To edit a file in PowerShell, you can use the `Set-Content` cmdlet to overwrite the file's contents or the `Add-Content` cmdlet to append data to it.
Here's an example of using `Set-Content`:
Set-Content -Path 'C:\path\to\your\file.txt' -Value 'This is the new content of the file.'
Understanding PowerShell and File Editing
What is PowerShell?
PowerShell is a powerful command-line interface and scripting language designed for system administration and automation. Unlike traditional command-line interfaces, PowerShell integrates with .NET, allowing it to interact seamlessly with system objects, making it a versatile tool for managing files and configurations.
Key Concepts in File Editing
When dealing with file editing in PowerShell, it's essential to understand what types of files you might typically encounter. Common file types include:
- Text files (.txt): Simple files containing plain text.
- Comma-Separated Values (.csv): Often used for data import/export.
- JSON (.json): Commonly used for configuration files in modern applications.
Understanding file encoding is also crucial. Different types of files may use different encoding formats, such as UTF-8 or ASCII. This can influence how data is read from or written to files.
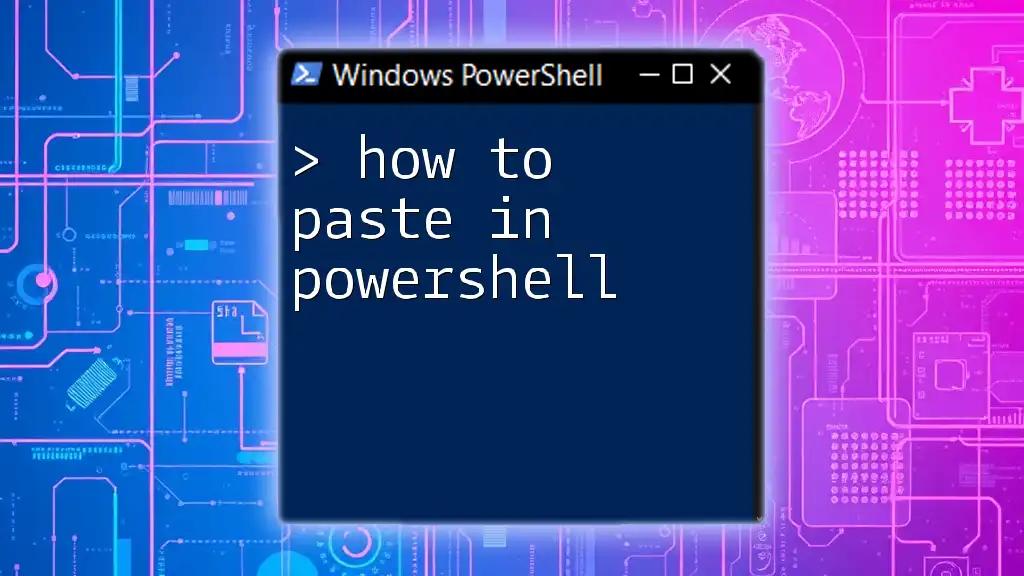
Opening a File for Editing in PowerShell
Using `Get-Content` to View File Content
Before making any edits, you often need to view the file's current state. The `Get-Content` cmdlet allows you to accomplish this easily. For example:
Get-Content -Path "C:\example\file.txt"
This command will display the contents of `file.txt` in the console. You can specify the full path where the file is located, and PowerShell will read the information line by line.
Editing Files with Out-File
Once you know the content, you might want to overwrite it. The `Out-File` cmdlet allows you to create a new file or replace an existing one with the new data. Here’s how you can use it:
"New content" | Out-File -FilePath "C:\example\file.txt" -Encoding UTF8
In this example, `Out-File` writes the string "New content" to `file.txt`. By using the `-Encoding` parameter, you can specify the encoding format, such as UTF8, ensuring that special characters are handled correctly.
Explanation of Overwriting vs. Appending
Using `Out-File` by default will overwrite any existing content in the file. If you want to add additional information instead of replacing it, consider using the `-Append` flag:
"Additional content" | Out-File -FilePath "C:\example\file.txt" -Append
This will append "Additional content" to whatever is already in `file.txt`.
