A batch file allows users to execute a PowerShell script easily by simply running the batch file, making automation and script execution more convenient.
Here's a code snippet for creating a batch file that runs a PowerShell script:
@echo off
powershell -ExecutionPolicy Bypass -File "C:\Path\To\YourScript.ps1"
Make sure to replace `"C:\Path\To\YourScript.ps1"` with the actual path to your PowerShell script.
Understanding PowerShell Scripts
What is a PowerShell Script?
A PowerShell script is essentially a text file that contains a series of PowerShell commands which automate tasks that you would normally execute manually in the Windows environment. PowerShell scripts use the .ps1 file extension and can carry out a wide array of functions, from system management to data manipulation.
Creating Your First PowerShell Script
To create a simple PowerShell script, open a text editor, like Notepad, and input the following line of code:
Write-Host "Hello, PowerShell!"
Save this file with a `.ps1` extension, for example, `HelloWorld.ps1`. To execute this script, you would typically run it directly in a PowerShell console.
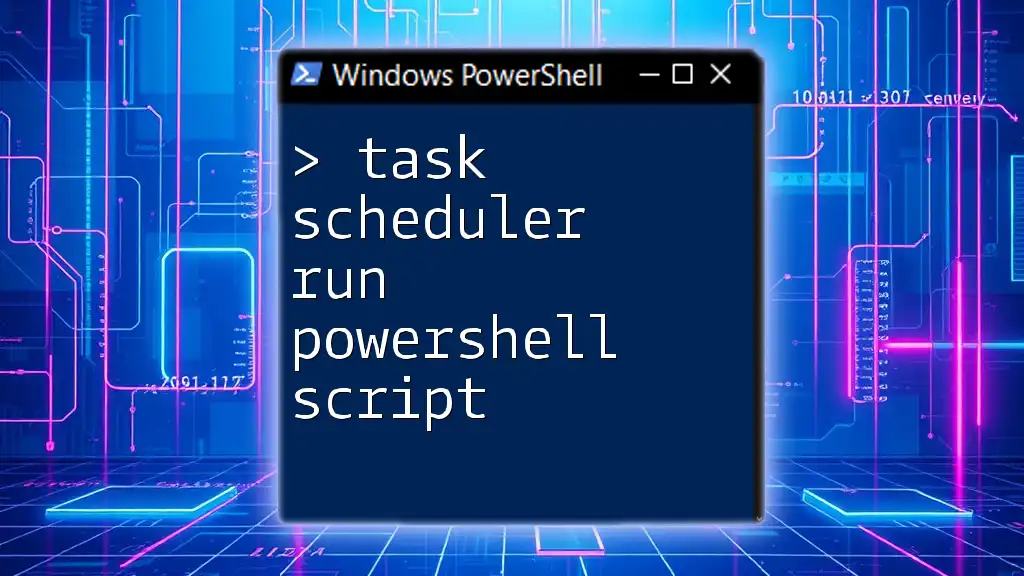
Batch Files Explained
Understanding Batch Files
Batch files are simple text files that contain a series of commands for the Windows command line. When you run a batch file, the Windows Command Prompt executes the commands within, allowing users to automate repetitive tasks efficiently. The basic structure typically starts and ends with `@echo off`, which prevents command echoing in console output.
Creating a Simple Batch File
Creating a basic batch file is straightforward. Open your text editor and write:
@echo off
echo "This is a batch file."
Save the file with a `.bat` extension, such as `SimpleBatch.bat`. When executed, this batch file will output the message within the quotes to the command line.
