To comment in PowerShell, use the `#` symbol for single-line comments or `<#` and `#>` for multi-line comments.
# This is a single-line comment
Write-Host 'Hello, World!'
<#
This is a
multi-line comment
#>
Write-Host 'Hello again!'
What is Commenting in PowerShell?
Commenting is a fundamental practice in programming that allows developers to embed explanatory notes within their code. These notes do not affect how the code runs, but they serve as valuable context for anyone reading or maintaining the code later. In the context of PowerShell, commenting helps clarify the purpose and functionality of scripts, improving readability and maintainability.
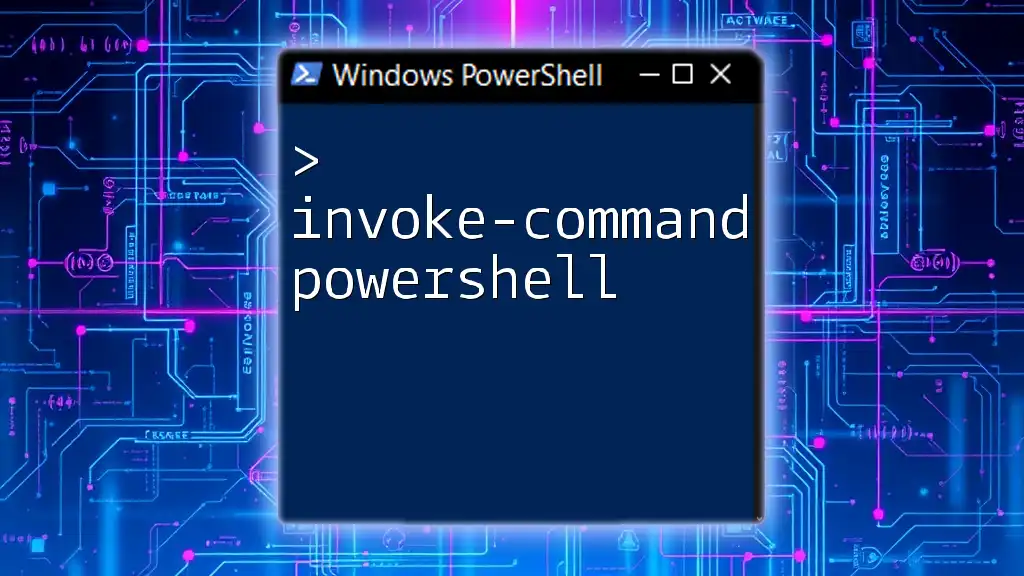
Types of Comments in PowerShell
Single-Line Comments
In PowerShell, you can write single-line comments using the hash symbol (`#`). Anything following the `#` on that line will be ignored by the PowerShell engine. This is suitable for brief notes or reminders without taking up too much space.
Example:
# This is a single-line comment
Write-Output "Hello, World!" # This prints a message to the console
Single-line comments are particularly useful for quick annotations next to relevant lines of code. They can help clarify what a particular line does without overwhelming the reader with too much information.
Multi-Line Comments
For longer explanations, use multi-line comments that begin with `<#` and end with `#>`. This allows you to span comments over multiple lines, making it ideal for more detailed descriptions or instructions.
Example:
<#
This is a multi-line comment.
It can span multiple lines.
#>
Write-Output "Hello, World!"
Multi-line comments can be especially handy when you want to document an entire section of code or explain a complex series of commands without cluttering individual lines with comments.
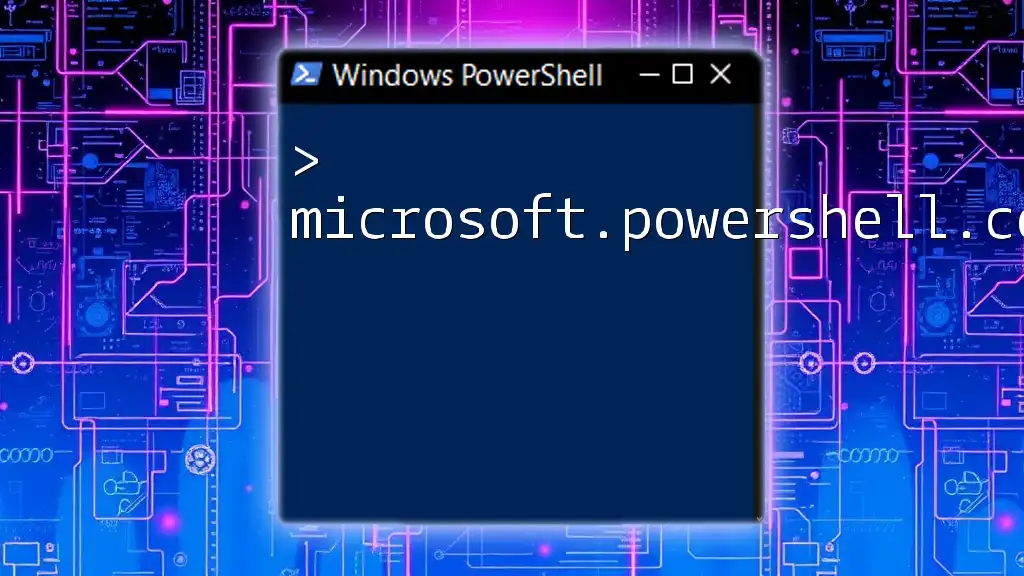
Best Practices for Commenting PowerShell Scripts
Writing Clear and Concise Comments
When commenting, clarity is paramount. Comments should convey information simply and directly. Avoid jargon or overly complex language that might confuse the reader. Instead, strive for straightforward, actionable comments that anyone can understand.
