NoTypeInformation
What is a CSV File?
CSV (Comma-Separated Values) files are a widely used file format for storing tabular data. Each line in a CSV file represents a single record, and each record consists of fields separated by commas. This simplicity and compatibility with various applications, including spreadsheets and databases, make CSV files essential in data management.
PowerShell serves as a powerful tool to manipulate CSV files efficiently, allowing for quick reads, edits, and writes. By leveraging PowerShell, users can automate tasks, analyze data, and manage files seamlessly.
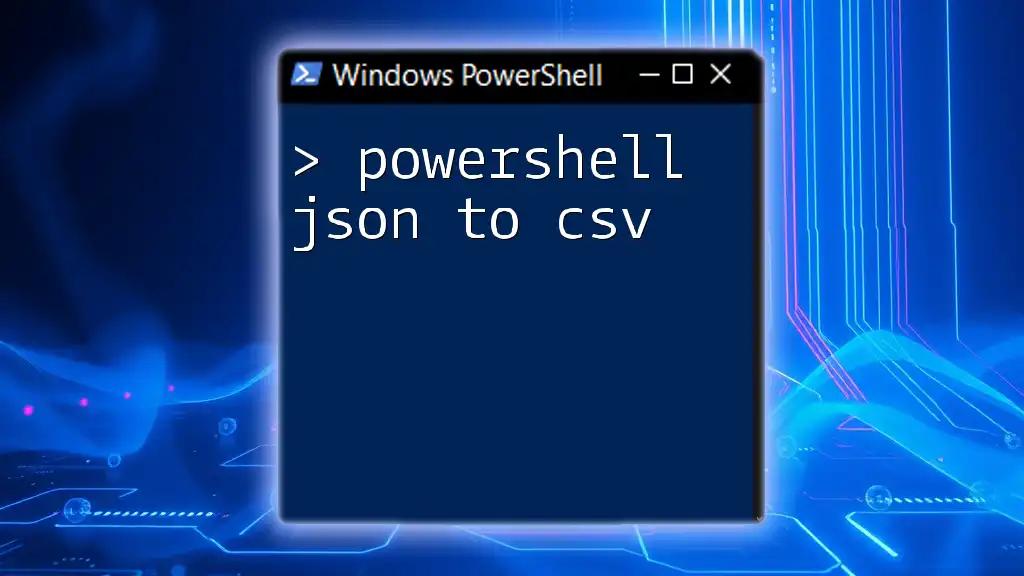
Understanding PowerShell Basics
What is PowerShell?
PowerShell is an advanced scripting language and command-line shell designed specifically for system administration and automation. It offers a myriad of capabilities that differentiate it from traditional command-line interfaces by enabling administrators to work with .NET objects rather than simple text output. This object-oriented approach enhances the ability to manage and manipulate data more effectively.
Common PowerShell Cmdlets
To work with CSV files in PowerShell, certain cmdlets are indispensable. Here are a few that are critical for manipulating CSV data:
- `Import-Csv`: Reads a CSV file and imports its data into PowerShell objects.
- `Export-Csv`: Exports PowerShell objects into a CSV format, with control over how the data is structured.
- `Select-Object`: Allows users to select specific properties to output, which can include newly added columns.
Understanding these cmdlets is crucial for handling CSV files efficiently in PowerShell.