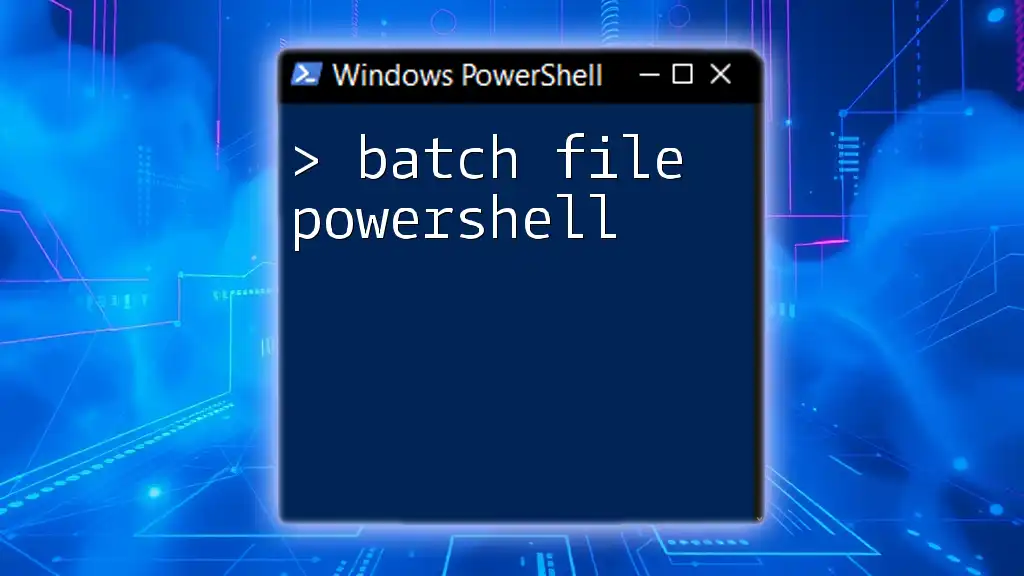
How to Run a PowerShell Script from a Batch File
Basic Method to Call PowerShell Script from Batch File
To execute a PowerShell script from a batch file, you can use the following command within your `.bat` file:
powershell -ExecutionPolicy Bypass -File "C:\path\to\your\script.ps1"
In this command:
- `powershell` launches the PowerShell environment.
- `-ExecutionPolicy Bypass` allows the script to run without being blocked by execution policies.
- `-File` specifies the path to the PowerShell script you want to execute.
Setting Execution Policies
PowerShell execution policies control the conditions under which PowerShell loads configuration files and runs scripts. The default policy may prevent some scripts from executing. Using the `Bypass` option allows for a temporary exception, making it easier to execute your scripts without being hindered by security settings.
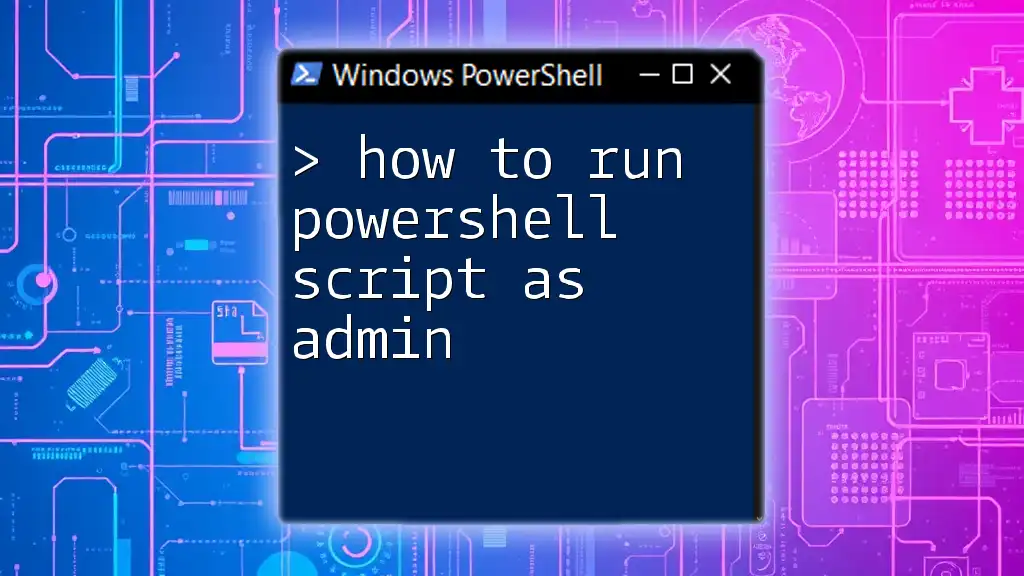
Advanced Techniques
Using Parameters in PowerShell Scripts
Sometimes, you need to pass parameters to your PowerShell scripts for them to customize their behavior. You can achieve this by adding parameters to your script and then calling them from your batch file as shown below:
param (
[string]$Param1,
