You can download a file using PowerShell and execute it by using the `Invoke-WebRequest` command followed by the `Start-Process` command for execution; here’s a quick example.
Invoke-WebRequest -Uri 'http://example.com/file.exe' -OutFile 'C:\path\to\file.exe'; Start-Process 'C:\path\to\file.exe'
Prerequisites
Before diving into how to download something then execute it using PowerShell, it's essential to have a basic understanding of PowerShell commands. Familiarity with command-line interfaces and having the right permissions is also crucial.
Ensure you have Administrator access to change execution policies if needed. This access helps you execute scripts that might enhance your productivity and automate tasks more efficiently.

Setting Up Your Environment
Opening PowerShell
To begin using PowerShell, you first need to open it. The method may vary slightly depending on your Windows version:
- For Windows 10/11: Right-click the Start button and select "Windows PowerShell" or "Windows Terminal."
- For Windows 7/8: Click the Start button, type `powershell` in the search box, and press Enter.
To confirm you are using a supported version of PowerShell, run the following command:
$PSVersionTable.PSVersion
Execution Policy
PowerShell execution policies control the ability to run scripts. Knowing about this aspect is vital before executing downloaded files.
Understanding Execution Policies: PowerShell's execution policies include:
- Restricted: No scripts can be run. This is the default setting.
- RemoteSigned: Downloaded scripts must be signed by a trusted publisher.
- Unrestricted: All scripts can be run, but you receive a warning for downloaded scripts.
To check your current execution policy, run:
Get-ExecutionPolicy
If the policy restricts you from executing scripts, temporarily change it for the current user with:
Set-ExecutionPolicy RemoteSigned -Scope CurrentUser

Step 1: Downloading Files via PowerShell
Using Invoke-WebRequest
One of the primary cmdlets used for downloading files in PowerShell is Invoke-WebRequest. This cmdlet allows you to retrieve files from the internet seamlessly.
Overview: The Invoke-WebRequest cmdlet makes it easy to fetch web content and files. Its basic syntax is:
Invoke-WebRequest -Uri "URL" -OutFile "FilePath"
Here's a concrete example of downloading a file:
Invoke-WebRequest -Uri "http://example.com/myfile.zip" -OutFile "C:\path\to\save\myfile.zip"
In this command:
- -Uri specifies the internet location of the file.
- -OutFile determines where the file will be saved on your local machine.
Using Start-BitsTransfer
Another powerful method for downloading files is Start-BitsTransfer. This cmdlet is particularly useful for larger file transfers, as it manages download progress and can resume interrupted transfers.
Example: The code snippet below demonstrates how to use it:
Start-BitsTransfer -Source "http://example.com/myfile.zip" -Destination "C:\path\to\save\myfile.zip"

Step 2: Executing the Downloaded File
After successfully downloading your file, the next step is to execute it. It’s crucial to identify the type of file you've downloaded, as running a script differs from executing an executable (.exe).
Executing PowerShell Scripts
If you've downloaded a PowerShell script (.ps1), you can run it directly from the PowerShell prompt.
Running a Script: Use the following command to execute the script:
. "C:\path\to\save\myscript.ps1"
Alternatively, you can invoke it using Start-Process:
Start-Process -FilePath "C:\path\to\save\myscript.ps1"
Executing Executable Files
For executable files (like .exe), the process is straightforward. You can run the downloaded executable by using:
Start-Process -FilePath "C:\path\to\save\myprogram.exe"
This command launches the specified executable file, allowing you to run applications or installers that you have downloaded.

Tips for Error Handling
While executing commands, you might encounter errors. Here are some common issues:
- Permission Denied: If you face permission issues, ensure you have the relevant rights to the files and directories involved.
- File Not Found: Double-check your file path and ensure that the download was successful.
To manage errors effectively, you can implement Try-Catch blocks, which help capture exceptions and provide meaningful feedback. Here’s how this might look when downloading:
try {
Invoke-WebRequest -Uri "http://example.com/myfile.zip" -OutFile "C:\path\to\save\myfile.zip"
}
catch {
Write-Host "An error occurred: $_"
}

Security Considerations
When learning how to download something then execute it using PowerShell, security should be a top priority. Always ensure that you’re downloading files from trusted sources to prevent malware or malicious scripts from running on your system.
Moreover, consider running antivirus scans on any downloaded files before execution. This practice helps you safeguard your environment from potential threats.