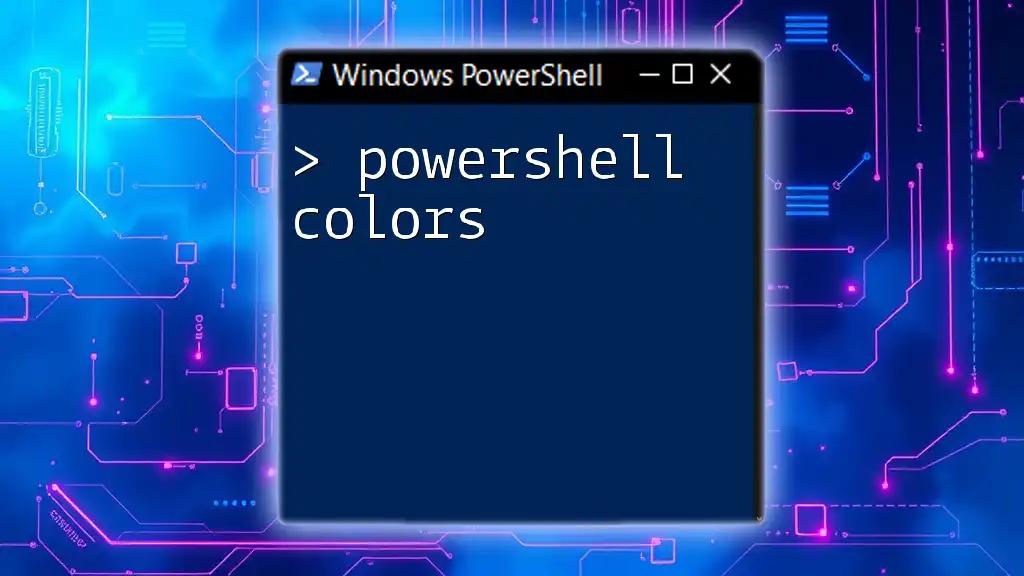
Preparing Your Environment
Installing PowerShell
To start using PowerShell, you should ensure that it is installed on your system.
- Windows: PowerShell is installed by default; you can upgrade to PowerShell Core for additional features.
- macOS: Use the Homebrew package manager to install PowerShell with the command `brew install --cask powershell`.
- Linux: Follow the installation instructions specific to your distribution on the PowerShell GitHub page.
It is important to check that you have the latest version by running:
$PSVersionTable
Opening PowerShell
Launching PowerShell varies by platform:
- Windows: Search for "PowerShell" in the Start Menu and select Windows PowerShell.
- macOS/Linux: Open your terminal and type `pwsh` to start PowerShell Core.
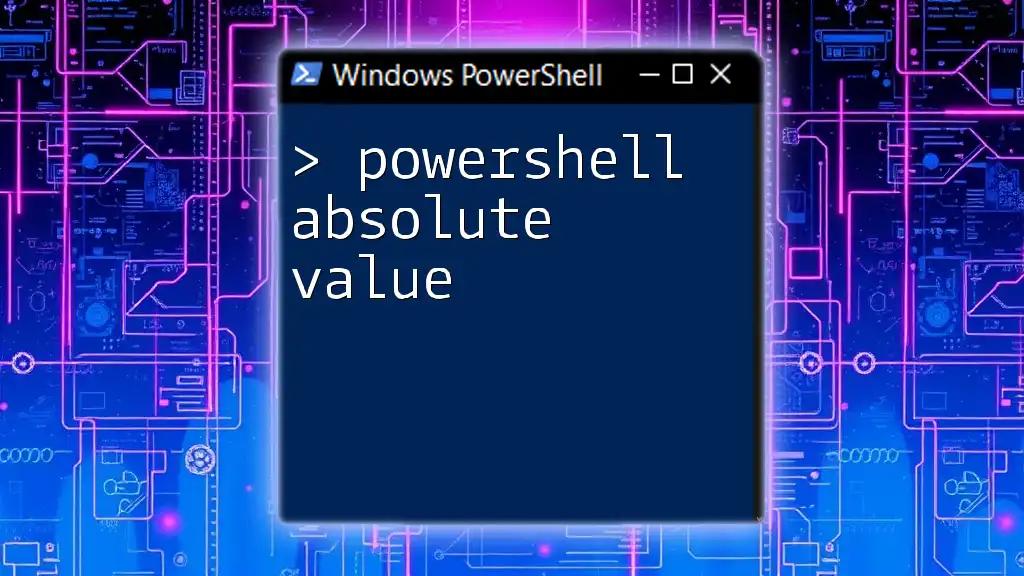
Load Your CSV File
Using Import-Csv Cmdlet
To manipulate data within a CSV file, the first step is to import it into PowerShell. You can do this with the `Import-Csv` cmdlet:
$csvData = Import-Csv -Path 'path\to\yourfile.csv'
This command reads the specified CSV file and converts its content into an array of PowerShell objects, making it easy to manipulate. Ensure you check the CSV headers, as they dictate how the data is represented in the resulting objects.
