To run a PowerShell command, simply open the PowerShell application and type the command you wish to execute, followed by pressing Enter; for example:
Write-Host 'Hello, World!'
Understanding PowerShell Basics
What is PowerShell?
PowerShell is a powerful command-line shell and scripting language designed for automation and configuration management. It allows users to execute commands and automate tasks by leveraging a vast ecosystem of cmdlets. These cmdlets follow a consistent naming convention and utilize a modular approach to provide a wide range of functionalities.
PowerShell Versions
PowerShell has evolved over the years, with notable versions being Windows PowerShell and PowerShell Core. Windows PowerShell is built on the .NET Framework, while PowerShell Core is cross-platform and built on .NET Core, allowing it to run on Windows, macOS, and Linux.
To check your PowerShell version, use the following command:
$PSVersionTable.PSVersion
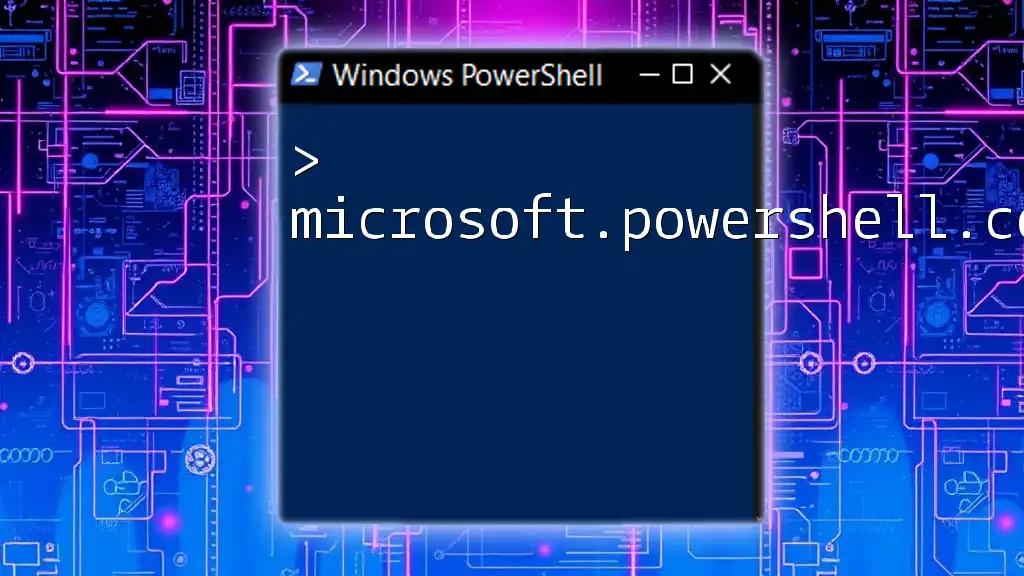
Preparing to Run PowerShell Commands
Opening PowerShell
You have several options to open PowerShell:
- Using Windows Search: Click on the Start Menu and type PowerShell.
- Using Run Dialog: Press `Win + R`, type powershell, and hit Enter.
- Using Command Prompt: Launch Command Prompt and type powershell to switch to PowerShell.
Understanding the Environment
PowerShell Integrated Scripting Environment (ISE) and Windows Terminal are two popular interfaces for running PowerShell commands. While ISE provides a rich scripting experience, Windows Terminal allows for a more versatile command-line experience and supports multiple tabs and shells.