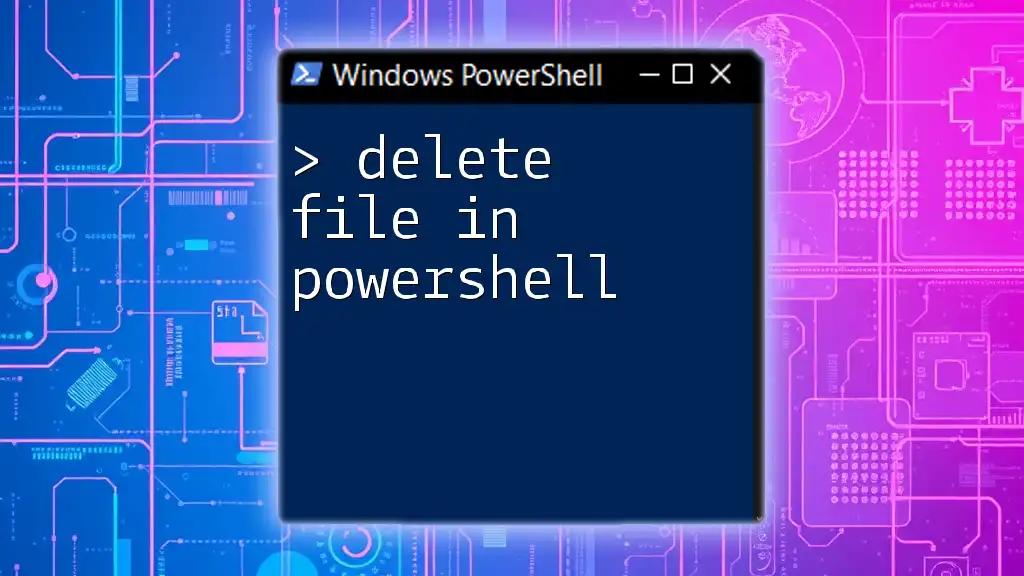
Editing Files In-Place
Using `Set-Content` to Modify File Content
To change existing content directly in a file, use the `Set-Content` cmdlet. For example:
Set-Content -Path "C:\example\file.txt" -Value "Updated content"
With this command, the entire content of `file.txt` will be replaced with "Updated content". It's handy for quickly changing text.
Using `Add-Content` to Append Data
If your goal is to add rather than replace, the `Add-Content` cmdlet is perfect. It allows you to insert new lines or text seamlessly. For instance:
Add-Content -Path "C:\example\file.txt" -Value "This line is appended."
This command will add "This line is appended." to the end of `file.txt`, preserving all previous information.
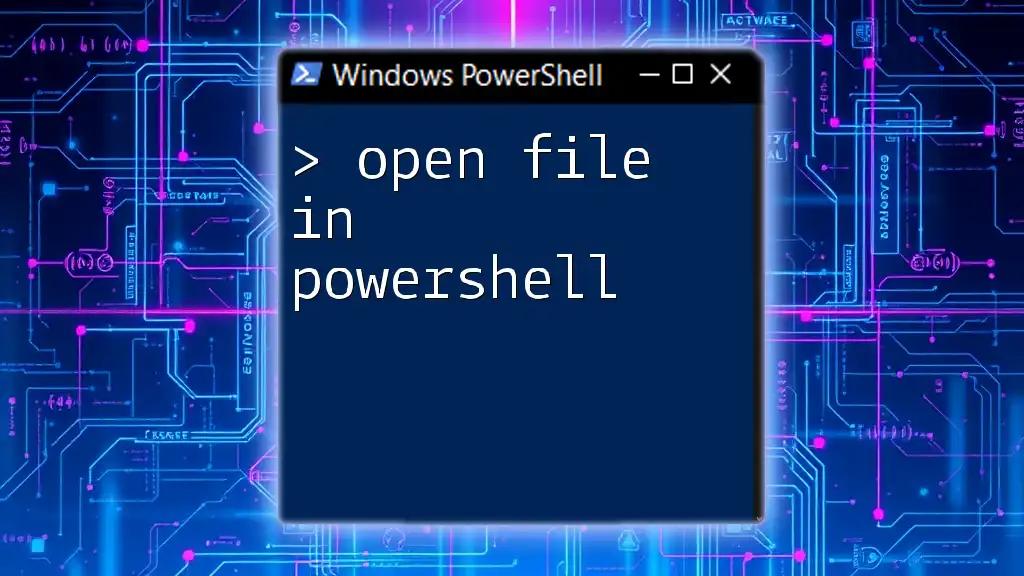
More Advanced Editing Techniques
Regular Expressions for Complex Replacements
When you need to perform more complex text manipulations, Regular Expressions (Regex) come into play. Here's a brief example of how to replace occurrences of a string within a file:
(Get-Content -Path "C:\example\file.txt") -replace 'old', 'new' | Set-Content -Path "C:\example\file.txt"
In this scenario, `Get-Content` retrieves the file's contents, the `-replace` operator modifies it by substituting "old" with "new," and finally, `Set-Content` writes the changes back to the file. This method allows you to replace multiple occurrences easily.