[string]$Param2
)
Write-Host "Parameter 1: $Param1"
Write-Host "Parameter 2: $Param2"
Then, in your batch file, you would execute it like this:
powershell -ExecutionPolicy Bypass -File "C:\path\to\your\script.ps1" -Param1 "Value1" -Param2 "Value2"
Error Handling in Batch Files
Error handling is crucial, especially when automating tasks that include multiple steps. You can check if the PowerShell script executed correctly in your batch file. For example:
powershell -ExecutionPolicy Bypass -File "C:\path\to\your\script.ps1"
IF ERRORLEVEL 1 (
echo "PowerShell script failed to execute!"
) ELSE (
echo "PowerShell script executed successfully!"
)
This checks the error level after running the PowerShell script, providing feedback based on the script's success or failure.
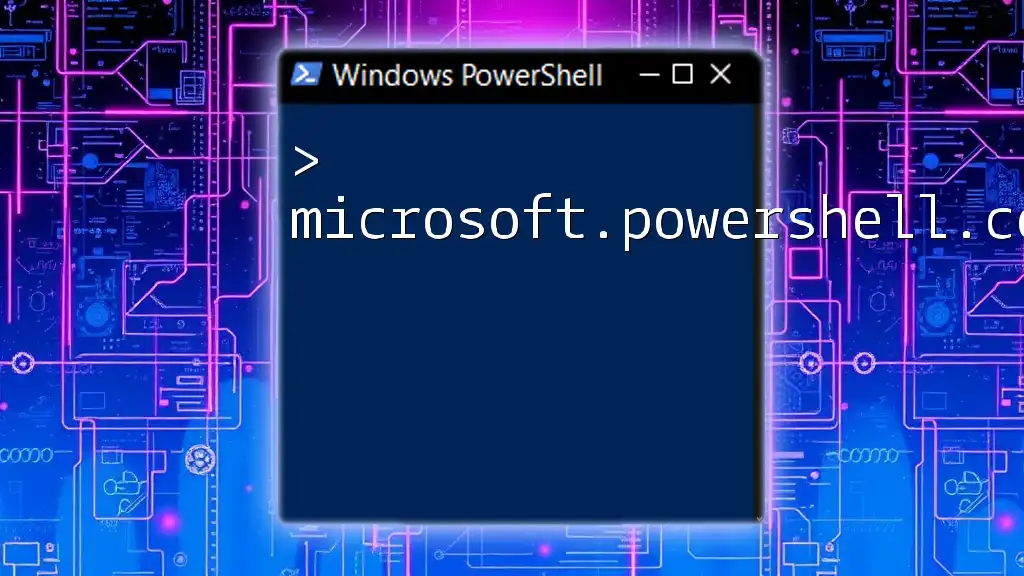
Practical Examples
Example 1: Running a PowerShell Script to Fetch System Info
Suppose you want to create a PowerShell script that gathers system information. Your `GetSystemInfo.ps1` might look like this:
Get-ComputerInfo | Out-File "C:\path\to\output.txt"
Then, you would create a batch file `RunSystemInfo.bat` to execute this script:
powershell -ExecutionPolicy Bypass -File "C:\path\to\GetSystemInfo.ps1"
echo "System information has been saved."