Before diving deeper, ensure your environment is set up properly. Adjust the execution policy to allow scripts to run with:
Set-ExecutionPolicy RemoteSigned
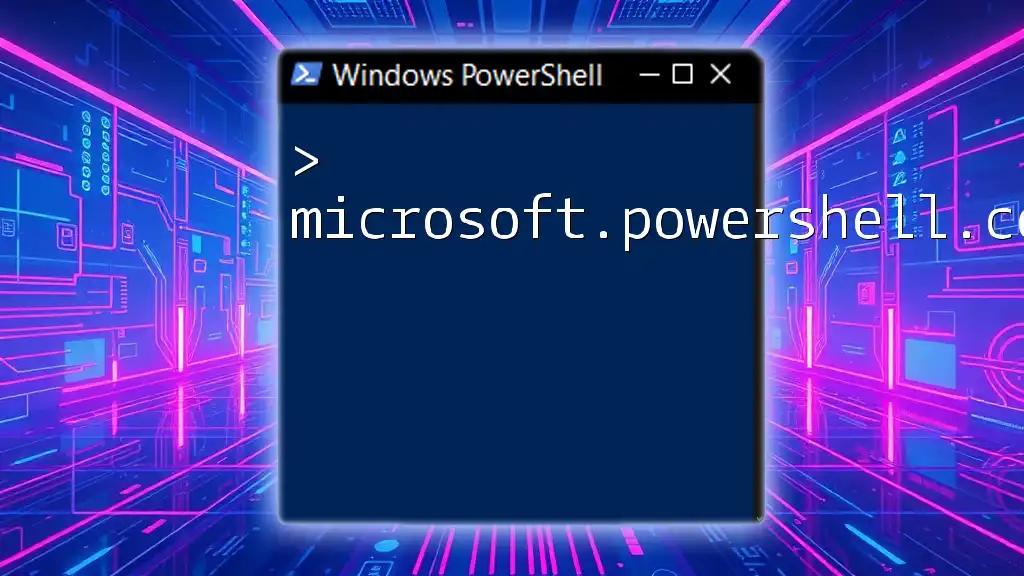
How to Run a Basic PowerShell Command
Syntax and Structure of a Cmdlet
PowerShell cmdlets follow a Verb-Noun structure, such as `Get-Process`. This syntax makes it easy to understand the action being performed.
Running a Simple Cmdlet
To get a list of all running processes on your system, simply run:
Get-Process
This command displays a list of all active processes, along with details like their IDs, CPU usage, and memory footprint. It's a simple yet powerful tool for system monitoring.
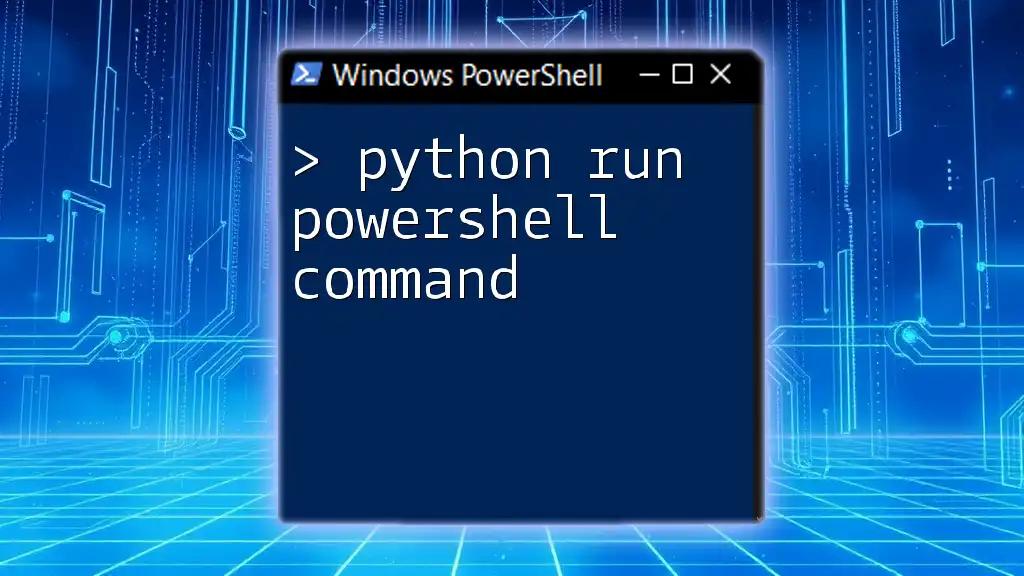
Using Parameters with Cmdlets
What are Parameters?
Parameters modify the behavior of cmdlets. They allow you to specify what data you want or how you want the cmdlet to operate.
How to Use Command Parameters
For instance, if you're interested in a specific process named "powershell," you can run:
Get-Process -Name "powershell"
This command fetches only the process matching the specified name, showcasing the power of parameters in narrowing down results.
Positional vs. Named Parameters
Positional parameters are provided in sequence without specifying parameter names, while named parameters require you to use the parameter name explicitly. For example:
Get-Process "powershell" # Positional parameter
Get-Process -Name "powershell" # Named parameter
Both commands yield the same result, but named parameters enhance clarity.