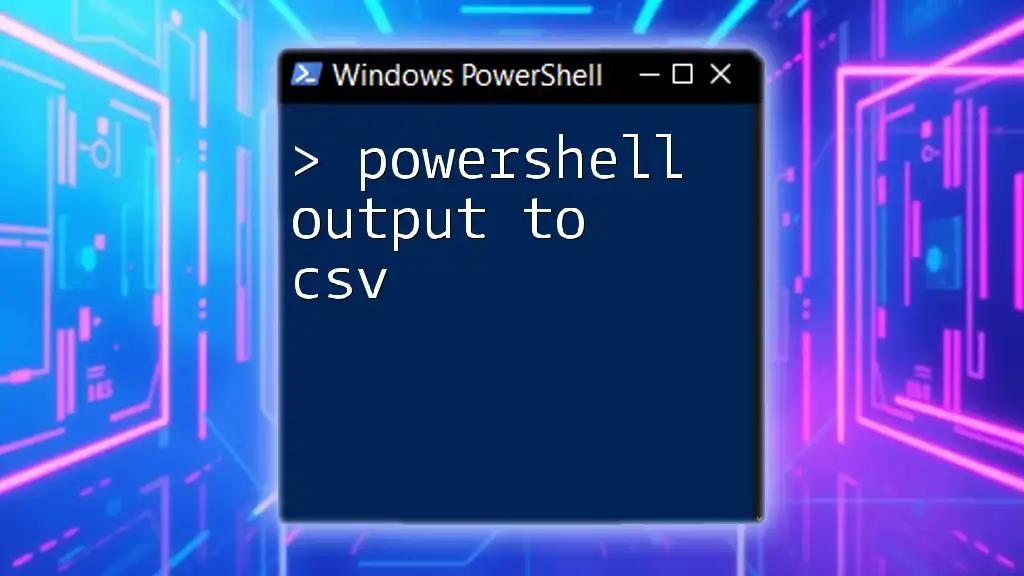
Adding a New Column to CSV
Understanding the Structure of the Data
Before proceeding to add a new column, you should analyze the existing columns in your CSV file. Understanding the data's structure helps ensure that the new column complements your data format and meets your needs, whether for adding new attributes or calculated values.
Creating and Adding a Column
Method 1: Using ForEach-Object
A common way to add a new column is through the `ForEach-Object` cmdlet. You may initialize a new property (or column) in each object as follows:
$csvData | ForEach-Object { $_ | Add-Member -MemberType NoteProperty -Name 'NewColumn' -Value 'YourValue' }
This method iteratively adds the new column named `NewColumn` with a default value of `YourValue` to each row of your imported CSV data. You can customize the value based on your data requirements.
Method 2: Creating a New Object
Alternatively, you can create a new object that includes the existing columns and the new one. This method is particularly useful when you need to add calculated values. Here’s how you can do it:
$newCsv = $csvData | Select-Object *, @{Name='NewColumn'; Expression={'YourValue'}}
In this code, the `Select-Object` cmdlet is utilized to create a new array containing all the original columns, along with the added `NewColumn` populated with the value of `YourValue`. This allows for greater control over the data structure.