Example 2: Automating File Management
If you want to automate the cleanup of a specific directory, you could create a PowerShell script like this:
Remove-Item "C:\path\to\directory\*" -Force
To run this script via a batch file, create `Cleanup.bat`:
powershell -ExecutionPolicy Bypass -File "C:\path\to\Cleanup.ps1"
echo "Cleanup completed."
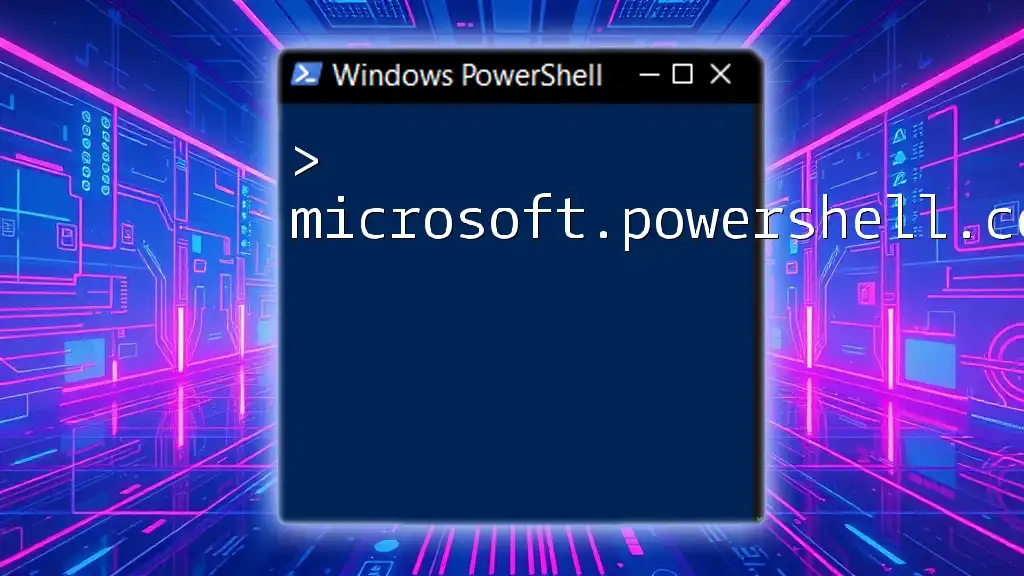
Best Practices
Using Paths Correctly
Ensure that the paths referenced in both your batch and PowerShell scripts are correct. Use quotes around file paths that contain spaces to avoid errors.
Scheduling Batch Files with Task Scheduler
You can automate the execution of your batch file using Windows Task Scheduler. This allows you to run your batch files periodically or at specific times, enabling scheduled tasks without manual intervention.
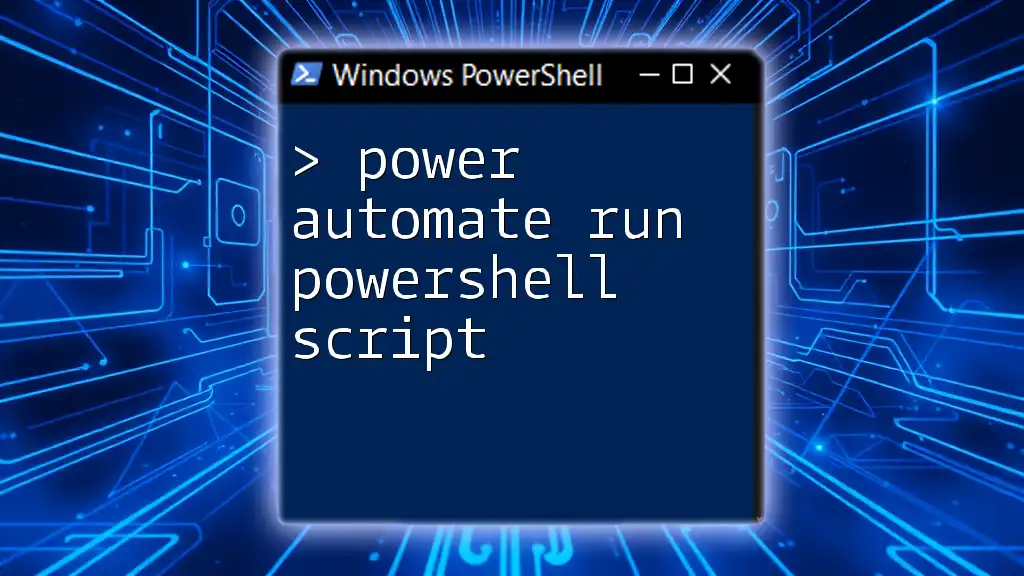
Troubleshooting Common Issues
Common Errors Encountered
When running PowerShell scripts from batch files, you may encounter issues such as:
- Execution Policy Errors: If your scripts won’t run, check your execution policy settings.
