To execute a PowerShell command from Python, you can utilize the `subprocess` module to call the PowerShell executable along with your desired command.
import subprocess
subprocess.run(["powershell", "-Command", "Write-Host 'Hello, World!'"])
Understanding PowerShell
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed for system administration and automation. Essentially, it allows you to automate tasks across devices and applications in a Windows environment. Its built-in cmdlets (pronounced "command-lets") simplify the management of system resources.
Use Cases for PowerShell
PowerShell excels in various areas, particularly:
- System Administration Tasks: Managing user accounts, services, and processes.
- Automating Repetitive Tasks: Writing scripts to perform backups, monitoring system health, or configuring settings.
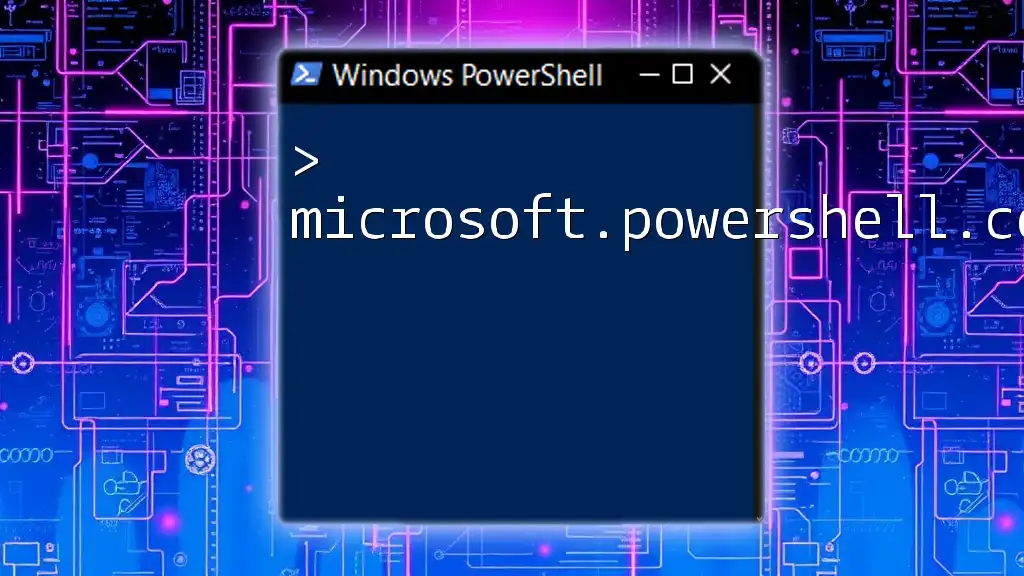
Introduction to Python
What is Python?
Python is a high-level, versatile programming language known for its readability and ease of use. It has gained immense popularity due to its vast ecosystem of libraries and frameworks, making it a go-to choice for developers worldwide.
How Python Can Interact with System Shells
Python has built-in support for interacting with system shell commands. The `subprocess` and `os` modules are particularly useful for this purpose, opening doors to execute shell commands, including those written in PowerShell.
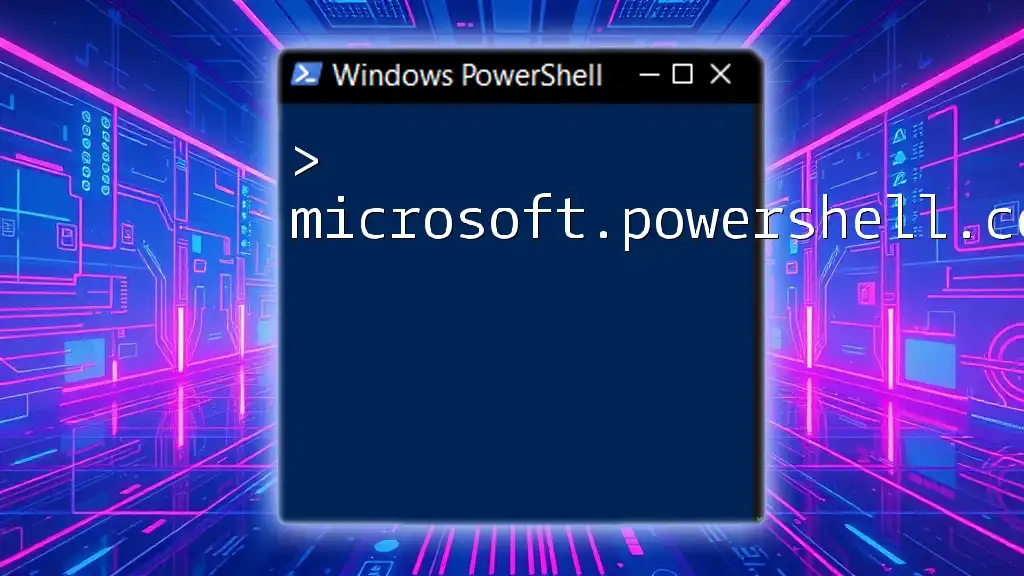
Preparing Your Environment
Setting Up Python
Before executing any code, it's vital to have Python installed on your system. You can download the latest version from the [official Python website](https://www.python.org/downloads/). Follow the installation instructions provided for your operating system.