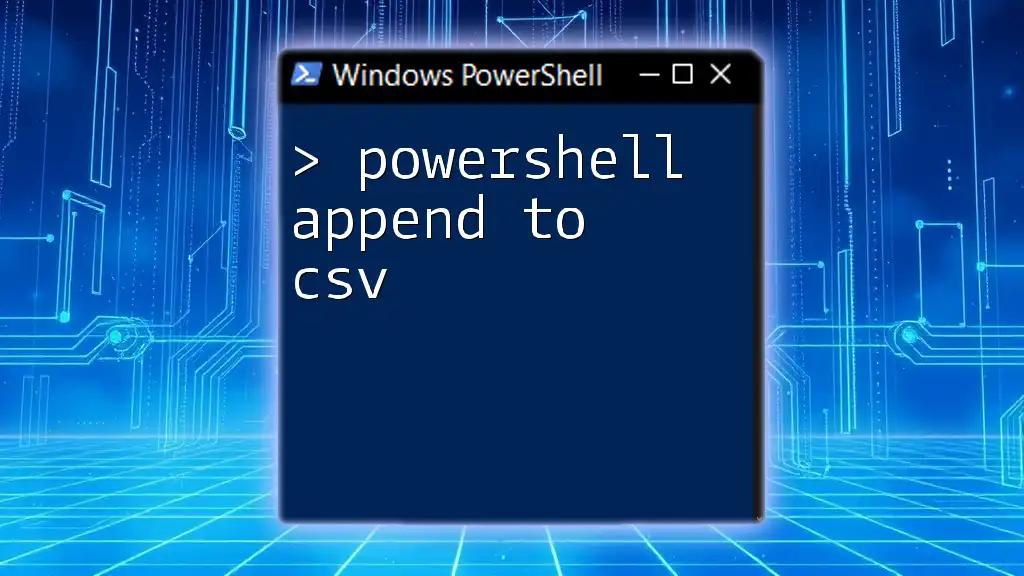
Exporting the Modified CSV
Using Export-Csv Cmdlet
Once you have added the desired columns to your CSV data, the next step is to export the modified data back to a new CSV file. PowerShell’s `Export-Csv` cmdlet makes this straightforward:
$newCsv | Export-Csv -Path 'path\to\outputfile.csv' -NoTypeInformation
In this command, the `-NoTypeInformation` switch is used to omit the type information from the exported CSV, ensuring a clean output. This finalizes the process of modifying your CSV files.
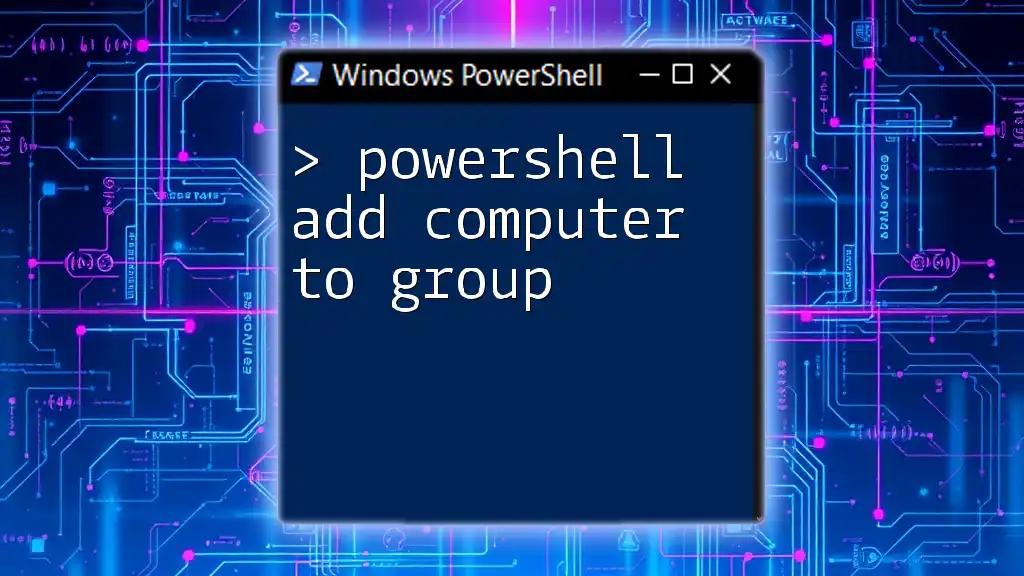
Best Practices for Manipulating CSVs
Error Handling
When working with CSV files in PowerShell, it’s essential to handle errors appropriately. Implementing `Try-Catch` blocks allows you to manage unexpected issues gracefully. For example, you might wrap your importing and exporting code in error-handling mechanisms to troubleshoot if something goes wrong.
