To retrieve all groups a user is a member of in PowerShell, you can use the following command:
Get-ADUser -Identity "username" -Properties MemberOf | Select-Object -ExpandProperty MemberOf
Replace `"username"` with the actual username you wish to query.
Understanding User Groups in Active Directory
What are User Groups?
User groups are collections of user accounts that help manage permissions and resource access within an organization. They serve as a way to streamline user management by allowing administrators to assign permissions to groups rather than to individual users. This not only simplifies account management but also enhances security by ensuring consistent permissions across users with similar roles.
There are two main types of user groups:
-
Local Groups: These are created and managed on a specific computer and can include user accounts from that local machine as well as accounts from other trusted domains.
-
Domain Groups: These are managed within a domain and can include user accounts from that domain, enabling centralized management across multiple systems.
Why is it Important to Know User Group Memberships?
Understanding a user's group memberships is crucial for effective security management and compliance. Knowing which groups a user belongs to helps in several scenarios:
-
Security Contexts: Group memberships dictate the permissions that users have within the environment. Understanding this ensures that users have appropriate access to resources without unnecessary privileges.
-
Auditing: Regular audits of user group memberships can help identify inactive accounts, role changes, and potential security risks.
-
User Management: It streamlines the process of onboarding and offboarding users by automating permissions based on their roles.
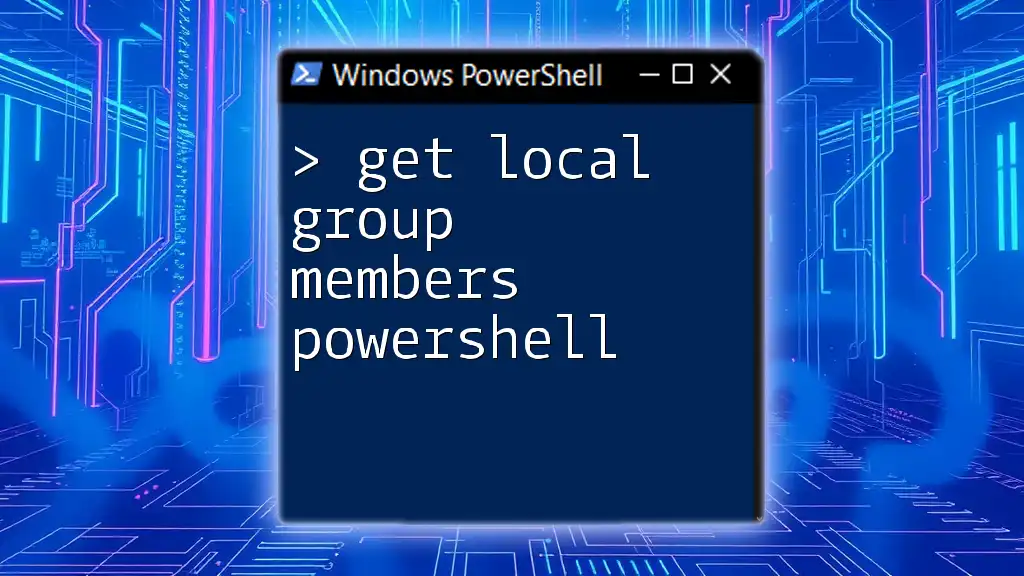
Prerequisites
To effectively get all groups a user is a member of PowerShell, you need to ensure that:
-
You have PowerShell installed, ideally version 5.0 or later, which includes enhancements and security improvements.