Once installed, verify by running:
python --version
Ensuring PowerShell Accessibility
PowerShell comes pre-installed on most Windows systems. You can launch it by typing "PowerShell" in the Start menu. To ensure it's accessible from Python, simply check that you can run it manually without any permission issues.
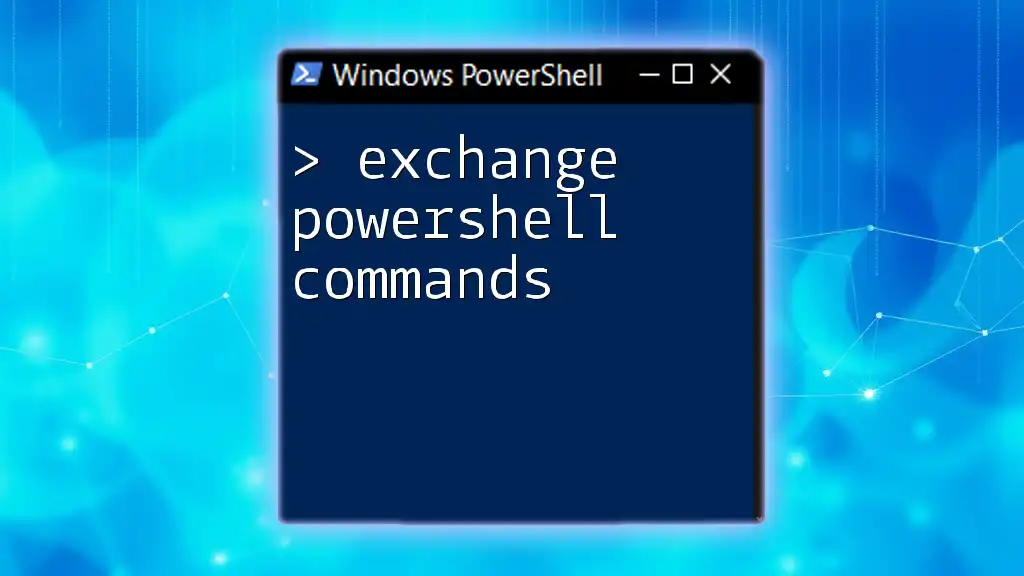
Running PowerShell Commands from Python
Using the Subprocess Module
The `subprocess` module enables the execution of system commands. When you want to run a PowerShell command from Python, this is the way to go.
Basic Example: Running a PowerShell Command
Here's a straightforward example demonstrating how to execute a basic PowerShell command to get a list of processes:
import subprocess
command = "Get-Process"
result = subprocess.run(["powershell", "-Command", command], capture_output=True, text=True)
print(result.stdout)
This code does the following:
- Imports the `subprocess` module.
- Sets the PowerShell command (`Get-Process`), which retrieves a list of running processes.
- Uses `subprocess.run()` to execute the command, capturing both output and errors.
Capturing and Handling Output
Once you successfully run a command, you can handle outputs and errors. Here's how:
if result.returncode == 0:
print("Success:", result.stdout)
else:
print("Error:", result.stderr)
- The `returncode` attribute indicates whether the command executed successfully (0) or encountered an error (anything else).