Keeping Comments Relevant
It's crucial to keep comments in sync with the code they describe. As code evolves, so should the comments. Forgetting to update comments after modifying code can lead to confusion and errors.
Example:
# Updated the output section to reflect new requirements
Write-Output "Updated Hello, World!"
In this example, the comment informs the reader that a change has been made, underscoring the importance of commenting relevance through continuous synchronization.
Using Comments to Enhance Code Readability
Organizing your code with comments can significantly enhance its readability. Effective code segmentation helps other developers (or future you) comprehend the structure of your script.
Example:
# Initialization
$name = "User"
# Output
Write-Output "Hello, $name!"
Here, comments delineate logical sections of the script, making it easier to follow the thought process behind the coding structure.
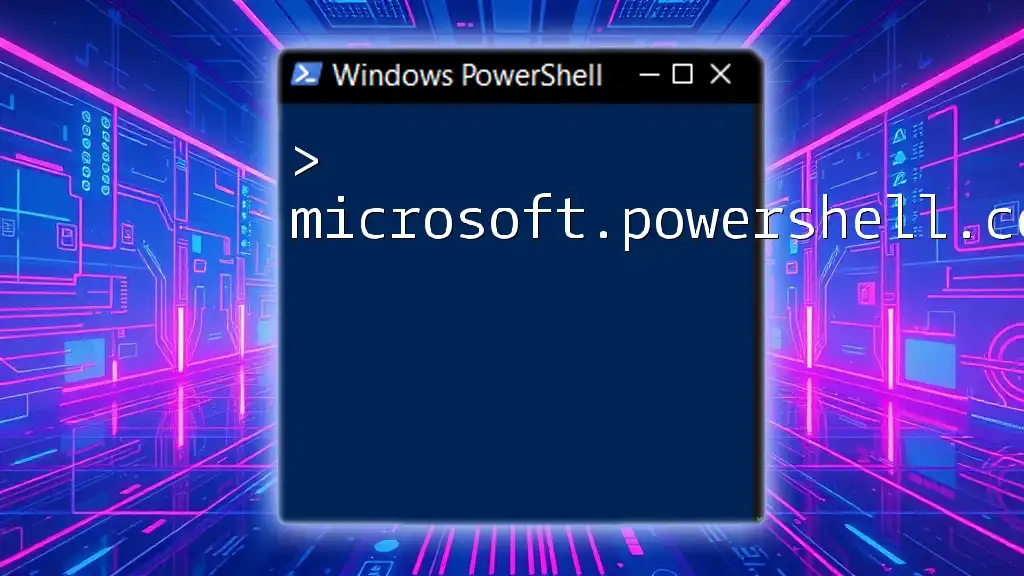
Common Pitfalls in Commenting PowerShell
Over-Commenting vs. Under-Commenting
Striking the right balance in commenting is crucial. Over-commenting, where every line is annotated, can clutter the code and dilute the significance of comments used. Conversely, under-commenting can leave readers confused about the script's functionality.
Using Comments as a Replacement for Clear Code
While comments are helpful, they should never replace the need for self-explanatory code. Instead of writing a lengthy comment to explain what the code does, aim for clarity in your variables and function names, making comments unnecessary.
Example:
# This adds 2 and 3
$sum = 2 + 3 # Not necessary if variable name is clear
In this case, the variable name `$sum` conveys the purpose of the code without requiring additional commentary.