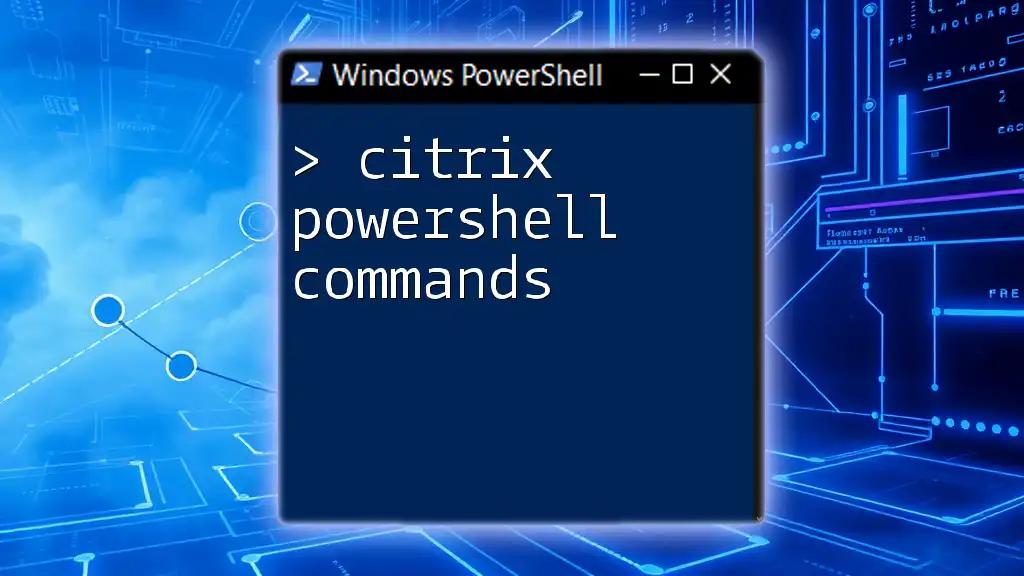
Utilizing Command Output
Understanding Output Objects
PowerShell cmdlets output objects, which means that the data returned can be complex structures, allowing for further manipulation and processing.
Formatting Output
To improve readability, you can format the output using cmdlets like `Format-Table` or `Format-List`. For example, to display basic properties of running processes in a table format, use:
Get-Process | Format-Table -Property Name, Id, CPU
This command presents the essential information clearly, making it easier to analyze process details at a glance.
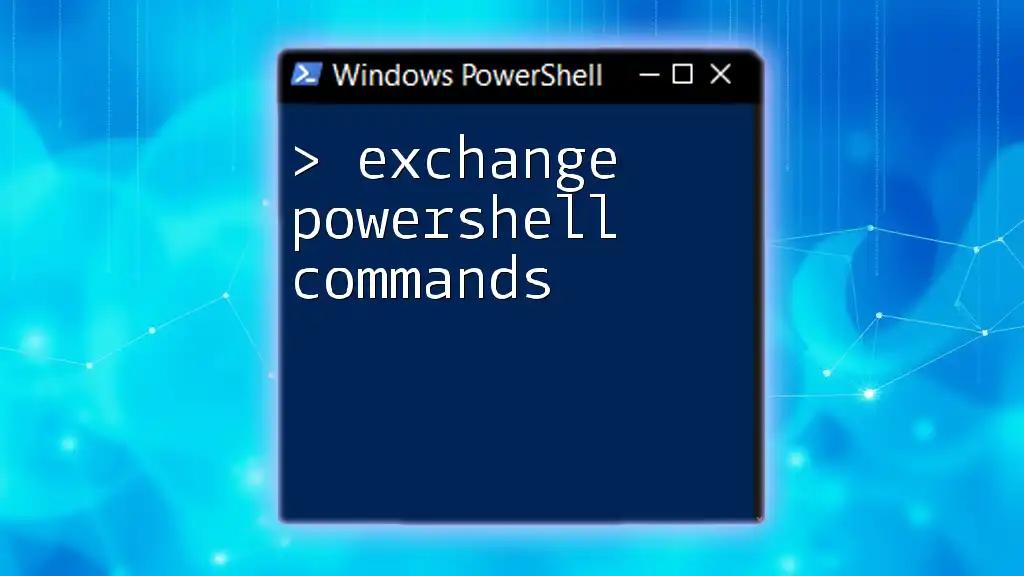
Running Scripts and Advanced Commands
Introduction to Scripting
PowerShell scripts, saved as `.ps1` files, allow for the automation of tasks across multiple cmdlets and commands. Writing scripts increases your productivity by executing a series of commands with a single call.
Creating and Running a Script
Creating a script file is straightforward. Open any text editor, write your commands, and save the file with a `.ps1` extension. For example:
# This script lists all running processes
Get-Process | Format-Table -Property Name, Id, CPU
To run your script, navigate to its directory in PowerShell and execute it:
.\scriptname.ps1
Advanced Command Techniques
As you become more comfortable with PowerShell, you can explore advanced commands such as pipelines, loops, and conditional statements, greatly enhancing your scripting ability.