-
The Active Directory Module is installed on your system. You can verify its installation by running `Get-Module -ListAvailable` in PowerShell. If it’s not loaded, you can import it with:
Import-Module ActiveDirectory
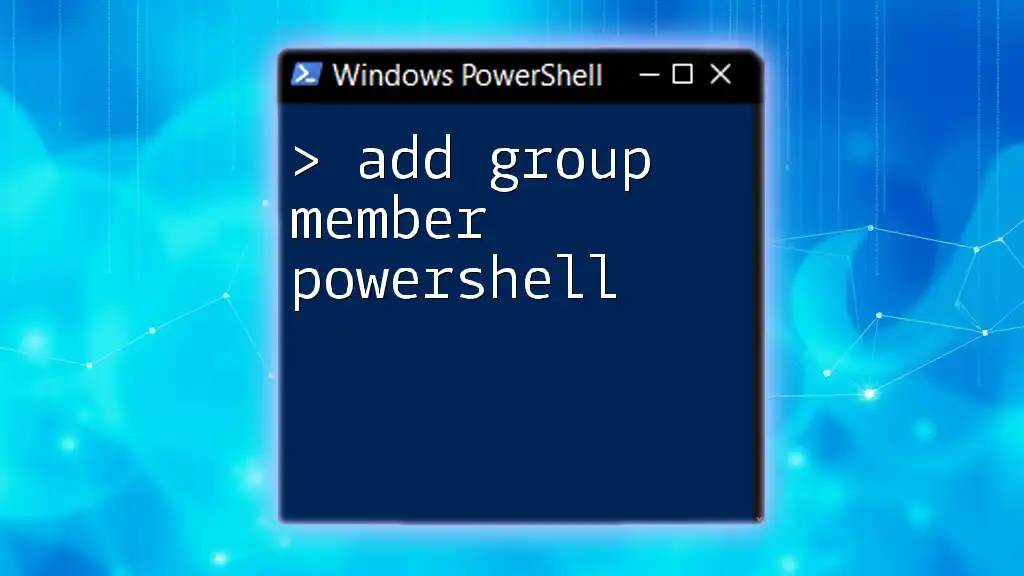
Basic Command to Get User Group Membership
Using `Get-ADUser` and `Get-ADPrincipalGroupMembership`
Overview of `Get-ADUser`
The `Get-ADUser` cmdlet retrieves user objects from Active Directory. It allows you to specify various parameters to filter your search, such as the username, so you can easily obtain the user you are interested in.
Basic Syntax of `Get-ADPrincipalGroupMembership`
The `Get-ADPrincipalGroupMembership` cmdlet is used to retrieve the groups that a specific user (or any principal like a computer or service) is a member of. The general syntax is:
Get-ADPrincipalGroupMembership -Identity <UserIdentity>
Example: Retrieve Groups for a User
To retrieve the group memberships for a specific user, you can combine these two cmdlets. Here’s a straightforward example for a user named "john.doe":
$Username = "john.doe"
$UserGroups = Get-ADUser -Identity $Username | Get-ADPrincipalGroupMembership
$UserGroups | Select-Object Name
In this snippet:
- You assign the username to the `$Username` variable.
- `Get-ADUser` retrieves the user object for "john.doe".
- `Get-ADPrincipalGroupMembership` fetches all groups associated with this user.
- Finally, `Select-Object` formats the output to show only the names of the groups.
The output will list all groups that "john.doe" is a member of, providing a clear view of his permissions.
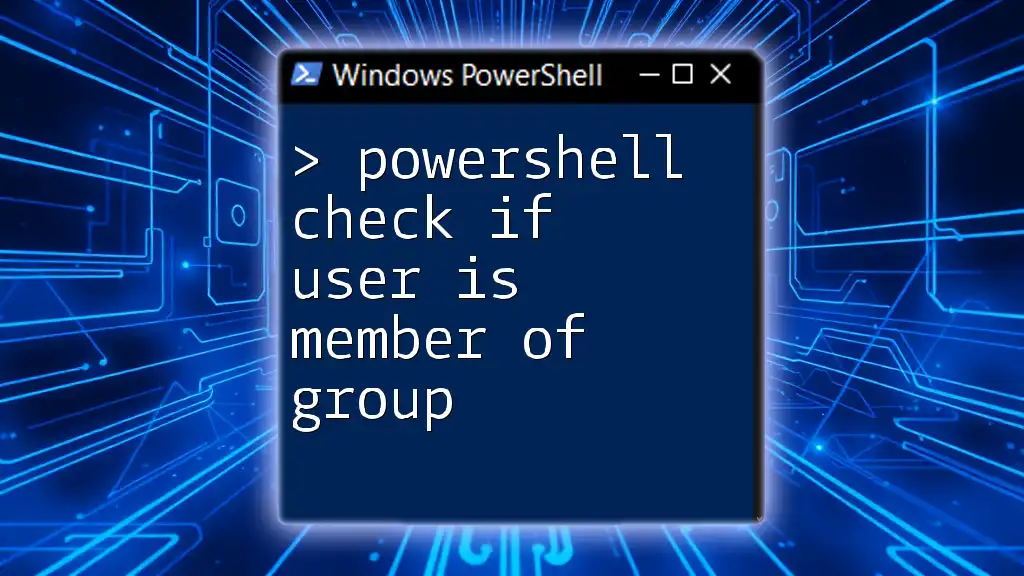
Filtering Group Results
Using `Where-Object` to Filter Groups
By default, the output may include various types of groups. You can refine the output using the `Where-Object` cmdlet, which filters objects based on specified criteria.