- Incorrect Path Errors: Ensure that the paths to your scripts are correct and accessible.
Debugging PowerShell Scripts
To troubleshoot script issues, consider running your PowerShell scripts in the PowerShell IDE first. This will allow you to see any immediate errors or issues before attempting to run them through your batch files.
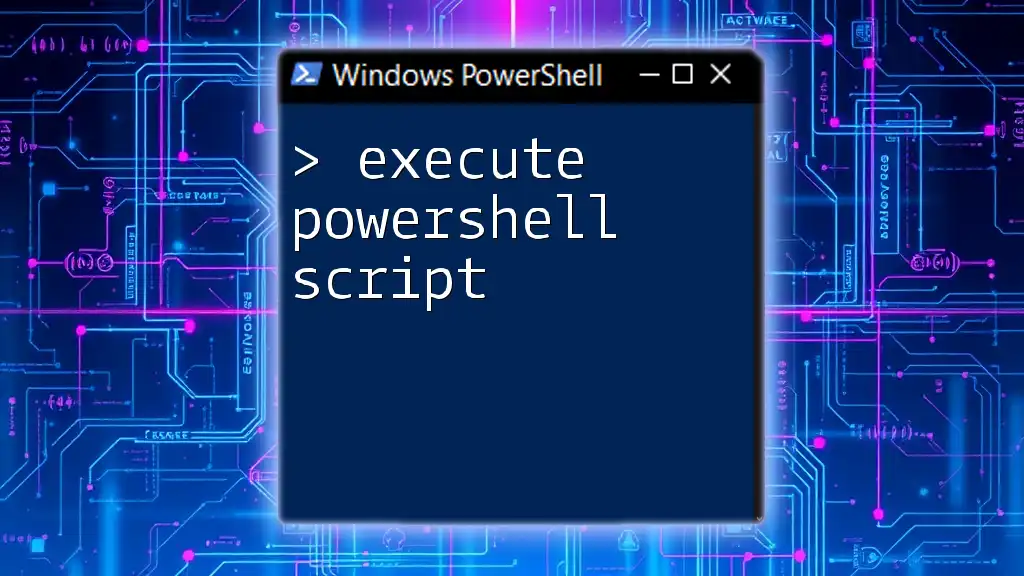
Conclusion
Utilizing a batch file to run a PowerShell script offers efficient automation that enhances productivity and reduces manual execution time. As you practice these techniques, you’ll find numerous applications that streamline your workflows and simplify complex commands into easily executable batch files.