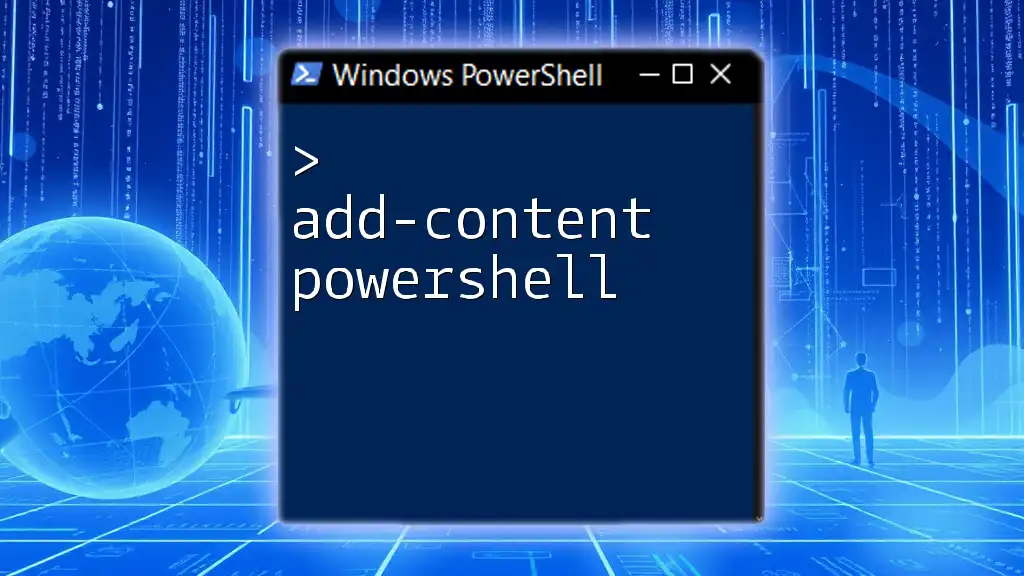
Advanced Commenting Techniques
Documenting Functions and Modules
In PowerShell, you can use comment-based help to document functions and modules systematically. This kind of commenting is crucial for usability, allowing others to understand how to use your functions immediately.
Example:
<#
.SYNOPSIS
Short description of the function
.DESCRIPTION
Detailed description of the function
.PARAMETER ParamName
Description of parameter
#>
function MyFunction {
param(
[string]$ParamName
)
}
This method of documentation not only informs readers about the function's purpose but also provides essential parameter descriptions, thereby enhancing usability and encouraging better coding practices.
Using Annotations for Version Control
Maintaining a history of changes through comments can be valuable, especially for team-based projects. Annotating significant changes, additions, or removals ensures that anyone reading the script understands its evolution.
Example of a revision history comment:
# Revision History
# 2023-10-01 - Initial creation of script
# 2023-10-15 - Added error handling
This kind of detailed commenting can facilitate better collaboration and code review processes, allowing all team members to stay informed about the developmental history of the script.
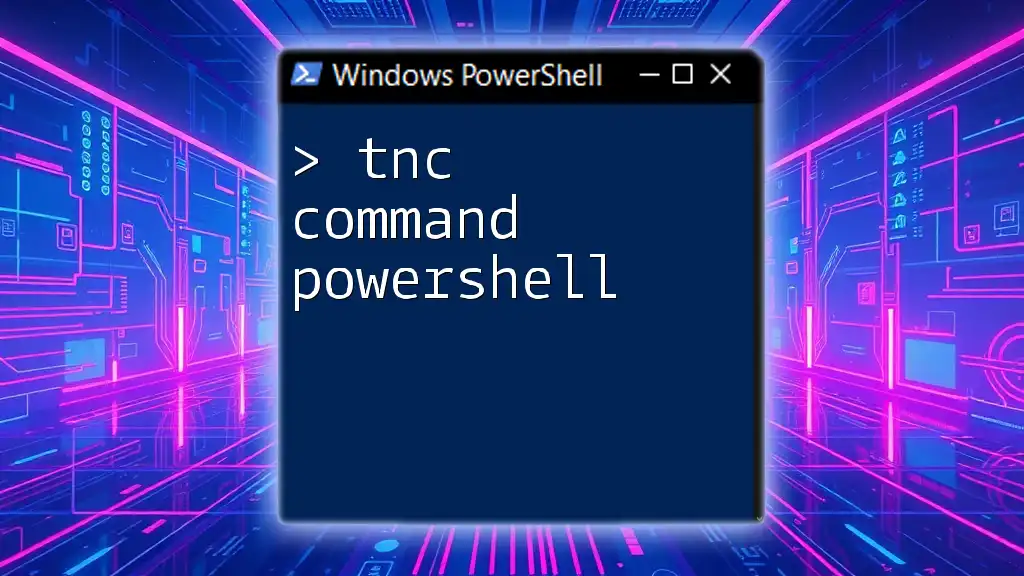
Tools and Resources for PowerShell Commenting
IDEs and Editors with Commenting Support
Using an Integrated Development Environment (IDE) or text editor tailored for PowerShell can dramatically enhance your coding efficiency. Tools like Visual Studio Code and PowerShell ISE provide commenting shortcuts, syntax highlighting, and other useful features that simplify the commenting process.