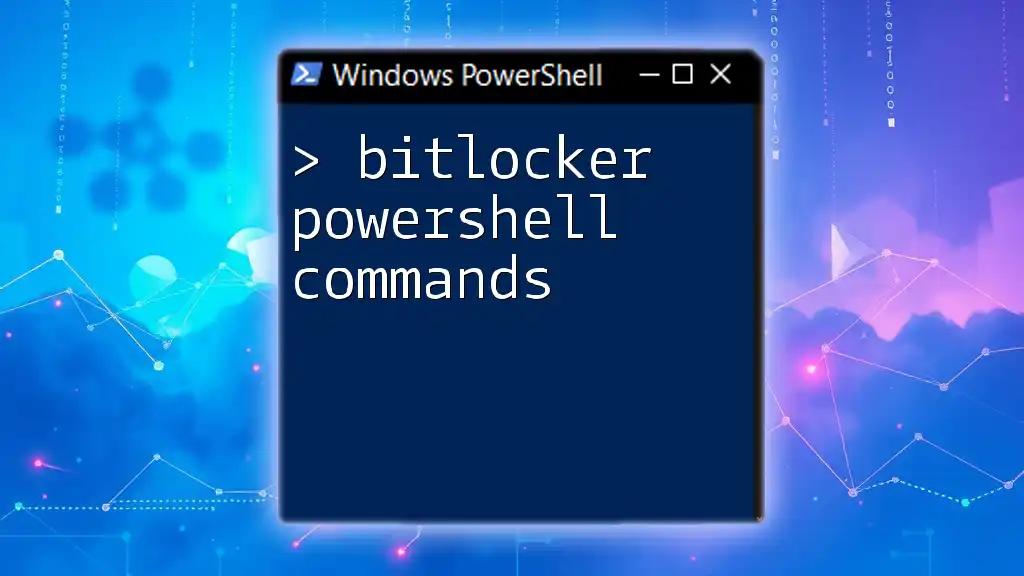
Common Errors and Troubleshooting
Common Mistakes
Encountering common errors is part of the learning process. For example, if you see an "Execution Policies" error, it indicates that your current policy doesn't allow script execution. Adjust this using the command mentioned earlier.
Debugging PowerShell Commands
Debugging scripts is vital for resolving issues. Utilize `Write-Debug` to print debug messages in your scripts, guiding you where necessary.
Tips for Effective Troubleshooting
The PowerShell help system is indispensable. Use `Get-Help` followed by the cmdlet name to access documentation and usage examples. For instance:
Get-Help Get-Process
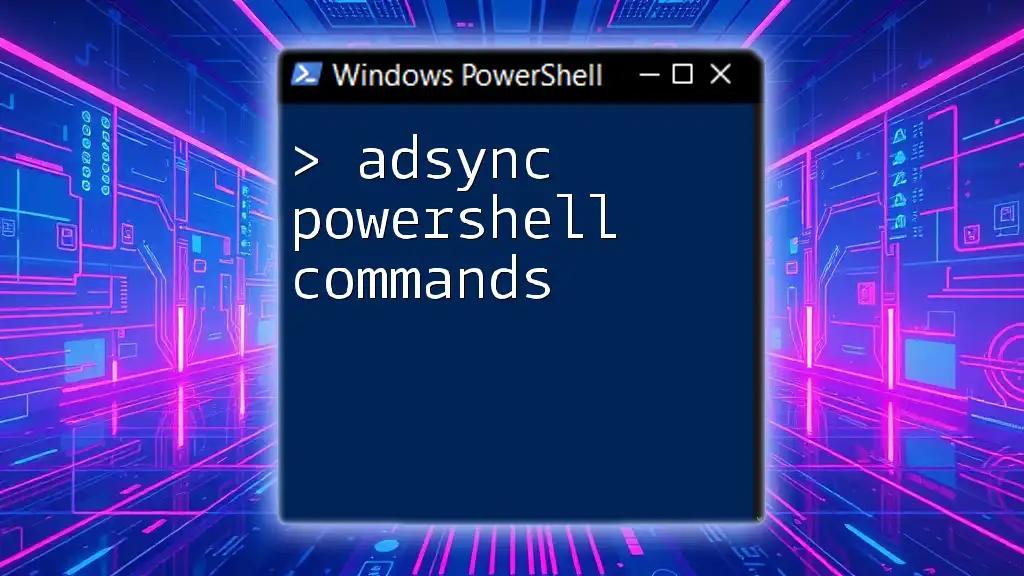
Conclusion
Mastering how to run a PowerShell command opens up a world of automation and efficiency. By understanding the basics, utilizing parameters effectively, and exploring scripts, you position yourself as a skilled user ready to tackle more complex tasks.