Backup Your Original Data
Before beginning any manipulations, it is wise to create a backup copy of the original CSV file. This step can prevent loss of data in case something goes wrong during editing, ensuring that you always have access to the unaltered version.
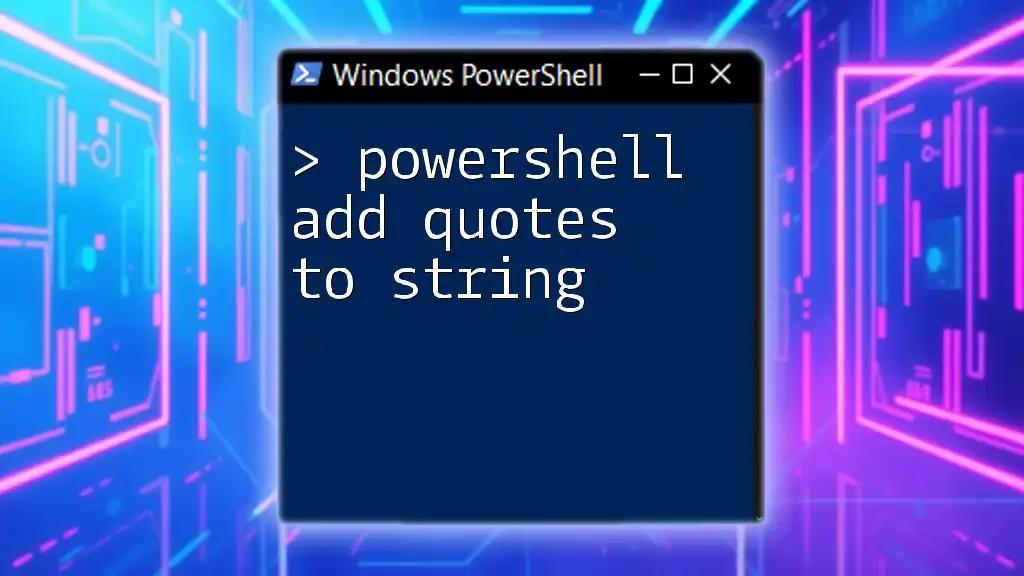
Conclusion
In this comprehensive guide, we covered the essential steps for using PowerShell to add a column to a CSV file. From importing CSV data to adding columns and exporting the modified data, PowerShell offers an efficient solution for data manipulation.
As you dive deeper into PowerShell, you’ll find that it provides numerous opportunities for advanced data management and automation. To enhance your skills, continue exploring more PowerShell commands and consider subscribing for additional tutorials and tips tailored to help you use PowerShell effectively.