Using Built-in PowerShell Cmdlets for File Editing
PowerShell provides a range of cmdlets that enhance your file editing capabilities. For example, the `Select-String` cmdlet helps you search for specific patterns within text files. Here’s how to use it:
Select-String -Path "C:\example\file.txt" -Pattern "search term"
This command searches for "search term" in `file.txt` and returns the line(s) containing it. This is invaluable when you are dealing with large files.
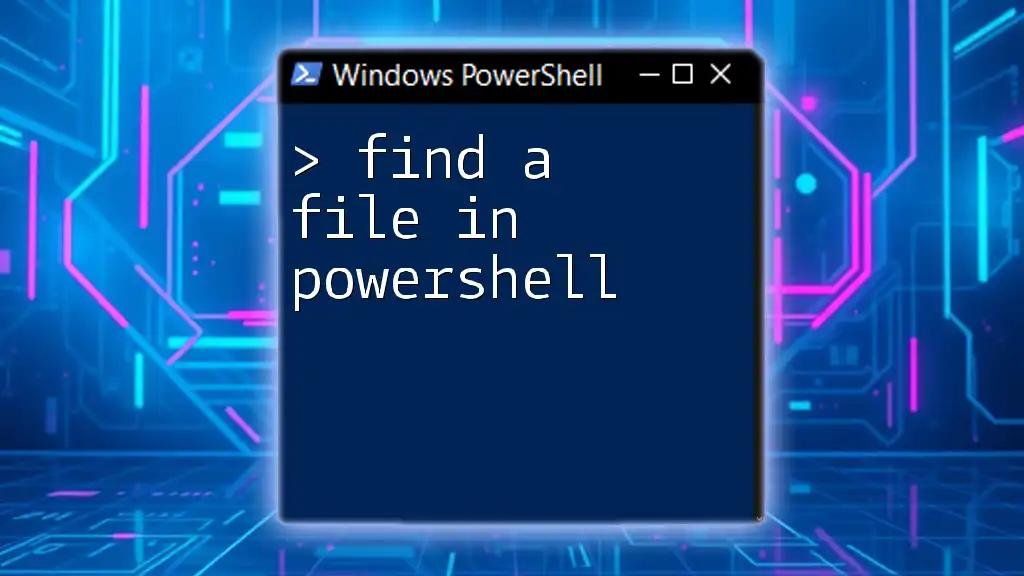
Practical Scenarios for File Editing
Editing Configuration Files
A common scenario involves editing configuration files essential for applications. For instance, if you need to change a setting in a JSON file, you might do something like this:
(Get-Content -Path "C:\example\config.json") -replace '"oldSetting": true', '"oldSetting": false' | Set-Content -Path "C:\example\config.json"
This command would change a setting in `config.json` from `true` to `false`, which can be crucial for performance tuning or feature enabling.