Example: Filter by Group Type
If you're only interested in security groups, you can modify your command this way:
$UserGroups = Get-ADUser -Identity "john.doe" | Get-ADPrincipalGroupMembership | Where-Object { $_.GroupCategory -eq "Security" }
$UserGroups | Select-Object Name
In this example, you add a condition to filter only those groups categorized as "Security".
Filtering results like this can help focus your analysis, particularly in environments with numerous group memberships.
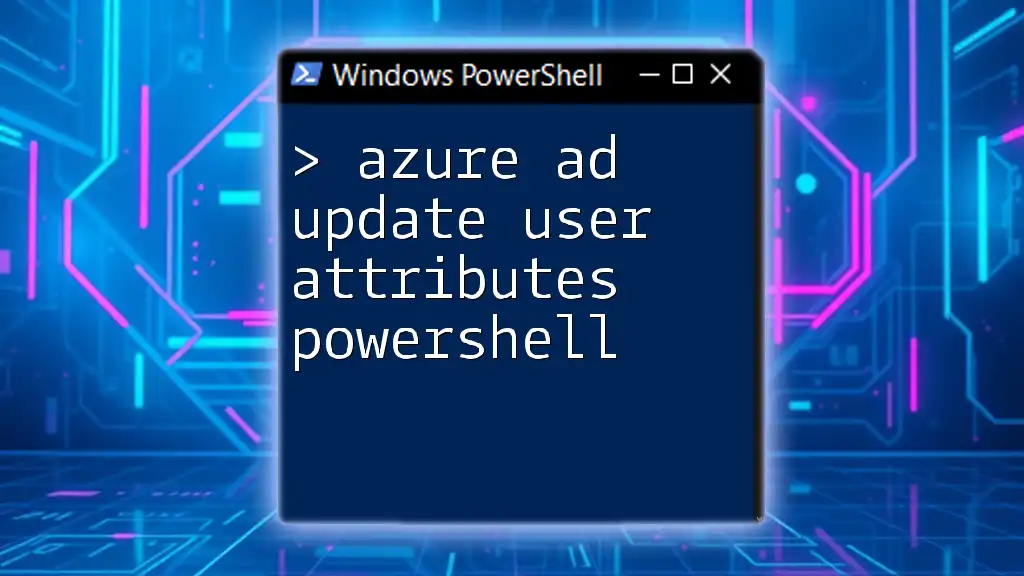
Detailed Group Information
Displaying Extended Properties of Groups
The `Get-ADPrincipalGroupMembership` cmdlet returns basic information, but you might need more detailed properties. Each group has various attributes such as `GroupScope` and `Description`.
Example: Retrieve Group Details
To obtain extended properties, modify your previous commands:
$UserGroups = Get-ADUser -Identity "john.doe" | Get-ADPrincipalGroupMembership
$UserGroups | Format-List Name, GroupScope, Description
This command provides a comprehensive overview of each group, including its name, scope (e.g., Global, Domain Local, Universal), and a brief description.
Having this detailed view helps in assessing the purpose and reach of each group associated with the user.