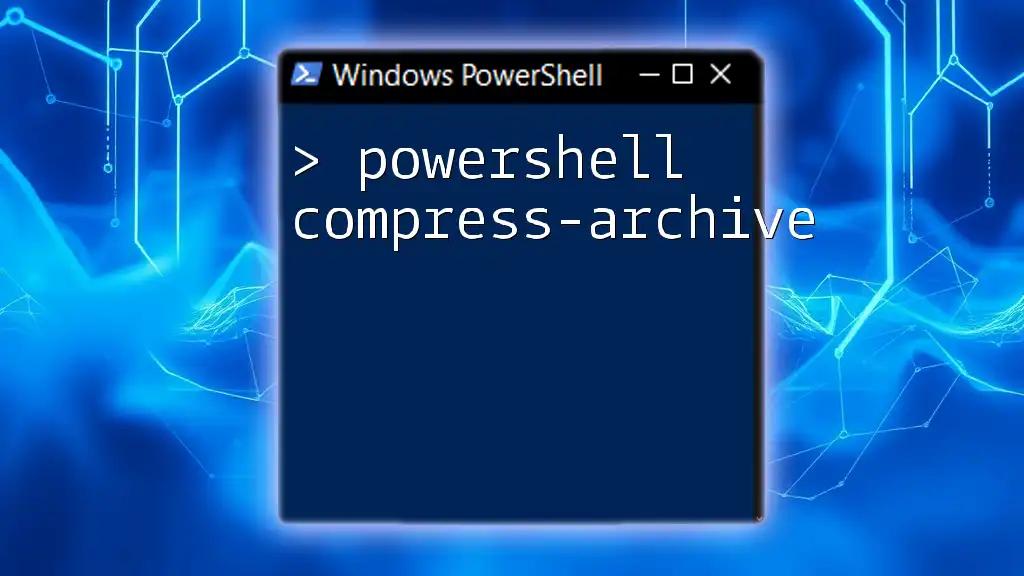
Additional Resources
For further assistance, you can refer to the official PowerShell documentation, which provides in-depth information on all cmdlets. Participating in community forums can also offer practical insights, sharing knowledge with fellow users interested in PowerShell.
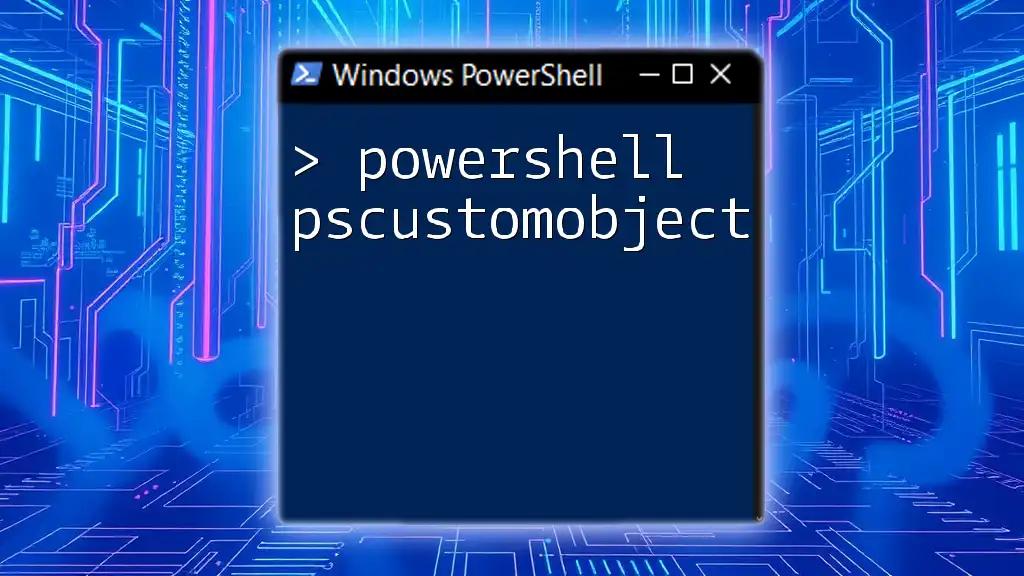
FAQs
How do I know if my CSV has headers?
You can inspect the first line of your imported CSV data. If the header row does not appear in the expected fields, you may need to address formatting issues before proceeding with column additions.
Can I add multiple columns at once?
Yes, you can extend the previous methods to include several new columns. You would simply replicate the addition process for each new column to achieve your desired structure.
What if I need to add calculated values to my new column?
You can use an expression within the `Select-Object` method to calculate values dynamically based on existing fields in your CSV data. For instance, you could use a calculation like `$_.ExistingField * 2` to populate your new column with calculated values based on another field.