- Capture and print either the success message or the error message for debugging.
Passing Arguments to PowerShell Commands
You can also pass parameters to your PowerShell commands directly from Python. Here's an example that retrieves information on a specific process:
param_value = "YourProcessName"
command = f"Get-Process -Name {param_value}"
result = subprocess.run(["powershell", "-Command", command], capture_output=True, text=True)
In this code:
- `YourProcessName` should be replaced with the actual name of a running process.
- The command constructs a dynamic PowerShell command that retrieves information about the specified process, showcasing how Python can make your commands flexible.
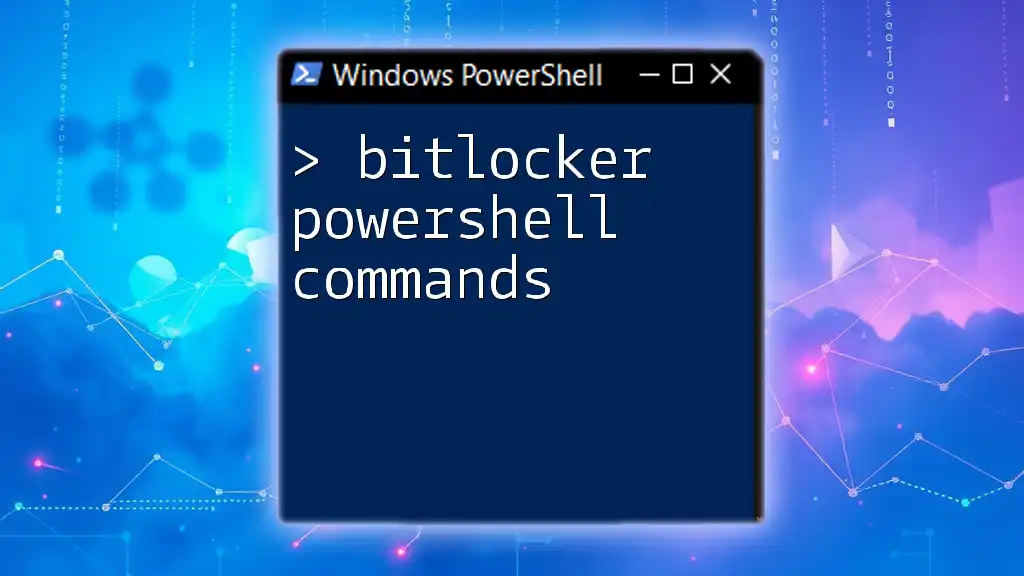
Advanced Techniques
Running PowerShell Scripts from Python
You may also want to execute entire PowerShell scripts instead of single commands. This can be accomplished with the `-File` parameter. Here’s how:
script_path = "C:\\Scripts\\MyScript.ps1"
result = subprocess.run(["powershell", "-File", script_path], capture_output=True, text=True)
In this example:
- Make sure to replace `C:\\Scripts\\MyScript.ps1` with your actual script path.
- This command allows you to execute complex logic encapsulated in a script file.
Using PowerShell with Python Libraries
You can enhance your interaction by leveraging third-party libraries like `pywin32`. Here’s a small demonstration of how to use it:
import win32com.client
shell = win32com.client.Dispatch("WScript.Shell")
shell.Run("powershell.exe Get-Process")
This code snippet provides:
- An alternative way to run a PowerShell command by interacting with the Windows Scripting Host.
- It exemplifies how Python can manage administrative tasks through PowerShell without having to rely solely on command-line interfaces.