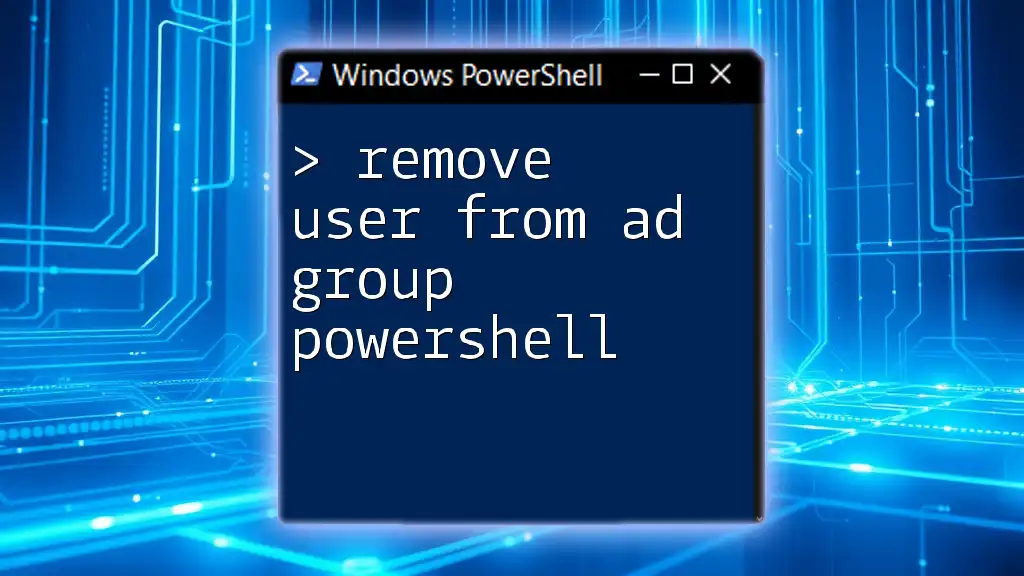
Handling Errors and Troubleshooting
Common Issues When Running the Command
When executing the command to get all groups a user is a member of, you might face some common errors:
-
User Not Found: This usually means the username was misspelled or doesn’t exist in Active Directory.
-
Permission Issues: If you do not have adequate permissions to query user details, PowerShell will return an error.
Tips for Troubleshooting
- Verify User Existence: Use the `Get-ADUser` cmdlet to ensure the user exists:
Get-ADUser -Identity "john.doe"
- Check Your Active Directory Connection: Ensure you can communicate with the domain controllers. This includes confirming your network connection and user credentials are set correctly.
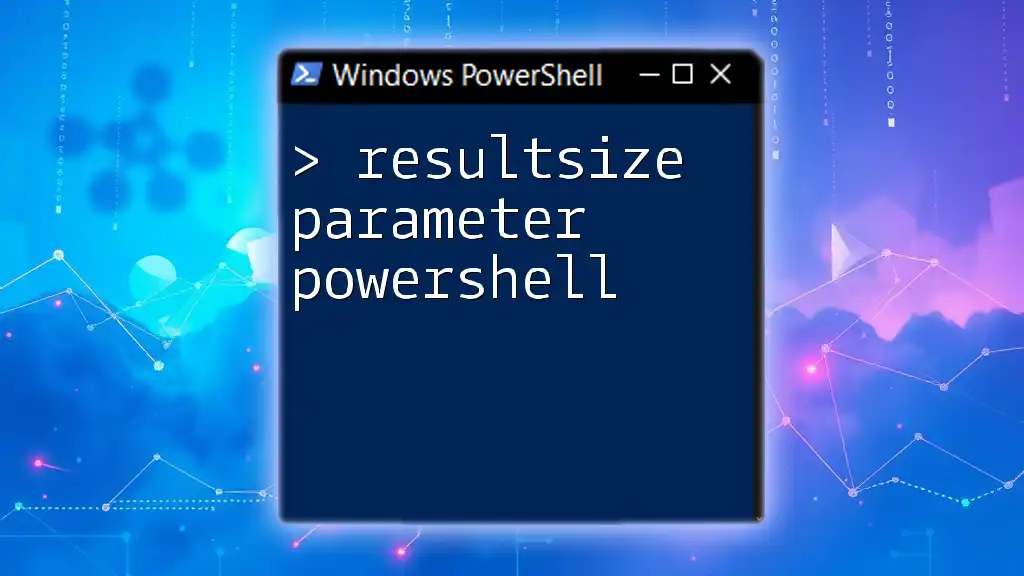
Advanced Techniques
Using PowerShell to Export Group Memberships
Sometimes, you need to share or analyze the data externally. PowerShell provides various options to export command output.
Overview of Export Options
You can export the output to formats like CSV, which is handy for reports or further analysis in spreadsheet applications.
Example: Export User Group Memberships to CSV
If you want to save "john.doe"'s group memberships to a CSV file, you can use:
$Username = "john.doe"
$UserGroups = Get-ADUser -Identity $Username | Get-ADPrincipalGroupMembership | Select-Object Name
$UserGroups | Export-Csv -Path "C:\UserGroups.csv" -NoTypeInformation
This script not only retrieves the group memberships but also writes that data to "C:\UserGroups.csv".
Using the `-NoTypeInformation` parameter prevents additional type information from cluttering your CSV, ensuring it remains clean and easy to read.