Batch Editing Multiple Files
PowerShell shines when it comes to bulk operations. If you need to update multiple files in a directory, you can employ a loop:
Get-ChildItem -Path "C:\example\*.txt" | ForEach-Object {
(Get-Content -Path $_.FullName) -replace 'old', 'new' | Set-Content -Path $_.FullName
}
This script finds all `.txt` files in the specified folder, replaces "old" with "new" in each, and saves the changes.
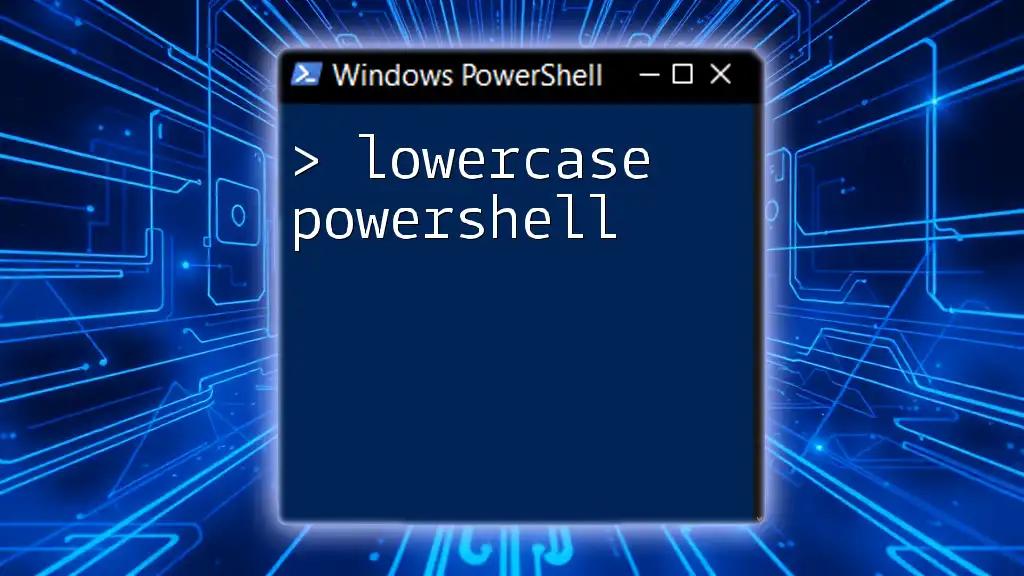
Best Practices for Editing Files in PowerShell
When editing files in PowerShell, it’s essential to follow a few best practices for a smooth experience:
-
Always Backup Files Before Editing: Before making changes, ensure you have a copy of the original file. This provides a safety net in case things go wrong.
-
Use Version Control Systems: For larger projects, using tools like Git for version tracking can save time and help manage revisions effectively.
-
Test Changes in a Controlled Environment: If possible, test scripts on non-critical systems before deploying to live environments. This minimizes the risk of unintended consequences.
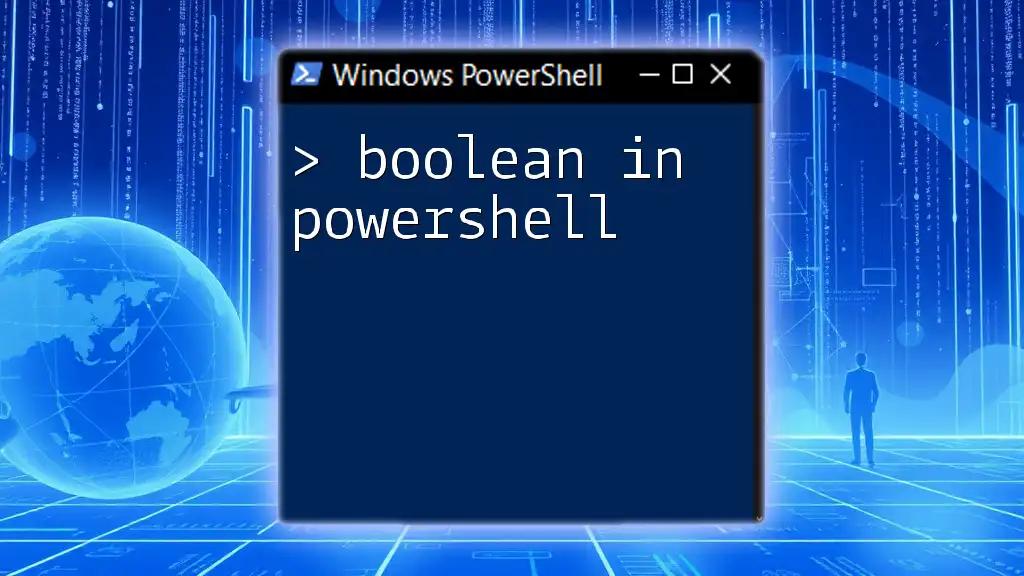
Troubleshooting Common Issues
Permissions Issues
Sometimes, you may encounter permission errors when trying to edit a file. This often occurs if your user account does not have the required rights to modify the file. In such cases, consider running PowerShell as an administrator or adjusting the file permissions via the properties window.