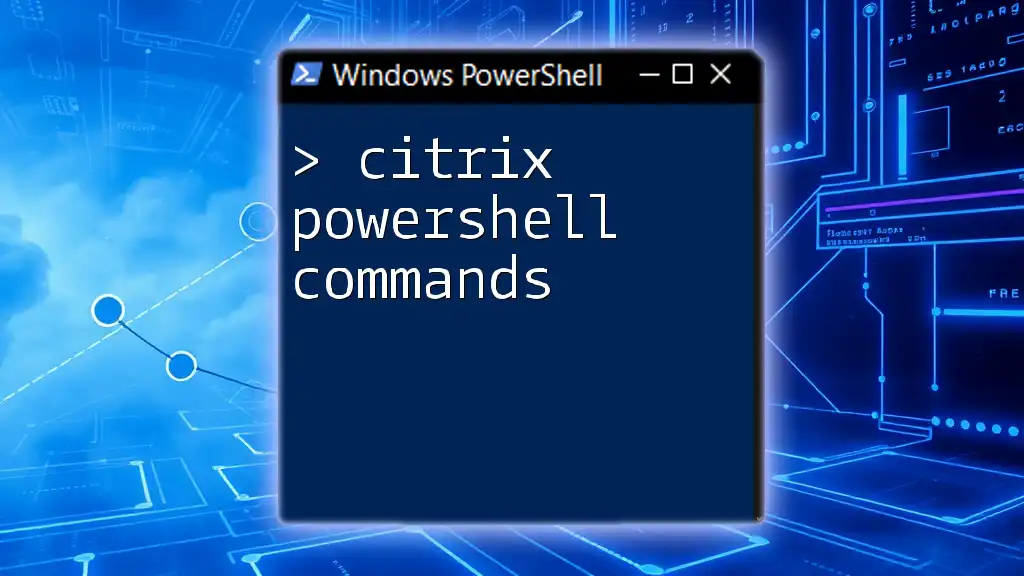
Troubleshooting Common Issues
As with any scripting and automation, you may encounter errors while executing PowerShell commands from Python. Here are a few common issues:
- Access Denied: Running PowerShell commands might require administrative privileges. If you face this issue, ensure that you run your Python script in an elevated command prompt.
- Execution Policy Errors: If PowerShell’s execution policy is too restrictive, it may prevent script execution. To resolve this, you may need to set the policy to allow scripts using:
Set-ExecutionPolicy RemoteSigned
Always double-check script permissions and user rights when encountering such errors.
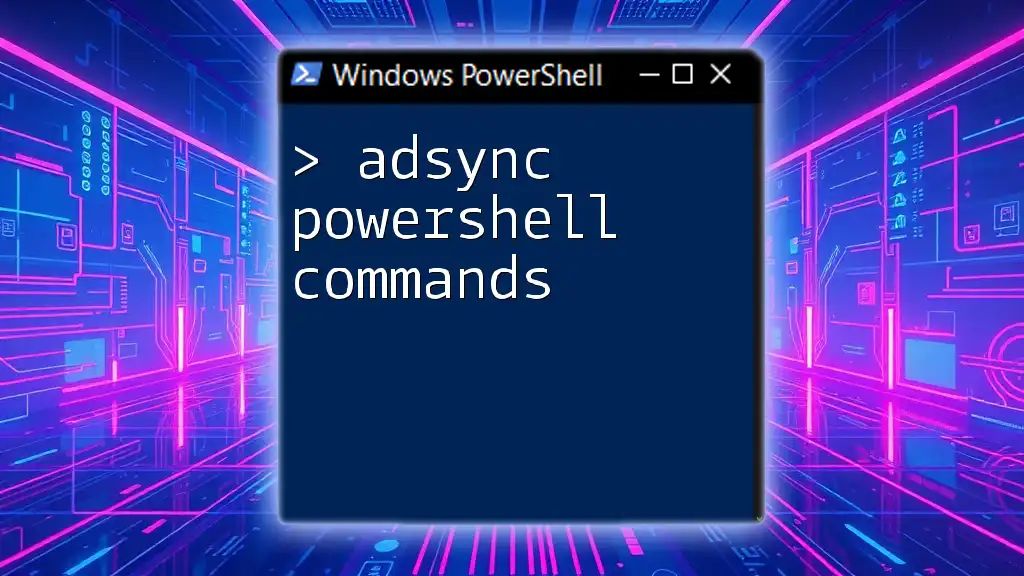
Conclusion
Throughout this guide, we explored how to efficiently and effectively run PowerShell commands from Python using the `subprocess` module and other libraries. By integrating Python and PowerShell, you can significantly enhance your scripting capabilities and automate repetitive tasks.
Whether you're a seasoned developer or just beginning your journey into automation, understanding how to bridge these two powerful tools opens a world of possibilities. Feel free to dive deeper into this integration, and don't hesitate to explore more resources to bolster your knowledge!