Conclusion
In this guide, we've explored how to download something then execute it using PowerShell. By leveraging commands like Invoke-WebRequest and Start-BitsTransfer for downloading, and utilizing effective execution commands – you can smoothly integrate these processes into your scripting tasks.
As you continue practicing, remember to handle errors appropriately and maintain a focus on security. PowerShell is a powerful scripting language that, once mastered, can greatly enhance your productivity and workflow automation.
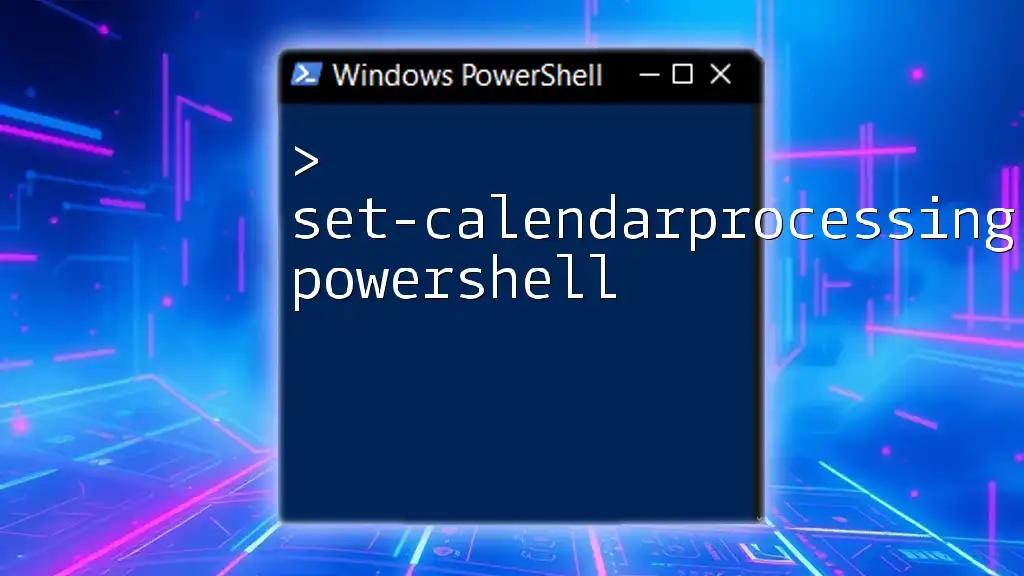
Additional Resources
To further enhance your PowerShell skills, consider exploring various online courses, reputable books, and tutorials. The official Microsoft PowerShell documentation is also an invaluable resource for more advanced commands and techniques.
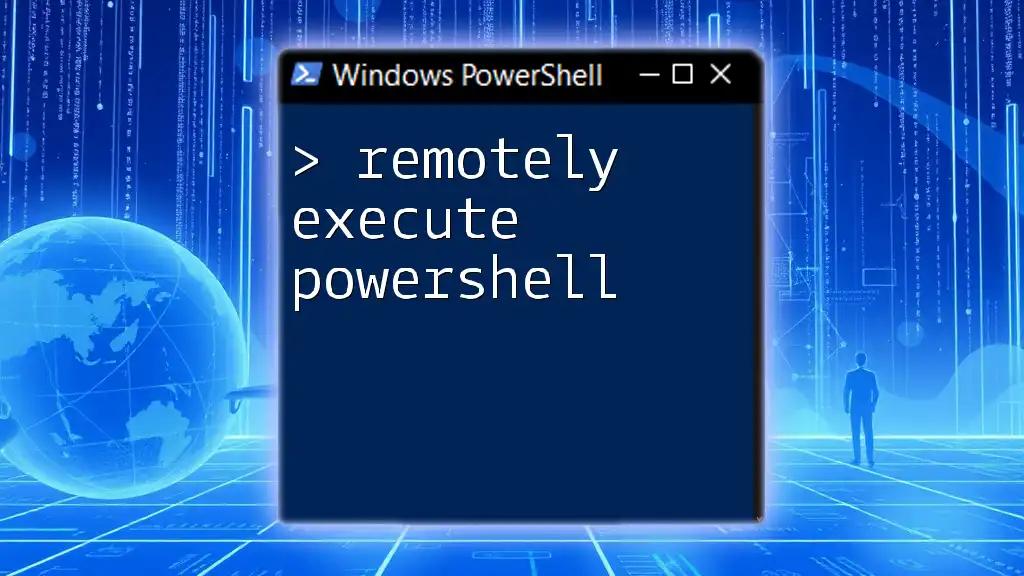
Call to Action
If you're eager to dive deeper into PowerShell or seek more resources, subscribe to our newsletter for insightful tips and guidance on mastering PowerShell commands!