PowerShell Errors during File Editing
Understanding PowerShell error messages is crucial for troubleshooting. Common errors might indicate problems like file access issues or incorrect command syntax. Always read the error message carefully; they can guide you to the underlying issue and help you find a solution.

Conclusion
Knowing how to edit a file in PowerShell is an invaluable skill that can streamline your workflow and enhance your productivity. By familiarizing yourself with various cmdlets and methods for file manipulation, you empower yourself to manage configurations, automate processes, and manipulate data efficiently.
As you continue to explore PowerShell, remember that practice is key. The more you work with these commands, the more proficient you'll become. Consider experimenting with different files and scenarios to deepen your understanding and build your confidence!
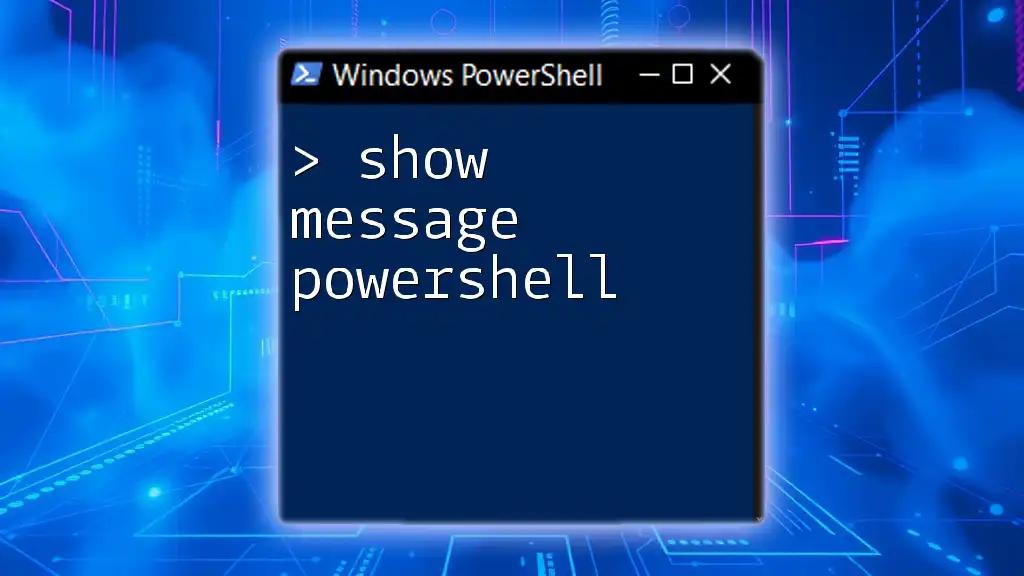
Call to Action
We encourage you to share your experiences with file editing in PowerShell or any tips you've found useful. Additionally, consider signing up for our upcoming courses to continue expanding your PowerShell skills!
Appendix
For further enlightenment, check out additional cmdlets related to file management and save valuable time on your administrative tasks. A wealth of resources is available online and in community forums to aid your learning journey. Happy scripting!