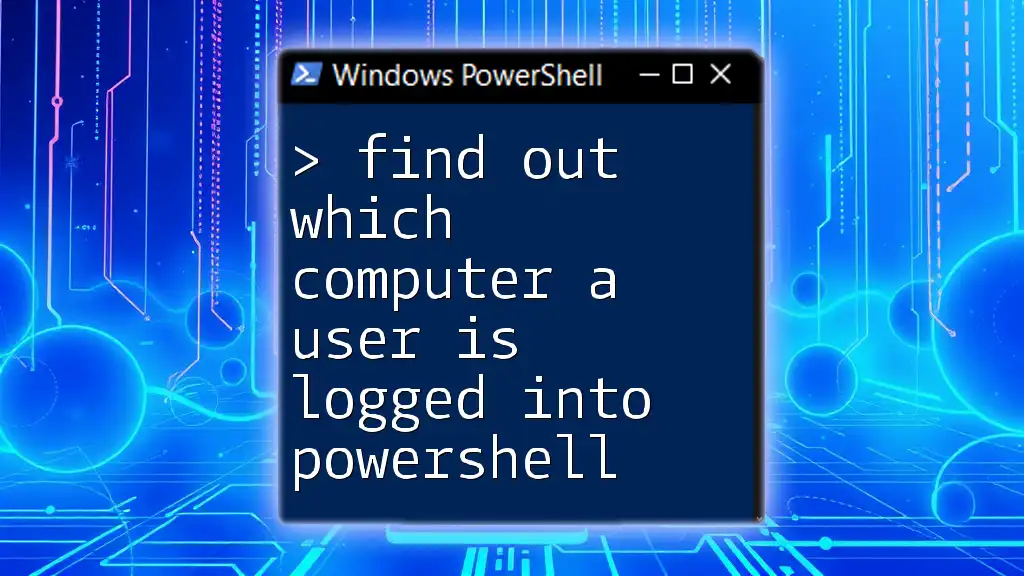
Conclusion
Understanding how to get all groups a user is a member of in PowerShell is essential for effective IT management. It empowers administrators to maintain security, streamline user management processes, and conduct audits systematically.
By leveraging the PowerShell commands discussed in this article, you will be well-equipped to handle user group memberships efficiently. Regularly using PowerShell for such tasks will enhance your productivity and improve overall administrative operations.
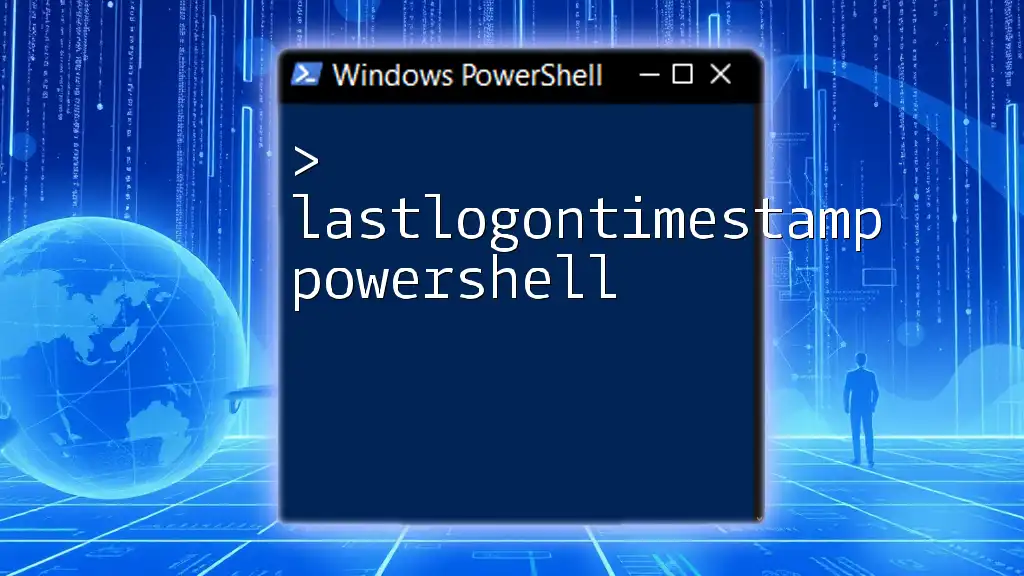
Call to Action
Share your experiences and challenges with PowerShell group management. If you're interested in deepening your PowerShell skills, consider enrolling in our tutorials for advanced techniques and best practices.