Online Resources for Further Learning
To deepen your understanding of commenting and other PowerShell practices, various resources, tutorials, and online forums can provide valuable insights. Investing time in these resources will pay off, as effective commenting is a crucial skill for any developer working with PowerShell.
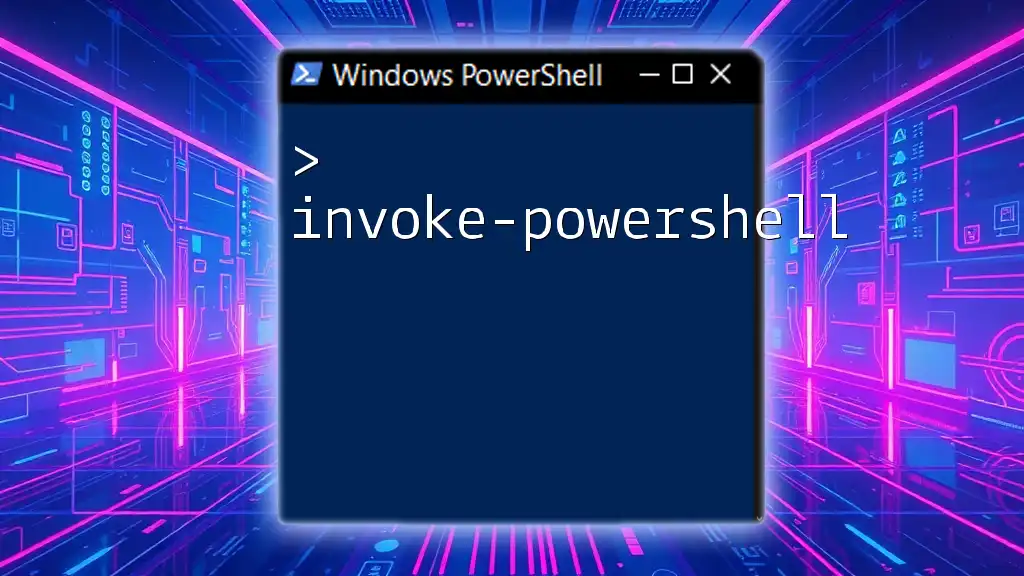
Conclusion
In summary, understanding how to comment PowerShell effectively is key to writing readable, maintainable, and user-friendly scripts. Implementing clear, relevant comments can greatly enhance the coding experience, whether for yourself or others who may work with your code in the future. By adhering to best practices in commenting and avoiding common pitfalls, you can ensure your PowerShell scripts are comprehensible and useful long after they’ve been written.
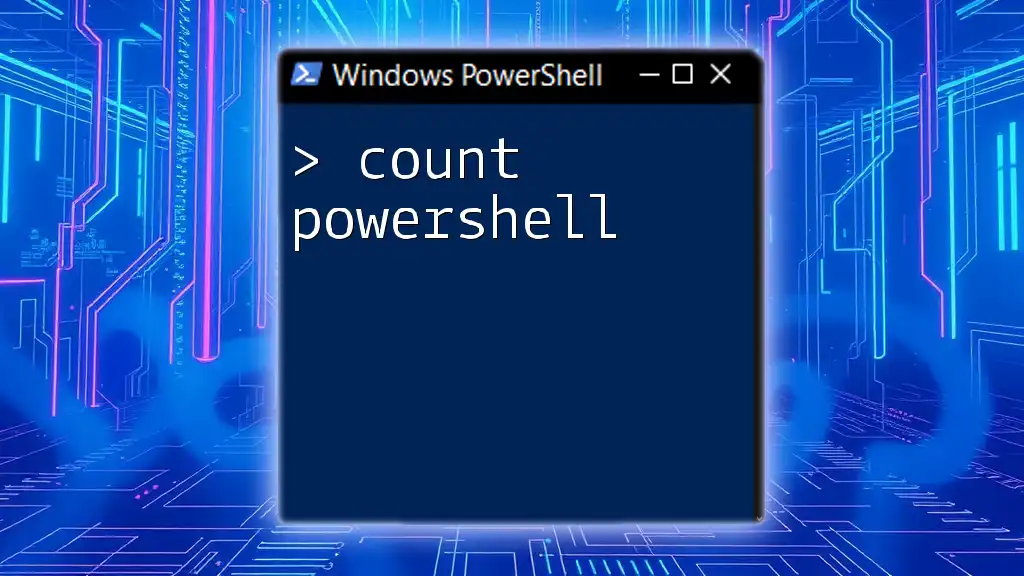
Call to Action
If you’re eager to learn more about PowerShell and elevate your scripting skills, explore the additional resources and courses offered by our company. Feel free to share your thoughts or questions in the comments below; we’d love to hear from you!