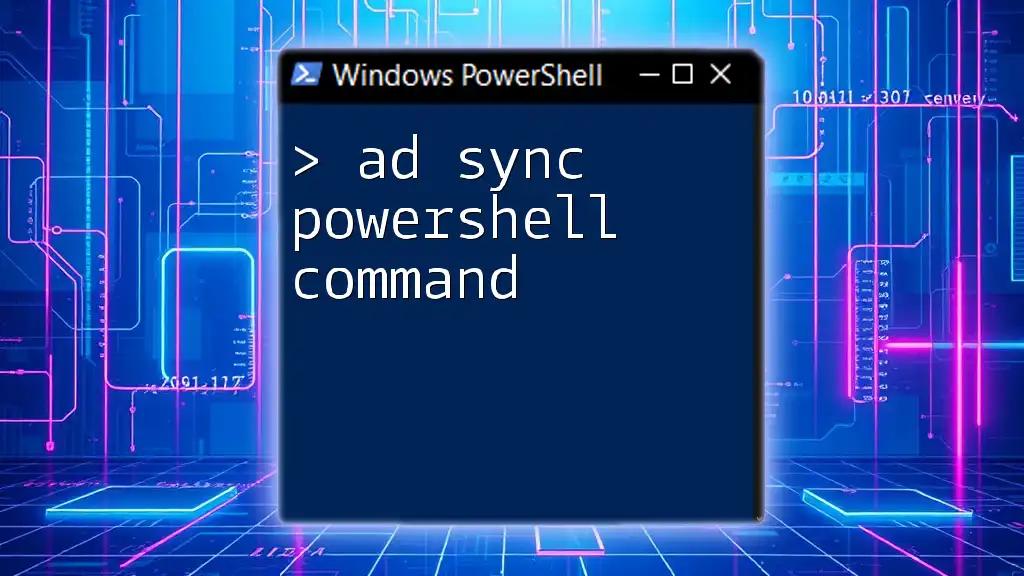
Call to Action
Don't miss out on our upcoming workshops where you can deepen your PowerShell knowledge and skills. Join us to become proficient in using PowerShell commands efficiently!
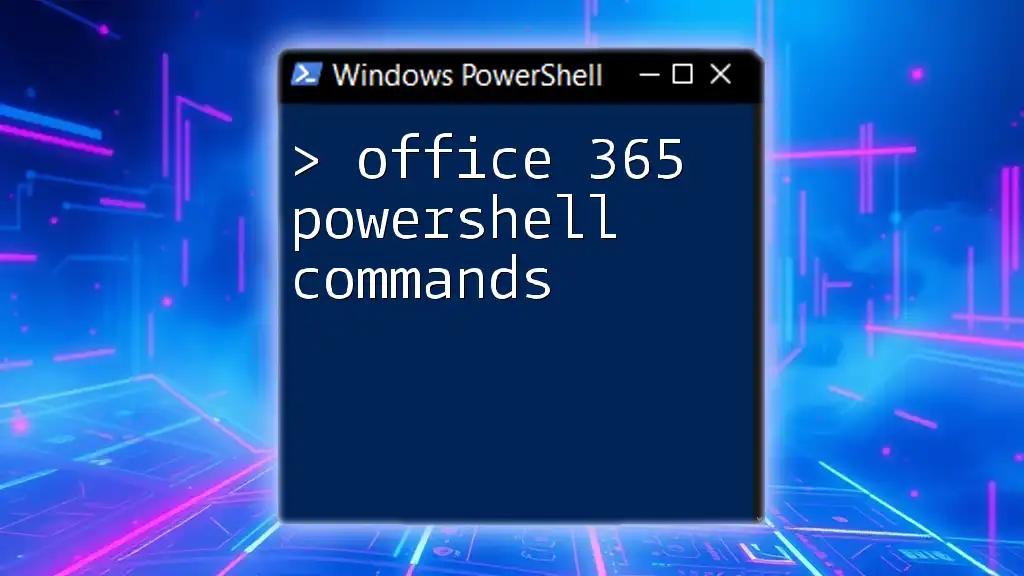
Additional Resources
For further learning, check out the official Microsoft documentation, community forums, and tutorials. Keep a handy reference of common cmdlets to enhance your PowerShell journey.