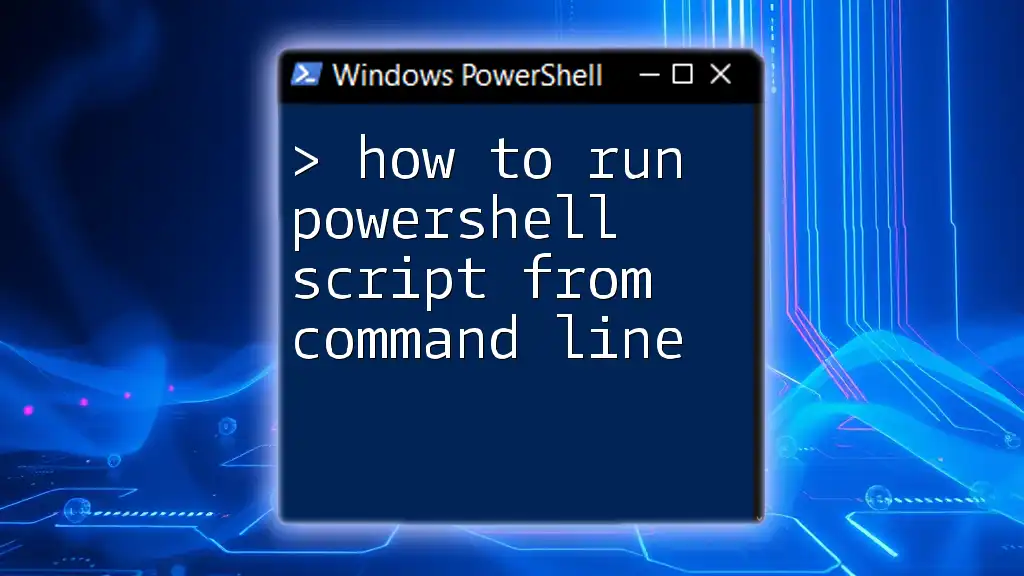
Call to Action
Feel free to share any of your scripts or inquire further if you have questions. Let’s continue exploring the powerful capabilities of PowerShell together!
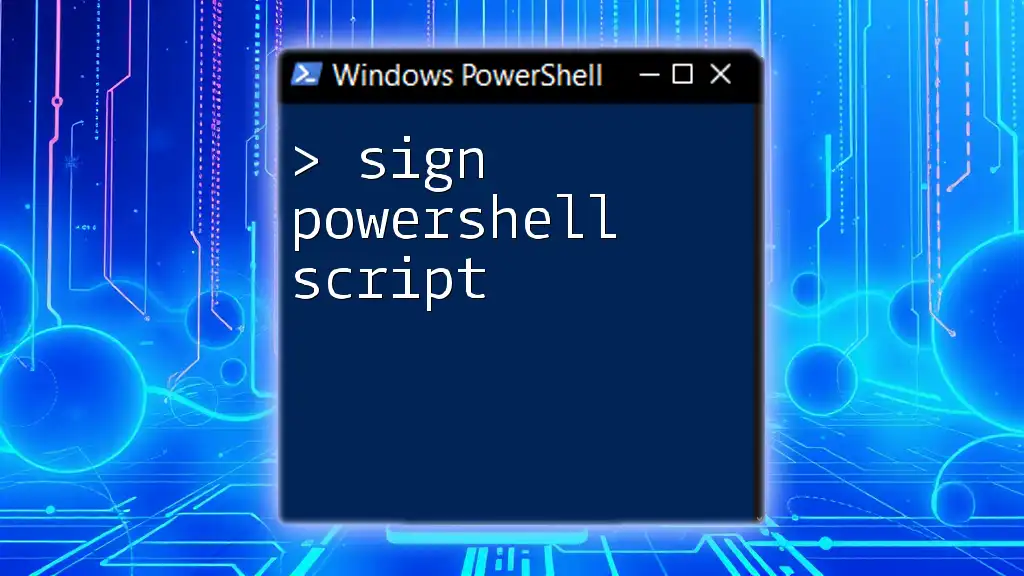
Additional Resources
For deeper insight into PowerShell scripting and batch files, check out the official PowerShell documentation and consider exploring forums and books dedicated to scripting practices.